
Example program to test AES-GCM functionality. Used for a workshop
Embed:
(wiki syntax)
Show/hide line numbers
ecp.h
Go to the documentation of this file.
00001 /** 00002 * \file ecp.h 00003 * 00004 * \brief Elliptic curves over GF(p) 00005 * 00006 * Copyright (C) 2006-2013, Brainspark B.V. 00007 * 00008 * This file is part of PolarSSL (http://www.polarssl.org) 00009 * Lead Maintainer: Paul Bakker <polarssl_maintainer at polarssl.org> 00010 * 00011 * All rights reserved. 00012 * 00013 * This program is free software; you can redistribute it and/or modify 00014 * it under the terms of the GNU General Public License as published by 00015 * the Free Software Foundation; either version 2 of the License, or 00016 * (at your option) any later version. 00017 * 00018 * This program is distributed in the hope that it will be useful, 00019 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00020 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00021 * GNU General Public License for more details. 00022 * 00023 * You should have received a copy of the GNU General Public License along 00024 * with this program; if not, write to the Free Software Foundation, Inc., 00025 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00026 */ 00027 #ifndef POLARSSL_ECP_H 00028 #define POLARSSL_ECP_H 00029 00030 #include "bignum.h" 00031 00032 /* 00033 * ECP error codes 00034 */ 00035 #define POLARSSL_ERR_ECP_BAD_INPUT_DATA -0x4F80 /**< Bad input parameters to function. */ 00036 #define POLARSSL_ERR_ECP_BUFFER_TOO_SMALL -0x4F00 /**< The buffer is too small to write to. */ 00037 #define POLARSSL_ERR_ECP_FEATURE_UNAVAILABLE -0x4E80 /**< Requested curve not available. */ 00038 #define POLARSSL_ERR_ECP_VERIFY_FAILED -0x4E00 /**< The signature is not valid. */ 00039 #define POLARSSL_ERR_ECP_MALLOC_FAILED -0x4D80 /**< Memory allocation failed. */ 00040 #define POLARSSL_ERR_ECP_RANDOM_FAILED -0x4D00 /**< Generation of random value, such as (ephemeral) key, failed. */ 00041 #define POLARSSL_ERR_ECP_INVALID_KEY -0x4C80 /**< Invalid private or public key. */ 00042 #define POLARSSL_ERR_ECP_SIG_LEN_MISMATCH -0x4C00 /**< Signature is valid but shorter than the user-supplied length. */ 00043 00044 #ifdef __cplusplus 00045 extern "C" { 00046 #endif 00047 00048 /** 00049 * Domain parameters (curve, subgroup and generator) identifiers. 00050 * 00051 * Only curves over prime fields are supported. 00052 * 00053 * \warning This library does not support validation of arbitrary domain 00054 * parameters. Therefore, only well-known domain parameters from trusted 00055 * sources should be used. See ecp_use_known_dp(). 00056 */ 00057 typedef enum 00058 { 00059 POLARSSL_ECP_DP_NONE = 0, 00060 POLARSSL_ECP_DP_SECP192R1 , /*!< 192-bits NIST curve */ 00061 POLARSSL_ECP_DP_SECP224R1 , /*!< 224-bits NIST curve */ 00062 POLARSSL_ECP_DP_SECP256R1 , /*!< 256-bits NIST curve */ 00063 POLARSSL_ECP_DP_SECP384R1 , /*!< 384-bits NIST curve */ 00064 POLARSSL_ECP_DP_SECP521R1 , /*!< 521-bits NIST curve */ 00065 POLARSSL_ECP_DP_BP256R1 , /*!< 256-bits Brainpool curve */ 00066 POLARSSL_ECP_DP_BP384R1 , /*!< 384-bits Brainpool curve */ 00067 POLARSSL_ECP_DP_BP512R1 , /*!< 512-bits Brainpool curve */ 00068 POLARSSL_ECP_DP_M221 , /*!< (not implemented yet) */ 00069 POLARSSL_ECP_DP_M255 , /*!< Curve25519 */ 00070 POLARSSL_ECP_DP_M383 , /*!< (not implemented yet) */ 00071 POLARSSL_ECP_DP_M511 , /*!