
Example program to test AES-GCM functionality. Used for a workshop
Embed:
(wiki syntax)
Show/hide line numbers
blowfish.h
Go to the documentation of this file.
00001 /** 00002 * \file blowfish.h 00003 * 00004 * \brief Blowfish block cipher 00005 * 00006 * Copyright (C) 2012-2014, Brainspark B.V. 00007 * 00008 * This file is part of PolarSSL (http://www.polarssl.org) 00009 * Lead Maintainer: Paul Bakker <polarssl_maintainer at polarssl.org> 00010 * 00011 * All rights reserved. 00012 * 00013 * This program is free software; you can redistribute it and/or modify 00014 * it under the terms of the GNU General Public License as published by 00015 * the Free Software Foundation; either version 2 of the License, or 00016 * (at your option) any later version. 00017 * 00018 * This program is distributed in the hope that it will be useful, 00019 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00020 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00021 * GNU General Public License for more details. 00022 * 00023 * You should have received a copy of the GNU General Public License along 00024 * with this program; if not, write to the Free Software Foundation, Inc., 00025 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00026 */ 00027 #ifndef POLARSSL_BLOWFISH_H 00028 #define POLARSSL_BLOWFISH_H 00029 00030 #if !defined(POLARSSL_CONFIG_FILE) 00031 #include "config.h" 00032 #else 00033 #include POLARSSL_CONFIG_FILE 00034 #endif 00035 00036 #include <string.h> 00037 00038 #if defined(_MSC_VER) && !defined(EFIX64) && !defined(EFI32) 00039 #include <basetsd.h> 00040 typedef UINT32 uint32_t; 00041 #else 00042 #include <inttypes.h> 00043 #endif 00044 00045 #define BLOWFISH_ENCRYPT 1 00046 #define BLOWFISH_DECRYPT 0 00047 #define BLOWFISH_MAX_KEY 448 00048 #define BLOWFISH_MIN_KEY 32 00049 #define BLOWFISH_ROUNDS 16 /**< Rounds to use. When increasing this value, make sure to extend the initialisation vectors */ 00050 #define BLOWFISH_BLOCKSIZE 8 /* Blowfish uses 64 bit blocks */ 00051 00052 #define POLARSSL_ERR_BLOWFISH_INVALID_KEY_LENGTH -0x0016 /**< Invalid key length. */ 00053 #define POLARSSL_ERR_BLOWFISH_INVALID_INPUT_LENGTH -0x0018 /**< Invalid data input length. */ 00054 00055 #if !defined(POLARSSL_BLOWFISH_ALT) 00056 // Regular implementation 00057 // 00058 00059 #ifdef __cplusplus 00060 extern "C" { 00061 #endif 00062 00063 /** 00064 * \brief Blowfish context structure 00065 */ 00066 typedef struct 00067 { 00068 uint32_t P[BLOWFISH_ROUNDS + 2]; /*!< Blowfish round keys */ 00069 uint32_t S[4][256]; /*!< key dependent S-boxes */ 00070 } 00071 blowfish_context; 00072 00073 /** 00074 * \brief Blowfish key schedule 00075 * 00076 * \param ctx Blowfish context to be initialized 00077 * \param key encryption key 00078 * \param keysize must be between 32 and 448 bits 00079 * 00080 * \return 0 if successful, or POLARSSL_ERR_BLOWFISH_INVALID_KEY_LENGTH 00081 */ 00082 int blowfish_setkey( blowfish_context *ctx, const unsigned char *key, 00083 unsigned int keysize ); 00084 00085 /** 00086 * \brief Blowfish-ECB block encryption/decryption 00087 * 00088 * \param ctx Blowfish context 00089 * \param mode BLOWFISH_ENCRYPT or BLOWFISH_DECRYPT 00090 * \param input 8-byte input block 00091 * \param output 8-byte output block 00092 * 00093 * \return 0 if successful 00094 */ 00095 int blowfish_crypt_ecb( blowfish_context *ctx, 00096 int mode, 00097 const unsigned char input[BLOWFISH_BLOCKSIZE], 00098 unsigned char output[BLOWFISH_BLOCKSIZE] ); 00099 00100 #if defined(POLARSSL_CIPHER_MODE_CBC) 00101 /** 00102 * \brief Blowfish-CBC buffer encryption/decryption 00103 * Length should be a multiple of the block 00104 * size (8 bytes) 00105 * 00106 * \param ctx Blowfish context 00107 * \param mode BLOWFISH_ENCRYPT or BLOWFISH_DECRYPT 00108 * \param length length of the input data 00109 * \param iv initialization vector (updated after use) 00110 * \param input buffer holding the input data 00111 * \param output buffer holding the output data 00112 * 00113 * \return 0 if successful, or 00114 * POLARSSL_ERR_BLOWFISH_INVALID_INPUT_LENGTH 00115 */ 00116 int blowfish_crypt_cbc( blowfish_context *ctx, 00117 int mode, 00118 size_t length, 00119 unsigned char iv[BLOWFISH_BLOCKSIZE], 00120 const unsigned char *input, 00121 unsigned char *output ); 00122 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00123 00124 #if defined(POLARSSL_CIPHER_MODE_CFB) 00125 /** 00126 * \brief Blowfish CFB buffer encryption/decryption. 00127 * 00128 * \param ctx Blowfish context 00129 * \param mode BLOWFISH_ENCRYPT or BLOWFISH_DECRYPT 00130 * \param length length of the input data 00131 * \param iv_off offset in IV (updated after use) 00132 * \param iv initialization vector (updated after use) 00133 * \param input buffer holding the input data 00134 * \param output buffer holding the output data 00135 * 00136 * \return 0 if successful 00137 */ 00138 int blowfish_crypt_cfb64( blowfish_context *ctx, 00139 int mode, 00140 size_t length, 00141 size_t *iv_off, 00142 unsigned char iv[BLOWFISH_BLOCKSIZE], 00143 const unsigned char *input, 00144 unsigned char *output ); 00145 #endif /*POLARSSL_CIPHER_MODE_CFB */ 00146 00147 #if defined(POLARSSL_CIPHER_MODE_CTR) 00148 /** 00149 * \brief Blowfish-CTR buffer encryption/decryption 00150 * 00151 * Warning: You have to keep the maximum use of your counter in mind! 00152 * 00153 * \param ctx Blowfish context 00154 * \param length The length of the data 00155 * \param nc_off The offset in the current stream_block (for resuming 00156 * within current cipher stream). The offset pointer to 00157 * should be 0 at the start of a stream. 00158 * \param nonce_counter The 64-bit nonce and counter. 00159 * \param stream_block The saved stream-block for resuming. Is overwritten 00160 * by the function. 00161 * \param input The input data stream 00162 * \param output The output data stream 00163 * 00164 * \return 0 if successful 00165 */ 00166 int blowfish_crypt_ctr( blowfish_context *ctx, 00167 size_t length, 00168 size_t *nc_off, 00169 unsigned char nonce_counter[BLOWFISH_BLOCKSIZE], 00170 unsigned char stream_block[BLOWFISH_BLOCKSIZE], 00171 const unsigned char *input, 00172 unsigned char *output ); 00173 #endif /* POLARSSL_CIPHER_MODE_CTR */ 00174 00175 #ifdef __cplusplus 00176 } 00177 #endif 00178 00179 #else /* POLARSSL_BLOWFISH_ALT */ 00180 #include "blowfish_alt.h" 00181 #endif /* POLARSSL_BLOWFISH_ALT */ 00182 00183 #endif /* blowfish.h */ 00184 00185
Generated on Tue Jul 12 2022 19:40:15 by
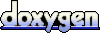