BLE_Nano nRF51 Central heart rate
Embed:
(wiki syntax)
Show/hide line numbers
softdevice_handler.c
00001 /* Copyright (c) 2012 Nordic Semiconductor. All Rights Reserved. 00002 * 00003 * The information contained herein is property of Nordic Semiconductor ASA. 00004 * Terms and conditions of usage are described in detail in NORDIC 00005 * SEMICONDUCTOR STANDARD SOFTWARE LICENSE AGREEMENT. 00006 * 00007 * Licensees are granted free, non-transferable use of the information. NO 00008 * WARRANTY of ANY KIND is provided. This heading must NOT be removed from 00009 * the file. 00010 * 00011 */ 00012 00013 #include "softdevice_handler.h " 00014 #include <stdlib.h> 00015 #include <stdint.h> 00016 #include <stdio.h> 00017 #include <string.h> 00018 #include "nordic_common.h" 00019 #include "app_error.h " 00020 #include "nrf_assert.h" 00021 #include "nrf_nvic.h" 00022 #include "nrf.h" 00023 #include "nrf_log.h" 00024 #include "sdk_common.h" 00025 #include "nrf_drv_config.h" 00026 #if CLOCK_ENABLED 00027 #include "nrf_drv_clock.h" 00028 #endif 00029 00030 #if defined(ANT_STACK_SUPPORT_REQD) && defined(BLE_STACK_SUPPORT_REQD) 00031 #include "ant_interface.h" 00032 #elif defined(ANT_STACK_SUPPORT_REQD) 00033 #include "ant_interface.h" 00034 #elif defined(BLE_STACK_SUPPORT_REQD) 00035 #include "ble.h" 00036 #endif 00037 00038 00039 #if defined(NRF_LOG_USES_RTT) && NRF_LOG_USES_RTT == 1 00040 #define SD_HANDLER_LOG(...) NRF_LOG_PRINTF(__VA_ARGS__) 00041 #else 00042 #define SD_HANDLER_LOG(...) 00043 #endif 00044 00045 #if defined(NRF_LOG_USES_RTT) && NRF_LOG_USES_RTT == 1 00046 #define SD_HANDLER_LOG_INIT() NRF_LOG_INIT() 00047 #else 00048 #define SD_HANDLER_LOG_INIT() 00049 #endif 00050 00051 00052 00053 #define RAM_START_ADDRESS 0x20000000 00054 #define SOFTDEVICE_EVT_IRQ SD_EVT_IRQn /**< SoftDevice Event IRQ number. Used for both protocol events and SoC events. */ 00055 #define SOFTDEVICE_EVT_IRQHandler SD_EVT_IRQHandler 00056 #define RAM_TOTAL_SIZE ((NRF_FICR->INFO.RAM)*1024) 00057 #define RAM_END_ADDRESS (RAM_START_ADDRESS + RAM_TOTAL_SIZE) 00058 00059 00060 #define SOFTDEVICE_VS_UUID_COUNT 0 00061 #define SOFTDEVICE_GATTS_ATTR_TAB_SIZE BLE_GATTS_ATTR_TAB_SIZE_DEFAULT 00062 #define SOFTDEVICE_GATTS_SRV_CHANGED 0 00063 #define SOFTDEVICE_PERIPH_CONN_COUNT 1 00064 #define SOFTDEVICE_CENTRAL_CONN_COUNT 4 00065 #define SOFTDEVICE_CENTRAL_SEC_COUNT 1 00066 00067 /* Global nvic state instance, required by nrf_nvic.h */ 00068 nrf_nvic_state_t nrf_nvic_state; 00069 00070 static softdevice_evt_schedule_func_t m_evt_schedule_func; /**< Pointer to function for propagating SoftDevice events to the scheduler. */ 00071 00072 static volatile bool m_softdevice_enabled = false; /**< Variable to indicate whether the SoftDevice is enabled. */ 00073 00074 #ifdef BLE_STACK_SUPPORT_REQD 00075 // The following three definitions is needed only if BLE events are needed to be pulled from the stack. 00076 static uint8_t * mp_ble_evt_buffer; /**< Buffer for receiving BLE events from the SoftDevice. */ 00077 static uint16_t m_ble_evt_buffer_size; /**< Size of BLE event buffer. */ 00078 static ble_evt_handler_t m_ble_evt_handler; /**< Application event handler for handling BLE events. */ 00079 #endif 00080 00081 #ifdef ANT_STACK_SUPPORT_REQD 00082 // The following two definition is needed only if ANT events are needed to be pulled from the stack. 