< (not implemented yet) */ 00072 POLARSSL_ECP_DP_SECP192K1 , /*!< 192-bits "Koblitz" curve */ 00073 POLARSSL_ECP_DP_SECP224K1 , /*!< 224-bits "Koblitz" curve */ 00074 POLARSSL_ECP_DP_SECP256K1 , /*!< 256-bits "Koblitz" curve */ 00075 } ecp_group_id; 00076 00077 /** 00078 * Number of supported curves (plus one for NONE). 00079 * 00080 * (Montgomery curves excluded for now.) 00081 */ 00082 #define POLARSSL_ECP_DP_MAX 12 00083 00084 /** 00085 * Curve information for use by other modules 00086 */ 00087 typedef struct 00088 { 00089 ecp_group_id grp_id ; /*!< Internal identifier */ 00090 uint16_t tls_id ; /*!< TLS NamedCurve identifier */ 00091 uint16_t size ; /*!< Curve size in bits */ 00092 const char *name ; /*!< Human-friendly name */ 00093 } ecp_curve_info; 00094 00095 /** 00096 * \brief ECP point structure (jacobian coordinates) 00097 * 00098 * \note All functions expect and return points satisfying 00099 * the following condition: Z == 0 or Z == 1. (Other 00100 * values of Z are used by internal functions only.) 00101 * The point is zero, or "at infinity", if Z == 0. 00102 * Otherwise, X and Y are its standard (affine) coordinates. 00103 */ 00104 typedef struct 00105 { 00106 mpi X ; /*!< the point's X coordinate */ 00107 mpi Y ; /*!< the point's Y coordinate */ 00108 mpi Z ; /*!< the point's Z coordinate */ 00109 } 00110 ecp_point; 00111 00112 /** 00113 * \brief ECP group structure 00114 * 00115 * We consider two types of curves equations: 00116 * 1. Short Weierstrass y^2 = x^3 + A x + B mod P (SEC1 + RFC 4492) 00117 * 2. Montgomery, y^2 = x^3 + A x^2 + x mod P (M255 + draft) 00118 * In both cases, a generator G for a prime-order subgroup is fixed. In the 00119 * short weierstrass, this subgroup is actually the whole curve, and its 00120 * cardinal is denoted by N. 00121 * 00122 * In the case of Short Weierstrass curves, our code requires that N is an odd 00123 * prime. (Use odd in ecp_mul() and prime in ecdsa_sign() for blinding.) 00124 * 00125 * In the case of Montgomery curves, we don't store A but (A + 2) / 4 which is 00126 * the quantity actually used in the formulas. Also, nbits is not the size of N 00127 * but the required size for private keys. 00128 * 00129 * If modp is NULL, reduction modulo P is done using a generic algorithm. 00130 * Otherwise, it must point to a function that takes an mpi in the range 00131 * 0..2^(2*pbits)-1 and transforms it in-place in an integer of little more 00132 * than pbits, so that the integer may be efficiently brought in the 0..P-1 00133 * range by a few additions or substractions. It must return 0 on success and 00134 * non-zero on failure. 00135 */ 00136 typedef struct 00137 { 00138 ecp_group_id id ; /*!< internal group identifier */ 00139 mpi P ; /*!< prime modulus of the base field */ 00140 mpi A ; /*!< 1. A in the equation, or 2. (A + 2) / 4 */ 00141 mpi B ; /*!< 1. B in the equation, or 2. unused */ 00142 ecp_point G ; /*!< generator of the (sub)group used */ 00143 mpi N ; /*!< 1. the order of G, or 2. unused */ 00144 size_t pbits ; /*!< number of bits in P */ 00145 size_t nbits ; /*!< number of bits in 1. P, or 2. private keys */ 00146 unsigned int h ; /*!< internal: 1 if the constants are static */ 00147 int (*modp)(mpi *); /*!< function for fast reduction mod P */ 00148 int (*t_pre)(ecp_point *, void *); /*!< unused */ 00149 int (*t_post)(ecp_point *, void *); /*!