
Example program to test AES-GCM functionality. Used for a workshop
Embed:
(wiki syntax)
Show/hide line numbers
ripemd160.c
00001 /* 00002 * RIPE MD-160 implementation 00003 * 00004 * Copyright (C) 2014-2014, Brainspark B.V. 00005 * 00006 * This file is part of PolarSSL (http://www.polarssl.org) 00007 * Lead Maintainer: Paul Bakker <polarssl_maintainer at polarssl.org> 00008 * 00009 * All rights reserved. 00010 * 00011 * This program is free software; you can redistribute it and/or modify 00012 * it under the terms of the GNU General Public License as published by 00013 * the Free Software Foundation; either version 2 of the License, or 00014 * (at your option) any later version. 00015 * 00016 * This program is distributed in the hope that it will be useful, 00017 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00018 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00019 * GNU General Public License for more details. 00020 * 00021 * You should have received a copy of the GNU General Public License along 00022 * with this program; if not, write to the Free Software Foundation, Inc., 00023 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00024 */ 00025 00026 /* 00027 * The RIPEMD-160 algorithm was designed by RIPE in 1996 00028 * http://homes.esat.kuleuven.be/~bosselae/ripemd160.html 00029 * http://ehash.iaik.tugraz.at/wiki/RIPEMD-160 00030 */ 00031 00032 #if !defined(POLARSSL_CONFIG_FILE) 00033 #include "polarssl/config.h" 00034 #else 00035 #include POLARSSL_CONFIG_FILE 00036 #endif 00037 00038 #if defined(POLARSSL_RIPEMD160_C) 00039 00040 #include "polarssl/ripemd160.h" 00041 00042 #if defined(POLARSSL_FS_IO) || defined(POLARSSL_SELF_TEST) 00043 #include <stdio.h> 00044 #endif 00045 00046 #if defined(POLARSSL_SELF_TEST) 00047 #include <string.h> 00048 #endif 00049 00050 #if defined(POLARSSL_PLATFORM_C) 00051 #include "polarssl/platform.h" 00052 #else 00053 #define polarssl_printf printf 00054 #endif 00055 00056 /* 00057 * 32-bit integer manipulation macros (little endian) 00058 */ 00059 #ifndef GET_UINT32_LE 00060 #define GET_UINT32_LE(n,b,i) \ 00061 { \ 00062 (n) = ( (uint32_t) (b)[(i) ] ) \ 00063 | ( (uint32_t) (b)[(i) + 1] << 8 ) \ 00064 | ( (uint32_t) (b)[(i) + 2] << 16 ) \ 00065 | ( (uint32_t) (b)[(i) + 3] << 24 ); \ 00066 } 00067 #endif 00068 00069 #ifndef PUT_UINT32_LE 00070 #define PUT_UINT32_LE(n,b,i) \ 00071 { \ 00072 (b)[(i) ] = (unsigned char) ( (n) ); \ 00073 (b)[(i) + 1] = (unsigned char) ( (n) >> 8 ); \ 00074 (b)[(i) + 2] = (unsigned char) ( (n) >> 16 ); \ 00075 (b)[(i) + 3] = (unsigned