
Sports tracker
Dependencies: C12832_lcd CMPS03 FatFileSystem GPS MMA7660 mbed
Fork of Data_Read_Write by
main.cpp
00001 #include "mbed.h"//mbed library 00002 #include "MSCFileSystem.h"//usb Library 00003 #include "MMA7660.h"//accelerometer library 00004 #include "CMPS03.h"//compass library 00005 #include "GPS.h"//GPS library 00006 #include "C12832_lcd.h" // LCD screen library 00007 MSCFileSystem fs("usb"); // Mount flash drive under the name "usb" 00008 C12832_LCD lcd;// Local name for the LCD 00009 GPS gps(p13,p14);//pin for RX and TX of the gps 00010 Serial pc(USBTX, USBRX); 00011 MMA7660 MMA(p28, p27);// local name for the Accelerometer 00012 DigitalIn fire(p12);//pin for down movement joystick 00013 DigitalIn right(p16);//pin for right movement for joystick 00014 DigitalIn left(p13);//pin for left movement for joystick 00015 CMPS03 compass(p9, p10, CMPS03_DEFAULT_I2C_ADDRESS);//pin for compass 00016 DigitalOut connectionLed(LED1); // debug LED 00017 int lock = 0; 00018 int lock2 = 0; 00019 int lock3 = 0; 00020 int lock4 = 0; 00021 int choice = 0; 00022 int counter = 0; 00023 Timer writeTime;//timer 00024 void run(); 00025 00026 void start()//starts and stops the game 00027 { 00028 00029 int counter = 0; 00030 lcd.cls(); 00031 lcd.locate(0,0); 00032 lcd.printf("Press down to start game"); 00033 lcd.locate(0,20); 00034 lcd.printf("Hold down to stop game"); 00035 wait(1.5); 00036 while (counter == 0) { 00037 if(fire) { 00038 run(); 00039 counter = 1; 00040 } 00041 } 00042 00043 } 00044 void run()// main funtion to get data and write it to files 00045 { 00046 lcd.cls();//clear LCD for next reading round 00047 while(1) { 00048 writeTime.reset();//resets timer 00049 writeTime.start();//starts timer 00050 FILE *longd = fopen( "/usb/long.txt", "a");//opens a file on the usb in append mode 00051 FILE *latd = fopen( "/usb/lat.txt","a"); 00052 FILE *bear = fopen( "/usb/bearing.txt","a"); 00053 FILE *xData = fopen( "/usb/xData.txt","a"); 00054 FILE *yData = fopen( "/usb/yData.txt","a"); 00055 FILE *zData = fopen( "/usb/zData.txt","a"); 00056 FILE *timeFile = fopen( "/usb/test.txt","a"); 00057 if(gps.sample()) {//checks if it can get a gps position 00058 lcd.locate(0,10);//initial LCD location 00059 lcd.printf("Long: %.4f ", gps.longitude);//print to LCD 00060 lcd.printf("Lat: %.4f", gps.latitude);//print to LCD 00061 fprintf(longd,"%f\n",gps.longitude);//prints gps lonitude to file 00062 fprintf(latd,"%f\n",gps.latitude);//prints gps latitude to file 00063 writeTime.stop();//stops timer 00064 fprintf(timeFile,"%f\t",writeTime.read());//write time to file 00065 fclose(timeFile); 00066 } else { 00067 lcd.cls();//clear LCD for next reading round 00068 lcd.locate(3,3);//initial LCD location for x component of acceleration 00069 lcd.printf("Oh Dear! No lock :(\n"); 00070 fprintf(longd,"%c\n","Oh Dear! No Lock :("); 00071 lcd.cls();//clear LCD for next reading round 00072 } 00073 fclose(latd);//closes file 00074 fclose(longd);//closes file 00075 lcd.locate(0,20);//initial LCD location 00076 lcd.printf("Bearing is: %f\n", compass.readBearing() / 10.0);//print to LCD 00077 lcd.locate(0,0);//initial LCD location 00078 lcd.printf("X:%.2f\n",MMA.x());//print x to LCD at locate position 00079 lcd.locate(40,0);//move LCD location for y component 00080 lcd.printf("Y:%.2f\n",MMA.y());//print y to LCD to new locate