< unused */ 00150 void *t_data ; /*!< unused */ 00151 ecp_point *T ; /*!< pre-computed points for ecp_mul_comb() */ 00152 size_t T_size ; /*!< number for pre-computed points */ 00153 } 00154 ecp_group; 00155 00156 /** 00157 * \brief ECP key pair structure 00158 * 00159 * A generic key pair that could be used for ECDSA, fixed ECDH, etc. 00160 * 00161 * \note Members purposefully in the same order as struc ecdsa_context. 00162 */ 00163 typedef struct 00164 { 00165 ecp_group grp ; /*!< Elliptic curve and base point */ 00166 mpi d ; /*!< our secret value */ 00167 ecp_point Q ; /*!< our public value */ 00168 } 00169 ecp_keypair; 00170 00171 /** 00172 * \name SECTION: Module settings 00173 * 00174 * The configuration options you can set for this module are in this section. 00175 * Either change them in config.h or define them on the compiler command line. 00176 * \{ 00177 */ 00178 00179 #if !defined(POLARSSL_ECP_MAX_BITS) 00180 /** 00181 * Maximum size of the groups (that is, of N and P) 00182 */ 00183 #define POLARSSL_ECP_MAX_BITS 521 /**< Maximum bit size of groups */ 00184 #endif 00185 00186 #define POLARSSL_ECP_MAX_BYTES ( ( POLARSSL_ECP_MAX_BITS + 7 ) / 8 ) 00187 #define POLARSSL_ECP_MAX_PT_LEN ( 2 * POLARSSL_ECP_MAX_BYTES + 1 ) 00188 00189 #if !defined(POLARSSL_ECP_WINDOW_SIZE) 00190 /* 00191 * Maximum "window" size used for point multiplication. 00192 * Default: 6. 00193 * Minimum value: 2. Maximum value: 7. 00194 * 00195 * Result is an array of at most ( 1 << ( POLARSSL_ECP_WINDOW_SIZE - 1 ) ) 00196 * points used for point multiplication. This value is directly tied to EC 00197 * peak memory usage, so decreasing it by one should roughly cut memory usage 00198 * by two (if large curves are in use). 00199 * 00200 * Reduction in size may reduce speed, but larger curves are impacted first. 00201 * Sample performances (in ECDHE handshakes/s, with FIXED_POINT_OPTIM = 1): 00202 * w-size: 6 5 4 3 2 00203 * 521 145 141 135 120 97 00204 * 384 214 209 198 177 146 00205 * 256 320 320 303 262 226 00206 00207 * 224 475 475 453 398 342 00208 * 192 640 640 633 587 476 00209 */ 00210 #define POLARSSL_ECP_WINDOW_SIZE 6 /**< Maximum window size used */ 00211 #endif 00212 00213 #if !defined(POLARSSL_ECP_FIXED_POINT_OPTIM) 00214 /* 00215 * Trade memory for speed on fixed-point multiplication. 00216 * 00217 * This speeds up repeated multiplication of the generator (that is, the 00218 * multiplication in ECDSA signatures, and half of the multiplications in 00219 * ECDSA verification and ECDHE) by a factor roughly 3 to 4. 00220 * 00221 * The cost is increasing EC peak memory usage by a factor roughly 2. 00222 * 00223 * Change this value to 0 to reduce peak memory usage. 00224 */ 00225 #define POLARSSL_ECP_FIXED_POINT_OPTIM 1 /**< Enable fixed-point speed-up */ 00226 #endif 00227 00228 /* \} name SECTION: Module settings */ 00229 00230 /* 00231 * Point formats, from RFC 4492's enum ECPointFormat 00232 */ 00233 #define POLARSSL_ECP_PF_UNCOMPRESSED 0 /**< Uncompressed point format */ 00234 #define POLARSSL_ECP_PF_COMPRESSED 1 /**< Compressed point format */ 00235 00236 /* 00237 * Some other constants from RFC 4492 00238 */ 00239 #define POLARSSL_ECP_TLS_NAMED_CURVE 3 /**< ECCurveType's named_curve */ 00240 00241 /** 00242 * \brief Get the list of supported curves in order of preferrence 00243 * (full information) 00244 * 00245 * \return A statically allocated array, the last entry is 0. 