
Example program to test AES-GCM functionality. Used for a workshop
Embed:
(wiki syntax)
Show/hide line numbers
cipher_wrap.c
Go to the documentation of this file.
00001 /** 00002 * \file cipher_wrap.c 00003 * 00004 * \brief Generic cipher wrapper for PolarSSL 00005 * 00006 * \author Adriaan de Jong <dejong@fox-it.com> 00007 * 00008 * Copyright (C) 2006-2014, Brainspark B.V. 00009 * 00010 * This file is part of PolarSSL (http://www.polarssl.org) 00011 * Lead Maintainer: Paul Bakker <polarssl_maintainer at polarssl.org> 00012 * 00013 * All rights reserved. 00014 * 00015 * This program is free software; you can redistribute it and/or modify 00016 * it under the terms of the GNU General Public License as published by 00017 * the Free Software Foundation; either version 2 of the License, or 00018 * (at your option) any later version. 00019 * 00020 * This program is distributed in the hope that it will be useful, 00021 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00022 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00023 * GNU General Public License for more details. 00024 * 00025 * You should have received a copy of the GNU General Public License along 00026 * with this program; if not, write to the Free Software Foundation, Inc., 00027 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00028 */ 00029 00030 #if !defined(POLARSSL_CONFIG_FILE) 00031 #include "polarssl/config.h" 00032 #else 00033 #include POLARSSL_CONFIG_FILE 00034 #endif 00035 00036 #if defined(POLARSSL_CIPHER_C) 00037 00038 #include "polarssl/cipher_wrap.h" 00039 00040 #if defined(POLARSSL_AES_C) 00041 #include "polarssl/aes.h" 00042 #endif 00043 00044 #if defined(POLARSSL_ARC4_C) 00045 #include "polarssl/arc4.h" 00046 #endif 00047 00048 #if defined(POLARSSL_CAMELLIA_C) 00049 #include "polarssl/camellia.h" 00050 #endif 00051 00052 #if defined(POLARSSL_DES_C) 00053 #include "polarssl/des.h" 00054 #endif 00055 00056 #if defined(POLARSSL_BLOWFISH_C) 00057 #include "polarssl/blowfish.h" 00058 #endif 00059 00060 #if defined(POLARSSL_GCM_C) 00061 #include "polarssl/gcm.h" 00062 #endif 00063 00064 #if defined(POLARSSL_PLATFORM_C) 00065 #include "polarssl/platform.h" 00066 #else 00067 #define polarssl_malloc malloc 00068 #define polarssl_free free 00069 #endif 00070 00071 #include <stdlib.h> 00072 00073 #if defined(POLARSSL_GCM_C) 00074 /* shared by all GCM ciphers */ 00075 static void *gcm_ctx_alloc( void ) 00076 { 00077 return polarssl_malloc( sizeof( gcm_context ) ); 00078 } 00079 00080 static void gcm_ctx_free( void *ctx ) 00081 { 00082 gcm_free( ctx ); 00083 polarssl_free( ctx ); 00084 } 00085 #endif /* POLARSSL_GCM_C */ 00086 00087 #if defined(POLARSSL_AES_C) 00088 00089 static int aes_crypt_ecb_wrap( void *ctx, operation_t operation, 00090 const unsigned char *input, unsigned char *output ) 00091 { 00092 return aes_crypt_ecb( (aes_context *) ctx, operation, input, output ); 00093 } 00094 00095 static int aes_crypt_cbc_wrap( void *ctx, operation_t operation, size_t length, 00096 unsigned char *iv, const unsigned char *input, unsigned char *output ) 00097 { 00098 #if defined(POLARSSL_CIPHER_MODE_CBC) 00099 return aes_crypt_cbc( (aes_context *) ctx, operation, length, iv, input, 00100 output ); 00101 #else 00102 ((void) ctx); 00103 ((void) operation); 00104 ((void) length); 00105 ((void) iv); 00106 ((void) input); 00107 ((void) output); 00108 00109 return POLARSSL_ERR_CIPHER_FEATURE_UNAVAILABLE; 00110 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00111 } 00112 00113 static int aes_crypt_cfb128_wrap( void *ctx, operation_t operation, 00114 size_t length, size_t *iv_off, unsigned char *iv, 00115 const unsigned char *input, unsigned char *output ) 00116 { 00117 #if defined(POLARSSL_CIPHER_MODE_CFB) 00118 return aes_crypt_cfb128( (aes_context *) ctx, operation, length, iv_off, iv, 00119 input, output ); 00120 #else 00121 ((void) ctx); 00122 ((void) operation); 00123 ((void) length); 00124 ((void) iv_off); 00125 ((void) iv); 00126 ((void) input); 00127 ((void) output); 00128 00129 return POLARSSL_ERR_CIPHER_FEATURE_UNAVAILABLE; 00130 #endif /* POLARSSL_CIPHER_MODE_CFB */ 00131 } 00132 00133 static int aes_crypt_ctr_wrap( void *ctx, size_t length, size_t *nc_off, 00134 unsigned char *nonce_counter, unsigned char *stream_block, 00135 const unsigned char *input, unsigned