position 00081 lcd.locate(80,0);//move LCD location for z component 00082 lcd.printf("Z:%.2f\n",MMA.z());//print z to LCD 00083 fprintf(bear,"%.4f\n",compass.readBearing() / 10.0); 00084 fclose(bear);//closes file 00085 fprintf(xData,"%f\n",MMA.x());//writes to file 00086 fclose(xData);//closes file 00087 fprintf(yData,"%f\n",MMA.y()); 00088 fclose(yData);//closes file 00089 fprintf(zData,"%f\n",MMA.z()); 00090 fclose(zData);//closes file 00091 if(fire) { 00092 start(); 00093 counter = 0; 00094 } 00095 } 00096 } 00097 void pitchSetUp()//function to set up the pitch 00098 { 00099 lcd.cls(); 00100 FILE *set = fopen( "/usb/setup.txt","w"); 00101 00102 while (lock == 0) { 00103 lcd.locate(3,3); 00104 lcd.printf("Move to position 1\n"); 00105 if(fire) {//if joystick is pressed down 00106 if(gps.sample()) { 00107 fprintf(set,"%.5f ",gps.longitude); 00108 fprintf(set,"%.5f\n",gps.latitude); 00109 wait (0.5); 00110 lock = 1; 00111 } else { 00112 lcd.cls(); 00113 lcd.printf("No Lock Please try again\n"); 00114 lcd.cls();//clear LCD for next reading round 00115 lock = 0; 00116 } 00117 } 00118 } 00119 while (lock2 == 0) { 00120 lcd.locate(3,3); 00121 lcd.printf("Move to position 2\n"); 00122 if(fire) { 00123 if(gps.sample()) { 00124 fprintf(set,"%.5f ",gps.longitude); 00125 fprintf(set,"%.5f\n",gps.latitude); 00126 wait (0.5); 00127 lock2 = 1; 00128 } else { 00129 lcd.cls(); 00130 lcd.printf("No Lock Please try again\n"); 00131 lcd.cls();//clear LCD for next reading round 00132 lock2 = 0; 00133 } 00134 } 00135 } 00136 while (lock3 == 0) { 00137 lcd.locate(3,3); 00138 lcd.printf("Move to position 3\n"); 00139 if(fire) { 00140 if(gps.sample()) { 00141 fprintf(set,"%.5f ",gps.longitude); 00142 fprintf(set,"%.5f\n",gps.latitude); 00143 wait (0.5); 00144 lock3 = 1; 00145 } else { 00146 lcd.cls(); 00147 lcd.printf("No Lock Please try again\n"); 00148 lcd.cls();//clear LCD for next reading round 00149 lock3 = 0; 00150 } 00151 } 00152 } 00153 while (lock4 == 0) { 00154 lcd.locate(3,3); 00155 lcd.printf("Move to position 4\n"); 00156 if(fire) { 00157 if(gps.sample()) { 00158 fprintf(set,"%.5f ",gps.longitude); 00159 fprintf(set,"%.5f\n",gps.latitude); 00160 wait (0.5); 00161 lock4 = 1; 00162 } else { 00163 lcd.cls(); 00164 lcd.printf("No Lock Please try again\n"); 00165 lcd.cls();//clear LCD for next reading round 00166 lock4 = 0; 00167 } 00168 } 00169 } 00170 fclose(set); 00171 start();//runs the run fution 00172 00173 } 00174 int main()//main funtion 00175 { 00176 //give choice to what the user wants to do 00177 lcd.cls(); 00178 while (choice == 0) { 00179 int choice2 =0; 00180 lcd.locate(3,3); 00181 lcd.printf("Pitch Set Up\n"); 00182 if (fire) {//if joystick is pressed down 00183 pitchSetUp();//runs pitch setup funtion 00184 choice =1; 00185 } 00186 if(right) {//if joystick is pressed right 00187 lcd.cls(); 00188 lcd.locate(3,3); 00189 lcd.printf("Run Game\n"); 00190 //counter = counter++; 00191 wait(0.5);//wait for half a second 00192 00193 while (choice2 == 0) { 00194 if (fire) { 00195 start();//runs the run funtion 00196 choice =1; 00197 choice2 = 1; 00198 } 00199 if (left) {//if joystick is pressed to the left 00200 choice2 = 1; 00201 choice = 0; 00202 main();//runs the main funtion 00203 } 00204 } 00205 } 00206 } 00207 }
Generated on Thu Jul 14 2022 07:16:25 by
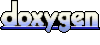