test
Fork of nRF51822 by
Embed:
(wiki syntax)
Show/hide line numbers
pstorage.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) Nordic Semiconductor ASA 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without modification, 00006 * are permitted provided that the following conditions are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright notice, this 00009 * list of conditions and the following disclaimer. 00010 * 00011 * 2. Redistributions in binary form must reproduce the above copyright notice, this 00012 * list of conditions and the following disclaimer in the documentation and/or 00013 * other materials provided with the distribution. 00014 * 00015 * 3. Neither the name of Nordic Semiconductor ASA nor the names of other 00016 * contributors to this software may be used to endorse or promote products 00017 * derived from this software without specific prior written permission. 00018 * 00019 * 00020 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 00021 * ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00022 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00023 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR 00024 * ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00025 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00026 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON 00027 * ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00028 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00029 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00030 * 00031 */ 00032 00033 /**@file 00034 * 00035 * @defgroup persistent_storage Persistent Storage Interface 00036 * @{ 00037 * @ingroup app_common 00038 * @brief Abstracted flash interface. 00039 * 00040 * @details In order to ensure that the SDK and application be moved to alternate persistent storage 00041 * options other than the default provided with NRF solution, an abstracted interface is provided 00042 * by the module to ensure SDK modules and application can be ported to alternate option with ease. 00043 */ 00044 00045 #ifndef PSTORAGE_H__ 00046 #define PSTORAGE_H__ 00047 00048 #include "pstorage_platform.h" 00049 00050 #ifdef __cplusplus 00051 extern "C" { 00052 #endif /* #ifdef __cplusplus */ 00053 00054 00055 /**@defgroup ps_opcode Persistent Storage Access Operation Codes 00056 * @{ 00057 * @brief Persistent Storage Access Operation Codes. These are used to report any error during 00058 * a persistent storage access operation or any general error that may occur in the 00059 * interface. 00060 * 00061 * @details Persistent Storage Access Operation Codes used in error notification callback 00062 * registered with the interface to report any error during an persistent storage access 00063 * operation or any general error that may occur in the interface. 00064 */ 00065 #define PSTORAGE_ERROR_OP_CODE 0x01 /**< General Error Code */ 00066 #define PSTORAGE_STORE_OP_CODE 0x02 /**< Error when Store Operation was requested */ 00067 #define PSTORAGE_LOAD_OP_CODE 0x03 /**< Error when Load Operation was requested */ 00068 #define PSTORAGE_CLEAR_OP_CODE 0x04 /**< Error when Clear Operation was requested */ 00069 #define PSTORAGE_UPDATE_OP_CODE 0x05 /**< Update an already touched storage block */ 00070 00071 /**@} */ 00072 00073 /**@defgroup pstorage_data_types Persistent Memory Interface Data Types 00074 * @{ 00075 * @brief Data Types needed for interfacing with persistent memory. 00076 * 00077 * @details Data Types needed for interfacing with persistent memory. 00078 */ 00079 00080 /**@brief Persistent Storage Error Reporting Callback 00081 * 00082 * @details Persistent Storage Error Reporting Callback that is used by the interface to report 00083 * success or failure of a flash operation. Therefore, for any operations, application 00084 * can know when the procedure was complete. For store operation, since no data copy 00085 * is made, receiving a success or failure notification, indicated by the reason 00086 * parameter of callback is an indication that the resident memory could now be reused 00087 * or freed, as the case may be. 00088 * 00089 * @param[in] handle Identifies module and block for which callback is received. 00090 * @param[in] op_code Identifies the operation for which the event is notified. 00091 * @param[in] result Identifies the result of flash access operation. 00092 * NRF_SUCCESS implies, operation succeeded. 