
Example program to test AES-GCM functionality. Used for a workshop
Embed:
(wiki syntax)
Show/hide line numbers
memory_buffer_alloc.c
00001 /* 00002 * Buffer-based memory allocator 00003 * 00004 * Copyright (C) 2006-2014, Brainspark B.V. 00005 * 00006 * This file is part of PolarSSL (http://www.polarssl.org) 00007 * Lead Maintainer: Paul Bakker <polarssl_maintainer at polarssl.org> 00008 * 00009 * All rights reserved. 00010 * 00011 * This program is free software; you can redistribute it and/or modify 00012 * it under the terms of the GNU General Public License as published by 00013 * the Free Software Foundation; either version 2 of the License, or 00014 * (at your option) any later version. 00015 * 00016 * This program is distributed in the hope that it will be useful, 00017 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00018 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00019 * GNU General Public License for more details. 00020 * 00021 * You should have received a copy of the GNU General Public License along 00022 * with this program; if not, write to the Free Software Foundation, Inc., 00023 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00024 */ 00025 00026 #if !defined(POLARSSL_CONFIG_FILE) 00027 #include "polarssl/config.h" 00028 #else 00029 #include POLARSSL_CONFIG_FILE 00030 #endif 00031 00032 #if defined(POLARSSL_MEMORY_BUFFER_ALLOC_C) 00033 00034 #include "polarssl/memory_buffer_alloc.h" 00035 00036 #include <string.h> 00037 00038 #if defined(POLARSSL_MEMORY_DEBUG) 00039 #include <stdio.h> 00040 #if defined(POLARSSL_MEMORY_BACKTRACE) 00041 #include <execinfo.h> 00042 #endif 00043 #endif 00044 00045 #if defined(POLARSSL_THREADING_C) 00046 #include "polarssl/threading.h" 00047 #endif 00048 00049 #if defined(POLARSSL_PLATFORM_C) 00050 #include "polarssl/platform.h" 00051 #else 00052 #define polarssl_fprintf fprintf 00053 #endif 00054 00055 #define MAGIC1 0xFF00AA55 00056 #define MAGIC2 0xEE119966 00057 #define MAX_BT 20 00058 00059 typedef struct _memory_header memory_header; 00060 struct _memory_header 00061 { 00062 size_t magic1; 00063 size_t size; 00064 size_t alloc; 00065 memory_header *prev; 00066 memory_header *next; 00067 memory_header *prev_free; 00068 memory_header *next_free; 00069 #if defined(POLARSSL_MEMORY_BACKTRACE) 00070 char **trace; 00071 size_t trace_count; 00072 #endif 00073 size_t magic2; 00074 }; 00075 00076 typedef struct 00077 { 00078 unsigned char *buf; 00079 size_t len; 00080 memory_header *first; 00081 memory_header *first_free; 00082 size_t current_alloc_size; 00083 int verify; 00084 #if defined(POLARSSL_MEMORY_DEBUG) 00085 size_t malloc_count; 00086 size_t free_count; 00087 size_t total_used; 00088 size_t maximum_used; 00089 size_t header_count; 00090 size_t maximum_header_count; 00091 #endif 00092 #if defined(POLARSSL_THREADING_C) 00093 threading_mutex_t mutex; 00094 #endif 00095 } 00096 buffer_alloc_ctx; 00097 00098 static buffer_alloc_ctx heap; 00099 00100 #if defined(POLARSSL_MEMORY_DEBUG) 00101 static void debug_header( memory_header *hdr ) 00102 { 00103 #if defined(POLARSSL_MEMORY_BACKTRACE) 00104 size_t i; 00105 #endif 00106 00107 polarssl_fprintf( stderr, "HDR: PTR(%10u), PREV(%10u), NEXT(%10u), " 00108 "ALLOC(%u), SIZE(%10u)\n", 00109 (size_t) hdr, (size_t) hdr->prev, (size_t) hdr->next, 00110 hdr->alloc, hdr->size ); 00111 polarssl_fprintf( stderr, " FPREV(%10u), FNEXT(%10u)\n", 00112 (size_t) hdr->prev_free, (size_t) hdr->next_free ); 00113 00114 #if defined(POLARSSL_MEMORY_BACKTRACE) 00115 polarssl_fprintf( stderr, "TRACE: \n" ); 00116 for( i = 0; i < hdr->trace_count; i++ ) 00117 polarssl_fprintf( stderr, "%s\n", hdr->trace[i] ); 00118 polarssl_fprintf( stderr, "\n" ); 00119 #endif 00120 } 00121 00122 static void debug_chain() 00123 { 00124 memory_header *cur = heap.first; 00125 00126 polarssl_fprintf( stderr, "\nBlock list\n" ); 00127 while( cur != NULL ) 00128 { 00129 debug_header( cur ); 00130 cur = cur->next; 00131 } 00132 00133 polarssl_fprintf( stderr, "Free list\n" ); 00134 cur = heap.first_free; 00135 00136 while( cur != NULL ) 00137 { 00138 debug_header( cur ); 00139 cur = cur->next_free; 00140 } 00141 } 00142 #endif /* POLARSSL_MEMORY_DEBUG */ 00143 00144 static int verify_header( memory_header *hdr ) 00145 { 00146 if( hdr->magic1 != MAGIC1 ) 00147 { 00148 #if defined(POLARSSL_MEMORY_DEBUG) 00149 polarssl_fprintf( stderr, "FATAL: MAGIC1 mismatch\n" ); 00150 #endif 00151 return( 1 ); 00152 } 00153 00154 if( hdr->magic2 != MAGIC2 ) 00155 { 00156 #if defined(POLARSSL_MEMORY_DEBUG) 00157 polarssl_fprintf( stderr, "FATAL: MAGIC2 mismatch\n" ); 00158 #endif 00159 return( 1 ); 00160 } 00161 00162 if( hdr->alloc > 1 ) 00163 { 00164 #if defined(POLARSSL_MEMORY_DEBUG) 00165 polarssl_fprintf( stderr, "FATAL: alloc has illegal value\n" ); 00166 #endif 00167 return( 1 ); 00168 } 00169 00170 if( hdr->prev != NULL && hdr->prev == hdr->next ) 00171 { 00172 #if defined(POLARSSL_MEMORY_DEBUG) 00173 polarssl_fprintf( stderr, "FATAL: prev == next\n" ); 00174 #endif 00175 return( 1 ); 00176 } 00177 00178 if( hdr->prev_free != NULL && hdr->prev_free == hdr->next_free ) 00179 { 00180 #if defined(POLARSSL_MEMORY_DEBUG) 00181 polarssl_fprintf( stderr, "FATAL: prev_free == next_free\n" ); 00182 #endif 00183 return( 1 ); 00184 } 00185 00186 return( 0 ); 00187 } 00188 00189 static int verify_chain() 00190 { 00191 memory_header *prv = heap.first, *cur = heap.first->next; 00192 00193 if( verify_header( heap.first ) != 0 ) 00194 { 00195 #if defined(POLARSSL_MEMORY_DEBUG) 00196 polarssl_fprintf( stderr, "FATAL: verification of first header " 00197 "failed\n" ); 00198 #endif 00199 return( 1 ); 00200 } 00201 00202 if( heap.first->prev != NULL ) 00203 { 00204 #if defined(POLARSSL_MEMORY_DEBUG) 00205 polarssl_fprintf( stderr, "FATAL: verification failed: " 00206 "first->prev != NULL\n" ); 00207 #endif 00208 return( 1 ); 00209 } 00210 00211 while( cur != NULL ) 00212 { 00213 if( verify_header( cur ) != 0 ) 00214 { 00215 #if defined(POLARSSL_MEMORY_DEBUG) 00216 polarssl_fprintf( stderr, "FATAL: verification of header " 00217 "failed\n" ); 00218 #endif 00219 return( 1 ); 00220 } 00221 00222 if( cur->prev != prv ) 00223 { 00224 #if defined(POLARSSL_MEMORY_DEBUG) 00225 polarssl_fprintf( stderr, "FATAL: verification failed: " 00226 "cur->prev != prv\n" ); 00227 #endif 00228 return( 1 ); 00229 } 00230 00231 