char) ( (n) >> 24 ); \ 00076 } 00077 #endif 00078 00079 /* 00080 * RIPEMD-160 context setup 00081 */ 00082 void ripemd160_starts( ripemd160_context *ctx ) 00083 { 00084 ctx->total [0] = 0; 00085 ctx->total [1] = 0; 00086 00087 ctx->state [0] = 0x67452301; 00088 ctx->state [1] = 0xEFCDAB89; 00089 ctx->state [2] = 0x98BADCFE; 00090 ctx->state [3] = 0x10325476; 00091 ctx->state [4] = 0xC3D2E1F0; 00092 } 00093 00094 /* 00095 * Process one block 00096 */ 00097 void ripemd160_process( ripemd160_context *ctx, const unsigned char data[64] ) 00098 { 00099 uint32_t A, B, C, D, E, Ap, Bp, Cp, Dp, Ep, X[16]; 00100 00101 GET_UINT32_LE( X[ 0], data, 0 ); 00102 GET_UINT32_LE( X[ 1], data, 4 ); 00103 GET_UINT32_LE( X[ 2], data, 8 ); 00104 GET_UINT32_LE( X[ 3], data, 12 ); 00105 GET_UINT32_LE( X[ 4], data, 16 ); 00106 GET_UINT32_LE( X[ 5], data, 20 ); 00107 GET_UINT32_LE( X[ 6], data, 24 ); 00108 GET_UINT32_LE( X[ 7], data, 28 ); 00109 GET_UINT32_LE( X[ 8], data, 32 ); 00110 GET_UINT32_LE( X[ 9], data, 36 ); 00111 GET_UINT32_LE( X[10], data, 40 ); 00112 GET_UINT32_LE( X[11], data, 44 ); 00113 GET_UINT32_LE( X[12], data, 48 ); 00114 GET_UINT32_LE( X[13], data, 52 ); 00115 GET_UINT32_LE( X[14], data, 56 ); 00116 GET_UINT32_LE( X[15], data, 60 ); 00117 00118 A = Ap = ctx->state [0]; 00119 B = Bp = ctx->state [1]; 00120 C = Cp = ctx->state [2]; 00121 D = Dp = ctx->state [3]; 00122 E = Ep = ctx->state [4]; 00123 00124 #define F1( x, y, z ) ( x ^ y ^ z ) 00125 #define F2( x, y, z ) ( ( x & y ) | ( ~x & z ) ) 00126 #define F3( x, y, z ) ( ( x | ~y ) ^ z ) 00127 #define F4( x, y, z ) ( ( x & z ) | ( y & ~z ) ) 00128 #define F5( x, y, z ) ( x ^ ( y | ~z ) ) 00129 00130 #define S( x, n ) ( ( x << n ) | ( x >> (32 - n) ) ) 00131 00132 #define P( a, b, c, d, e, r, s, f, k ) \ 00133 a += f( b, c, d ) + X[r] + k; \ 00134 a = S( a, s ) + e; \ 00135 c = S( c, 10 ); 00136 00137 #define P2( a, b, c, d, e, r, s, rp, sp ) \ 00138 P( a, b, c, d, e, r, s, F, K ); \ 00139 P( a ## p, b ## p, c ## p, d ## p, e ## p, rp, sp, Fp, Kp ); 00140 00141 #define F F1 00142 #define K 0x00000000 00143 #define Fp F5 00144 #define Kp 0x50A28BE6 00145 P2( A, B, C, D, E, 0, 11, 5, 8 ); 00146 P2( E, A, B, C, D, 1, 14, 14, 9 ); 00147 P2( D, E, A, B, C, 2, 15, 7, 9 ); 00148 P2( C, D, E, A, B, 3, 12, 0, 11 ); 00149 P2( B, C, D, E, A, 4, 5, 9, 13 ); 00150 P2( A, B, C, D, E, 5, 8, 2, 15 ); 00151 P2( E, A, B, C, D, 6, 7, 11, 15 ); 00152 P2( D, E, A, B, C, 7, 9, 4, 5 ); 00153 P2( C, D, E, A, B, 8, 11, 13, 7 ); 00154 P2( B, C, D, E, A, 9, 13, 6, 7 ); 00155 P2( A, B, C, D, E, 