
Example program to test AES-GCM functionality. Used for a workshop
Embed:
(wiki syntax)
Show/hide line numbers
md_wrap.c
Go to the documentation of this file.
00001 /** 00002 * \file md_wrap.c 00003 00004 * \brief Generic message digest wrapper for PolarSSL 00005 * 00006 * \author Adriaan de Jong <dejong@fox-it.com> 00007 * 00008 * Copyright (C) 2006-2014, Brainspark B.V. 00009 * 00010 * This file is part of PolarSSL (http://www.polarssl.org) 00011 * Lead Maintainer: Paul Bakker <polarssl_maintainer at polarssl.org> 00012 * 00013 * All rights reserved. 00014 * 00015 * This program is free software; you can redistribute it and/or modify 00016 * it under the terms of the GNU General Public License as published by 00017 * the Free Software Foundation; either version 2 of the License, or 00018 * (at your option) any later version. 00019 * 00020 * This program is distributed in the hope that it will be useful, 00021 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00022 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00023 * GNU General Public License for more details. 00024 * 00025 * You should have received a copy of the GNU General Public License along 00026 * with this program; if not, write to the Free Software Foundation, Inc., 00027 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00028 */ 00029 00030 #if !defined(POLARSSL_CONFIG_FILE) 00031 #include "polarssl/config.h" 00032 #else 00033 #include POLARSSL_CONFIG_FILE 00034 #endif 00035 00036 #if defined(POLARSSL_MD_C) 00037 00038 #include "polarssl/md_wrap.h" 00039 00040 #if defined(POLARSSL_MD2_C) 00041 #include "polarssl/md2.h" 00042 #endif 00043 00044 #if defined(POLARSSL_MD4_C) 00045 #include "polarssl/md4.h" 00046 #endif 00047 00048 #if defined(POLARSSL_MD5_C) 00049 #include "polarssl/md5.h" 00050 #endif 00051 00052 #if defined(POLARSSL_RIPEMD160_C) 00053 #include "polarssl/ripemd160.h" 00054 #endif 00055 00056 #if defined(POLARSSL_SHA1_C) 00057 #include "polarssl/sha1.h" 00058 #endif 00059 00060 #if defined(POLARSSL_SHA256_C) 00061 #include "polarssl/sha256.h" 00062 #endif 00063 00064 #if defined(POLARSSL_SHA512_C) 00065 #include "polarssl/sha512.h" 00066 #endif 00067 00068 #if defined(POLARSSL_PLATFORM_C) 00069 #include "polarssl/platform.h" 00070 #else 00071 #define polarssl_malloc malloc 00072 #define polarssl_free free 00073 #endif 00074 00075 #include <stdlib.h> 00076 00077 #if defined(POLARSSL_MD2_C) 00078 00079 static void md2_starts_wrap( void *ctx ) 00080 { 00081 md2_starts( (md2_context *) ctx ); 00082 } 00083 00084 static void md2_update_wrap( void *ctx, const unsigned char *input, 00085 size_t ilen ) 00086 { 00087 md2_update( (md2_context *) ctx, input, ilen ); 00088 } 00089 00090 static void md2_finish_wrap( void *ctx, unsigned char *output ) 00091 { 00092 md2_finish( (md2_context *) ctx, output ); 00093 } 00094 00095 static int md2_file_wrap( const char *path, unsigned char *output ) 00096 { 00097 #if defined(POLARSSL_FS_IO) 00098 return md2_file( path, output ); 00099 #else 00100 ((void) path); 00101 ((void) output); 