00083 static ant_evt_t m_ant_evt_buffer; /**< Buffer for receiving ANT events from the SoftDevice. */ 00084 static ant_evt_handler_t m_ant_evt_handler; /**< Application event handler for handling ANT events. */ 00085 #endif 00086 00087 static sys_evt_handler_t m_sys_evt_handler; /**< Application event handler for handling System (SOC) events. */ 00088 00089 00090 /**@brief Callback function for asserts in the SoftDevice. 00091 * 00092 * @details A pointer to this function will be passed to the SoftDevice. This function will be 00093 * called by the SoftDevice if certain unrecoverable errors occur within the 00094 * application or SoftDevice. 00095 * 00096 * See @ref nrf_fault_handler_t for more details. 00097 * 00098 * @param[in] id Fault identifier. See @ref NRF_FAULT_IDS. 00099 * @param[in] pc The program counter of the instruction that triggered the fault. 00100 * @param[in] info Optional additional information regarding the fault. Refer to each fault 00101 * identifier for details. 00102 */ 00103 void softdevice_fault_handler(uint32_t id, uint32_t pc, uint32_t info) 00104 { 00105 app_error_fault_handler(id, pc, info); 00106 } 00107 00108 00109 void intern_softdevice_events_execute(void) 00110 { 00111 if (!m_softdevice_enabled) 00112 { 00113 // SoftDevice not enabled. This can be possible if the SoftDevice was enabled by the 00114 // application without using this module's API (i.e softdevice_handler_init) 00115 00116 return; 00117 } 00118 #if CLOCK_ENABLED 00119 bool no_more_soc_evts = false; 00120 #else 00121 bool no_more_soc_evts = (m_sys_evt_handler == NULL); 00122 #endif 00123 #ifdef BLE_STACK_SUPPORT_REQD 00124 bool no_more_ble_evts = (m_ble_evt_handler == NULL); 00125 #endif 00126 #ifdef ANT_STACK_SUPPORT_REQD 00127 bool no_more_ant_evts = (m_ant_evt_handler == NULL); 00128 #endif 00129 00130 for (;;) 00131 { 00132 uint32_t err_code; 00133 00134 if (!no_more_soc_evts) 00135 { 00136 uint32_t evt_id; 00137 00138 // Pull event from SOC. 00139 err_code = sd_evt_get(&evt_id); 00140 00141 if (err_code == NRF_ERROR_NOT_FOUND) 00142 { 00143 no_more_soc_evts = true; 00144 } 00145 else if (err_code != NRF_SUCCESS) 00146 { 00147 APP_ERROR_HANDLER(err_code); 00148 } 00149 else 00150 { 00151 // Call application's SOC event handler. 00152 #if CLOCK_ENABLED 00153 nrf_drv_clock_on_soc_event(evt_id); 00154 if (m_sys_evt_handler) 00155 { 00156 m_sys_evt_handler(evt_id); 00157 } 00158 #else 00159 m_sys_evt_handler(evt_id); 00160 #endif 00161 } 00162 } 00163 00164 #ifdef BLE_STACK_SUPPORT_REQD 00165 // Fetch BLE Events. 00166 if (!no_more_ble_evts) 00167 { 00168 // Pull event from stack 00169 uint16_t evt_len = m_ble_evt_buffer_size; 00170 00171 err_code = sd_ble_evt_get(mp_ble_evt_buffer, &evt_len); 00172 if (err_code == NRF_ERROR_NOT_FOUND) 00173 { 00174 no_more_ble_evts = true; 00175 } 00176 else if (err_code != NRF_SUCCESS) 00177 { 00178 APP_ERROR_HANDLER(err_code); 00179 } 00180 else 00181 { 00182 // Call application's BLE stack event handler. 00183 m_ble_evt_handler((ble_evt_t *)mp_ble_evt_buffer); 00184 } 00185 } 00186 #endif 00187 00188 #ifdef ANT_STACK_SUPPORT_REQD 00189 // Fetch ANT Events. 