10, 14, 15, 8 ); 00156 P2( E, A, B, C, D, 11, 15, 8, 11 ); 00157 P2( D, E, A, B, C, 12, 6, 1, 14 ); 00158 P2( C, D, E, A, B, 13, 7, 10, 14 ); 00159 P2( B, C, D, E, A, 14, 9, 3, 12 ); 00160 P2( A, B, C, D, E, 15, 8, 12, 6 ); 00161 #undef F 00162 #undef K 00163 #undef Fp 00164 #undef Kp 00165 00166 #define F F2 00167 #define K 0x5A827999 00168 #define Fp F4 00169 #define Kp 0x5C4DD124 00170 P2( E, A, B, C, D, 7, 7, 6, 9 ); 00171 P2( D, E, A, B, C, 4, 6, 11, 13 ); 00172 P2( C, D, E, A, B, 13, 8, 3, 15 ); 00173 P2( B, C, D, E, A, 1, 13, 7, 7 ); 00174 P2( A, B, C, D, E, 10, 11, 0, 12 ); 00175 P2( E, A, B, C, D, 6, 9, 13, 8 ); 00176 P2( D, E, A, B, C, 15, 7, 5, 9 ); 00177 P2( C, D, E, A, B, 3, 15, 10, 11 ); 00178 P2( B, C, D, E, A, 12, 7, 14, 7 ); 00179 P2( A, B, C, D, E, 0, 12, 15, 7 ); 00180 P2( E, A, B, C, D, 9, 15, 8, 12 ); 00181 P2( D, E, A, B, C, 5, 9, 12, 7 ); 00182 P2( C, D, E, A, B, 2, 11, 4, 6 ); 00183 P2( B, C, D, E, A, 14, 7, 9, 15 ); 00184 P2( A, B, C, D, E, 11, 13, 1, 13 ); 00185 P2( E, A, B, C, D, 8, 12, 2, 11 ); 00186 #undef F 00187 #undef K 00188 #undef Fp 00189 #undef Kp 00190 00191 #define F F3 00192 #define K 0x6ED9EBA1 00193 #define Fp F3 00194 #define Kp 0x6D703EF3 00195 P2( D, E, A, B, C, 3, 11, 15, 9 ); 00196 P2( C, D, E, A, B, 10, 13, 5, 7 ); 00197 P2( B, C, D, E, A, 14, 6, 1, 15 ); 00198 P2( A, B, C, D, E, 4, 7, 3, 11 ); 00199 P2( E, A, B, C, D, 9, 14, 7, 8 ); 00200 P2( D, E, A, B, C, 15, 9, 14, 6 ); 00201 P2( C, D, E, A, B, 8, 13, 6, 6 ); 00202 P2( B, C, D, E, A, 1, 15, 9, 14 ); 00203 P2( A, B, C, D, E, 2, 14, 11, 12 ); 00204 P2( E, A, B, C, D, 7, 8, 8, 13 ); 00205 P2( D, E, A, B, C, 0, 13, 12, 5 ); 00206 P2( C, D, E, A, B, 6, 6, 2, 14 ); 00207 P2( B, C, D, E, A, 13, 5, 10, 13 ); 00208 P2( A, B, C, D, E, 11, 12, 0, 13 ); 00209 P2( E, A, B, C, D, 5, 7, 4, 7 ); 00210 P2( D, E, A, B, C, 12, 5, 13, 5 ); 00211 #undef F 00212 #undef K 00213 #undef Fp 00214 #undef Kp 00215 00216 #define F F4 00217 #define K 0x8F1BBCDC 00218 #define Fp F2 00219 #define Kp 0x7A6D76E9 00220 P2( C, D, E, A, B, 1, 11, 8, 15 ); 00221 P2( B, C, D, E, A, 9, 12, 6, 5 ); 00222 P2( A, B, C, D, E, 11, 14, 4, 8 ); 00223 P2( E, A, B, C, D, 10, 15, 1, 11 ); 00224 P2( D, E, A, B, C, 0, 14, 3, 14 ); 00225 P2( C, D, E, A, B, 8, 15, 11, 14 ); 00226 P2( B, C, D, E, A, 12, 9, 15, 6 ); 00227 P2( A, B, C, D, E, 4, 8, 0, 14 ); 00228 P2( E, A, B, C, D, 13, 9, 5, 6 ); 00229 P2( D, E, A, B, C, 3, 14, 12, 9 ); 00230 P2( C, D, E, A, B, 7, 5, 2, 12 ); 00231 P2( B, C, D, E, A, 15, 6, 13, 9 ); 00232 P2( A, B, C, D, E, 14, 8, 9, 12 ); 00233 