00102 return POLARSSL_ERR_MD_FEATURE_UNAVAILABLE; 00103 #endif 00104 } 00105 00106 static void md2_hmac_starts_wrap( void *ctx, const unsigned char *key, 00107 size_t keylen ) 00108 { 00109 md2_hmac_starts( (md2_context *) ctx, key, keylen ); 00110 } 00111 00112 static void md2_hmac_update_wrap( void *ctx, const unsigned char *input, 00113 size_t ilen ) 00114 { 00115 md2_hmac_update( (md2_context *) ctx, input, ilen ); 00116 } 00117 00118 static void md2_hmac_finish_wrap( void *ctx, unsigned char *output ) 00119 { 00120 md2_hmac_finish( (md2_context *) ctx, output ); 00121 } 00122 00123 static void md2_hmac_reset_wrap( void *ctx ) 00124 { 00125 md2_hmac_reset( (md2_context *) ctx ); 00126 } 00127 00128 static void * md2_ctx_alloc( void ) 00129 { 00130 return polarssl_malloc( sizeof( md2_context ) ); 00131 } 00132 00133 static void md2_ctx_free( void *ctx ) 00134 { 00135 polarssl_free( ctx ); 00136 } 00137 00138 static void md2_process_wrap( void *ctx, const unsigned char *data ) 00139 { 00140 ((void) data); 00141 00142 md2_process( (md2_context *) ctx ); 00143 } 00144 00145 const md_info_t md2_info = { 00146 POLARSSL_MD_MD2, 00147 "MD2", 00148 16, 00149 md2_starts_wrap, 00150 md2_update_wrap, 00151 md2_finish_wrap, 00152 md2, 00153 md2_file_wrap, 00154 md2_hmac_starts_wrap, 00155 md2_hmac_update_wrap, 00156 md2_hmac_finish_wrap, 00157 md2_hmac_reset_wrap, 00158 md2_hmac, 00159 md2_ctx_alloc, 00160 md2_ctx_free, 00161 md2_process_wrap, 00162 }; 00163 00164 #endif /* POLARSSL_MD2_C */ 00165 00166 #if defined(POLARSSL_MD4_C) 00167 00168 static void md4_starts_wrap( void *ctx ) 00169 { 00170 md4_starts( (md4_context *) ctx ); 00171 } 00172 00173 static void md4_update_wrap( void *ctx, const unsigned char *input, 00174 size_t ilen ) 00175 { 00176 md4_update( (md4_context *) ctx, input, ilen ); 00177 } 00178 00179 static void md4_finish_wrap( void *ctx, unsigned char *output ) 00180 { 00181 md4_finish( (md4_context *) ctx, output ); 00182 } 00183 00184 static int md4_file_wrap( const char *path, unsigned char *output ) 00185 { 00186 #if defined(POLARSSL_FS_IO) 00187 return md4_file( path, output ); 00188 #else 00189 ((void) path); 00190 ((void) output); 00191 return POLARSSL_ERR_MD_FEATURE_UNAVAILABLE; 00192 #endif 00193 } 00194 00195 static void md4_hmac_starts_wrap( void *ctx, const unsigned char *key, 00196 size_t keylen ) 00197 { 00198 md4_hmac_starts( (md4_context *) ctx, key, keylen ); 00199 } 00200 00201 static void md4_hmac_update_wrap( void *ctx, const unsigned char *input, 00202 size_t ilen ) 00203 { 00204 md4_hmac_update( (md4_context *) ctx, input, ilen ); 00205 } 00206 00207 static void md4_hmac_finish_wrap( void *ctx, unsigned char *output ) 00208 { 00209 md4_hmac_finish( (md4_context *) ctx, output ); 00210 } 00211 00212 static void md4_hmac_reset_wrap( void *ctx ) 00213 { 00214 md4_hmac_reset( (md4_context *) ctx ); 00215 } 00216 00217 static void *md4_ctx_alloc( void ) 00218 { 00219 return polarssl_malloc( sizeof( md4_context ) ); 00220 } 00221 00222 static void md4_ctx_free( void *ctx ) 00223 { 00224 polarssl_free( ctx ); 00225 } 00226 00227 static void md4_process_wrap( void *ctx, const unsigned char *data ) 00228 { 00229 md4_process( (md4_context *) ctx, data ); 00230 } 00231 00232 const md_info_t md4_info = { 00233 POLARSSL_MD_MD4, 00234 "MD4", 00235 16, 00236 md4_starts_wrap, 00237 md4_update_wrap, 00238 md4_finish_wrap, 00239 md4, 00240 md4_file_wrap, 00241 md4_hmac_starts_wrap, 00242 md4_hmac_update_wrap, 00243 md4_hmac_finish_wrap, 00244 md4_hmac_reset_wrap, 00245 md4_hmac, 00246 md4_ctx_alloc, 00247 md4_ctx_free, 00248 md4_process_wrap, 00249 }; 00250 00251 #endif /* POLARSSL_MD4_C */ 00252 00253 #if defined(POLARSSL_MD5_C) 00254 00255 static void md5_starts_wrap( void *ctx ) 00256 { 00257 md5_starts( (md5_context *) ctx ); 00258 } 00259 00260 static void md5_update_wrap( void *ctx, const unsigned char *input, 00261 size_t ilen ) 00262 { 00263 md5_update( (md5_context *) ctx, input, ilen ); 00264 } 00265 00266 static void md5_finish_wrap( void *ctx, unsigned char *output ) 00267 { 00268 md5_finish( (md5_context *) ctx, output ); 00269 } 00270 00271 static int md5_file_wrap( const char *path, unsigned char *output ) 00272 { 00273 #if defined(POLARSSL_FS_IO) 00274 return md5_file( path, output ); 00275 #else 00276 ((void) path); 00277 ((void) output); 00278 return POLARSSL_ERR_MD_FEATURE_UNAVAILABLE; 00279 #endif 00280 } 00281 00282 static void md5_hmac_starts_wrap( void *ctx, const unsigned char *key, 00283 size_t keylen ) 00284 { 00285 md5_hmac_starts( (md5_context *) ctx, key, keylen ); 00286 } 00287 00288 static void md5_hmac_update_wrap( void *ctx, const unsigned char *input, 00289 size_t ilen ) 00290 { 00291 md5_hmac_update( (md5_context *) ctx, input, ilen ); 00292 } 00293 00294 static void md5_hmac_finish_wrap( void *ctx, unsigned char *output ) 00295 { 00296 md5_hmac_finish( (md5_context *) ctx, output ); 00297 } 00298 00299 static void md5_hmac_reset_wrap( void *ctx ) 00300 { 00301 md5_hmac_reset( (md5_context *) ctx ); 00302 } 00303 00304 static void * md5_ctx_alloc( void ) 00305 { 00306 return polarssl_malloc( sizeof( md5_context ) ); 00307 } 00308 00309 static void md5_ctx_free( void *ctx ) 00310 { 00311 polarssl_free( ctx ); 00312 } 00313 00314 static void md5_process_wrap( void *ctx, const unsigned char *data ) 00315 { 00316 md5_process( (md5_context *) ctx, data ); 00317 } 00318 00319 const md_info_t md5_info = { 00320 POLARSSL_MD_MD5, 00321 "MD5", 00322 16, 00323 md5_starts_wrap, 00324 md5_update_wrap, 00325 md5_finish_wrap, 00326 md5, 00327 md5_file_wrap, 00328 md5_hmac_starts_wrap, 00329 md5_hmac_update_wrap, 00330 md5_hmac_finish_wrap, 00331 md5_hmac_reset_wrap, 00332 md5_hmac, 00333 md5_ctx_alloc, 00334 md5_ctx_free, 00335 md5_process_wrap, 00336 }; 00337 00338 #endif /* POLARSSL_MD5_C */ 00339 00340 #if defined(POLARSSL_RIPEMD160_C) 00341 00342 static void ripemd160_starts_wrap( void *ctx ) 00343 { 00344 ripemd160_starts( (ripemd160_context *) ctx ); 00345 } 00346 00347 static void ripemd160_update_wrap( void *ctx, const unsigned char *input, 00348 size_t ilen ) 