char *output ) 00136 { 00137 #if defined(POLARSSL_CIPHER_MODE_CTR) 00138 return aes_crypt_ctr( (aes_context *) ctx, length, nc_off, nonce_counter, 00139 stream_block, input, output ); 00140 #else 00141 ((void) ctx); 00142 ((void) length); 00143 ((void) nc_off); 00144 ((void) nonce_counter); 00145 ((void) stream_block); 00146 ((void) input); 00147 ((void) output); 00148 00149 return POLARSSL_ERR_CIPHER_FEATURE_UNAVAILABLE; 00150 #endif /* POLARSSL_CIPHER_MODE_CTR */ 00151 } 00152 00153 static int aes_setkey_dec_wrap( void *ctx, const unsigned char *key, 00154 unsigned int key_length ) 00155 { 00156 return aes_setkey_dec( (aes_context *) ctx, key, key_length ); 00157 } 00158 00159 static int aes_setkey_enc_wrap( void *ctx, const unsigned char *key, 00160 unsigned int key_length ) 00161 { 00162 return aes_setkey_enc( (aes_context *) ctx, key, key_length ); 00163 } 00164 00165 static void * aes_ctx_alloc( void ) 00166 { 00167 return polarssl_malloc( sizeof( aes_context ) ); 00168 } 00169 00170 static void aes_ctx_free( void *ctx ) 00171 { 00172 polarssl_free( ctx ); 00173 } 00174 00175 const cipher_base_t aes_info = { 00176 POLARSSL_CIPHER_ID_AES, 00177 aes_crypt_ecb_wrap, 00178 aes_crypt_cbc_wrap, 00179 aes_crypt_cfb128_wrap, 00180 aes_crypt_ctr_wrap, 00181 NULL, 00182 aes_setkey_enc_wrap, 00183 aes_setkey_dec_wrap, 00184 aes_ctx_alloc, 00185 aes_ctx_free 00186 }; 00187 00188 const cipher_info_t aes_128_ecb_info = { 00189 POLARSSL_CIPHER_AES_128_ECB, 00190 POLARSSL_MODE_ECB, 00191 128, 00192 "AES-128-ECB", 00193 16, 00194 0, 00195 16, 00196 &aes_info 00197 }; 00198 00199 const cipher_info_t aes_192_ecb_info = { 00200 POLARSSL_CIPHER_AES_192_ECB, 00201 POLARSSL_MODE_ECB, 00202 192, 00203 "AES-192-ECB", 00204 16, 00205 0, 00206 16, 00207 &aes_info 00208 }; 00209 00210 const cipher_info_t aes_256_ecb_info = { 00211 POLARSSL_CIPHER_AES_256_ECB, 00212 POLARSSL_MODE_ECB, 00213 256, 00214 "AES-256-ECB", 00215 16, 00216 0, 00217 16, 00218 &aes_info 00219 }; 00220 00221 #if defined(POLARSSL_CIPHER_MODE_CBC) 00222 const cipher_info_t aes_128_cbc_info = { 00223 POLARSSL_CIPHER_AES_128_CBC, 00224 POLARSSL_MODE_CBC, 00225 128, 00226 "AES-128-CBC", 00227 16, 00228 0, 00229 16, 00230 &aes_info 00231 }; 00232 00233 const cipher_info_t aes_192_cbc_info = { 00234 POLARSSL_CIPHER_AES_192_CBC, 00235 POLARSSL_MODE_CBC, 00236 192, 00237 "AES-192-CBC", 00238 16, 00239 0, 00240 16, 00241 &aes_info 00242 }; 00243 00244 const cipher_info_t aes_256_cbc_info = { 00245 POLARSSL_CIPHER_AES_256_CBC, 00246 POLARSSL_MODE_CBC, 00247 256, 00248 "AES-256-CBC", 00249 16, 00250 0, 00251 16, 00252 &aes_info 00253 }; 00254 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00255 00256 #if defined(POLARSSL_CIPHER_MODE_CFB) 00257 const cipher_info_t aes_128_cfb128_info = { 00258 POLARSSL_CIPHER_AES_128_CFB128, 00259 POLARSSL_MODE_CFB, 00260 128, 00261 "AES-128-CFB128", 00262 16, 00263 0, 00264 16, 00265 &aes_info 00266 }; 00267 00268 const cipher_info_t aes_192_cfb128_info = { 00269 POLARSSL_CIPHER_AES_192_CFB128, 00270 POLARSSL_MODE_CFB, 00271 192, 00272 "AES-192-CFB128", 00273 16, 00274 0, 00275 16, 00276 &aes_info 00277 }; 00278 00279 const cipher_info_t aes_256_cfb128_info = { 00280 POLARSSL_CIPHER_AES_256_CFB128, 00281 POLARSSL_MODE_CFB, 00282 256, 00283 "AES-256-CFB128", 00284 16, 00285 0, 00286 16, 00287 &aes_info 00288 }; 00289 #endif /* POLARSSL_CIPHER_MODE_CFB */ 00290 00291 #if defined(POLARSSL_CIPHER_MODE_CTR) 00292 const cipher_info_t aes_128_ctr_info = { 00293 POLARSSL_CIPHER_AES_128_CTR, 00294 POLARSSL_MODE_CTR, 00295 128, 00296 "AES-128-CTR", 00297 16, 00298 0, 00299 16, 00300 &aes_info 00301 }; 00302 00303 const cipher_info_t aes_192_ctr_info = { 00304 POLARSSL_CIPHER_AES_192_CTR, 00305 POLARSSL_MODE_CTR, 00306 192, 00307 "AES-192-CTR", 00308 16, 00309 0, 00310 16, 00311 &aes_info 00312 }; 00313 00314 const cipher_info_t aes_256_ctr_info = { 00315 POLARSSL_CIPHER_AES_256_CTR, 00316 POLARSSL_MODE_CTR, 00317 256, 00318 "AES-256-CTR", 00319 16, 00320 0, 00321 16, 00322 &aes_info 00323 }; 00324 #endif /* POLARSSL_CIPHER_MODE_CTR */ 00325 00326 #if defined(POLARSSL_GCM_C) 00327 static int gcm_aes_setkey_wrap( void *ctx, const unsigned char *key, 00328 unsigned int key_length ) 00329 { 00330 return gcm_init( (gcm_context *) ctx, POLARSSL_CIPHER_ID_AES, 00331 key, key_length ); 00332 } 00333 00334 const cipher_base_t gcm_aes_info = { 00335 POLARSSL_CIPHER_ID_AES, 00336 NULL, 00337 NULL, 00338 NULL, 00339 NULL, 00340 NULL, 00341 gcm_aes_setkey_wrap, 00342 gcm_aes_setkey_wrap, 00343 gcm_ctx_alloc, 00344 gcm_ctx_free, 00345 }; 00346 00347 const cipher_info_t aes_128_gcm_info = { 00348 POLARSSL_CIPHER_AES_128_GCM, 00349 POLARSSL_MODE_GCM, 00350 128, 00351 "AES-128-GCM", 00352 12, 00353 1, 00354 16, 00355 &gcm_aes_info 00356 }; 00357 00358 const cipher_info_t aes_192_gcm_info = { 00359 POLARSSL_CIPHER_AES_192_GCM, 00360 POLARSSL_MODE_GCM, 00361 192, 00362 "AES-192-GCM", 00363 12, 00364 1, 00365 16, 00366 &gcm_aes_info 00367 }; 00368 00369 const cipher_info_t aes_256_gcm_info = { 00370 POLARSSL_CIPHER_AES_256_GCM, 00371 POLARSSL_MODE_GCM, 00372 256, 00373 "AES-256-GCM", 00374 12, 00375 1, 00376 16, 00377 &gcm_aes_info 00378 }; 00379 #endif /* POLARSSL_GCM_C */ 00380 00381 #endif /* POLARSSL_AES_C */ 00382 00383 #if defined(POLARSSL_CAMELLIA_C) 00384 00385 static int camellia_crypt_ecb_wrap( void *ctx, operation_t operation, 00386 const unsigned char *input, unsigned char *output ) 00387 { 00388 return camellia_crypt_ecb( (camellia_context *) ctx, operation, input, 00389 output ); 00390 } 00391 00392 static int camellia_crypt_cbc_wrap( void *ctx, operation_t operation, 00393 size_t length, unsigned char *iv, 00394 const unsigned char *input, unsigned char *output ) 00395 { 00396 #if defined(POLARSSL_CIPHER_MODE_CBC) 00397 return camellia_crypt_cbc( (camellia_context *) ctx, operation, length, iv, 00398 input, output ); 00399 #else 00400 ((void) ctx); 00401 ((void) operation); 00402 ((void) length); 00403 ((void) iv); 00404 ((void) input); 00405 ((void) output); 00406 00407 return POLARSSL_ERR_CIPHER_FEATURE_UNAVAILABLE; 00408 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00409 } 00410 00411 static int camellia_crypt_cfb128_wrap( void *ctx, operation_t operation, 00412 size_t length, size_t *iv_off, unsigned char *iv, 00413 const unsigned char *input, unsigned char *output ) 00414 { 00415 #if defined(POLARSSL_CIPHER_MODE_CFB) 00416 return camellia_crypt_cfb128( (camellia_context *) ctx, operation, length, 00417 iv_off, iv, input, output ); 00418 #else 00419 ((void) ctx); 00420 ((void) operation); 00421 ((void) length); 00422 ((void) iv_off); 00423 ((void) iv); 00424 ((void) input); 00425 ((void) output); 00426 00427 return POLARSSL_ERR_CIPHER_FEATURE_UNAVAILABLE; 00428 #endif /* POLARSSL_CIPHER_MODE_CFB */ 00429 } 00430 00431 static int camellia_crypt_ctr_wrap( void *ctx, size_t length, size_t *nc_off, 00432 unsigned char *nonce_counter, unsigned char *stream_block, 00433 const unsigned char *input, unsigned char *output ) 00434 { 00435 #if defined(POLARSSL_CIPHER_MODE_CTR) 00436 return camellia_crypt_ctr( (camellia_context *) ctx, length, nc_off, 00437 nonce_counter, stream_block, input, output ); 00438 #else 00439 ((void) ctx); 00440 ((void) length); 00441 ((void) nc_off); 00442 ((void) nonce_counter); 00443 ((void) stream_block); 00444 ((void) input); 00445 ((void) output); 00446 00447 return POLARSSL_ERR_CIPHER_FEATURE_UNAVAILABLE; 00448 #endif /* POLARSSL_CIPHER_MODE_CTR */ 00449 } 00450 00451 static int camellia_setkey_dec_wrap( void *ctx, const unsigned char *key, 00452 unsigned int key_length ) 00453 { 00454 return camellia_setkey_dec( (camellia_context *) ctx, key, key_length ); 00455 } 00456 00457 static int camellia_setkey_enc_wrap( void *ctx, const unsigned char *key, 00458 unsigned int key_length ) 00459 { 00460 return camellia_setkey_enc( (camellia_context *) ctx, key, key_length ); 00461 } 00462 00463 static void * camellia_ctx_alloc( void ) 00464 { 00465 return polarssl_malloc( sizeof( camellia_context ) ); 00466 } 00467 00468 static void camellia_ctx_free( void *ctx ) 00469 { 00470 polarssl_free( ctx ); 00471 } 00472 00473 const cipher_base_t camellia_info = { 00474 POLARSSL_CIPHER_ID_CAMELLIA, 00475 camellia_crypt_ecb_wrap, 00476 camellia_crypt_cbc_wrap, 00477 camellia_crypt_cfb128_wrap, 00478 camellia_crypt_ctr_wrap, 00479 NULL, 00480 camellia_setkey_enc_wrap, 00481 camellia_setkey_dec_wrap, 00482 camellia_ctx_alloc, 00483 camellia_ctx_free 00484 }; 00485 00486 const cipher_info_t camellia_128_ecb_info = { 00487 POLARSSL_CIPHER_CAMELLIA_128_ECB, 00488 POLARSSL_MODE_ECB, 00489 128, 00490 "CAMELLIA-128-ECB", 00491 16, 00492 0, 00493 16, 00494 &camellia_info 00495 }; 00496 00497 const cipher_info_t camellia_192_ecb_info = { 00498 POLARSSL_CIPHER_CAMELLIA_192_ECB, 00499 POLARSSL_MODE_ECB, 00500 192, 00501 "CAMELLIA-192-ECB", 00502 16, 00503 0, 00504 16, 00505 &camellia_info 00506 }; 00507 00508 const cipher_info_t camellia_256_ecb_info = { 00509 POLARSSL_CIPHER_CAMELLIA_256_ECB, 00510 POLARSSL_MODE_ECB, 00511 256, 00512 "CAMELLIA-256-ECB", 00513 16, 00514 0, 00515 16, 00516 &camellia_info 00517 }; 00518 00519 #if defined(POLARSSL_CIPHER_MODE_CBC) 00520 const cipher_info_t camellia_128_cbc_info = { 00521 POLARSSL_CIPHER_CAMELLIA_128_CBC, 00522 POLARSSL_MODE_CBC, 00523 128, 00524 "CAMELLIA-128-CBC", 00525 16, 00526 0, 00527 16, 00528 &camellia_info 00529 }; 00530 00531 const cipher_info_t camellia_192_cbc_info = { 00532 POLARSSL_CIPHER_CAMELLIA_192_CBC, 00533 POLARSSL_MODE_CBC, 00534 192, 00535 "CAMELLIA-192-CBC", 00536 16, 00537 0, 00538 16, 00539 &camellia_info 00540 }; 00541 00542 const cipher_info_t camellia_256_cbc_info = { 00543 POLARSSL_CIPHER_CAMELLIA_256_CBC, 00544 POLARSSL_MODE_CBC, 00545 256, 00546 "CAMELLIA-256-CBC", 00547 16, 00548 0, 00549 16, 00550 &camellia_info 00551 }; 00552 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00553 00554 #if defined(POLARSSL_CIPHER_MODE_CFB) 00555 const cipher_info_t camellia_128_cfb128_info = { 00556 POLARSSL_CIPHER_CAMELLIA_128_CFB128, 00557 POLARSSL_MODE_CFB, 00558 128, 00559 "CAMELLIA-128-CFB128", 00560 16, 00561 0, 00562 16, 00563 &camellia_info 00564 }; 00565 00566 const cipher_info_t camellia_192_cfb128_info = { 00567 POLARSSL_CIPHER_CAMELLIA_192_CFB128, 00568 POLARSSL_MODE_CFB, 00569 192, 00570 "CAMELLIA-192-CFB128", 00571 16, 00572 0, 00573 16, 00574 &camellia_info 00575 }; 00576 00577 const cipher_info_t camellia_256_cfb128_info = { 00578 POLARSSL_CIPHER_CAMELLIA_256_CFB128, 00579 POLARSSL_MODE_CFB, 00580 256, 00581 "CAMELLIA-256-CFB128", 00582 16, 00583 0, 00584 16, 00585 &camellia_info 00586 }; 00587 #endif /* POLARSSL_CIPHER_MODE_CFB */ 00588 00589 #if defined(POLARSSL_CIPHER_MODE_CTR) 00590 const cipher_info_t camellia_128_ctr_info = { 00591 POLARSSL_CIPHER_CAMELLIA_128_CTR, 00592 POLARSSL_MODE_CTR, 00593 128, 00594 "CAMELLIA-128-CTR", 00595 16, 00596 0, 00597 16, 00598 &camellia_info 00599 }; 00600 00601 const cipher_info_t camellia_192_ctr_info = { 00602 POLARSSL_CIPHER_CAMELLIA_192_CTR, 00603 POLARSSL_MODE_CTR, 00604 192, 00605 "CAMELLIA-192-CTR", 00606 16, 00607 0, 00608 16, 00609 &camellia_info 00610 }; 00611 00612 const cipher_info_t camellia_256_ctr_info = { 00613 POLARSSL_CIPHER_CAMELLIA_256_CTR, 00614 POLARSSL_MODE_CTR, 00615 256, 00616 "CAMELLIA-256-CTR", 00617 16, 00618 0, 00619 16, 00620 &camellia_info 00621 }; 00622 #endif /* POLARSSL_CIPHER_MODE_CTR */ 00623 00624 #if defined(POLARSSL_GCM_C) 00625 static int gcm_camellia_setkey_wrap( void *ctx, const unsigned char *key, 00626 unsigned int key_length ) 00627 { 00628 return gcm_init( (gcm_context *) ctx, POLARSSL_CIPHER_ID_CAMELLIA, 00629 key, key_length ); 00630 } 00631 00632 const cipher_base_t gcm_camellia_info = { 00633 POLARSSL_CIPHER_ID_CAMELLIA, 00634 NULL, 00635 NULL, 00636 NULL, 00637 NULL, 00638 NULL, 00639 gcm_camellia_setkey_wrap, 00640 gcm_camellia_setkey_wrap, 00641 gcm_ctx_alloc, 00642 gcm_ctx_free, 00643 }; 00644 00645 const cipher_info_t camellia_128_gcm_info = { 00646 POLARSSL_CIPHER_CAMELLIA_128_GCM, 00647 POLARSSL_MODE_GCM, 00648 128, 00649 "CAMELLIA-128-GCM", 00650 12, 00651 1, 00652 16, 00653 &gcm_camellia_info 00654 }; 00655 00656 const cipher_info_t camellia_192_gcm_info = { 00657 POLARSSL_CIPHER_CAMELLIA_192_GCM, 00658 POLARSSL_MODE_GCM, 00659 192, 00660 "CAMELLIA-192-GCM", 00661 12, 00662 1, 00663 16, 00664 &gcm_camellia_info 00665 }; 00666 00667 const cipher_info_t camellia_256_gcm_info = { 00668 POLARSSL_CIPHER_CAMELLIA_256_GCM, 00669 POLARSSL_MODE_GCM, 00670 256, 00671 "CAMELLIA-256-GCM", 00672 12, 00673 1, 00674 16, 00675 &gcm_camellia_info 00676 }; 00677 #endif /* POLARSSL_GCM_C */ 00678 00679 #endif /* POLARSSL_CAMELLIA_C */ 00680 00681 #if defined(POLARSSL_DES_C) 00682 00683 static int des_crypt_ecb_wrap( void *ctx, operation_t operation, 00684 const unsigned char *input, unsigned char *output ) 00685 { 00686 ((void) operation); 00687 return des_crypt_ecb( (des_context *) ctx, input, output ); 00688 } 00689 00690 static int des3_crypt_ecb_wrap( void *ctx, operation_t operation, 00691 const unsigned char *input, unsigned char *output ) 00692 { 00693 ((void) operation); 00694 return des3_crypt_ecb( (des3_context *) ctx, input, output ); 00695 } 00696 00697 static int des_crypt_cbc_wrap( void *ctx, operation_t operation, size_t length, 00698 unsigned char *iv, const unsigned char *input, unsigned char *output ) 00699 { 00700 #if defined(POLARSSL_CIPHER_MODE_CBC) 00701 return des_crypt_cbc( (des_context *) ctx, operation, length, iv, input, 00702 output ); 00703 #else 00704 ((void) ctx); 00705 ((void) operation); 00706 ((void) length); 00707 ((void) iv); 00708 ((void) input); 00709 ((void) output); 00710 00711 return POLARSSL_ERR_CIPHER_FEATURE_UNAVAILABLE; 00712 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00713 } 00714 00715 static int des3_crypt_cbc_wrap( void *ctx, operation_t operation, size_t length, 00716 unsigned char *iv, const unsigned char *input, unsigned char *output ) 00717 { 00718 #if defined(POLARSSL_CIPHER_MODE_CBC) 00719 return des3_crypt_cbc( (des3_context *) ctx, operation, length, iv, input, 00720 output ); 00721 #else 00722 ((void) ctx); 00723 ((void) operation); 00724 ((void) length); 00725 ((void) iv); 00726 ((void) input); 00727 ((void) output); 00728 00729 return POLARSSL_ERR_CIPHER_FEATURE_UNAVAILABLE; 00730 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00731 } 00732 00733 static int des_crypt_cfb128_wrap( void *ctx, operation_t operation, 00734 size_t length, size_t *iv_off, unsigned char *iv, 00735 const unsigned char *input, unsigned char *output ) 00736 { 00737 ((void) ctx); 00738 ((void) operation); 00739 ((void) length); 00740 ((void) iv_off); 00741 ((void) iv); 00742 ((void) input); 00743 ((void) output); 00744 00745 return POLARSSL_ERR_CIPHER_FEATURE_UNAVAILABLE; 00746 } 00747 00748 static int des_crypt_ctr_wrap( void *ctx, size_t length, size_t *nc_off, 00749 unsigned char *nonce_counter, unsigned char *stream_block, 00750 const unsigned char *input, unsigned char *output ) 00751 { 00752 ((void) ctx); 00753 ((void) length); 00754 ((void) nc_off); 00755 ((void) nonce_counter); 00756 ((void) stream_block); 00757 ((void) input); 00758 ((void) output); 00759 00760 return POLARSSL_ERR_CIPHER_FEATURE_UNAVAILABLE; 00761 } 00762 00763 static int des_setkey_dec_wrap( void *ctx, const unsigned char *key, 00764 unsigned int key_length ) 00765 { 00766 ((void) key_length); 00767 00768 return des_setkey_dec( (des_context *) ctx, key ); 00769 } 00770 00771 static int des_setkey_enc_wrap( void *ctx, const unsigned char *key, 00772 unsigned int key_length ) 00773 { 00774 ((void) key_length); 00775 00776 return des_setkey_enc( (des_context *) ctx, key ); 00777 } 00778 00779 static int des3_set2key_dec_wrap( void *ctx, const unsigned char *key, 00780 unsigned int key_length ) 00781 { 00782 ((void) key_length); 00783 00784 return des3_set2key_dec( (des3_context *) ctx, key ); 00785 } 00786 00787 static int des3_set2key_enc_wrap( void *ctx, const unsigned char *key, 00788 unsigned int key_length ) 00789 { 00790 ((void) key_length); 00791 00792 return des3_set2key_enc( (des3_context *) ctx, key ); 00793 } 00794 00795 static int des3_set3key_dec_wrap( void *ctx, const unsigned char *key, 00796 unsigned int key_length ) 00797 { 00798 ((void) key_length); 00799 00800 return des3_set3key_dec( (des3_context *) ctx, key ); 00801 } 00802 00803 static int des3_set3key_enc_wrap( void *ctx, const unsigned char *key, 00804 unsigned int key_length ) 00805 { 00806 ((void) key_length); 00807 00808 return des3_set3key_enc( (des3_context *) ctx, key ); 00809 } 00810 00811 static void * des_ctx_alloc( void ) 00812 { 00813 return polarssl_malloc( sizeof( des_context ) ); 00814 } 00815 00816 static void * des3_ctx_alloc( void ) 00817 { 00818 return polarssl_malloc( sizeof( des3_context ) ); 00819 } 00820 00821 static void des_ctx_free( void *ctx ) 00822 { 00823 polarssl_free( ctx ); 00824 } 00825 00826 const cipher_base_t des_info = { 00827 POLARSSL_CIPHER_ID_DES, 00828 des_crypt_ecb_wrap, 00829 des_crypt_cbc_wrap, 00830 des_crypt_cfb128_wrap, 00831 des_crypt_ctr_wrap, 00832 NULL, 00833 des_setkey_enc_wrap, 00834 des_setkey_dec_wrap, 00835 des_ctx_alloc, 00836 des_ctx_free 00837 }; 00838 00839 const cipher_info_t