00190 if (!no_more_ant_evts) 00191 { 00192 // Pull event from stack 00193 err_code = sd_ant_event_get(&m_ant_evt_buffer.channel, 00194 &m_ant_evt_buffer.event, 00195 m_ant_evt_buffer.msg.evt_buffer); 00196 if (err_code == NRF_ERROR_NOT_FOUND) 00197 { 00198 no_more_ant_evts = true; 00199 } 00200 else if (err_code != NRF_SUCCESS) 00201 { 00202 APP_ERROR_HANDLER(err_code); 00203 } 00204 else 00205 { 00206 // Call application's ANT stack event handler. 00207 m_ant_evt_handler(&m_ant_evt_buffer); 00208 } 00209 } 00210 #endif 00211 00212 if (no_more_soc_evts) 00213 { 00214 // There are no remaining System (SOC) events to be fetched from the SoftDevice. 00215 #if defined(ANT_STACK_SUPPORT_REQD) && defined(BLE_STACK_SUPPORT_REQD) 00216 // Check if there are any remaining BLE and ANT events. 00217 if (no_more_ble_evts && no_more_ant_evts) 00218 { 00219 break; 00220 } 00221 #elif defined(BLE_STACK_SUPPORT_REQD) 00222 // Check if there are any remaining BLE events. 00223 if (no_more_ble_evts) 00224 { 00225 break; 00226 } 00227 #elif defined(ANT_STACK_SUPPORT_REQD) 00228 // Check if there are any remaining ANT events. 00229 if (no_more_ant_evts) 00230 { 00231 break; 00232 } 00233 #else 00234 // No need to check for BLE or ANT events since there is no support for BLE and ANT 00235 // required. 00236 break; 00237 #endif 00238 } 00239 } 00240 } 00241 00242 bool softdevice_handler_isEnabled(void) 00243 { 00244 return m_softdevice_enabled; 00245 } 00246 00247 uint32_t softdevice_handler_init(nrf_clock_lf_cfg_t * p_clock_lf_cfg, 00248 void * p_ble_evt_buffer, 00249 uint16_t ble_evt_buffer_size, 00250 softdevice_evt_schedule_func_t evt_schedule_func) 00251 { 00252 uint32_t err_code; 00253 00254 SD_HANDLER_LOG_INIT(); 00255 00256 // Save configuration. 00257 #if defined (BLE_STACK_SUPPORT_REQD) 00258 // Check that buffer is not NULL. 00259 if (p_ble_evt_buffer == NULL) 00260 { 00261 return NRF_ERROR_INVALID_PARAM; 00262 } 00263 00264 // Check that buffer is correctly aligned. 00265 if (!is_word_aligned(p_ble_evt_buffer)) 00266 { 00267 return NRF_ERROR_INVALID_PARAM; 00268 } 00269 00270 mp_ble_evt_buffer = (uint8_t *)p_ble_evt_buffer; 00271 m_ble_evt_buffer_size = ble_evt_buffer_size; 00272 #else 00273 // The variables p_ble_evt_buffer and ble_evt_buffer_size is not needed if BLE Stack support 00274 // is not required. 00275 UNUSED_PARAMETER(p_ble_evt_buffer); 00276 UNUSED_PARAMETER(ble_evt_buffer_size); 00277 #endif 00278 00279 m_evt_schedule_func = evt_schedule_func; 00280 00281 // Initialize SoftDevice. 00282 #if defined(S212) || defined(S332) 00283 err_code = sd_softdevice_enable(p_clock_lf_cfg, softdevice_fault_handler, ANT_LICENSE_KEY); 00284 #else 00285 err_code = sd_softdevice_enable(p_clock_lf_cfg, softdevice_fault_handler); 00286 #endif 00287 if (err_code != NRF_SUCCESS) 00288 { 00289 return err_code; 00290 } 00291 00292 m_softdevice_enabled = true; 00293 00294 // Enable BLE event interrupt (interrupt priority has already been set by the stack). 00295 #ifdef SOFTDEVICE_PRESENT 00296 return sd_nvic_EnableIRQ((IRQn_Type )SOFTDEVICE_EVT_IRQ); 00297 #else 00298 //In case of Serialization NVIC must be accessed directly. 