00349 { 00350 ripemd160_update( (ripemd160_context *) ctx, input, ilen ); 00351 } 00352 00353 static void ripemd160_finish_wrap( void *ctx, unsigned char *output ) 00354 { 00355 ripemd160_finish( (ripemd160_context *) ctx, output ); 00356 } 00357 00358 static int ripemd160_file_wrap( const char *path, unsigned char *output ) 00359 { 00360 #if defined(POLARSSL_FS_IO) 00361 return ripemd160_file( path, output ); 00362 #else 00363 ((void) path); 00364 ((void) output); 00365 return POLARSSL_ERR_MD_FEATURE_UNAVAILABLE; 00366 #endif 00367 } 00368 00369 static void ripemd160_hmac_starts_wrap( void *ctx, const unsigned char *key, 00370 size_t keylen ) 00371 { 00372 ripemd160_hmac_starts( (ripemd160_context *) ctx, key, keylen ); 00373 } 00374 00375 static void ripemd160_hmac_update_wrap( void *ctx, const unsigned char *input, 00376 size_t ilen ) 00377 { 00378 ripemd160_hmac_update( (ripemd160_context *) ctx, input, ilen ); 00379 } 00380 00381 static void ripemd160_hmac_finish_wrap( void *ctx, unsigned char *output ) 00382 { 00383 ripemd160_hmac_finish( (ripemd160_context *) ctx, output ); 00384 } 00385 00386 static void ripemd160_hmac_reset_wrap( void *ctx ) 00387 { 00388 ripemd160_hmac_reset( (ripemd160_context *) ctx ); 00389 } 00390 00391 static void * ripemd160_ctx_alloc( void ) 00392 { 00393 return polarssl_malloc( sizeof( ripemd160_context ) ); 00394 } 00395 00396 static void ripemd160_ctx_free( void *ctx ) 00397 { 00398 polarssl_free( ctx ); 00399 } 00400 00401 static void ripemd160_process_wrap( void *ctx, const unsigned char *data ) 00402 { 00403 ripemd160_process( (ripemd160_context *) ctx, data ); 00404 } 00405 00406 const md_info_t ripemd160_info = { 00407 POLARSSL_MD_RIPEMD160, 00408 "RIPEMD160", 00409 20, 00410 ripemd160_starts_wrap, 00411 ripemd160_update_wrap, 00412 ripemd160_finish_wrap, 00413 ripemd160, 00414 ripemd160_file_wrap, 00415 ripemd160_hmac_starts_wrap, 00416 ripemd160_hmac_update_wrap, 00417 ripemd160_hmac_finish_wrap, 00418 ripemd160_hmac_reset_wrap, 00419 ripemd160_hmac, 00420 ripemd160_ctx_alloc, 00421 ripemd160_ctx_free, 00422 ripemd160_process_wrap, 00423 }; 00424 00425 #endif /* POLARSSL_RIPEMD160_C */ 00426 00427 #if defined(POLARSSL_SHA1_C) 00428 00429 static void sha1_starts_wrap( void *ctx ) 00430 { 00431 sha1_starts( (sha1_context *) ctx ); 00432 } 00433 00434 static void sha1_update_wrap( void *ctx, const unsigned char *input, 00435 size_t ilen ) 00436 { 00437 sha1_update( (sha1_context *) ctx, input, ilen ); 00438 } 00439 00440 static void sha1_finish_wrap( void *ctx, unsigned char *output ) 00441 { 00442 sha1_finish( (sha1_context *) ctx, output ); 00443 } 00444 00445 static int sha1_file_wrap( const char *path, unsigned char *output ) 00446 { 00447 #if defined(POLARSSL_FS_IO) 00448 return sha1_file( path, output ); 00449 #else 00450 ((void) path); 00451 ((void) output); 00452 return POLARSSL_ERR_MD_FEATURE_UNAVAILABLE; 00453 #endif 00454 } 00455 00456 static void sha1_hmac_starts_wrap( void *ctx, const unsigned char *key, 00457 size_t keylen ) 00458 { 00459 