des_ecb_info = { 00840 POLARSSL_CIPHER_DES_ECB, 00841 POLARSSL_MODE_ECB, 00842 POLARSSL_KEY_LENGTH_DES, 00843 "DES-ECB", 00844 8, 00845 0, 00846 8, 00847 &des_info 00848 }; 00849 00850 #if defined(POLARSSL_CIPHER_MODE_CBC) 00851 const cipher_info_t des_cbc_info = { 00852 POLARSSL_CIPHER_DES_CBC, 00853 POLARSSL_MODE_CBC, 00854 POLARSSL_KEY_LENGTH_DES, 00855 "DES-CBC", 00856 8, 00857 0, 00858 8, 00859 &des_info 00860 }; 00861 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00862 00863 const cipher_base_t des_ede_info = { 00864 POLARSSL_CIPHER_ID_DES, 00865 des3_crypt_ecb_wrap, 00866 des3_crypt_cbc_wrap, 00867 des_crypt_cfb128_wrap, 00868 des_crypt_ctr_wrap, 00869 NULL, 00870 des3_set2key_enc_wrap, 00871 des3_set2key_dec_wrap, 00872 des3_ctx_alloc, 00873 des_ctx_free 00874 }; 00875 00876 const cipher_info_t des_ede_ecb_info = { 00877 POLARSSL_CIPHER_DES_EDE_ECB, 00878 POLARSSL_MODE_ECB, 00879 POLARSSL_KEY_LENGTH_DES_EDE, 00880 "DES-EDE-ECB", 00881 8, 00882 0, 00883 8, 00884 &des_ede_info 00885 }; 00886 00887 #if defined(POLARSSL_CIPHER_MODE_CBC) 00888 const cipher_info_t des_ede_cbc_info = { 00889 POLARSSL_CIPHER_DES_EDE_CBC, 00890 POLARSSL_MODE_CBC, 00891 POLARSSL_KEY_LENGTH_DES_EDE, 00892 "DES-EDE-CBC", 00893 8, 00894 0, 00895 8, 00896 &des_ede_info 00897 }; 00898 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00899 00900 const cipher_base_t des_ede3_info = { 00901 POLARSSL_CIPHER_ID_DES, 00902 des3_crypt_ecb_wrap, 00903 des3_crypt_cbc_wrap, 00904 des_crypt_cfb128_wrap, 00905 des_crypt_ctr_wrap, 00906 NULL, 00907 des3_set3key_enc_wrap, 00908 des3_set3key_dec_wrap, 00909 des3_ctx_alloc, 00910 des_ctx_free 00911 }; 00912 00913 const cipher_info_t des_ede3_ecb_info = { 00914 POLARSSL_CIPHER_DES_EDE3_ECB, 00915 POLARSSL_MODE_ECB, 00916 POLARSSL_KEY_LENGTH_DES_EDE3, 00917 "DES-EDE3-ECB", 00918 8, 00919 0, 00920 8, 00921 &des_ede3_info 00922 }; 00923 #if defined(POLARSSL_CIPHER_MODE_CBC) 00924 const cipher_info_t des_ede3_cbc_info = { 00925 POLARSSL_CIPHER_DES_EDE3_CBC, 00926 POLARSSL_MODE_CBC, 00927 POLARSSL_KEY_LENGTH_DES_EDE3, 00928 "DES-EDE3-CBC", 00929 8, 00930 0, 00931 8, 00932 &des_ede3_info 00933 }; 00934 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00935 #endif /* POLARSSL_DES_C */ 00936 00937 #if defined(POLARSSL_BLOWFISH_C) 00938 00939 static int blowfish_crypt_ecb_wrap( void *ctx, operation_t operation, 00940 const unsigned char *input, unsigned char *output ) 00941 { 00942 return blowfish_crypt_ecb( (blowfish_context *) ctx, operation, input, 00943 output ); 00944 } 00945 00946 static int blowfish_crypt_cbc_wrap( void *ctx, operation_t operation, 00947 size_t length, unsigned char *iv, const unsigned char *input, 00948 unsigned char *output ) 00949 { 00950 #if defined(POLARSSL_CIPHER_MODE_CBC) 00951 return blowfish_crypt_cbc( (blowfish_context *) ctx, operation, length, iv, 00952 input, output ); 00953 #else 00954 ((void) ctx); 00955 ((void) operation); 00956 ((void) length); 00957 ((void) iv); 00958 ((void) input); 00959 ((void) output); 00960 00961 return POLARSSL_ERR_CIPHER_FEATURE_UNAVAILABLE; 00962 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00963 } 00964 00965 static int blowfish_crypt_cfb64_wrap( void *ctx, operation_t operation, 00966 size_t length, size_t *iv_off, unsigned char *iv, 00967 const unsigned char *input, unsigned char *output ) 00968 { 00969 #if defined(POLARSSL_CIPHER_MODE_CFB) 00970 return blowfish_crypt_cfb64( (blowfish_context *) ctx, operation, length, 00971 iv_off, iv, input, output ); 00972 #else 00973 ((void) ctx); 00974 ((void) operation); 00975 ((void) length); 00976 ((void) iv_off); 00977 ((void) iv); 00978 ((void) input); 00979 ((void) output); 00980 00981 return POLARSSL_ERR_CIPHER_FEATURE_UNAVAILABLE; 00982 #endif /* POLARSSL_CIPHER_MODE_CFB */ 00983 } 00984 00985 static int blowfish_crypt_ctr_wrap( void *ctx, size_t length, size_t *nc_off, 00986 unsigned char *nonce_counter, unsigned char *stream_block, 00987 const unsigned char *input, unsigned char *output ) 00988 { 00989 #if defined(POLARSSL_CIPHER_MODE_CTR) 00990 return blowfish_crypt_ctr( (blowfish_context *) ctx, length, nc_off, 00991 nonce_counter, stream_block, input, output ); 00992 #else 00993 ((void) ctx); 00994 ((void) length); 00995 ((void) nc_off); 00996 ((void) nonce_counter); 00997 ((void) stream_block); 