00299 NVIC_EnableIRQ(SOFTDEVICE_EVT_IRQ); 00300 return NRF_SUCCESS; 00301 #endif 00302 } 00303 00304 00305 uint32_t softdevice_handler_sd_disable(void) 00306 { 00307 uint32_t err_code = sd_softdevice_disable(); 00308 00309 m_softdevice_enabled = !(err_code == NRF_SUCCESS); 00310 00311 return err_code; 00312 } 00313 00314 00315 #ifdef BLE_STACK_SUPPORT_REQD 00316 uint32_t softdevice_ble_evt_handler_set(ble_evt_handler_t ble_evt_handler) 00317 { 00318 VERIFY_PARAM_NOT_NULL(ble_evt_handler); 00319 00320 m_ble_evt_handler = ble_evt_handler; 00321 00322 return NRF_SUCCESS; 00323 } 00324 #endif 00325 00326 00327 #ifdef ANT_STACK_SUPPORT_REQD 00328 uint32_t softdevice_ant_evt_handler_set(ant_evt_handler_t ant_evt_handler) 00329 { 00330 VERIFY_PARAM_NOT_NULL(ant_evt_handler); 00331 00332 m_ant_evt_handler = ant_evt_handler; 00333 00334 return NRF_SUCCESS; 00335 } 00336 #endif 00337 00338 00339 uint32_t softdevice_sys_evt_handler_set(sys_evt_handler_t sys_evt_handler) 00340 { 00341 VERIFY_PARAM_NOT_NULL(sys_evt_handler); 00342 00343 m_sys_evt_handler = sys_evt_handler; 00344 00345 return NRF_SUCCESS; 00346 } 00347 00348 00349 /**@brief Function for handling the Application's BLE Stack events interrupt. 00350 * 00351 * @details This function is called whenever an event is ready to be pulled. 00352 */ 00353 void SOFTDEVICE_EVT_IRQHandler(void) 00354 { 00355 if (m_evt_schedule_func != NULL) 00356 { 00357 uint32_t err_code = m_evt_schedule_func(); 00358 APP_ERROR_CHECK(err_code); 00359 } 00360 else 00361 { 00362 intern_softdevice_events_execute(); 00363 } 00364 } 00365 00366 #if defined(BLE_STACK_SUPPORT_REQD) 00367 uint32_t softdevice_enable_get_default_config(uint8_t central_links_count, 00368 uint8_t periph_links_count, 00369 ble_enable_params_t * p_ble_enable_params) 00370 { 00371 memset(p_ble_enable_params, 0, sizeof(ble_enable_params_t)); 00372 p_ble_enable_params->common_enable_params.vs_uuid_count = 1; 00373 p_ble_enable_params->gatts_enable_params.attr_tab_size = SOFTDEVICE_GATTS_ATTR_TAB_SIZE; 00374 p_ble_enable_params->gatts_enable_params.service_changed = SOFTDEVICE_GATTS_SRV_CHANGED; 00375 p_ble_enable_params->gap_enable_params.periph_conn_count = periph_links_count; 00376 p_ble_enable_params->gap_enable_params.central_conn_count = central_links_count; 00377 if (p_ble_enable_params->gap_enable_params.central_conn_count != 0) 00378 { 00379 p_ble_enable_params->gap_enable_params.central_sec_count = SOFTDEVICE_CENTRAL_SEC_COUNT; 00380 } 00381 00382 return NRF_SUCCESS; 00383 } 00384 00385 00386 #if defined(NRF_LOG_USES_RTT) && NRF_LOG_USES_RTT == 1 00387 static inline uint32_t ram_total_size_get(void) 00388 { 00389 #ifdef NRF51 00390 uint32_t size_ram_blocks = (uint32_t)NRF_FICR->SIZERAMBLOCKS; 00391 uint32_t total_ram_size = size_ram_blocks; 00392 total_ram_size = total_ram_size*(NRF_FICR->NUMRAMBLOCK); 00393 return total_ram_size; 00394 #elif defined (NRF52) 00395 return RAM_TOTAL_SIZE; 00396 #endif /* NRF51 */ 00397 } 00398 00399 /*lint --e{528} -save suppress 528: symbol not referenced */ 00400 /**@brief Function for finding the end address of the RAM. 00401 * 00402 * @retval ram_end_address Address of the end of the RAM. 00403 */ 00404 static inline uint32_t ram_end_address_get(void) 