prv = cur; 00232 cur = cur->next; 00233 } 00234 00235 return( 0 ); 00236 } 00237 00238 static void *buffer_alloc_malloc( size_t len ) 00239 { 00240 memory_header *new, *cur = heap.first_free; 00241 unsigned char *p; 00242 #if defined(POLARSSL_MEMORY_BACKTRACE) 00243 void *trace_buffer[MAX_BT]; 00244 size_t trace_cnt; 00245 #endif 00246 00247 if( heap.buf == NULL || heap.first == NULL ) 00248 return( NULL ); 00249 00250 if( len % POLARSSL_MEMORY_ALIGN_MULTIPLE ) 00251 { 00252 len -= len % POLARSSL_MEMORY_ALIGN_MULTIPLE; 00253 len += POLARSSL_MEMORY_ALIGN_MULTIPLE; 00254 } 00255 00256 // Find block that fits 00257 // 00258 while( cur != NULL ) 00259 { 00260 if( cur->size >= len ) 00261 break; 00262 00263 cur = cur->next_free; 00264 } 00265 00266 if( cur == NULL ) 00267 return( NULL ); 00268 00269 if( cur->alloc != 0 ) 00270 { 00271 #if defined(POLARSSL_MEMORY_DEBUG) 00272 polarssl_fprintf( stderr, "FATAL: block in free_list but allocated " 00273 "data\n" ); 00274 #endif 00275 exit( 1 ); 00276 } 00277 00278 #if defined(POLARSSL_MEMORY_DEBUG) 00279 heap.malloc_count++; 00280 #endif 00281 00282 // Found location, split block if > memory_header + 4 room left 00283 // 00284 if( cur->size - len < sizeof(memory_header) + 00285 POLARSSL_MEMORY_ALIGN_MULTIPLE ) 00286 { 00287 cur->alloc = 1; 00288 00289 // Remove from free_list 00290 // 00291 if( cur->prev_free != NULL ) 00292 cur->prev_free->next_free = cur->next_free; 00293 else 00294 heap.first_free = cur->next_free; 00295 00296 if( cur->next_free != NULL ) 00297 cur->next_free->prev_free = cur->prev_free; 00298 00299 cur->prev_free = NULL; 00300 cur->next_free = NULL; 00301 00302 #if defined(POLARSSL_MEMORY_DEBUG) 00303 heap.total_used += cur->size; 00304 if( heap.total_used > heap.maximum_used) 00305 heap.maximum_used = heap.total_used; 00306 #endif 00307 #if defined(POLARSSL_MEMORY_BACKTRACE) 00308 trace_cnt = backtrace( trace_buffer, MAX_BT ); 00309 cur->trace = backtrace_symbols( trace_buffer, trace_cnt ); 00310 cur->trace_count = trace_cnt; 00311 #endif 00312 00313 if( ( heap.verify & MEMORY_VERIFY_ALLOC ) && verify_chain() != 0 ) 00314 exit( 1 ); 00315 00316 return ( (unsigned char *) cur ) + sizeof(memory_header); 00317 } 00318 00319 p = ( (unsigned char *) cur ) + sizeof(memory_header) + len; 00320 new = (memory_header *) p; 00321 00322 new->size = cur->size - len - sizeof(memory_header); 00323 new->alloc = 0; 00324 new->prev = cur; 00325 new->next = cur->next; 00326 #if defined(POLARSSL_MEMORY_BACKTRACE) 00327 new->trace = NULL; 00328 new->trace_count = 0; 00329 #endif 00330 new->magic1 = MAGIC1; 00331 new->magic2 = MAGIC2; 00332 00333 if( new->next != NULL ) 00334 new->next->prev = new; 00335 00336 // Replace cur with new in free_list 00337 // 00338 new->prev_free = cur->prev_free; 00339 new->next_free = cur->next_free; 00340 if( new->prev_free != NULL ) 00341 new->prev_free->next_free = new; 00342 else 00343 heap.first_free = new; 00344 00345 if( new->next_free != NULL ) 00346 new->next_free->prev_free = new; 00347 00348 cur->alloc = 1; 00349 cur->size = len; 00350 cur->next = new; 00351 cur->prev_free = NULL; 00352 cur->next_free = NULL; 00353 00354 #if defined(POLARSSL_MEMORY_DEBUG) 