P2( E, A, B, C, D, 5, 6, 7, 5 ); 00234 P2( D, E, A, B, C, 6, 5, 10, 15 ); 00235 P2( C, D, E, A, B, 2, 12, 14, 8 ); 00236 #undef F 00237 #undef K 00238 #undef Fp 00239 #undef Kp 00240 00241 #define F F5 00242 #define K 0xA953FD4E 00243 #define Fp F1 00244 #define Kp 0x00000000 00245 P2( B, C, D, E, A, 4, 9, 12, 8 ); 00246 P2( A, B, C, D, E, 0, 15, 15, 5 ); 00247 P2( E, A, B, C, D, 5, 5, 10, 12 ); 00248 P2( D, E, A, B, C, 9, 11, 4, 9 ); 00249 P2( C, D, E, A, B, 7, 6, 1, 12 ); 00250 P2( B, C, D, E, A, 12, 8, 5, 5 ); 00251 P2( A, B, C, D, E, 2, 13, 8, 14 ); 00252 P2( E, A, B, C, D, 10, 12, 7, 6 ); 00253 P2( D, E, A, B, C, 14, 5, 6, 8 ); 00254 P2( C, D, E, A, B, 1, 12, 2, 13 ); 00255 P2( B, C, D, E, A, 3, 13, 13, 6 ); 00256 P2( A, B, C, D, E, 8, 14, 14, 5 ); 00257 P2( E, A, B, C, D, 11, 11, 0, 15 ); 00258 P2( D, E, A, B, C, 6, 8, 3, 13 ); 00259 P2( C, D, E, A, B, 15, 5, 9, 11 ); 00260 P2( B, C, D, E, A, 13, 6, 11, 11 ); 00261 #undef F 00262 #undef K 00263 #undef Fp 00264 #undef Kp 00265 00266 C = ctx->state [1] + C + Dp; 00267 ctx->state [1] = ctx->state [2] + D + Ep; 00268 ctx->state [2] = ctx->state [3] + E + Ap; 00269 ctx->state [3] = ctx->state [4] + A + Bp; 00270 ctx->state [4] = ctx->state [0] + B + Cp; 00271 ctx->state [0] = C; 00272 } 00273 00274 /* 00275 * RIPEMD-160 process buffer 00276 */ 00277 void ripemd160_update( ripemd160_context *ctx, 00278 const unsigned char *input, size_t ilen ) 00279 { 00280 size_t fill; 00281 uint32_t left; 00282 00283 if( ilen <= 0 ) 00284 return; 00285 00286 left = ctx->total [0] & 0x3F; 00287 fill = 64 - left; 00288 00289 ctx->total [0] += (uint32_t) ilen; 00290 ctx->total [0] &= 0xFFFFFFFF; 00291 00292 if( ctx->total [0] < (uint32_t) ilen ) 00293 ctx->total [1]++; 00294 00295 if( left && ilen >= fill ) 00296 { 00297 memcpy( (void *) (ctx->buffer + left), input, fill ); 00298 ripemd160_process( ctx, ctx->buffer ); 00299 input += fill; 00300 ilen -= fill; 00301 left = 0; 00302 } 00303 00304 while( ilen >= 64 ) 00305 { 00306 ripemd160_process( ctx, input ); 00307 input += 64; 00308 ilen -= 64; 00309 } 00310 00311 if( ilen > 0 ) 00312 { 00313 memcpy( (void *) (ctx->buffer + left), input, ilen ); 00314 } 00315 } 00316 00317 static const unsigned char ripemd160_padding[64] = 00318 { 00319 0x80, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 00320 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 00321 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 00322 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 00323 }; 00324 00325 /* 00326 * RIPEMD-160 final digest 00327 */ 00328 void ripemd160_finish( ripemd160_context *ctx, unsigned char output[20] ) 00329 { 00330 uint32_t last, padn; 00331 uint32_t high, low; 00332 unsigned char msglen[8]; 00333 00334 high = ( ctx->total [0] >> 29 ) 00335 | ( ctx->total [1] << 3 ); 00336 low = ( ctx->total [0] << 3 ); 00337 00338 PUT_UINT32_LE( low, msglen, 0 ); 00339 PUT_UINT32_LE( high, msglen, 4 ); 00340 00341 last = ctx->total [0] & 0x3F; 00342 padn = ( last < 56 ) ? ( 56 - last ) : ( 120 - last ); 00343 00344 ripemd160_update( ctx, ripemd160_padding, padn ); 00345 ripemd160_update( ctx, msglen, 8 ); 00346 00347 PUT_UINT32_LE( ctx->state [0], output, 0 ); 00348 PUT_UINT32_LE( ctx->state [1], output, 4 ); 00349 PUT_UINT32_LE( ctx->state [2], output, 8 ); 00350 PUT_UINT32_LE( ctx->state [3], output, 12 ); 00351 PUT_UINT32_LE( ctx->state [4], output, 16 ); 00352 } 00353 00354 /* 00355 * output = RIPEMD-160( input buffer ) 00356 */ 00357 void ripemd160( const unsigned char *input, size_t ilen, 00358 unsigned char output[20] ) 00359 { 00360 ripemd160_context ctx; 00361 00362 ripemd160_starts( &ctx ); 00363 ripemd160_update( &ctx, input, ilen ); 00364 ripemd160_finish( &ctx, output ); 00365 00366 memset( &ctx, 0, sizeof( ripemd160_context ) ); 00367 } 00368 00369 #if defined(POLARSSL_FS_IO) 00370 /* 00371 * output = RIPEMD-160( file contents ) 00372 */ 00373 int ripemd160_file( const char *path, unsigned char output[20] ) 00374 { 00375 FILE *f; 00376 size_t n; 00377 ripemd160_context ctx; 00378 unsigned char buf[1024]; 00379 00380 if( ( f = fopen( path, "rb" ) ) == NULL ) 00381 return( POLARSSL_ERR_RIPEMD160_FILE_IO_ERROR ); 00382 00383 ripemd160_starts( &ctx ); 00384 00385 while( ( n = fread( buf, 1, sizeof( buf ), f ) ) > 0 ) 00386 ripemd160_update( &ctx, buf, n ); 00387 00388 ripemd160_finish( &ctx, output ); 00389 00390 memset( &ctx, 0, sizeof( ripemd160_context ) ); 00391 00392 if( ferror( f ) != 0 ) 00393 { 00394 fclose( f ); 00395 return( POLARSSL_ERR_RIPEMD160_FILE_IO_ERROR ); 00396 } 00397 00398 fclose( f ); 00399 return( 0 ); 00400 } 00401 #endif /* POLARSSL_FS_IO */ 00402 00403 /* 00404 * RIPEMD-160 HMAC context setup 00405 */ 00406 void ripemd160_hmac_starts( ripemd160_context *ctx, 00407 const unsigned char *key, size_t keylen ) 00408 { 00409 size_t i; 00410 unsigned char sum[20]; 00411 00412 if( keylen > 64 ) 00413 { 00414 ripemd160( key, keylen, sum ); 00415 keylen = 20; 00416 key = sum; 00417 } 00418 00419 memset( ctx->ipad , 0x36, 64 ); 00420 memset( ctx->opad , 0x5C, 64 ); 00421 00422 for( i = 0; i < keylen; i++ ) 00423 { 00424 