00093 * @param[in] p_data Identifies the application data pointer. In case of store operation, this 00094 * points to the resident source of application memory that application can now 00095 * free or reuse. In case of clear, this is NULL as no application pointer is 00096 * needed for this operation. 00097 * @param[in] data_len Length data application had provided for the operation. 00098 * 00099 */ 00100 typedef void (*pstorage_ntf_cb_t)(pstorage_handle_t * p_handle, 00101 uint8_t op_code, 00102 uint32_t result, 00103 uint8_t * p_data, 00104 uint32_t data_len); 00105 00106 00107 typedef struct 00108 { 00109 pstorage_ntf_cb_t cb; /**< Callback registered with the module to be notified of any error occurring in persistent memory management */ 00110 pstorage_size_t block_size; /**< Desired block size for persistent memory storage, for example, if a module has a table with 10 entries, each entry is size 64 bytes, 00111 * it can request 10 blocks with block size 64 bytes. On the other hand, the module can also request one block of size 640 based on 00112 * how it would like to access or alter memory in persistent memory. 00113 * First option is preferred when single entries that need to be updated often when having no impact on the other entries. 00114 * While second option is preferred when entries of table are not changed on individually but have common point of loading and storing 00115 * data. */ 00116 pstorage_size_t block_count; /** Number of blocks requested by the module, minimum values is 1. */ 00117 } pstorage_module_param_t; 00118 00119 /**@} */ 00120 00121 /**@defgroup pstorage_routines Persistent Storage Access Routines 00122 * @{ 00123 * @brief Functions/Interface SDK modules use to persistently store data. 00124 * 00125 * @details Interface for Application & SDK module to load/store information persistently. 00126 * Note: that while implementation of each of the persistent storage access function 00127 * depends on the system and can specific to system/solution, the signature of the 00128 * interface routines should not be altered. 00129 */ 00130 00131 /**@brief Module Initialization Routine. 00132 * 00133 * @details Initializes module. To be called once before any other APIs of the module are used. 00134 * 00135 * @retval NRF_SUCCESS on success, else an error code indicating reason for failure. 00136 */ 00137 uint32_t pstorage_init(void); 00138 void pstorage_sys_event_handler (uint32_t sys_evt); 00139 00140 00141 /**@brief Register with persistent storage interface. 00142 * 00143 * @param[in] p_module_param Module registration param. 00144 * @param[out] p_block_id Block identifier to identify persistent memory blocks in case 00145 * registration succeeds. Application is expected to use the block ids 00146 * for subsequent operations on requested persistent memory. Maximum 00147 * registrations permitted is determined by configuration parameter 00148 * PSTORAGE_MAX_APPLICATIONS. 00149 * In case more than one memory blocks are requested, the identifier provided here is 00150 * the base identifier for the first block and to identify subsequent block, 00151 * application shall use \@ref pstorage_block_identifier_get with this base identifier 00152 * and block number. Therefore if 10 blocks of size 64 are requested and application 00153 * wishes to store memory in 6th block, it shall use 00154 * \@ref pstorage_block_identifier_get with based id and provide a block number of 5. 00155 * This way application is only expected to remember the base block identifier. 00156 * 00157 * @note To register an area with a total size (block count * block size) larger than the 00158 * page size (usually 1024 bytes), the block size must be a divisor of the page size 00159 * (page size % block size == 0). 00160 * 00161 * @retval NRF_SUCCESS on success, else an error code indicating reason for failure. 00162 * @retval NRF_ERROR_INVALID_STATE is returned is API is called without module initialization. 00163 * @retval NRF_ERROR_NULL if NULL parameter has been passed. 00164 * @retval NRF_ERROR_INVALID_PARAM if invalid parameters are passed to the API. 00165 * @retval NRF_ERROR_NO_MEM in case no more registrations can be supported. 00166 */ 00167 uint32_t pstorage_register(pstorage_module_param_t * p_module_param, 00168 pstorage_handle_t * p_block_id); 00169 00170 00171 /** 00172 * @brief Function to get block id with reference to base block identifier provided at time of 00173 * registration. 00174 * 00175 * @details Function to get block id with reference to base block identifier provided at time of 00176 * registration. 