00246 */ 00247 const ecp_curve_info *ecp_curve_list( void ); 00248 00249 /** 00250 * \brief Get the list of supported curves in order of preferrence 00251 * (grp_id only) 00252 * 00253 * \return A statically allocated array, 00254 * terminated with POLARSSL_ECP_DP_NONE. 00255 */ 00256 const ecp_group_id *ecp_grp_id_list( void ); 00257 00258 /** 00259 * \brief Get curve information from an internal group identifier 00260 * 00261 * \param grp_id A POLARSSL_ECP_DP_XXX value 00262 * 00263 * \return The associated curve information or NULL 00264 */ 00265 const ecp_curve_info *ecp_curve_info_from_grp_id( ecp_group_id grp_id ); 00266 00267 /** 00268 * \brief Get curve information from a TLS NamedCurve value 00269 * 00270 * \param tls_id A POLARSSL_ECP_DP_XXX value 00271 * 00272 * \return The associated curve information or NULL 00273 */ 00274 const ecp_curve_info *ecp_curve_info_from_tls_id( uint16_t tls_id ); 00275 00276 /** 00277 * \brief Get curve information from a human-readable name 00278 * 00279 * \param name The name 00280 * 00281 * \return The associated curve information or NULL 00282 */ 00283 const ecp_curve_info *ecp_curve_info_from_name( const char *name ); 00284 00285 /** 00286 * \brief Initialize a point (as zero) 00287 */ 00288 void ecp_point_init( ecp_point *pt ); 00289 00290 /** 00291 * \brief Initialize a group (to something meaningless) 00292 */ 00293 void ecp_group_init( ecp_group *grp ); 00294 00295 /** 00296 * \brief Initialize a key pair (as an invalid one) 00297 */ 00298 void ecp_keypair_init( ecp_keypair *key ); 00299 00300 /** 00301 * \brief Free the components of a point 00302 */ 00303 void ecp_point_free( ecp_point *pt ); 00304 00305 /** 00306 * \brief Free the components of an ECP group 00307 */ 00308 void ecp_group_free( ecp_group *grp ); 00309 00310 /** 00311 * \brief Free the components of a key pair 00312 */ 00313 void ecp_keypair_free( ecp_keypair *key ); 00314 00315 /** 00316 * \brief Copy the contents of point Q into P 00317 * 00318 * \param P Destination point 00319 * \param Q Source point 00320 * 00321 * \return 0 if successful, 00322 * POLARSSL_ERR_MPI_MALLOC_FAILED if memory allocation failed 00323 */ 00324 int ecp_copy( ecp_point *P, const ecp_point *Q ); 00325 00326 /** 00327 * \brief Copy the contents of a group object 00328 * 00329 * \param dst Destination group 00330 * \param src Source group 00331 * 00332 * \return 0 if successful, 00333 * POLARSSL_ERR_MPI_MALLOC_FAILED if memory allocation failed 00334 */ 00335 int ecp_group_copy( ecp_group *dst, const ecp_group *src ); 00336 00337 /** 00338 * \brief Set a point to zero 00339 * 00340 * \param pt Destination point 00341 * 00342 * \return 0 if successful, 00343 * POLARSSL_ERR_MPI_MALLOC_FAILED if memory allocation failed 00344 */ 00345 int ecp_set_zero( ecp_point *pt ); 00346 00347 /** 00348 * \brief Tell if a point is zero 00349 * 00350 * \param pt Point to test 00351 * 00352 * \return 1 if point is zero, 0 otherwise 00353 */ 00354 int ecp_is_zero( ecp_point *pt ); 00355 00356 /** 00357 * \brief Import a non-zero point from two ASCII strings 00358 * 00359 * \param P Destination point 00360 * \param radix Input numeric base 00361 * \param x First affine coordinate as a null-terminated string 00362 * \param y Second affine coordinate as a null-terminated string 00363 * 00364 * \return 0 if successful, or a POLARSSL_ERR_MPI_XXX error code 00365 */ 00366 int ecp_point_read_string( ecp_point *P, int radix, 00367 const char *x, const char *y ); 00368 00369 /** 00370 * \brief Export a point into unsigned binary data 00371 * 00372 * \param grp Group to which the point should belong 00373 * \param P Point to export 00374 * \param format Point format, should be a POLARSSL_ECP_PF_XXX macro 00375 * \param olen Length of the actual output 00376 * \param buf Output buffer 00377 * \param buflen Length of the output buffer 00378 * 00379 * \return 0 if successful, 00380 * or POLARSSL_ERR_ECP_BAD_INPUT_DATA 00381 * or POLARSSL_ERR_ECP_BUFFER_TOO_SMALL 00382 */ 00383 int ecp_point_write_binary( const ecp_group *grp, const ecp_point *P, 00384 int format, size_t *olen, 00385 unsigned char *buf, size_t buflen ); 00386 00387 /** 00388 * \brief Import a point from unsigned binary data 00389 * 00390 * \param grp Group to which the point should belong 00391 * \param P Point to import 00392 * \param buf Input buffer 00393 * \param ilen Actual length of input 00394 * 00395 * \return 0 if successful, 00396 * POLARSSL_ERR_ECP_BAD_INPUT_DATA if input is invalid, 00397 * POLARSSL_ERR_MPI_MALLOC_FAILED if memory allocation failed, 00398 * POLARSSL_ERR_ECP_FEATURE_UNAVAILABLE if the point format 00399 * is not implemented. 00400 * 00401 * \note This function does NOT check that the point actually 00402 * belongs to the given group, see ecp_check_pubkey() for 00403 * that. 00404 */ 00405 int ecp_point_read_binary( const ecp_group *grp, ecp_point *P, 00406 const unsigned char *buf, size_t ilen ); 00407 00408 /** 00409 * \brief Import a point from a TLS ECPoint record 00410 * 00411 * \param grp ECP group used 00412 * \param pt Destination point 00413 * \param buf $(Start of input buffer) 00414 * \param len Buffer length 00415 * 00416 * \return O if successful, 00417 * POLARSSL_ERR_MPI_XXX if initialization failed 00418 * POLARSSL_ERR_ECP_BAD_INPUT_DATA if input is invalid 00419 */ 00420 int ecp_tls_read_point( const ecp_group *grp, ecp_point *pt, 00421 const unsigned char **buf, size_t len ); 00422 00423 /** 00424 * \brief Export a point as a TLS ECPoint record 00425 * 00426 * \param grp ECP group used 00427 * \param pt Point to export 00428 * \param format Export format 00429 * \param olen length of data written 00430 * \param buf Buffer to write to 00431 * \param blen Buffer length 00432 * 00433 * \return 0 if successful, 00434 * or POLARSSL_ERR_ECP_BAD_INPUT_DATA 00435 * or POLARSSL_ERR_ECP_BUFFER_TOO_SMALL 00436 */ 00437 int ecp_tls_write_point( const ecp_group *grp, const ecp_point *pt, 00438 int format, size_t *olen, 00439 unsigned char *buf, size_t blen ); 00440 00441 /** 00442 * \brief Import an ECP group from null-terminated ASCII strings 00443 * 00444 * \param grp Destination group 00445 * \param radix Input numeric base 00446 * \param p Prime modulus of the base field 00447 * \param b Constant term in the equation 00448 * \param gx The generator's X coordinate 00449 * \param gy The generator's Y coordinate 00450 * \param n The generator's order 00451 * 00452 * \return 0 if successful, or a POLARSSL_ERR_MPI_XXX error code 00453 * 00454 * \note Sets all fields except modp. 00455 */ 00456 int ecp_group_read_string( ecp_group *grp, int radix, 00457 const char *p, const char *b, 00458 const char *gx, const char *gy, const char *n); 00459 00460 /** 00461 * \brief Set a group using well-known domain parameters 00462 * 00463 * \param grp Destination group 00464 * \param index Index in the list of well-known domain parameters 00465 * 00466 * \return O if successful, 00467 * POLARSSL_ERR_MPI_XXX if initialization failed 00468 * POLARSSL_ERR_ECP_FEATURE_UNAVAILABLE for unkownn groups 00469 * 00470 * \note Index should be a value of RFC 4492's enum NamdeCurve, 00471 * possibly in the form of a POLARSSL_ECP_DP_XXX macro. 