sha1_hmac_starts( (sha1_context *) ctx, key, keylen ); 00460 } 00461 00462 static void sha1_hmac_update_wrap( void *ctx, const unsigned char *input, 00463 size_t ilen ) 00464 { 00465 sha1_hmac_update( (sha1_context *) ctx, input, ilen ); 00466 } 00467 00468 static void sha1_hmac_finish_wrap( void *ctx, unsigned char *output ) 00469 { 00470 sha1_hmac_finish( (sha1_context *) ctx, output ); 00471 } 00472 00473 static void sha1_hmac_reset_wrap( void *ctx ) 00474 { 00475 sha1_hmac_reset( (sha1_context *) ctx ); 00476 } 00477 00478 static void * sha1_ctx_alloc( void ) 00479 { 00480 return polarssl_malloc( sizeof( sha1_context ) ); 00481 } 00482 00483 static void sha1_ctx_free( void *ctx ) 00484 { 00485 polarssl_free( ctx ); 00486 } 00487 00488 static void sha1_process_wrap( void *ctx, const unsigned char *data ) 00489 { 00490 sha1_process( (sha1_context *) ctx, data ); 00491 } 00492 00493 const md_info_t sha1_info = { 00494 POLARSSL_MD_SHA1, 00495 "SHA1", 00496 20, 00497 sha1_starts_wrap, 00498 sha1_update_wrap, 00499 sha1_finish_wrap, 00500 sha1, 00501 sha1_file_wrap, 00502 sha1_hmac_starts_wrap, 00503 sha1_hmac_update_wrap, 00504 sha1_hmac_finish_wrap, 00505 sha1_hmac_reset_wrap, 00506 sha1_hmac, 00507 sha1_ctx_alloc, 00508 sha1_ctx_free, 00509 sha1_process_wrap, 00510 }; 00511 00512 #endif /* POLARSSL_SHA1_C */ 00513 00514 /* 00515 * Wrappers for generic message digests 00516 */ 00517 #if defined(POLARSSL_SHA256_C) 00518 00519 static void sha224_starts_wrap( void *ctx ) 00520 { 00521 sha256_starts( (sha256_context *) ctx, 1 ); 00522 } 00523 00524 static void sha224_update_wrap( void *ctx, const unsigned char *input, 00525 size_t ilen ) 00526 { 00527 sha256_update( (sha256_context *) ctx, input, ilen ); 00528 } 00529 00530 static void sha224_finish_wrap( void *ctx, unsigned char *output ) 00531 { 00532 sha256_finish( (sha256_context *) ctx, output ); 00533 } 00534 00535 static void sha224_wrap( const unsigned char *input, size_t ilen, 00536 unsigned char *output ) 00537 { 00538 sha256( input, ilen, output, 1 ); 00539 } 00540 00541 static int sha224_file_wrap( const char *path, unsigned char *output ) 00542 { 00543 #if defined(POLARSSL_FS_IO) 00544 return sha256_file( path, output, 1 ); 00545 #else 00546 ((void) path); 00547 ((void) output); 00548 return POLARSSL_ERR_MD_FEATURE_UNAVAILABLE; 00549 #endif 00550 } 00551 00552 static void sha224_hmac_starts_wrap( void *ctx, const unsigned char *key, 00553 size_t keylen ) 00554 { 00555 sha256_hmac_starts( (sha256_context *) ctx, key, keylen, 1 ); 00556 } 00557 00558 static void sha224_hmac_update_wrap( void *ctx, const unsigned char *input, 00559 size_t ilen ) 00560 { 00561 sha256_hmac_update( (sha256_context *) ctx, input, ilen ); 00562 } 00563 00564 static void sha224_hmac_finish_wrap( void *ctx, unsigned char *output ) 00565 { 00566 sha256_hmac_finish( (sha256_context *) ctx, output ); 00567 } 00568 00569 static void sha224_hmac_reset_wrap( void *ctx ) 00570 { 00571 sha256_hmac_reset( (sha256_context *) ctx ); 00572 } 00573 00574 static void sha224_hmac_wrap( const unsigned