00177 * In case more than one memory blocks were requested when registering, the identifier 00178 * provided here is the base identifier for the first block and to identify subsequent 00179 * block, application shall use this routine to get block identifier providing input as 00180 * base identifier and block number. Therefore if 10 blocks of size 64 are requested and 00181 * application wishes to store memory in 6th block, it shall use 00182 * \@ref pstorage_block_identifier_get with based id and provide a block number of 5. 00183 * This way application is only expected to remember the base block identifier. 00184 * 00185 * @param[in] p_base_id Base block id received at the time of registration. 00186 * @param[in] block_num Block Number, with first block numbered zero. 00187 * @param[out] p_block_id Block identifier for the block number requested in case the API succeeds. 00188 * 00189 * @retval NRF_SUCCESS on success, else an error code indicating reason for failure. 00190 * @retval NRF_ERROR_INVALID_STATE is returned is API is called without module initialization. 00191 * @retval NRF_ERROR_NULL if NULL parameter has been passed. 00192 * @retval NRF_ERROR_INVALID_PARAM if invalid parameters are passed to the API. 00193 */ 00194 uint32_t pstorage_block_identifier_get(pstorage_handle_t * p_base_id, 00195 pstorage_size_t block_num, 00196 pstorage_handle_t * p_block_id); 00197 00198 00199 /**@brief Routine to persistently store data of length 'size' contained in 'p_src' address 00200 * in storage module at 'p_dest' address; Equivalent to Storage Write. 00201 * 00202 * @param[in] p_dest Destination address where data is to be stored persistently. 00203 * @param[in] p_src Source address containing data to be stored. API assumes this to be resident 00204 * memory and no intermediate copy of data is made by the API. 00205 * @param[in] size Size of data to be stored expressed in bytes. Should be word aligned. 00206 * @param[in] offset Offset in bytes to be applied when writing to the block. 00207 * For example, if within a block of 100 bytes, application wishes to 00208 * write 20 bytes at offset of 12, then this field should be set to 12. 00209 * Should be word aligned. 00210 * 00211 * @retval NRF_SUCCESS on success, else an error code indicating reason for failure. 00212 * @retval NRF_ERROR_INVALID_STATE is returned is API is called without module initialization. 00213 * @retval NRF_ERROR_NULL if NULL parameter has been passed. 00214 * @retval NRF_ERROR_INVALID_PARAM if invalid parameters are passed to the API. 00215 * @retval NRF_ERROR_INVALID_ADDR in case data address 'p_src' is not aligned. 00216 * @retval NRF_ERROR_NO_MEM in case request cannot be processed. 00217 * 00218 * @warning No copy of the data is made, and hence memory provided for data source to be written 00219 * to flash cannot be freed or reused by the application until this procedure 00220 * is complete. End of this procedure is notified to the application using the 00221 * notification callback registered by the application. 00222 */ 00223 uint32_t pstorage_store(pstorage_handle_t * p_dest, 00224 uint8_t * p_src, 00225 pstorage_size_t size, 00226 pstorage_size_t offset); 00227 00228 /**@brief Routine to update persistently stored data of length 'size' contained in 'p_src' address 00229 * in storage module at 'p_dest' address. 00230 * 00231 * @param[in] p_dest Destination address where data is to be updated. 00232 * @param[in] p_src Source address containing data to be stored. API assumes this to be resident 00233 * memory and no intermediate copy of data is made by the API. 00234 * @param[in] size Size of data to be stored expressed in bytes. Should be word aligned. 00235 * @param[in] offset Offset in bytes to be applied when writing to the block. 00236 * For example, if within a block of 100 bytes, application wishes to 00237 * write 20 bytes at offset of 12, then this field should be set to 12. 00238 * Should be word aligned. 00239 * 00240 * @retval NRF_SUCCESS on success, else an error code indicating reason for failure. 00241 * @retval NRF_ERROR_INVALID_STATE is returned is API is called without module initialization. 00242 * @retval NRF_ERROR_NULL if NULL parameter has been passed. 00243 * @retval NRF_ERROR_INVALID_PARAM if invalid parameters are passed to the API. 00244 * @retval NRF_ERROR_INVALID_ADDR in case data address 'p_src' is not aligned. 00245 * @retval NRF_ERROR_NO_MEM in case request cannot be processed. 