
Example program to test AES-GCM functionality. Used for a workshop
Embed:
(wiki syntax)
Show/hide line numbers
des.h
Go to the documentation of this file.
00001 /** 00002 * \file des.h 00003 * 00004 * \brief DES block cipher 00005 * 00006 * Copyright (C) 2006-2014, Brainspark B.V. 00007 * 00008 * This file is part of PolarSSL (http://www.polarssl.org) 00009 * Lead Maintainer: Paul Bakker <polarssl_maintainer at polarssl.org> 00010 * 00011 * All rights reserved. 00012 * 00013 * This program is free software; you can redistribute it and/or modify 00014 * it under the terms of the GNU General Public License as published by 00015 * the Free Software Foundation; either version 2 of the License, or 00016 * (at your option) any later version. 00017 * 00018 * This program is distributed in the hope that it will be useful, 00019 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00020 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00021 * GNU General Public License for more details. 00022 * 00023 * You should have received a copy of the GNU General Public License along 00024 * with this program; if not, write to the Free Software Foundation, Inc., 00025 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00026 */ 00027 #ifndef POLARSSL_DES_H 00028 #define POLARSSL_DES_H 00029 00030 #if !defined(POLARSSL_CONFIG_FILE) 00031 #include "config.h" 00032 #else 00033 #include POLARSSL_CONFIG_FILE 00034 #endif 00035 00036 #include <string.h> 00037 00038 #if defined(_MSC_VER) && !defined(EFIX64) && !defined(EFI32) 00039 #include <basetsd.h> 00040 typedef UINT32 uint32_t; 00041 #else 00042 #include <inttypes.h> 00043 #endif 00044 00045 #define DES_ENCRYPT 1 00046 #define DES_DECRYPT 0 00047 00048 #define POLARSSL_ERR_DES_INVALID_INPUT_LENGTH -0x0032 /**< The data input has an invalid length. */ 00049 00050 #define DES_KEY_SIZE 8 00051 00052 #if !defined(POLARSSL_DES_ALT) 00053 // Regular implementation 00054 // 00055 00056 #ifdef __cplusplus 00057 extern "C" { 00058 #endif 00059 00060 /** 00061 * \brief DES context structure 00062 */ 00063 typedef struct 00064 { 00065 int mode ; /*!< encrypt/decrypt */ 00066 uint32_t sk[32]; /*!< DES subkeys */ 00067 } 00068 des_context; 00069 00070 /** 00071 * \brief Triple-DES context structure 00072 */ 00073 typedef struct 00074 { 00075 int mode ; /*!< encrypt/decrypt */ 00076 uint32_t sk[96]; /*!< 3DES subkeys */ 00077 } 00078 des3_context; 00079 00080 /** 00081 * \brief Set key parity on the given key to odd. 00082 * 00083 * DES keys are 56 bits long, but each byte is padded with 00084 * a parity bit to allow verification. 00085 * 00086 * \param key 8-byte secret key 00087 */ 00088 void des_key_set_parity( unsigned char key[DES_KEY_SIZE] ); 00089 00090 /** 00091 * \brief Check that key parity on the given key is odd. 00092 * 00093 * DES keys are 56 bits long, but each byte is padded with 00094 * a parity bit to allow verification. 00095 * 00096 * \param key 8-byte secret key 00097 * 00098 * \return 0 is parity was ok, 1 if parity was not correct. 00099 */ 00100 int des_key_check_key_parity( const unsigned char key[DES_KEY_SIZE] ); 00101 00102 /** 00103 * \brief Check that key is not a weak or semi-weak DES key 00104 * 00105 * \param key 8-byte secret key 00106 * 00107 * \return 0 if no weak key was found, 1 if a weak key was identified. 00108 */ 00109 int des_key_check_weak( const unsigned char key[DES_KEY_SIZE] ); 00110 00111 /** 00112 * \brief DES key schedule (56-bit, encryption) 00113 * 00114 * \param ctx DES context to be initialized 00115 * \param key 8-byte secret key 00116 * 00117 * \return 0 00118 */ 00119 int des_setkey_enc( des_context *ctx, const unsigned char key[DES_KEY_SIZE] ); 00120 00121 /** 00122 * \brief DES key schedule (56-bit, decryption) 00123 * 00124 * \param ctx DES context to be initialized 00125 * \param key 8-byte secret key 00126 * 00127 * \return 0 00128 */ 00129 int des_setkey_dec( des_context *ctx, const unsigned char key[DES_KEY_SIZE] ); 00130 00131 /** 00132 * \brief Triple-DES key schedule (112-bit, encryption) 00133 * 00134 * \param ctx 3DES context to be initialized 00135 * \param key 16-byte secret key 00136 * 00137 * \return 0 00138 */ 00139 int des3_set2key_enc( des3_context *ctx, 00140 const unsigned char key[DES_KEY_SIZE * 2] ); 00141 00142 /** 00143 * \brief Triple-DES key schedule (112-bit, decryption) 00144 * 00145 * \param ctx 3DES context to be initialized 00146 * \param key 16-byte secret key 00147 * 00148 * \return 0 00149 */ 00150 int des3_set2key_dec( des3_context *ctx, 00151 const unsigned char key[DES_KEY_SIZE * 2] ); 00152 00153 /** 00154 * \brief Triple-DES key schedule (168-bit, encryption) 00155 * 00156 * \param ctx 3DES context to be initialized 00157 * \param key 24-byte secret key 00158 * 00159 * \return 0 00160 */ 00161 int des3_set3key_enc( des3_context *ctx, 00162 const unsigned char key[DES_KEY_SIZE * 3] ); 00163 00164 /** 00165 * \brief Triple-DES key schedule (168-bit, decryption) 00166 * 00167 * \param ctx 3DES context to be initialized 00168 * \param key 24-byte secret key 00169 * 00170 * \return 0 00171 */ 00172 int des3_set3key_dec( des3_context *ctx, 00173 const unsigned char key[DES_KEY_SIZE * 3] ); 00174 00175 /** 00176 * \brief DES-ECB block encryption/decryption 00177 * 00178 * \param ctx DES context 00179 * \param input 64-bit input block 00180 * \param output 64-bit output block 00181 * 00182 * \return 0 if successful 00183 */ 00184 int des_crypt_ecb( des_context *ctx, 00185 const unsigned char input[8], 00186 unsigned char output[8] ); 00187 00188 #if defined(POLARSSL_CIPHER_MODE_CBC) 00189 /** 00190 * \brief DES-CBC buffer encryption/decryption 00191 * 00192 * \param ctx DES context 00193 * \param mode DES_ENCRYPT or DES_DECRYPT 00194 * \param length length of the input data 00195 * \param iv initialization vector (updated after use) 00196 * \param input buffer holding the input data 00197 * \param output buffer holding the output data 00198 */ 00199 int des_crypt_cbc( des_context *ctx, 00200 int mode, 00201 size_t length, 00202 unsigned char iv[8], 00203 const unsigned char *input, 00204 unsigned char *output ); 00205 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00206 00207 /** 00208 * \brief 3DES-ECB block encryption/decryption 00209 * 00210 * \param ctx 3DES context 00211 * \param input 64-bit input block 00212 * \param output 64-bit output block 00213 * 00214 * \return 0 if successful 00215 */ 00216 int des3_crypt_ecb( des3_context *ctx, 00217 const unsigned char input[8], 00218 unsigned char output[8] ); 00219 00220 #if defined(POLARSSL_CIPHER_MODE_CBC) 00221 /** 00222 * \brief 3DES-CBC buffer encryption/decryption 00223 * 00224 * \param ctx 3DES context 00225 * \param mode DES_ENCRYPT or DES_DECRYPT 00226 * \param length length of the input data 00227 * \param iv initialization vector (updated after use) 00228 * \param input buffer holding the input data 00229 * \param output buffer holding the output data 00230 * 00231 * \return 0 if successful, or POLARSSL_ERR_DES_INVALID_INPUT_LENGTH 00232 */ 00233 int des3_crypt_cbc( des3_context *ctx, 00234 int mode, 00235 size_t length, 00236 unsigned char iv[8], 00237 const unsigned char *input, 00238 unsigned char *output ); 00239 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00240 00241 #ifdef __cplusplus 00242 } 00243 #endif 00244 00245 #else /* POLARSSL_DES_ALT */ 00246 #include "des_alt.h" 00247 #endif /* POLARSSL_DES_ALT */ 00248 00249 #ifdef __cplusplus 00250 extern "C" { 00251 #endif 00252 00253 /** 00254 * \brief Checkup routine 00255 * 00256 * \return 0 if successful, or 1 if the test failed 00257 */ 00258 int des_self_test( int verbose ); 00259 00260 #ifdef __cplusplus 00261 } 00262 #endif 00263 00264 #endif /* des.h */ 00265 00266
Generated on Tue Jul 12 2022 19:40:15 by
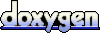