char *key, size_t keylen, 00575 const unsigned char *input, size_t ilen, 00576 unsigned char *output ) 00577 { 00578 sha256_hmac( key, keylen, input, ilen, output, 1 ); 00579 } 00580 00581 static void * sha224_ctx_alloc( void ) 00582 { 00583 return polarssl_malloc( sizeof( sha256_context ) ); 00584 } 00585 00586 static void sha224_ctx_free( void *ctx ) 00587 { 00588 polarssl_free( ctx ); 00589 } 00590 00591 static void sha224_process_wrap( void *ctx, const unsigned char *data ) 00592 { 00593 sha256_process( (sha256_context *) ctx, data ); 00594 } 00595 00596 const md_info_t sha224_info = { 00597 POLARSSL_MD_SHA224, 00598 "SHA224", 00599 28, 00600 sha224_starts_wrap, 00601 sha224_update_wrap, 00602 sha224_finish_wrap, 00603 sha224_wrap, 00604 sha224_file_wrap, 00605 sha224_hmac_starts_wrap, 00606 sha224_hmac_update_wrap, 00607 sha224_hmac_finish_wrap, 00608 sha224_hmac_reset_wrap, 00609 sha224_hmac_wrap, 00610 sha224_ctx_alloc, 00611 sha224_ctx_free, 00612 sha224_process_wrap, 00613 }; 00614 00615 static void sha256_starts_wrap( void *ctx ) 00616 { 00617 sha256_starts( (sha256_context *) ctx, 0 ); 00618 } 00619 00620 static void sha256_update_wrap( void *ctx, const unsigned char *input, 00621 size_t ilen ) 00622 { 00623 sha256_update( (sha256_context *) ctx, input, ilen ); 00624 } 00625 00626 static void sha256_finish_wrap( void *ctx, unsigned char *output ) 00627 { 00628 sha256_finish( (sha256_context *) ctx, output ); 00629 } 00630 00631 static void sha256_wrap( const unsigned char *input, size_t ilen, 00632 unsigned char *output ) 00633 { 00634 sha256( input, ilen, output, 0 ); 00635 } 00636 00637 static int sha256_file_wrap( const char *path, unsigned char *output ) 00638 { 00639 #if defined(POLARSSL_FS_IO) 00640 return sha256_file( path, output, 0 ); 00641 #else 00642 ((void) path); 00643 ((void) output); 00644 return POLARSSL_ERR_MD_FEATURE_UNAVAILABLE; 00645 #endif 00646 } 00647 00648 static void sha256_hmac_starts_wrap( void *ctx, const unsigned char *key, 00649 size_t keylen ) 00650 { 00651 sha256_hmac_starts( (sha256_context *) ctx, key, keylen, 0 ); 00652 } 00653 00654 static void sha256_hmac_update_wrap( void *ctx, const unsigned char *input, 00655 size_t ilen ) 00656 { 00657 sha256_hmac_update( (sha256_context *) ctx, input, ilen ); 00658 } 00659 00660 static void sha256_hmac_finish_wrap( void *ctx, unsigned char *output ) 00661 { 00662 sha256_hmac_finish( (sha256_context *) ctx, output ); 00663 } 00664 00665 static void sha256_hmac_reset_wrap( void *ctx ) 00666 { 00667 sha256_hmac_reset( (sha256_context *) ctx ); 00668 } 00669 00670 static void sha256_hmac_wrap( const unsigned char *key, size_t keylen, 00671 const unsigned char *input, size_t ilen, 00672 unsigned char *output ) 00673 { 00674 sha256_hmac( key, keylen, input, ilen, output, 0 ); 00675 } 00676 00677 static void * sha256_ctx_alloc( void ) 00678 { 00679 return polarssl_malloc( sizeof( sha256_context ) ); 00680 } 00681 00682 static void sha256_ctx_free( void *ctx ) 00683 { 00684 polarssl_free( ctx ); 00685 } 00686 00687 static