00998 ((void) input); 00999 ((void) output); 01000 01001 return POLARSSL_ERR_CIPHER_FEATURE_UNAVAILABLE; 01002 #endif /* POLARSSL_CIPHER_MODE_CTR */ 01003 } 01004 01005 static int blowfish_setkey_wrap( void *ctx, const unsigned char *key, 01006 unsigned int key_length ) 01007 { 01008 return blowfish_setkey( (blowfish_context *) ctx, key, key_length ); 01009 } 01010 01011 static void * blowfish_ctx_alloc( void ) 01012 { 01013 return polarssl_malloc( sizeof( blowfish_context ) ); 01014 } 01015 01016 static void blowfish_ctx_free( void *ctx ) 01017 { 01018 polarssl_free( ctx ); 01019 } 01020 01021 const cipher_base_t blowfish_info = { 01022 POLARSSL_CIPHER_ID_BLOWFISH, 01023 blowfish_crypt_ecb_wrap, 01024 blowfish_crypt_cbc_wrap, 01025 blowfish_crypt_cfb64_wrap, 01026 blowfish_crypt_ctr_wrap, 01027 NULL, 01028 blowfish_setkey_wrap, 01029 blowfish_setkey_wrap, 01030 blowfish_ctx_alloc, 01031 blowfish_ctx_free 01032 }; 01033 01034 const cipher_info_t blowfish_ecb_info = { 01035 POLARSSL_CIPHER_BLOWFISH_ECB, 01036 POLARSSL_MODE_ECB, 01037 128, 01038 "BLOWFISH-ECB", 01039 8, 01040 0, 01041 8, 01042 &blowfish_info 01043 }; 01044 01045 #if defined(POLARSSL_CIPHER_MODE_CBC) 01046 const cipher_info_t blowfish_cbc_info = { 01047 POLARSSL_CIPHER_BLOWFISH_CBC, 01048 POLARSSL_MODE_CBC, 01049 128, 01050 "BLOWFISH-CBC", 01051 8, 01052 0, 01053 8, 01054 &blowfish_info 01055 }; 01056 #endif /* POLARSSL_CIPHER_MODE_CBC */ 01057 01058 #if defined(POLARSSL_CIPHER_MODE_CFB) 01059 const cipher_info_t blowfish_cfb64_info = { 01060 POLARSSL_CIPHER_BLOWFISH_CFB64, 01061 POLARSSL_MODE_CFB, 01062 128, 01063 "BLOWFISH-CFB64", 01064 8, 01065 0, 01066 8, 01067 &blowfish_info 01068 }; 01069 #endif /* POLARSSL_CIPHER_MODE_CFB */ 01070 01071 #if defined(POLARSSL_CIPHER_MODE_CTR) 01072 const cipher_info_t blowfish_ctr_info = { 01073 POLARSSL_CIPHER_BLOWFISH_CTR, 01074 POLARSSL_MODE_CTR, 01075 128, 01076 "BLOWFISH-CTR", 01077 8, 01078 0, 01079 8, 01080 &blowfish_info 01081 }; 01082 #endif /* POLARSSL_CIPHER_MODE_CTR */ 01083 #endif /* POLARSSL_BLOWFISH_C */ 01084 01085 #if defined(POLARSSL_ARC4_C) 01086 static int arc4_crypt_stream_wrap( void *ctx, size_t length, 01087 const unsigned char *input, 01088 unsigned char *output ) 01089 { 01090 return( arc4_crypt( (arc4_context *) ctx, length, input, output ) ); 01091 } 01092 01093 static int arc4_setkey_wrap( void *ctx, const unsigned char *key, 01094 unsigned int key_length ) 01095 { 01096 /* we get key_length in bits, arc4 expects it in bytes */ 01097 if( key_length % 8 != 0) 01098 return( POLARSSL_ERR_CIPHER_BAD_INPUT_DATA ); 01099 01100 arc4_setup( (arc4_context *) ctx, key, key_length / 8 ); 01101 return( 0 ); 01102 } 01103 01104 static void * arc4_ctx_alloc( void ) 01105 { 01106 return polarssl_malloc( sizeof( arc4_context ) ); 01107 } 01108 01109 static void arc4_ctx_free( void *ctx ) 01110 { 01111 polarssl_free( ctx ); 01112 } 01113 01114 const cipher_base_t arc4_base_info = { 01115 POLARSSL_CIPHER_ID_ARC4, 01116 NULL, 01117 NULL, 01118 NULL, 01119 NULL, 01120 arc4_crypt_stream_wrap, 01121 arc4_setkey_wrap, 01122 arc4_setkey_wrap, 01123 arc4_ctx_alloc, 01124 arc4_ctx_free 01125 }; 01126 01127 const cipher_info_t arc4_128_info = { 01128 POLARSSL_CIPHER_ARC4_128, 01129 POLARSSL_MODE_STREAM, 01130 128, 01131 "ARC4-128", 01132 0, 01133 0, 01134 1, 01135 &arc4_base_info 01136 }; 01137 #endif /* POLARSSL_ARC4_C */ 01138 01139 #if defined(POLARSSL_CIPHER_NULL_CIPHER) 01140 static int null_crypt_stream( void *ctx, size_t length, 01141 const unsigned char *input, 01142 unsigned char *output ) 01143 { 01144 ((void) ctx); 01145 memmove( output, input, length ); 01146 return( 0 ); 01147 } 01148 01149 static int null_setkey( void *ctx, const unsigned char *key, 01150 unsigned int key_length ) 01151 { 01152 ((void) ctx); 01153 ((void) key); 01154 ((void) key_length); 01155 01156 return( 0 ); 01157 } 01158 01159 static void * null_ctx_alloc( void ) 01160 { 01161 return (void *) 1; 01162 } 01163 01164 static void null_ctx_free( void *ctx ) 01165 { 01166 ((void) ctx); 01167 } 01168 01169 const cipher_base_t null_base_info = { 01170 POLARSSL_CIPHER_ID_NULL, 01171 NULL, 01172 NULL, 01173 NULL, 01174 NULL, 01175 null_crypt_stream, 01176 null_setkey, 01177 null_setkey, 01178 null_ctx_alloc, 01179 null_ctx_free 01180 }; 01181 01182 const cipher_info_t