00472 */ 00473 int ecp_use_known_dp( ecp_group *grp, ecp_group_id index ); 00474 00475 /** 00476 * \brief Set a group from a TLS ECParameters record 00477 * 00478 * \param grp Destination group 00479 * \param buf &(Start of input buffer) 00480 * \param len Buffer length 00481 * 00482 * \return O if successful, 00483 * POLARSSL_ERR_MPI_XXX if initialization failed 00484 * POLARSSL_ERR_ECP_BAD_INPUT_DATA if input is invalid 00485 */ 00486 int ecp_tls_read_group( ecp_group *grp, const unsigned char **buf, size_t len ); 00487 00488 /** 00489 * \brief Write the TLS ECParameters record for a group 00490 * 00491 * \param grp ECP group used 00492 * \param olen Number of bytes actually written 00493 * \param buf Buffer to write to 00494 * \param blen Buffer length 00495 * 00496 * \return 0 if successful, 00497 * or POLARSSL_ERR_ECP_BUFFER_TOO_SMALL 00498 */ 00499 int ecp_tls_write_group( const ecp_group *grp, size_t *olen, 00500 unsigned char *buf, size_t blen ); 00501 00502 /** 00503 * \brief Addition: R = P + Q 00504 * 00505 * \param grp ECP group 00506 * \param R Destination point 00507 * \param P Left-hand point 00508 * \param Q Right-hand point 00509 * 00510 * \return 0 if successful, 00511 * POLARSSL_ERR_MPI_MALLOC_FAILED if memory allocation failed 00512 * 00513 * \note This function does not support Montgomery curves, such as 00514 * Curve25519. 00515 */ 00516 int ecp_add( const ecp_group *grp, ecp_point *R, 00517 const ecp_point *P, const ecp_point *Q ); 00518 00519 /** 00520 * \brief Subtraction: R = P - Q 00521 * 00522 * \param grp ECP group 00523 * \param R Destination point 00524 * \param P Left-hand point 00525 * \param Q Right-hand point 00526 * 00527 * \return 0 if successful, 00528 * POLARSSL_ERR_MPI_MALLOC_FAILED if memory allocation failed 00529 * 00530 * \note This function does not support Montgomery curves, such as 00531 * Curve25519. 00532 */ 00533 int ecp_sub( const ecp_group *grp, ecp_point *R, 00534 const ecp_point *P, const ecp_point *Q ); 00535 00536 /** 00537 * \brief Multiplication by an integer: R = m * P 00538 * (Not thread-safe to use same group in multiple threads) 00539 * 00540 * \param grp ECP group 00541 * \param R Destination point 00542 * \param m Integer by which to multiply 00543 * \param P Point to multiply 00544 * \param f_rng RNG function (see notes) 00545 * \param p_rng RNG parameter 00546 * 00547 * \return 0 if successful, 00548 * POLARSSL_ERR_ECP_INVALID_KEY if m is not a valid privkey 00549 * or P is not a valid pubkey, 00550 * POLARSSL_ERR_MPI_MALLOC_FAILED if memory allocation failed 00551 * 00552 * \note In order to prevent timing attacks, this function 00553 * executes the exact same sequence of (base field) 00554 * operations for any valid m. It avoids any if-branch or 00555 * array index depending on the value of m. 00556 * 00557 * \note If f_rng is not NULL, it is used to randomize intermediate 00558 * results in order to prevent potential timing attacks 00559 * targeting these results. It is recommended to always 00560 * provide a non-NULL f_rng (the overhead is negligible). 