ctx->ipad [i] = (unsigned char)( ctx->ipad [i] ^ key[i] ); 00425 ctx->opad [i] = (unsigned char)( ctx->opad [i] ^ key[i] ); 00426 } 00427 00428 ripemd160_starts( ctx ); 00429 ripemd160_update( ctx, ctx->ipad , 64 ); 00430 00431 memset( sum, 0, sizeof( sum ) ); 00432 } 00433 00434 /* 00435 * RIPEMD-160 HMAC process buffer 00436 */ 00437 void ripemd160_hmac_update( ripemd160_context *ctx, 00438 const unsigned char *input, size_t ilen ) 00439 { 00440 ripemd160_update( ctx, input, ilen ); 00441 } 00442 00443 /* 00444 * RIPEMD-160 HMAC final digest 00445 */ 00446 void ripemd160_hmac_finish( ripemd160_context *ctx, unsigned char output[20] ) 00447 { 00448 unsigned char tmpbuf[20]; 00449 00450 ripemd160_finish( ctx, tmpbuf ); 00451 ripemd160_starts( ctx ); 00452 ripemd160_update( ctx, ctx->opad , 64 ); 00453 ripemd160_update( ctx, tmpbuf, 20 ); 00454 ripemd160_finish( ctx, output ); 00455 00456 memset( tmpbuf, 0, sizeof( tmpbuf ) ); 00457 } 00458 00459 /* 00460 * RIPEMD-160 HMAC context reset 00461 */ 00462 void ripemd160_hmac_reset( ripemd160_context *ctx ) 00463 { 00464 ripemd160_starts( ctx ); 00465 ripemd160_update( ctx, ctx->ipad , 64 ); 00466 } 00467 00468 /* 00469 * output = HMAC-RIPEMD-160( hmac key, input buffer ) 00470 */ 00471 void ripemd160_hmac( const unsigned char *key, size_t keylen, 00472 const unsigned char *input, size_t ilen, 00473 unsigned char output[20] ) 00474 { 00475 ripemd160_context ctx; 00476 00477 ripemd160_hmac_starts( &ctx, key, keylen ); 00478 ripemd160_hmac_update( &ctx, input, ilen ); 00479 ripemd160_hmac_finish( &ctx, output ); 00480 00481 memset( &ctx, 0, sizeof( ripemd160_context ) ); 00482 } 00483 00484 00485 #if defined(POLARSSL_SELF_TEST) 00486 /* 00487 * Test vectors from the RIPEMD-160 paper and 00488 * http://homes.esat.kuleuven.be/~bosselae/ripemd160.html#HMAC 00489 */ 00490 #define TESTS 8 00491 #define KEYS 2 00492 static const char *ripemd160_test_input[TESTS] = 00493 { 00494 "", 00495 "a", 00496 "abc", 00497 "message digest", 00498 "abcdefghijklmnopqrstuvwxyz", 00499 "abcdbcdecdefdefgefghfghighijhijkijkljklmklmnlmnomnopnopq", 00500 "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789", 00501 "1234567890123456789012345678901234567890" 00502 "1234567890123456789012345678901234567890", 00503 }; 00504 00505 static const unsigned char ripemd160_test_md[TESTS][20] = 00506 { 00507 { 0x9c, 0x11, 0x85, 0xa5, 0xc5, 0xe9, 0xfc, 0x54, 0x61, 0x28, 00508 0x08, 0x97, 0x7e, 0xe8, 0xf5, 0x48, 0xb2, 0x25, 0x8d, 0x31 }, 00509 { 0x0b, 0xdc, 0x9d, 0x2d, 0x25, 0x6b, 0x3e, 0xe9, 0xda, 0xae, 