00355 heap.header_count++; 00356 if( heap.header_count > heap.maximum_header_count ) 00357 heap.maximum_header_count = heap.header_count; 00358 heap.total_used += cur->size; 00359 if( heap.total_used > heap.maximum_used) 00360 heap.maximum_used = heap.total_used; 00361 #endif 00362 #if defined(POLARSSL_MEMORY_BACKTRACE) 00363 trace_cnt = backtrace( trace_buffer, MAX_BT ); 00364 cur->trace = backtrace_symbols( trace_buffer, trace_cnt ); 00365 cur->trace_count = trace_cnt; 00366 #endif 00367 00368 if( ( heap.verify & MEMORY_VERIFY_ALLOC ) && verify_chain() != 0 ) 00369 exit( 1 ); 00370 00371 return ( (unsigned char *) cur ) + sizeof(memory_header); 00372 } 00373 00374 static void buffer_alloc_free( void *ptr ) 00375 { 00376 memory_header *hdr, *old = NULL; 00377 unsigned char *p = (unsigned char *) ptr; 00378 00379 if( ptr == NULL || heap.buf == NULL || heap.first == NULL ) 00380 return; 00381 00382 if( p < heap.buf || p > heap.buf + heap.len ) 00383 { 00384 #if defined(POLARSSL_MEMORY_DEBUG) 00385 polarssl_fprintf( stderr, "FATAL: polarssl_free() outside of managed " 00386 "space\n" ); 00387 #endif 00388 exit( 1 ); 00389 } 00390 00391 p -= sizeof(memory_header); 00392 hdr = (memory_header *) p; 00393 00394 if( verify_header( hdr ) != 0 ) 00395 exit( 1 ); 00396 00397 if( hdr->alloc != 1 ) 00398 { 00399 #if defined(POLARSSL_MEMORY_DEBUG) 00400 polarssl_fprintf( stderr, "FATAL: polarssl_free() on unallocated " 00401 "data\n" ); 00402 #endif 00403 exit( 1 ); 00404 } 00405 00406 hdr->alloc = 0; 00407 00408 #if defined(POLARSSL_MEMORY_DEBUG) 00409 heap.free_count++; 00410 heap.total_used -= hdr->size; 00411 #endif 00412 00413 // Regroup with block before 00414 // 00415 if( hdr->prev != NULL && hdr->prev->alloc == 0 ) 00416 { 00417 #if defined(POLARSSL_MEMORY_DEBUG) 00418 heap.header_count--; 00419 #endif 00420 hdr->prev->size += sizeof(memory_header) + hdr->size; 00421 hdr->prev->next = hdr->next; 00422 old = hdr; 00423 hdr = hdr->prev; 00424 00425 if( hdr->next != NULL ) 00426 hdr->next->prev = hdr; 00427 00428 #if defined(POLARSSL_MEMORY_BACKTRACE) 00429 free( old->trace ); 00430 #endif 00431 memset( old, 0, sizeof(memory_header) ); 00432 } 00433 00434 // Regroup with block after 00435 // 00436 if( hdr->next != NULL && hdr->next->alloc == 0 ) 00437 { 00438 #if defined(POLARSSL_MEMORY_DEBUG) 00439 heap.header_count--; 00440 #endif 00441 hdr->size += sizeof(memory_header) + hdr->next->size; 00442 old = hdr->next; 00443 hdr->next = hdr->next->next; 00444 00445 if( hdr->prev_free != NULL || hdr->next_free != NULL ) 00446 { 00447 if( hdr->prev_free != NULL ) 00448 hdr->prev_free->next_free = hdr->next_free; 00449 else 00450 heap.first_free = hdr->next_free; 00451 00452 if( hdr->next_free != NULL ) 00453 hdr->next_free->prev_free = hdr->prev_free; 00454 } 00455 00456 hdr->prev_free = old->prev_free; 00457 hdr->next_free = old->next_free; 00458 00459 if( hdr->prev_free != NULL ) 00460 hdr->prev_free->next_free = hdr; 00461 else 00462 heap.first_free = hdr; 00463 00464 if( hdr->next_free != NULL ) 00465 hdr->next_free->prev_free = hdr; 00466 00467 if( hdr->next != NULL ) 00468 hdr->next->prev = hdr; 00469 00470 #if