00246 * 00247 * @warning No copy of the data is made, and hence memory provided for data source to be written 00248 * to flash cannot be freed or reused by the application until this procedure 00249 * is complete. End of this procedure is notified to the application using the 00250 * notification callback registered by the application. 00251 */ 00252 uint32_t pstorage_update(pstorage_handle_t * p_dest, 00253 uint8_t * p_src, 00254 pstorage_size_t size, 00255 pstorage_size_t offset); 00256 00257 /**@brief Routine to load persistently stored data of length 'size' from 'p_src' address 00258 * to 'p_dest' address; Equivalent to Storage Read. 00259 * 00260 * @param[in] p_dest Destination address where persistently stored data is to be loaded. 00261 * @param[in] p_src Source from where data is to be loaded from persistent memory. 00262 * @param[in] size Size of data to be loaded from persistent memory expressed in bytes. 00263 * Should be word aligned. 00264 * @param[in] offset Offset in bytes to be applied when loading from the block. 00265 * For example, if within a block of 100 bytes, application wishes to 00266 * load 20 bytes from offset of 12, then this field should be set to 12. 00267 * Should be word aligned. 00268 * 00269 * @retval NRF_SUCCESS on success, else an error code indicating reason for failure. 00270 * @retval NRF_ERROR_INVALID_STATE is returned is API is called without module initialization. 00271 * @retval NRF_ERROR_NULL if NULL parameter has been passed. 00272 * @retval NRF_ERROR_INVALID_PARAM if invalid parameters are passed to the API. 00273 * @retval NRF_ERROR_INVALID_ADDR in case data address 'p_dst' is not aligned. 00274 * @retval NRF_ERROR_NO_MEM in case request cannot be processed. 00275 */ 00276 uint32_t pstorage_load(uint8_t * p_dest, 00277 pstorage_handle_t * p_src, 00278 pstorage_size_t size, 00279 pstorage_size_t offset); 00280 00281 /**@brief Routine to clear data in persistent memory. 00282 * 00283 * @param[in] p_base_id Base block identifier in persistent memory that needs to cleared; 00284 * Equivalent to an Erase Operation. 00285 * 00286 * @param[in] size Size of data to be cleared from persistent memory expressed in bytes. 00287 * This parameter is to provision for clearing of certain blocks 00288 * of memory, or all memory blocks in a registered module. If the total size 00289 * of the application module is used (blocks * block size) in combination with 00290 * the identifier for the first block in the module, all blocks in the 00291 * module will be erased. 00292 * 00293 * @retval NRF_SUCCESS on success, else an error code indicating reason for failure. 00294 * @retval NRF_ERROR_INVALID_STATE is returned is API is called without module initialization. 00295 * @retval NRF_ERROR_NULL if NULL parameter has been passed. 00296 * @retval NRF_ERROR_INVALID_PARAM if invalid parameters are passed to the API. 00297 * @retval NRF_ERROR_INVALID_ADDR in case data address 'p_dst' is not aligned. 00298 * @retval NRF_ERROR_NO_MEM in case request cannot be processed. 00299 * 00300 * @note Clear operations may take time. This API however, does not block until the clear 00301 * procedure is complete. Application is notified of procedure completion using 00302 * notification callback registered by the application. 'result' parameter of the 00303 * callback suggests if the procedure was successful or not. 00304 */ 00305 uint32_t pstorage_clear(pstorage_handle_t * p_base_id, pstorage_size_t size); 00306 00307 /** 00308 * @brief API to get status of number of pending operations with the module. 00309 * 00310 * @param[out] p_count Number of storage operations pending with the module, if 0, 00311 * there are no outstanding requests. 00312 * 00313 * @retval NRF_SUCCESS on success, else an error code indicating reason for failure. 00314 * @retval NRF_ERROR_INVALID_STATE is returned is API is called without module initialization. 00315 * @retval NRF_ERROR_NULL if NULL parameter has been passed. 00316 */ 00317 uint32_t pstorage_access_status_get(uint32_t * p_count); 00318 00319 #ifdef PSTORAGE_RAW_MODE_ENABLE 00320 00321 /**@brief Function for registering with persistent storage interface. 00322 * 00323 * @param[in] p_module_param Module registration param. 00324 * @param[out] p_block_id Block identifier to identify persistent memory blocks in case 00325 * registration succeeds. Application is expected to use the block ids 00326 * for subsequent operations on requested persistent memory. 