
Example program to test AES-GCM functionality. Used for a workshop
Embed:
(wiki syntax)
Show/hide line numbers
pem.h
Go to the documentation of this file.
00001 /** 00002 * \file pem.h 00003 * 00004 * \brief Privacy Enhanced Mail (PEM) decoding 00005 * 00006 * Copyright (C) 2006-2013, Brainspark B.V. 00007 * 00008 * This file is part of PolarSSL (http://www.polarssl.org) 00009 * Lead Maintainer: Paul Bakker <polarssl_maintainer at polarssl.org> 00010 * 00011 * All rights reserved. 00012 * 00013 * This program is free software; you can redistribute it and/or modify 00014 * it under the terms of the GNU General Public License as published by 00015 * the Free Software Foundation; either version 2 of the License, or 00016 * (at your option) any later version. 00017 * 00018 * This program is distributed in the hope that it will be useful, 00019 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00020 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00021 * GNU General Public License for more details. 00022 * 00023 * You should have received a copy of the GNU General Public License along 00024 * with this program; if not, write to the Free Software Foundation, Inc., 00025 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00026 */ 00027 #ifndef POLARSSL_PEM_H 00028 #define POLARSSL_PEM_H 00029 00030 #include <string.h> 00031 00032 /** 00033 * \name PEM Error codes 00034 * These error codes are returned in case of errors reading the 00035 * PEM data. 00036 * \{ 00037 */ 00038 #define POLARSSL_ERR_PEM_NO_HEADER_FOOTER_PRESENT -0x1080 /**< No PEM header or footer found. */ 00039 #define POLARSSL_ERR_PEM_INVALID_DATA -0x1100 /**< PEM string is not as expected. */ 00040 #define POLARSSL_ERR_PEM_MALLOC_FAILED -0x1180 /**< Failed to allocate memory. */ 00041 #define POLARSSL_ERR_PEM_INVALID_ENC_IV -0x1200 /**< RSA IV is not in hex-format. */ 00042 #define POLARSSL_ERR_PEM_UNKNOWN_ENC_ALG -0x1280 /**< Unsupported key encryption algorithm. */ 00043 #define POLARSSL_ERR_PEM_PASSWORD_REQUIRED -0x1300 /**< Private key password can't be empty. */ 00044 #define POLARSSL_ERR_PEM_PASSWORD_MISMATCH -0x1380 /**< Given private key password does not allow for correct decryption. */ 00045 #define POLARSSL_ERR_PEM_FEATURE_UNAVAILABLE -0x1400 /**< Unavailable feature, e.g. hashing/encryption combination. */ 00046 #define POLARSSL_ERR_PEM_BAD_INPUT_DATA -0x1480 /**< Bad input parameters to function. */ 00047 /* \} name */ 00048 00049 #ifdef __cplusplus 00050 extern "C" { 00051 #endif 00052 00053 #if defined(POLARSSL_PEM_PARSE_C) 00054 /** 00055 * \brief PEM context structure 00056 */ 00057 typedef struct 00058 { 00059 unsigned char *buf ; /*!< buffer for decoded data */ 00060 size_t buflen ; /*!< length of the buffer */ 00061 unsigned char *info ; /*!< buffer for extra header information */ 00062 } 00063 pem_context; 00064 00065 /** 00066 * \brief PEM context setup 00067 * 00068 * \param ctx context to be initialized 00069 */ 00070 void pem_init( pem_context *ctx ); 00071 00072 /** 00073 * \brief Read a buffer for PEM information and store the resulting 00074 * data into the specified context buffers. 00075 * 00076 * \param ctx context to use 00077 * \param header header string to seek and expect 00078 * \param footer footer string to seek and expect 00079 * \param data source data to look in 00080 * \param pwd password for decryption (can be NULL) 00081 * \param pwdlen length of password 00082 * \param use_len destination for total length used (set after header is 00083 * correctly read, so unless you get 00084 * POLARSSL_ERR_PEM_BAD_INPUT_DATA or 00085 * POLARSSL_ERR_PEM_NO_HEADER_FOOTER_PRESENT, use_len is 00086 * the length to skip) 00087 * 00088 * \note Attempts to check password correctness by verifying if 00089 * the decrypted text starts with an ASN.1 sequence of 00090 * appropriate length 00091 * 00092 * \return 0 on success, or a specific PEM error code 00093 */ 00094 int pem_read_buffer( pem_context *ctx, const char *header, const char *footer, 00095 const unsigned char *data, 00096 const unsigned char *pwd, 00097 size_t pwdlen, size_t *use_len ); 00098 00099 /** 00100 * \brief PEM context memory freeing 00101 * 00102 * \param ctx context to be freed 00103 */ 00104 void pem_free( pem_context *ctx ); 00105 #endif /* POLARSSL_PEM_PARSE_C */ 00106 00107 #if defined(POLARSSL_PEM_WRITE_C) 00108 /** 00109 * \brief Write a buffer of PEM information from a DER encoded 00110 * buffer. 00111 * 00112 * \param header header string to write 00113 * \param footer footer string to write 00114 * \param der_data DER data to write 00115 * \param der_len length of the DER data 00116 * \param buf buffer to write to 00117 * \param buf_len length of output buffer 00118 * \param olen total length written / required (if buf_len is not enough) 00119 * 00120 * \return 0 on success, or a specific PEM or BASE64 error code. On 00121 * POLARSSL_ERR_BASE64_BUFFER_TOO_SMALL olen is the required 00122 * size. 00123 */ 00124 int pem_write_buffer( const char *header, const char *footer, 00125 const unsigned char *der_data, size_t der_len, 00126 unsigned char *buf, size_t buf_len, size_t *olen ); 00127 #endif /* POLARSSL_PEM_WRITE_C */ 00128 00129 #ifdef __cplusplus 00130 } 00131 #endif 00132 00133 #endif /* pem.h */ 00134 00135
Generated on Tue Jul 12 2022 19:40:19 by
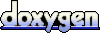