defined(POLARSSL_MEMORY_BACKTRACE) 00471 free( old->trace ); 00472 #endif 00473 memset( old, 0, sizeof(memory_header) ); 00474 } 00475 00476 // Prepend to free_list if we have not merged 00477 // (Does not have to stay in same order as prev / next list) 00478 // 00479 if( old == NULL ) 00480 { 00481 hdr->next_free = heap.first_free; 00482 heap.first_free->prev_free = hdr; 00483 heap.first_free = hdr; 00484 } 00485 00486 #if defined(POLARSSL_MEMORY_BACKTRACE) 00487 hdr->trace = NULL; 00488 hdr->trace_count = 0; 00489 #endif 00490 00491 if( ( heap.verify & MEMORY_VERIFY_FREE ) && verify_chain() != 0 ) 00492 exit( 1 ); 00493 } 00494 00495 void memory_buffer_set_verify( int verify ) 00496 { 00497 heap.verify = verify; 00498 } 00499 00500 int memory_buffer_alloc_verify() 00501 { 00502 return verify_chain(); 00503 } 00504 00505 #if defined(POLARSSL_MEMORY_DEBUG) 00506 void memory_buffer_alloc_status() 00507 { 00508 polarssl_fprintf( stderr, 00509 "Current use: %u blocks / %u bytes, max: %u blocks / " 00510 "%u bytes (total %u bytes), malloc / free: %u / %u\n", 00511 heap.header_count, heap.total_used, 00512 heap.maximum_header_count, heap.maximum_used, 00513 heap.maximum_header_count * sizeof( memory_header ) 00514 + heap.maximum_used, 00515 heap.malloc_count, heap.free_count ); 00516 00517 if( heap.first->next == NULL ) 00518 polarssl_fprintf( stderr, "All memory de-allocated in stack buffer\n" ); 00519 else 00520 { 00521 polarssl_fprintf( stderr, "Memory currently allocated:\n" ); 00522 debug_chain(); 00523 } 00524 } 00525 #endif /* POLARSSL_MEMORY_DEBUG */ 00526 00527 #if defined(POLARSSL_THREADING_C) 00528 static void *buffer_alloc_malloc_mutexed( size_t len ) 00529 { 00530 void *buf; 00531 polarssl_mutex_lock( &heap.mutex ); 00532 buf = buffer_alloc_malloc( len ); 00533 polarssl_mutex_unlock( &heap.mutex ); 00534 return( buf ); 00535 } 00536 00537 static void buffer_alloc_free_mutexed( void *ptr ) 00538 { 00539 polarssl_mutex_lock( &heap.mutex ); 00540 buffer_alloc_free( ptr ); 00541 polarssl_mutex_unlock( &heap.mutex ); 00542 } 00543 #endif /* POLARSSL_THREADING_C */ 00544 00545 int memory_buffer_alloc_init( unsigned char *buf, size_t len ) 00546 { 00547 memset( &heap, 0, sizeof(buffer_alloc_ctx) ); 00548 memset( buf, 0, len ); 00549 00550 #if defined(POLARSSL_THREADING_C) 00551 polarssl_mutex_init( &heap.mutex ); 00552 platform_set_malloc_free( buffer_alloc_malloc_mutexed, 00553 buffer_alloc_free_mutexed ); 00554 #else 00555 platform_set_malloc_free( buffer_alloc_malloc, buffer_alloc_free ); 00556 #endif 00557 00558 heap.buf = buf; 00559 heap.len = len; 00560 00561 heap.first = (memory_header *) buf; 00562 heap.first->size = len - sizeof(memory_header); 00563 heap.first->magic1 = MAGIC1; 00564 heap.first->magic2 = MAGIC2; 00565 heap.first_free = heap.first; 00566 return( 0 ); 00567 } 00568 00569 void memory_buffer_alloc_free() 00570 { 00571 #if defined(POLARSSL_THREADING_C) 00572 polarssl_mutex_free( &heap.mutex ); 00573 #endif 00574 memset( &heap, 0, sizeof(buffer_alloc_ctx) ); 00575 } 00576 00577 #endif /* POLARSSL_MEMORY_BUFFER_ALLOC_C */ 00578 00579
Generated on Tue Jul 12 2022 19:40:17 by
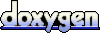