00561 */ 00562 int ecp_mul( ecp_group *grp, ecp_point *R, 00563 const mpi *m, const ecp_point *P, 00564 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ); 00565 00566 /** 00567 * \brief Check that a point is a valid public key on this curve 00568 * 00569 * \param grp Curve/group the point should belong to 00570 * \param pt Point to check 00571 * 00572 * \return 0 if point is a valid public key, 00573 * POLARSSL_ERR_ECP_INVALID_KEY otherwise. 00574 * 00575 * \note This function only checks the point is non-zero, has valid 00576 * coordinates and lies on the curve, but not that it is 00577 * indeed a multiple of G. This is additional check is more 00578 * expensive, isn't required by standards, and shouldn't be 00579 * necessary if the group used has a small cofactor. In 00580 * particular, it is useless for the NIST groups which all 00581 * have a cofactor of 1. 00582 * 00583 * \note Uses bare components rather than an ecp_keypair structure 00584 * in order to ease use with other structures such as 00585 * ecdh_context of ecdsa_context. 00586 */ 00587 int ecp_check_pubkey( const ecp_group *grp, const ecp_point *pt ); 00588 00589 /** 00590 * \brief Check that an mpi is a valid private key for this curve 00591 * 00592 * \param grp Group used 00593 * \param d Integer to check 00594 * 00595 * \return 0 if point is a valid private key, 00596 * POLARSSL_ERR_ECP_INVALID_KEY otherwise. 00597 * 00598 * \note Uses bare components rather than an ecp_keypair structure 00599 * in order to ease use with other structures such as 00600 * ecdh_context of ecdsa_context. 00601 */ 00602 int ecp_check_privkey( const ecp_group *grp, const mpi *d ); 00603 00604 /** 00605 * \brief Generate a keypair 00606 * 00607 * \param grp ECP group 00608 * \param d Destination MPI (secret part) 00609 * \param Q Destination point (public part) 00610 * \param f_rng RNG function 00611 * \param p_rng RNG parameter 00612 * 00613 * \return 0 if successful, 00614 * or a POLARSSL_ERR_ECP_XXX or POLARSSL_MPI_XXX error code 00615 * 00616 * \note Uses bare components rather than an ecp_keypair structure 00617 * in order to ease use with other structures such as 00618 * ecdh_context of ecdsa_context. 00619 */ 00620 int ecp_gen_keypair( ecp_group *grp, mpi *d, ecp_point *Q, 00621 int (*f_rng)(void *, unsigned char *, size_t), 00622 void *p_rng ); 00623 00624 /** 00625 * \brief Generate a keypair 00626 * 00627 * \param grp_id ECP group identifier 00628 * \param key Destination keypair 00629 * \param f_rng RNG function 00630 * \param p_rng RNG parameter 00631 * 00632 * \return 0 if successful, 00633 * or a POLARSSL_ERR_ECP_XXX or POLARSSL_MPI_XXX error code 00634 */ 00635 int ecp_gen_key( ecp_group_id grp_id, ecp_keypair *key, 00636 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ); 00637 00638 #if defined(POLARSSL_SELF_TEST) 00639 /** 00640 * \brief Checkup routine 00641 * 00642 * \return 0 if successful, or 1 if a test failed 00643 */ 00644 int ecp_self_test( int verbose ); 00645 #endif 00646 00647 #ifdef __cplusplus 00648 } 00649 #endif 00650 00651 #endif /* ecp.h */ 00652 00653
Generated on Tue Jul 12 2022 19:40:15 by
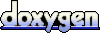