void sha256_process_wrap( void *ctx, const unsigned char *data ) 00688 { 00689 sha256_process( (sha256_context *) ctx, data ); 00690 } 00691 00692 const md_info_t sha256_info = { 00693 POLARSSL_MD_SHA256, 00694 "SHA256", 00695 32, 00696 sha256_starts_wrap, 00697 sha256_update_wrap, 00698 sha256_finish_wrap, 00699 sha256_wrap, 00700 sha256_file_wrap, 00701 sha256_hmac_starts_wrap, 00702 sha256_hmac_update_wrap, 00703 sha256_hmac_finish_wrap, 00704 sha256_hmac_reset_wrap, 00705 sha256_hmac_wrap, 00706 sha256_ctx_alloc, 00707 sha256_ctx_free, 00708 sha256_process_wrap, 00709 }; 00710 00711 #endif /* POLARSSL_SHA256_C */ 00712 00713 #if defined(POLARSSL_SHA512_C) 00714 00715 static void sha384_starts_wrap( void *ctx ) 00716 { 00717 sha512_starts( (sha512_context *) ctx, 1 ); 00718 } 00719 00720 static void sha384_update_wrap( void *ctx, const unsigned char *input, 00721 size_t ilen ) 00722 { 00723 sha512_update( (sha512_context *) ctx, input, ilen ); 00724 } 00725 00726 static void sha384_finish_wrap( void *ctx, unsigned char *output ) 00727 { 00728 sha512_finish( (sha512_context *) ctx, output ); 00729 } 00730 00731 static void sha384_wrap( const unsigned char *input, size_t ilen, 00732 unsigned char *output ) 00733 { 00734 sha512( input, ilen, output, 1 ); 00735 } 00736 00737 static int sha384_file_wrap( const char *path, unsigned char *output ) 00738 { 00739 #if defined(POLARSSL_FS_IO) 00740 return sha512_file( path, output, 1 ); 00741 #else 00742 ((void) path); 00743 ((void) output); 00744 return POLARSSL_ERR_MD_FEATURE_UNAVAILABLE; 00745 #endif 00746 } 00747 00748 static void sha384_hmac_starts_wrap( void *ctx, const unsigned char *key, 00749 size_t keylen ) 00750 { 00751 sha512_hmac_starts( (sha512_context *) ctx, key, keylen, 1 ); 00752 } 00753 00754 static void sha384_hmac_update_wrap( void *ctx, const unsigned char *input, 00755 size_t ilen ) 00756 { 00757 sha512_hmac_update( (sha512_context *) ctx, input, ilen ); 00758 } 00759 00760 static void sha384_hmac_finish_wrap( void *ctx, unsigned char *output ) 00761 { 00762 sha512_hmac_finish( (sha512_context *) ctx, output ); 00763 } 00764 00765 static void sha384_hmac_reset_wrap( void *ctx ) 00766 { 00767 sha512_hmac_reset( (sha512_context *) ctx ); 00768 } 00769 00770 static void sha384_hmac_wrap( const unsigned char *key, size_t keylen, 00771 const unsigned char *input, size_t ilen, 00772 unsigned char *output ) 00773 { 00774 sha512_hmac( key, keylen, input, ilen, output, 1 ); 00775 } 00776 00777 static void * sha384_ctx_alloc( void ) 00778 { 00779 return polarssl_malloc( sizeof( sha512_context ) ); 00780 } 00781 00782 static void sha384_ctx_free( void *ctx ) 00783 { 00784 polarssl_free( ctx ); 00785 } 00786 00787 static void sha384_process_wrap( void *ctx, const unsigned char *data ) 00788 { 00789 sha512_process( (sha512_context *) ctx, data ); 00790 } 00791 00792 const md_info_t sha384_info = { 00793 POLARSSL_MD_SHA384, 00794 "SHA384", 00795 48, 00796 sha384_starts_wrap, 00797 sha384_update_wrap, 00798 sha384_finish_wrap, 00799 sha384_wrap, 00800 