
Example program to test AES-GCM functionality. Used for a workshop
Embed:
(wiki syntax)
Show/hide line numbers
md2.h
Go to the documentation of this file.
00001 /** 00002 * \file md2.h 00003 * 00004 * \brief MD2 message digest algorithm (hash function) 00005 * 00006 * Copyright (C) 2006-2014, Brainspark B.V. 00007 * 00008 * This file is part of PolarSSL (http://www.polarssl.org) 00009 * Lead Maintainer: Paul Bakker <polarssl_maintainer at polarssl.org> 00010 * 00011 * All rights reserved. 00012 * 00013 * This program is free software; you can redistribute it and/or modify 00014 * it under the terms of the GNU General Public License as published by 00015 * the Free Software Foundation; either version 2 of the License, or 00016 * (at your option) any later version. 00017 * 00018 * This program is distributed in the hope that it will be useful, 00019 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00020 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00021 * GNU General Public License for more details. 00022 * 00023 * You should have received a copy of the GNU General Public License along 00024 * with this program; if not, write to the Free Software Foundation, Inc., 00025 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00026 */ 00027 #ifndef POLARSSL_MD2_H 00028 #define POLARSSL_MD2_H 00029 00030 #if !defined(POLARSSL_CONFIG_FILE) 00031 #include "config.h" 00032 #else 00033 #include POLARSSL_CONFIG_FILE 00034 #endif 00035 00036 #include <string.h> 00037 00038 #define POLARSSL_ERR_MD2_FILE_IO_ERROR -0x0070 /**< Read/write error in file. */ 00039 00040 #if !defined(POLARSSL_MD2_ALT) 00041 // Regular implementation 00042 // 00043 00044 #ifdef __cplusplus 00045 extern "C" { 00046 #endif 00047 00048 /** 00049 * \brief MD2 context structure 00050 */ 00051 typedef struct 00052 { 00053 unsigned char cksum[16]; /*!< checksum of the data block */ 00054 unsigned char state[48]; /*!< intermediate digest state */ 00055 unsigned char buffer[16]; /*!< data block being processed */ 00056 00057 unsigned char ipad[16]; /*!< HMAC: inner padding */ 00058 unsigned char opad[16]; /*!< HMAC: outer padding */ 00059 size_t left ; /*!< amount of data in buffer */ 00060 } 00061 md2_context; 00062 00063 /** 00064 * \brief MD2 context setup 00065 * 00066 * \param ctx context to be initialized 00067 */ 00068 void md2_starts( md2_context *ctx ); 00069 00070 /** 00071 * \brief MD2 process buffer 00072 * 00073 * \param ctx MD2 context 00074 * \param input buffer holding the data 00075 * \param ilen length of the input data 00076 */ 00077 void md2_update( md2_context *ctx, const unsigned char *input, size_t ilen ); 00078 00079 /** 00080 * \brief MD2 final digest 00081 * 00082 * \param ctx MD2 context 00083 * \param output MD2 checksum result 00084 */ 00085 void md2_finish( md2_context *ctx, unsigned char output[16] ); 00086 00087 #ifdef __cplusplus 00088 } 00089 #endif 00090 00091 #else /* POLARSSL_MD2_ALT */ 00092 #include "md2_alt.h" 00093 #endif /* POLARSSL_MD2_ALT */ 00094 00095 #ifdef __cplusplus 00096 extern "C" { 00097 #endif 00098 00099 /** 00100 * \brief Output = MD2( input buffer ) 00101 * 00102 * \param input buffer holding the data 00103 * \param ilen length of the input data 00104 * \param output MD2 checksum result 00105 */ 00106 void md2( const unsigned char *input, size_t ilen, unsigned char output[16] ); 00107 00108 /** 00109 * \brief Output = MD2( file contents ) 00110 * 00111 * \param path input file name 00112 * \param output MD2 checksum result 00113 * 00114 * \return 0 if successful, or POLARSSL_ERR_MD2_FILE_IO_ERROR 00115 */ 00116 int md2_file( const char *path, unsigned char output[16] ); 00117 00118 /** 00119 * \brief MD2 HMAC context setup 00120 * 00121 * \param ctx HMAC context to be initialized 00122 * \param key HMAC secret key 00123 * \param keylen length of the HMAC key 00124 */ 00125 void md2_hmac_starts( md2_context *ctx, const unsigned char *key, 00126 size_t keylen ); 00127 00128 /** 00129 * \brief MD2 HMAC process buffer 00130 * 00131 * \param ctx HMAC context 00132 * \param input buffer holding the data 00133 * \param ilen length of the input data 00134 */ 00135 void md2_hmac_update( md2_context *ctx, const unsigned char *input, 00136 size_t ilen ); 00137 00138 /** 00139 * \brief MD2 HMAC final digest 00140 * 00141 * \param ctx HMAC context 00142 * \param output MD2 HMAC checksum result 00143 */ 00144 void md2_hmac_finish( md2_context *ctx, unsigned char output[16] ); 00145 00146 /** 00147 * \brief MD2 HMAC context reset 00148 * 00149 * \param ctx HMAC context to be reset 00150 */ 00151 void md2_hmac_reset( md2_context *ctx ); 00152 00153 /** 00154 * \brief Output = HMAC-MD2( hmac key, input buffer ) 00155 * 00156 * \param key HMAC secret key 00157 * \param keylen length of the HMAC key 00158 * \param input buffer holding the data 00159 * \param ilen length of the input data 00160 * \param output HMAC-MD2 result 00161 */ 00162 void md2_hmac( const unsigned char *key, size_t keylen, 00163 const unsigned char *input, size_t ilen, 00164 unsigned char output[16] ); 00165 00166 /** 00167 * \brief Checkup routine 00168 * 00169 * \return 0 if successful, or 1 if the test failed 00170 */ 00171 int md2_self_test( int verbose ); 00172 00173 /* Internal use */ 00174 void md2_process( md2_context *ctx ); 00175 00176 #ifdef __cplusplus 00177 } 00178 #endif 00179 00180 #endif /* md2.h */ 00181 00182
Generated on Tue Jul 12 2022 19:40:15 by
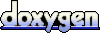