
Example program to test AES-GCM functionality. Used for a workshop
Embed:
(wiki syntax)
Show/hide line numbers
pkcs11.h
Go to the documentation of this file.
00001 /** 00002 * \file pkcs11.h 00003 * 00004 * \brief Wrapper for PKCS#11 library libpkcs11-helper 00005 * 00006 * \author Adriaan de Jong <dejong@fox-it.com> 00007 * 00008 * Copyright (C) 2006-2014, Brainspark B.V. 00009 * 00010 * This file is part of PolarSSL (http://www.polarssl.org) 00011 * Lead Maintainer: Paul Bakker <polarssl_maintainer at polarssl.org> 00012 * 00013 * All rights reserved. 00014 * 00015 * This program is free software; you can redistribute it and/or modify 00016 * it under the terms of the GNU General Public License as published by 00017 * the Free Software Foundation; either version 2 of the License, or 00018 * (at your option) any later version. 00019 * 00020 * This program is distributed in the hope that it will be useful, 00021 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00022 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00023 * GNU General Public License for more details. 00024 * 00025 * You should have received a copy of the GNU General Public License along 00026 * with this program; if not, write to the Free Software Foundation, Inc., 00027 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00028 */ 00029 #ifndef POLARSSL_PKCS11_H 00030 #define POLARSSL_PKCS11_H 00031 00032 #if !defined(POLARSSL_CONFIG_FILE) 00033 #include "config.h" 00034 #else 00035 #include POLARSSL_CONFIG_FILE 00036 #endif 00037 00038 #if defined(POLARSSL_PKCS11_C) 00039 00040 #include "x509_crt.h" 00041 00042 #include <pkcs11-helper-1.0/pkcs11h-certificate.h> 00043 00044 #if defined(_MSC_VER) && !defined(inline) 00045 #define inline _inline 00046 #else 00047 #if defined(__ARMCC_VERSION) && !defined(inline) 00048 #define inline __inline 00049 #endif /* __ARMCC_VERSION */ 00050 #endif /*_MSC_VER */ 00051 00052 #ifdef __cplusplus 00053 extern "C" { 00054 #endif 00055 00056 /** 00057 * Context for PKCS #11 private keys. 00058 */ 00059 typedef struct { 00060 pkcs11h_certificate_t pkcs11h_cert; 00061 int len; 00062 } pkcs11_context; 00063 00064 /** 00065 * Fill in a PolarSSL certificate, based on the given PKCS11 helper certificate. 00066 * 00067 * \param cert X.509 certificate to fill 00068 * \param pkcs11h_cert PKCS #11 helper certificate 00069 * 00070 * \return 0 on success. 00071 */ 00072 int pkcs11_x509_cert_init( x509_crt *cert, pkcs11h_certificate_t pkcs11h_cert ); 00073 00074 /** 00075 * Initialise a pkcs11_context, storing the given certificate. Note that the 00076 * pkcs11_context will take over control of the certificate, freeing it when 00077 * done. 00078 * 00079 * \param priv_key Private key structure to fill. 00080 * \param pkcs11_cert PKCS #11 helper certificate 00081 * 00082 * \return 0 on success 00083 */ 00084 int pkcs11_priv_key_init( pkcs11_context *priv_key, 00085 pkcs11h_certificate_t pkcs11_cert ); 00086 00087 /** 00088 * Free the contents of the given private key context. Note that the structure 00089 * itself is not freed. 00090 * 00091 * \param priv_key Private key structure to cleanup 00092 */ 00093 void pkcs11_priv_key_free( pkcs11_context *priv_key ); 00094 00095 /** 00096 * \brief Do an RSA private key decrypt, then remove the message 00097 * padding 00098 * 00099 * \param ctx PKCS #11 context 00100 * \param mode must be RSA_PRIVATE, for compatibility with rsa.c's signature 00101 * \param input buffer holding the encrypted data 00102 * \param output buffer that will hold the plaintext 00103 * \param olen will contain the plaintext length 00104 * \param output_max_len maximum length of the output buffer 00105 * 00106 * \return 0 if successful, or an POLARSSL_ERR_RSA_XXX error code 00107 * 00108 * \note The output buffer must be as large as the size 00109 * of ctx->N (eg. 128 bytes if RSA-1024 is used) otherwise 00110 * an error is thrown. 00111 */ 00112 int pkcs11_decrypt( pkcs11_context *ctx, 00113 int mode, size_t *olen, 00114 const unsigned char *input, 00115 unsigned char *output, 00116 size_t output_max_len ); 00117 00118 /** 00119 * \brief Do a private RSA to sign a message digest 00120 * 00121 * \param ctx PKCS #11 context 00122 * \param mode must be RSA_PRIVATE, for compatibility with rsa.c's signature 00123 * \param md_alg a POLARSSL_MD_* (use POLARSSL_MD_NONE for signing raw data) 00124 * \param hashlen message digest length (for POLARSSL_MD_NONE only) 00125 * \param hash buffer holding the message digest 00126 * \param sig buffer that will hold the ciphertext 00127 * 00128 * \return 0 if the signing operation was successful, 00129 * or an POLARSSL_ERR_RSA_XXX error code 00130 * 00131 * \note The "sig" buffer must be as large as the size 00132 * of ctx->N (eg. 128 bytes if RSA-1024 is used). 00133 */ 00134 int pkcs11_sign( pkcs11_context *ctx, 00135 int mode, 00136 md_type_t md_alg, 00137 unsigned int hashlen, 00138 const unsigned char *hash, 00139 unsigned char *sig ); 00140 00141 /** 00142 * SSL/TLS wrappers for PKCS#11 functions 00143 */ 00144 static inline int ssl_pkcs11_decrypt( void *ctx, int mode, size_t *olen, 00145 const unsigned char *input, unsigned char *output, 00146 size_t output_max_len ) 00147 { 00148 return pkcs11_decrypt( (pkcs11_context *) ctx, mode, olen, input, output, 00149 output_max_len ); 00150 } 00151 00152 static inline int ssl_pkcs11_sign( void *ctx, 00153 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng, 00154 int mode, md_type_t md_alg, unsigned int hashlen, 00155 const unsigned char *hash, unsigned char *sig ) 00156 { 00157 ((void) f_rng); 00158 ((void) p_rng); 00159 return pkcs11_sign( (pkcs11_context *) ctx, mode, md_alg, 00160 hashlen, hash, sig ); 00161 } 00162 00163 static inline size_t ssl_pkcs11_key_len( void *ctx ) 00164 { 00165 return ( (pkcs11_context *) ctx )->len; 00166 } 00167 00168 #ifdef __cplusplus 00169 } 00170 #endif 00171 00172 #endif /* POLARSSL_PKCS11_C */ 00173 00174 #endif /* POLARSSL_PKCS11_H */ 00175 00176
Generated on Tue Jul 12 2022 19:40:20 by
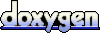