00510 0x34, 0x7b, 0xe6, 0xf4, 0xdc, 0x83, 0x5a, 0x46, 0x7f, 0xfe }, 00511 { 0x8e, 0xb2, 0x08, 0xf7, 0xe0, 0x5d, 0x98, 0x7a, 0x9b, 0x04, 00512 0x4a, 0x8e, 0x98, 0xc6, 0xb0, 0x87, 0xf1, 0x5a, 0x0b, 0xfc }, 00513 { 0x5d, 0x06, 0x89, 0xef, 0x49, 0xd2, 0xfa, 0xe5, 0x72, 0xb8, 00514 0x81, 0xb1, 0x23, 0xa8, 0x5f, 0xfa, 0x21, 0x59, 0x5f, 0x36 }, 00515 { 0xf7, 0x1c, 0x27, 0x10, 0x9c, 0x69, 0x2c, 0x1b, 0x56, 0xbb, 00516 0xdc, 0xeb, 0x5b, 0x9d, 0x28, 0x65, 0xb3, 0x70, 0x8d, 0xbc }, 00517 { 0x12, 0xa0, 0x53, 0x38, 0x4a, 0x9c, 0x0c, 0x88, 0xe4, 0x05, 00518 0xa0, 0x6c, 0x27, 0xdc, 0xf4, 0x9a, 0xda, 0x62, 0xeb, 0x2b }, 00519 { 0xb0, 0xe2, 0x0b, 0x6e, 0x31, 0x16, 0x64, 0x02, 0x86, 0xed, 00520 0x3a, 0x87, 0xa5, 0x71, 0x30, 0x79, 0xb2, 0x1f, 0x51, 0x89 }, 00521 { 0x9b, 0x75, 0x2e, 0x45, 0x57, 0x3d, 0x4b, 0x39, 0xf4, 0xdb, 00522 0xd3, 0x32, 0x3c, 0xab, 0x82, 0xbf, 0x63, 0x32, 0x6b, 0xfb }, 00523 }; 00524 00525 static const unsigned char ripemd160_test_hmac[KEYS][TESTS][20] = 00526 { 00527 { 00528 { 0xcf, 0x38, 0x76, 0x77, 0xbf, 0xda, 0x84, 0x83, 0xe6, 0x3b, 00529 0x57, 0xe0, 0x6c, 0x3b, 0x5e, 0xcd, 0x8b, 0x7f, 0xc0, 0x55 }, 00530 { 0x0d, 0x35, 0x1d, 0x71, 0xb7, 0x8e, 0x36, 0xdb, 0xb7, 0x39, 00531 0x1c, 0x81, 0x0a, 0x0d, 0x2b, 0x62, 0x40, 0xdd, 0xba, 0xfc }, 00532 { 0xf7, 0xef, 0x28, 0x8c, 0xb1, 0xbb, 0xcc, 0x61, 0x60, 0xd7, 00533 0x65, 0x07, 0xe0, 0xa3, 0xbb, 0xf7, 0x12, 0xfb, 0x67, 0xd6 }, 00534 { 0xf8, 0x36, 0x62, 0xcc, 0x8d, 0x33, 0x9c, 0x22, 0x7e, 0x60, 00535 0x0f, 0xcd, 0x63, 0x6c, 0x57, 0xd2, 0x57, 0x1b, 0x1c, 0x34 }, 00536 { 0x84, 0x3d, 0x1c, 0x4e, 0xb8, 0x80, 0xac, 0x8a, 0xc0, 0xc9, 00537 0xc9, 0x56, 0x96, 0x50, 0x79, 0x57, 0xd0, 0x15, 0x5d, 0xdb }, 00538 { 0x60, 0xf5, 0xef, 0x19, 0x8a, 0x2d, 0xd5, 0x74, 0x55, 0x45, 00539 0xc1, 0xf0, 0xc4, 0x7a, 0xa3, 0xfb, 0x57, 0x76, 0xf8, 0x81 }, 00540 { 0xe4, 0x9c, 0x13, 0x6a, 0x9e, 0x56, 0x27, 0xe0, 0x68, 0x1b, 00541 0x80, 0x8a, 0x3b, 0x97, 0xe6, 0xa6, 0xe6, 0x61, 0xae, 0x79 }, 00542 { 0x31, 0xbe, 0x3c, 0xc9, 0x8c, 0xee, 0x37, 0xb7, 0x9b, 0x06, 00543 0x19, 0xe3, 0xe1, 0xc2, 0xbe, 0x4f, 0x1a, 0xa5, 0x6e, 0x6c }, 00544 }, 00545 { 00546 { 0xfe, 0x69, 0xa6, 0x6c, 0x74, 0x23, 0xee, 0xa9, 0xc8, 0xfa, 00547 0x2e, 0xff, 0x8d, 0x9d, 0xaf, 0xb4, 0xf1, 0x7a, 0x62, 0xf5 }, 00548 { 0x85, 0x74, 0x3e, 0x89, 0x9b, 0xc8, 0x2d, 0xbf, 0xa3, 0x6f, 00549 0xaa, 0xa7, 0xa2, 0x5b, 0x7c, 0xfd, 0x37, 0x24, 0x32, 0xcd }, 00550 { 0x6e, 0x4a, 0xfd, 0x50, 0x1f, 0xa6, 0xb4, 0xa1, 0x82, 0x3c, 00551 0xa3, 0xb1, 0x0b, 0xd9, 0xaa, 0x0b, 0xa9, 0x7b, 0xa1, 0x82 }, 00552 { 0x2e, 0x06, 0x6e, 0x62, 0x4b, 0xad, 0xb7, 0x6a, 