sha384_file_wrap, 00801 sha384_hmac_starts_wrap, 00802 sha384_hmac_update_wrap, 00803 sha384_hmac_finish_wrap, 00804 sha384_hmac_reset_wrap, 00805 sha384_hmac_wrap, 00806 sha384_ctx_alloc, 00807 sha384_ctx_free, 00808 sha384_process_wrap, 00809 }; 00810 00811 static void sha512_starts_wrap( void *ctx ) 00812 { 00813 sha512_starts( (sha512_context *) ctx, 0 ); 00814 } 00815 00816 static void sha512_update_wrap( void *ctx, const unsigned char *input, 00817 size_t ilen ) 00818 { 00819 sha512_update( (sha512_context *) ctx, input, ilen ); 00820 } 00821 00822 static void sha512_finish_wrap( void *ctx, unsigned char *output ) 00823 { 00824 sha512_finish( (sha512_context *) ctx, output ); 00825 } 00826 00827 static void sha512_wrap( const unsigned char *input, size_t ilen, 00828 unsigned char *output ) 00829 { 00830 sha512( input, ilen, output, 0 ); 00831 } 00832 00833 static int sha512_file_wrap( const char *path, unsigned char *output ) 00834 { 00835 #if defined(POLARSSL_FS_IO) 00836 return sha512_file( path, output, 0 ); 00837 #else 00838 ((void) path); 00839 ((void) output); 00840 return POLARSSL_ERR_MD_FEATURE_UNAVAILABLE; 00841 #endif 00842 } 00843 00844 static void sha512_hmac_starts_wrap( void *ctx, const unsigned char *key, 00845 size_t keylen ) 00846 { 00847 sha512_hmac_starts( (sha512_context *) ctx, key, keylen, 0 ); 00848 } 00849 00850 static void sha512_hmac_update_wrap( void *ctx, const unsigned char *input, 00851 size_t ilen ) 00852 { 00853 sha512_hmac_update( (sha512_context *) ctx, input, ilen ); 00854 } 00855 00856 static void sha512_hmac_finish_wrap( void *ctx, unsigned char *output ) 00857 { 00858 sha512_hmac_finish( (sha512_context *) ctx, output ); 00859 } 00860 00861 static void sha512_hmac_reset_wrap( void *ctx ) 00862 { 00863 sha512_hmac_reset( (sha512_context *) ctx ); 00864 } 00865 00866 static void sha512_hmac_wrap( const unsigned char *key, size_t keylen, 00867 const unsigned char *input, size_t ilen, 00868 unsigned char *output ) 00869 { 00870 sha512_hmac( key, keylen, input, ilen, output, 0 ); 00871 } 00872 00873 static void * sha512_ctx_alloc( void ) 00874 { 00875 return polarssl_malloc( sizeof( sha512_context ) ); 00876 } 00877 00878 static void sha512_ctx_free( void *ctx ) 00879 { 00880 polarssl_free( ctx ); 00881 } 00882 00883 static void sha512_process_wrap( void *ctx, const unsigned char *data ) 00884 { 00885 sha512_process( (sha512_context *) ctx, data ); 00886 } 00887 00888 const md_info_t sha512_info = { 00889 POLARSSL_MD_SHA512, 00890 "SHA512", 00891 64, 00892 sha512_starts_wrap, 00893 sha512_update_wrap, 00894 sha512_finish_wrap, 00895 sha512_wrap, 00896 sha512_file_wrap, 00897 sha512_hmac_starts_wrap, 00898 sha512_hmac_update_wrap, 00899 sha512_hmac_finish_wrap, 00900 sha512_hmac_reset_wrap, 00901 sha512_hmac_wrap, 00902 sha512_ctx_alloc, 00903 sha512_ctx_free, 00904 sha512_process_wrap, 00905 }; 00906 00907 #endif /* POLARSSL_SHA512_C */ 00908 00909 #endif /* POLARSSL_MD_C */ 00910 00911
Generated on Tue Jul 12 2022 19:40:16 by
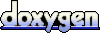