00327 * In case more than one memory blocks are requested, the identifier provided here is 00328 * the base identifier for the first block and to identify subsequent block, 00329 * application shall use \@ref pstorage_block_identifier_get with this base identifier 00330 * and block number. Therefore if 10 blocks of size 64 are requested and application 00331 * wishes to store memory in 6th block, it shall use 00332 * \@ref pstorage_block_identifier_get with based id and provide a block number of 5. 00333 * This way application is only expected to remember the base block identifier. 00334 * 00335 * @retval NRF_SUCCESS on success, else an error code indicating reason for failure. 00336 * @retval NRF_ERROR_INVALID_STATE is returned is API is called without module initialization. 00337 * @retval NRF_ERROR_NULL if NULL parameter has been passed. 00338 * @retval NRF_ERROR_INVALID_PARAM if invalid parameters are passed to the API. 00339 * @retval NRF_ERROR_NO_MEM in case no more registrations can be supported. 00340 */ 00341 uint32_t pstorage_raw_register(pstorage_module_param_t * p_module_param, 00342 pstorage_handle_t * p_block_id); 00343 00344 /**@brief Raw mode function for persistently storing data of length 'size' contained in 'p_src' 00345 * address in storage module at 'p_dest' address; Equivalent to Storage Write. 00346 * 00347 * @param[in] p_dest Destination address where data is to be stored persistently. 00348 * @param[in] p_src Source address containing data to be stored. API assumes this to be resident 00349 * memory and no intermediate copy of data is made by the API. 00350 * @param[in] size Size of data to be stored expressed in bytes. Should be word aligned. 00351 * @param[in] offset Offset in bytes to be applied when writing to the block. 00352 * For example, if within a block of 100 bytes, application wishes to 00353 * write 20 bytes at offset of 12, then this field should be set to 12. 00354 * Should be word aligned. 00355 * 00356 * @retval NRF_SUCCESS on success, else an error code indicating reason for failure. 00357 * @retval NRF_ERROR_INVALID_STATE is returned is API is called without module initialization. 00358 * @retval NRF_ERROR_NULL if NULL parameter has been passed. 00359 * @retval NRF_ERROR_INVALID_PARAM if invalid parameters are passed to the API. 00360 * @retval NRF_ERROR_INVALID_ADDR in case data address 'p_src' is not aligned. 00361 * @retval NRF_ERROR_NO_MEM in case request cannot be processed. 00362 * 00363 * @warning No copy of the data is made, and hence memory provided for data source to be written 00364 * to flash cannot be freed or reused by the application until this procedure 00365 * is complete. End of this procedure is notified to the application using the 00366 * notification callback registered by the application. 00367 */ 00368 uint32_t pstorage_raw_store(pstorage_handle_t * p_dest, 00369 uint8_t * p_src, 00370 pstorage_size_t size, 00371 pstorage_size_t offset); 00372 00373 /**@brief Function for clearing data in persistent memory in raw mode. 00374 * 00375 * @param[in] p_dest Base block identifier in persistent memory that needs to cleared; 00376 * Equivalent to an Erase Operation. 00377 * @param[in] size Size of data to be cleared from persistent memory expressed in bytes. 00378 * This is currently unused. And a clear would mean clearing all blocks, 00379 * however, this parameter is to provision for clearing of certain blocks 00380 * of memory only and not all if need be. 00381 * 00382 * @retval NRF_SUCCESS on success, else an error code indicating reason for failure. 00383 * @retval NRF_ERROR_INVALID_STATE is returned is API is called without module initialization. 00384 * @retval NRF_ERROR_NULL if NULL parameter has been passed. 00385 * @retval NRF_ERROR_INVALID_PARAM if invalid parameters are passed to the API. 00386 * @retval NRF_ERROR_NO_MEM in case request cannot be processed. 00387 * 00388 * @note Clear operations may take time. This API however, does not block until the clear 00389 * procedure is complete. Application is notified of procedure completion using 00390 * notification callback registered by the application. 'result' parameter of the 00391 * callback suggests if the procedure was successful or not. 00392 */ 00393 uint32_t pstorage_raw_clear(pstorage_handle_t * p_dest, pstorage_size_t size); 00394 00395 #endif // PSTORAGE_RAW_MODE_ENABLE 00396 00397 /**@} */ 00398 /**@} */ 00399 00400 #ifdef __cplusplus 00401 } 00402 #endif /* #ifdef __cplusplus */ 00403 00404 #endif // PSTORAGE_H__
Generated on Tue Jul 12 2022 21:00:17 by
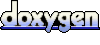