0x18, 0x4c, 00553 0x8f, 0x90, 0xfb, 0xa0, 0x53, 0x33, 0x0e, 0x65, 0x0e, 0x92 }, 00554 { 0x07, 0xe9, 0x42, 0xaa, 0x4e, 0x3c, 0xd7, 0xc0, 0x4d, 0xed, 00555 0xc1, 0xd4, 0x6e, 0x2e, 0x8c, 0xc4, 0xc7, 0x41, 0xb3, 0xd9 }, 00556 { 0xb6, 0x58, 0x23, 0x18, 0xdd, 0xcf, 0xb6, 0x7a, 0x53, 0xa6, 00557 0x7d, 0x67, 0x6b, 0x8a, 0xd8, 0x69, 0xad, 0xed, 0x62, 0x9a }, 00558 { 0xf1, 0xbe, 0x3e, 0xe8, 0x77, 0x70, 0x31, 0x40, 0xd3, 0x4f, 00559 0x97, 0xea, 0x1a, 0xb3, 0xa0, 0x7c, 0x14, 0x13, 0x33, 0xe2 }, 00560 { 0x85, 0xf1, 0x64, 0x70, 0x3e, 0x61, 0xa6, 0x31, 0x31, 0xbe, 00561 0x7e, 0x45, 0x95, 0x8e, 0x07, 0x94, 0x12, 0x39, 0x04, 0xf9 }, 00562 }, 00563 }; 00564 00565 static const unsigned char ripemd160_test_key[KEYS][20] = 00566 { 00567 { 0x00, 0x11, 0x22, 0x33, 0x44, 0x55, 0x66, 0x77, 0x88, 0x99, 00568 0xaa, 0xbb, 0xcc, 0xdd, 0xee, 0xff, 0x01, 0x23, 0x45, 0x67 }, 00569 { 0x01, 0x23, 0x45, 0x67, 0x89, 0xab, 0xcd, 0xef, 0xfe, 0xdc, 00570 0xba, 0x98, 0x76, 0x54, 0x32, 0x10, 0x00, 0x11, 0x22, 0x33 }, 00571 }; 00572 00573 /* 00574 * Checkup routine 00575 */ 00576 int ripemd160_self_test( int verbose ) 00577 { 00578 int i, j; 00579 unsigned char output[20]; 00580 00581 memset( output, 0, sizeof output ); 00582 00583 for( i = 0; i < TESTS; i++ ) 00584 { 00585 if( verbose != 0 ) 00586 polarssl_printf( " RIPEMD-160 test #%d: ", i + 1 ); 00587 00588 ripemd160( (const unsigned char *) ripemd160_test_input[i], 00589 strlen( ripemd160_test_input[i] ), 00590 output ); 00591 00592 if( memcmp( output, ripemd160_test_md[i], 20 ) != 0 ) 00593 { 00594 if( verbose != 0 ) 00595 polarssl_printf( "failed\n" ); 00596 00597 return( 1 ); 00598 } 00599 00600 if( verbose != 0 ) 00601 polarssl_printf( "passed\n" ); 00602 00603 for( j = 0; j < KEYS; j++ ) 00604 { 00605 if( verbose != 0 ) 00606 polarssl_printf( " HMAC-RIPEMD-160 test #%d, key #%d: ", 00607 i + 1, j + 1 ); 00608 00609 ripemd160_hmac( ripemd160_test_key[j], 20, 00610 (const unsigned char *) ripemd160_test_input[i], 00611 strlen( ripemd160_test_input[i] ), 00612 output ); 00613 00614 if( memcmp( output, ripemd160_test_hmac[j][i], 20 ) != 0 ) 00615 { 00616 if( verbose != 0 ) 00617 polarssl_printf( "failed\n" ); 00618 00619 return( 1 ); 00620 } 00621 00622 if( verbose != 0 ) 00623 polarssl_printf( "passed\n" ); 00624 } 00625 00626 if( verbose != 0 ) 00627 polarssl_printf( "\n" ); 00628 } 00629 00630 return( 0 ); 00631 } 00632 00633 #endif /* POLARSSL_SELF_TEST */ 00634 00635 #endif /* POLARSSL_RIPEMD160_C */ 00636 00637
Generated on Tue Jul 12 2022 19:40:20 by
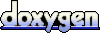