
Example program to test AES-GCM functionality. Used for a workshop
Embed:
(wiki syntax)
Show/hide line numbers
timing.h
Go to the documentation of this file.
00001 /** 00002 * \file timing.h 00003 * 00004 * \brief Portable interface to the CPU cycle counter 00005 * 00006 * Copyright (C) 2006-2014, Brainspark B.V. 00007 * 00008 * This file is part of PolarSSL (http://www.polarssl.org) 00009 * Lead Maintainer: Paul Bakker <polarssl_maintainer at polarssl.org> 00010 * 00011 * All rights reserved. 00012 * 00013 * This program is free software; you can redistribute it and/or modify 00014 * it under the terms of the GNU General Public License as published by 00015 * the Free Software Foundation; either version 2 of the License, or 00016 * (at your option) any later version. 00017 * 00018 * This program is distributed in the hope that it will be useful, 00019 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00020 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00021 * GNU General Public License for more details. 00022 * 00023 * You should have received a copy of the GNU General Public License along 00024 * with this program; if not, write to the Free Software Foundation, Inc., 00025 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00026 */ 00027 #ifndef POLARSSL_TIMING_H 00028 #define POLARSSL_TIMING_H 00029 00030 #if !defined(POLARSSL_CONFIG_FILE) 00031 #include "config.h" 00032 #else 00033 #include POLARSSL_CONFIG_FILE 00034 #endif 00035 00036 #if !defined(POLARSSL_TIMING_ALT) 00037 // Regular implementation 00038 // 00039 00040 #ifdef __cplusplus 00041 extern "C" { 00042 #endif 00043 00044 /** 00045 * \brief timer structure 00046 */ 00047 struct hr_time 00048 { 00049 unsigned char opaque[32]; 00050 }; 00051 00052 extern volatile int alarmed; 00053 00054 /** 00055 * \brief Return the CPU cycle counter value 00056 */ 00057 unsigned long hardclock( void ); 00058 00059 /** 00060 * \brief Return the elapsed time in milliseconds 00061 * 00062 * \param val points to a timer structure 00063 * \param reset if set to 1, the timer is restarted 00064 */ 00065 unsigned long get_timer( struct hr_time *val, int reset ); 00066 00067 /** 00068 * \brief Setup an alarm clock 00069 * 00070 * \param seconds delay before the "alarmed" flag is set 00071 */ 00072 void set_alarm( int seconds ); 00073 00074 /** 00075 * \brief Sleep for a certain amount of time 00076 * 00077 * \param milliseconds delay in milliseconds 00078 */ 00079 void m_sleep( int milliseconds ); 00080 00081 #if defined(POLARSSL_SELF_TEST) 00082 /** 00083 * \brief Checkup routine 00084 * 00085 * \return 0 if successful, or 1 if a test failed 00086 */ 00087 int timing_self_test( int verbose ); 00088 #endif 00089 00090 #ifdef __cplusplus 00091 } 00092 #endif 00093 00094 #else /* POLARSSL_TIMING_ALT */ 00095 #include "timing_alt.h" 00096 #endif /* POLARSSL_TIMING_ALT */ 00097 00098 #endif /* timing.h */ 00099 00100
Generated on Tue Jul 12 2022 19:40:21 by
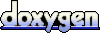