null_cipher_info = { 01183 POLARSSL_CIPHER_NULL, 01184 POLARSSL_MODE_STREAM, 01185 0, 01186 "NULL", 01187 0, 01188 0, 01189 1, 01190 &null_base_info 01191 }; 01192 #endif /* defined(POLARSSL_CIPHER_NULL_CIPHER) */ 01193 01194 const cipher_definition_t cipher_definitions[] = 01195 { 01196 #if defined(POLARSSL_AES_C) 01197 { POLARSSL_CIPHER_AES_128_ECB, &aes_128_ecb_info }, 01198 { POLARSSL_CIPHER_AES_192_ECB, &aes_192_ecb_info }, 01199 { POLARSSL_CIPHER_AES_256_ECB, &aes_256_ecb_info }, 01200 #if defined(POLARSSL_CIPHER_MODE_CBC) 01201 { POLARSSL_CIPHER_AES_128_CBC, &aes_128_cbc_info }, 01202 { POLARSSL_CIPHER_AES_192_CBC, &aes_192_cbc_info }, 01203 { POLARSSL_CIPHER_AES_256_CBC, &aes_256_cbc_info }, 01204 #endif 01205 #if defined(POLARSSL_CIPHER_MODE_CFB) 01206 { POLARSSL_CIPHER_AES_128_CFB128, &aes_128_cfb128_info }, 01207 { POLARSSL_CIPHER_AES_192_CFB128, &aes_192_cfb128_info }, 01208 { POLARSSL_CIPHER_AES_256_CFB128, &aes_256_cfb128_info }, 01209 #endif 01210 #if defined(POLARSSL_CIPHER_MODE_CTR) 01211 { POLARSSL_CIPHER_AES_128_CTR, &aes_128_ctr_info }, 01212 { POLARSSL_CIPHER_AES_192_CTR, &aes_192_ctr_info }, 01213 { POLARSSL_CIPHER_AES_256_CTR, &aes_256_ctr_info }, 01214 #endif 01215 #if defined(POLARSSL_GCM_C) 01216 { POLARSSL_CIPHER_AES_128_GCM, &aes_128_gcm_info }, 01217 { POLARSSL_CIPHER_AES_192_GCM, &aes_192_gcm_info }, 01218 { POLARSSL_CIPHER_AES_256_GCM, &aes_256_gcm_info }, 01219 #endif 01220 #endif /* POLARSSL_AES_C */ 01221 01222 #if defined(POLARSSL_ARC4_C) 01223 { POLARSSL_CIPHER_ARC4_128, &arc4_128_info }, 01224 #endif 01225 01226 #if defined(POLARSSL_BLOWFISH_C) 01227 { POLARSSL_CIPHER_BLOWFISH_ECB, &blowfish_ecb_info }, 01228 #if defined(POLARSSL_CIPHER_MODE_CBC) 01229 { POLARSSL_CIPHER_BLOWFISH_CBC, &blowfish_cbc_info }, 01230 #endif 01231 #if defined(POLARSSL_CIPHER_MODE_CFB) 01232 { POLARSSL_CIPHER_BLOWFISH_CFB64, &blowfish_cfb64_info }, 01233 #endif 01234 #if defined(POLARSSL_CIPHER_MODE_CTR) 01235 { POLARSSL_CIPHER_BLOWFISH_CTR, &blowfish_ctr_info }, 01236 #endif 01237 #endif /* POLARSSL_BLOWFISH_C */ 01238 01239 #if defined(POLARSSL_CAMELLIA_C) 01240 { POLARSSL_CIPHER_CAMELLIA_128_ECB, &camellia_128_ecb_info }, 01241 { POLARSSL_CIPHER_CAMELLIA_192_ECB, &camellia_192_ecb_info }, 01242 { POLARSSL_CIPHER_CAMELLIA_256_ECB, &camellia_256_ecb_info }, 01243 #if defined(POLARSSL_CIPHER_MODE_CBC) 01244 { POLARSSL_CIPHER_CAMELLIA_128_CBC, &camellia_128_cbc_info }, 01245 { POLARSSL_CIPHER_CAMELLIA_192_CBC, &camellia_192_cbc_info }, 01246 { POLARSSL_CIPHER_CAMELLIA_256_CBC, &camellia_256_cbc_info }, 01247 #endif 01248 #if defined(POLARSSL_CIPHER_MODE_CFB) 01249 { POLARSSL_CIPHER_CAMELLIA_128_CFB128, &camellia_128_cfb128_info }, 01250 { POLARSSL_CIPHER_CAMELLIA_192_CFB128, &camellia_192_cfb128_info }, 01251 { POLARSSL_CIPHER_CAMELLIA_256_CFB128, &camellia_256_cfb128_info }, 01252 #endif 01253 #if defined(POLARSSL_CIPHER_MODE_CTR) 01254 { POLARSSL_CIPHER_CAMELLIA_128_CTR, &camellia_128_ctr_info }, 01255 { POLARSSL_CIPHER_CAMELLIA_192_CTR, &camellia_192_ctr_info }, 01256 { POLARSSL_CIPHER_CAMELLIA_256_CTR, &camellia_256_ctr_info }, 01257 #endif 01258 #if defined(POLARSSL_GCM_C) 01259 { POLARSSL_CIPHER_CAMELLIA_128_GCM, &camellia_128_gcm_info }, 01260 { POLARSSL_CIPHER_CAMELLIA_192_GCM, &camellia_192_gcm_info }, 01261 { POLARSSL_CIPHER_CAMELLIA_256_GCM, &camellia_256_gcm_info }, 01262 #endif 01263 #endif /* POLARSSL_CAMELLIA_C */ 01264 01265 #if defined(POLARSSL_DES_C) 01266 { POLARSSL_CIPHER_DES_ECB, &des_ecb_info }, 01267 { POLARSSL_CIPHER_DES_EDE_ECB, &des_ede_ecb_info }, 01268 { POLARSSL_CIPHER_DES_EDE3_ECB, &des_ede3_ecb_info }, 01269 #if defined(POLARSSL_CIPHER_MODE_CBC) 01270 { POLARSSL_CIPHER_DES_CBC, &des_cbc_info }, 01271 { POLARSSL_CIPHER_DES_EDE_CBC, &des_ede_cbc_info }, 01272 { POLARSSL_CIPHER_DES_EDE3_CBC, &des_ede3_cbc_info }, 01273 #endif 01274 #endif /* POLARSSL_DES_C */ 01275 01276 #if defined(POLARSSL_CIPHER_NULL_CIPHER) 01277 { POLARSSL_CIPHER_NULL, &null_cipher_info }, 01278 #endif /* POLARSSL_CIPHER_NULL_CIPHER */ 01279 01280 { 0, NULL } 01281 }; 01282 01283 #define NUM_CIPHERS sizeof cipher_definitions / sizeof cipher_definitions[0] 01284 int supported_ciphers[NUM_CIPHERS]; 01285 01286 #endif /* POLARSSL_CIPHER_C */ 01287 01288
Generated on Tue Jul 12 2022 19:40:15 by
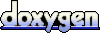