imu01c
Embed:
(wiki syntax)
Show/hide line numbers
vector.cpp
00001 #include <vector.h> 00002 #include <math.h> 00003 00004 void vector_cross(const vector *a,const vector *b, vector *out) 00005 { 00006 out->x = a->y*b->z - a->z*b->y; 00007 out->y = a->z*b->x - a->x*b->z; 00008 out->z = a->x*b->y - a->y*b->x; 00009 } 00010 00011 float vector_dot(const vector *a,const vector *b) 00012 { 00013 return a->x*b->x+a->y*b->y+a->z*b->z; 00014 } 00015 00016 void vector_normalize(vector *a) 00017 { 00018 float mag = sqrt(vector_dot(a,a)); 00019 a->x /= mag; 00020 a->y /= mag; 00021 a->z /= mag; 00022 } 00023 00024 void vector_norm_xz(vector*a) 00025 { 00026 float mag = sqrt((a->x*a->x) + (a->z*a->z)); 00027 a->x /= mag; 00028 a->y = 0; 00029 a->z /= mag; 00030 00031 } 00032 00033 void vector_norm_xy(vector*a) 00034 { 00035 float mag = sqrt(a->x*a->x + a->y*a->y); 00036 a->x /= mag; 00037 a->y /= mag; 00038 a->z = 0; 00039 } 00040 00041 void vector_norm_yz(vector*a) 00042 { 00043 float mag = sqrt(a->z*a->z + a->y*a->y); 00044 a->x = 0; 00045 a->y /= mag; 00046 a->z /= mag; 00047 }
Generated on Tue Jul 12 2022 17:15:09 by
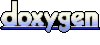