00405 { 00406 uint32_t ram_end_address = (uint32_t)RAM_START_ADDRESS; 00407 ram_end_address+= ram_total_size_get(); 00408 return ram_end_address; 00409 } 00410 /*lint -restore*/ 00411 #endif //ENABLE_DEBUG_LOG_SUPPORT 00412 00413 /*lint --e{10} --e{19} --e{27} --e{40} --e{529} -save suppress Error 27: Illegal character */ 00414 uint32_t sd_check_ram_start(uint32_t sd_req_ram_start) 00415 { 00416 #if (defined(S130) || defined(S132) || defined(S332)) 00417 #if defined ( __CC_ARM ) 00418 extern uint32_t Image$$RW_IRAM1$$Base; 00419 const volatile uint32_t ram_start = (uint32_t) &Image$$RW_IRAM1$$Base; 00420 #elif defined ( __ICCARM__ ) 00421 extern uint32_t __ICFEDIT_region_RAM_start__; 00422 volatile uint32_t ram_start = (uint32_t) &__ICFEDIT_region_RAM_start__; 00423 #elif defined ( __GNUC__ ) 00424 extern uint32_t __data_start__; 00425 volatile uint32_t ram_start = (uint32_t) &__data_start__; 00426 #endif//__CC_ARM 00427 if (ram_start != sd_req_ram_start) 00428 { 00429 #if defined(NRF_LOG_USES_RTT) && NRF_LOG_USES_RTT == 1 00430 uint32_t app_ram_size= ram_end_address_get(); 00431 SD_HANDLER_LOG("RAM START ADDR 0x%x should be adjusted to 0x%x\r\n", 00432 ram_start, 00433 sd_req_ram_start); 00434 app_ram_size -= sd_req_ram_start; 00435 SD_HANDLER_LOG("RAM SIZE should be adjusted to 0x%x \r\n", 00436 app_ram_size); 00437 #endif //NRF_LOG_USES_RTT 00438 return NRF_SUCCESS; 00439 } 00440 #endif//defined(S130) || defined(S132) || defined(S332) 00441 return NRF_SUCCESS; 00442 } 00443 00444 uint32_t softdevice_enable(ble_enable_params_t * p_ble_enable_params) 00445 { 00446 #if (defined(S130) || defined(S132) || defined(S332)) 00447 uint32_t err_code; 00448 uint32_t app_ram_base; 00449 00450 #if defined ( __CC_ARM ) 00451 extern uint32_t Image$$RW_IRAM1$$Base; 00452 const volatile uint32_t ram_start = (uint32_t) &Image$$RW_IRAM1$$Base; 00453 #elif defined ( __ICCARM__ ) 00454 extern uint32_t __ICFEDIT_region_RAM_start__; 00455 volatile uint32_t ram_start = (uint32_t) &__ICFEDIT_region_RAM_start__; 00456 #elif defined ( __GNUC__ ) 00457 extern uint32_t __data_start__; 00458 volatile uint32_t ram_start = (uint32_t) &__data_start__; 00459 #endif 00460 00461 app_ram_base = ram_start; 00462 SD_HANDLER_LOG("sd_ble_enable: RAM START at 0x%x\r\n", 00463 app_ram_base); 00464 err_code = sd_ble_enable(p_ble_enable_params, &app_ram_base); 00465 00466 #if defined(NRF_LOG_USES_RTT) && NRF_LOG_USES_RTT == 1 00467 if (app_ram_base != ram_start) 00468 { 00469 uint32_t app_ram_size= ram_end_address_get(); 00470 SD_HANDLER_LOG("sd_ble_enable: app_ram_base should be adjusted to 0x%x\r\n", 00471 app_ram_base); 00472 app_ram_size -= app_ram_base; 00473 SD_HANDLER_LOG("ram size should be adjusted to 0x%x \r\n", 00474 app_ram_size); 00475 } 00476 else if (err_code != NRF_SUCCESS) 00477 { 00478 SD_HANDLER_LOG("sd_ble_enable: error 0x%x\r\n", err_code); 00479 while(1); 00480 } 00481 #endif // NRF_LOG_USES_RTT 00482 return err_code; 00483 #else 00484 return NRF_SUCCESS; 00485 #endif //defined(S130) || defined(S132) || defined(S332) 00486 00487 } 00488 /*lint -restore*/ 00489 00490 #endif //BLE_STACK_SUPPORT_REQD 00491
Generated on Wed Jul 13 2022 07:07:19 by
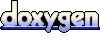