test
Fork of nRF51822 by
Embed:
(wiki syntax)
Show/hide line numbers
nRF5xn.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "nRF5xn.h" 00019 #include "nrf_soc.h" 00020 00021 #include "btle/btle.h" 00022 #include "nrf_delay.h" 00023 00024 #include "softdevice_handler.h " 00025 00026 /** 00027 * The singleton which represents the nRF51822 transport for the BLE. 00028 */ 00029 static nRF5xn deviceInstance; 00030 00031 /** 00032 * BLE-API requires an implementation of the following function in order to 00033 * obtain its transport handle. 00034 */ 00035 BLEInstanceBase * 00036 createBLEInstance(void) 00037 { 00038 return (&deviceInstance); 00039 } 00040 00041 nRF5xn::nRF5xn(void) 00042 { 00043 } 00044 00045 nRF5xn::~nRF5xn(void) 00046 { 00047 } 00048 00049 const char *nRF5xn::getVersion(void) 00050 { 00051 static char versionString[32]; 00052 static bool versionFetched = false; 00053 00054 if (!versionFetched) { 00055 ble_version_t version; 00056 if ((sd_ble_version_get(&version) == NRF_SUCCESS) && (version.company_id == 0x0059)) { 00057 switch (version.version_number) { 00058 case 0x07: 00059 case 0x08: 00060 snprintf(versionString, sizeof(versionString), "Nordic BLE4.1 ver:%u fw:%04x", version.version_number, version.subversion_number); 00061 break; 00062 default: 00063 snprintf(versionString, sizeof(versionString), "Nordic (spec unknown) ver:%u fw:%04x", version.version_number, version.subversion_number); 00064 break; 00065 } 00066 versionFetched = true; 00067 } else { 00068 strncpy(versionString, "unknown", sizeof(versionString)); 00069 } 00070 } 00071 00072 return versionString; 00073 } 00074 00075 ble_error_t nRF5xn::init(void) 00076 { 00077 /* ToDo: Clear memory contents, reset the SD, etc. */ 00078 btle_init(); 00079 00080 return BLE_ERROR_NONE; 00081 } 00082 00083 ble_error_t nRF5xn::shutdown(void) 00084 { 00085 return (softdevice_handler_sd_disable() == NRF_SUCCESS) ? BLE_ERROR_NONE : BLE_STACK_BUSY; 00086 } 00087 00088 void 00089 nRF5xn::waitForEvent(void) 00090 { 00091 sd_app_evt_wait(); 00092 }
Generated on Tue Jul 12 2022 21:00:16 by
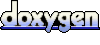