BLE_Nano nRF51 Central heart rate
Embed:
(wiki syntax)
Show/hide line numbers
core_cm0.h
Go to the documentation of this file.
00001 /**************************************************************************//** 00002 * @file core_cm0.h 00003 * @brief CMSIS Cortex-M0 Core Peripheral Access Layer Header File 00004 * @version V4.30 00005 * @date 20. October 2015 00006 ******************************************************************************/ 00007 /* Copyright (c) 2009 - 2015 ARM LIMITED 00008 00009 All rights reserved. 00010 Redistribution and use in source and binary forms, with or without 00011 modification, are permitted provided that the following conditions are met: 00012 - Redistributions of source code must retain the above copyright 00013 notice, this list of conditions and the following disclaimer. 00014 - Redistributions in binary form must reproduce the above copyright 00015 notice, this list of conditions and the following disclaimer in the 00016 documentation and/or other materials provided with the distribution. 00017 - Neither the name of ARM nor the names of its contributors may be used 00018 to endorse or promote products derived from this software without 00019 specific prior written permission. 00020 * 00021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00022 AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00023 IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE 00024 ARE DISCLAIMED. IN NO EVENT SHALL COPYRIGHT HOLDERS AND CONTRIBUTORS BE 00025 LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR 00026 CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF 00027 SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00028 INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00029 CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 00030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00031 POSSIBILITY OF SUCH DAMAGE. 00032 ---------------------------------------------------------------------------*/ 00033 00034 00035 #if defined ( __ICCARM__ ) 00036 #pragma system_include /* treat file as system include file for MISRA check */ 00037 #elif defined(__ARMCC_VERSION) && (__ARMCC_VERSION >= 6010050) 00038 #pragma clang system_header /* treat file as system include file */ 00039 #endif 00040 00041 #ifndef __CORE_CM0_H_GENERIC 00042 #define __CORE_CM0_H_GENERIC 00043 00044 #include <stdint.h> 00045 00046 #ifdef __cplusplus 00047 extern "C" { 00048 #endif 00049 00050 /** 00051 \page CMSIS_MISRA_Exceptions MISRA-C:2004 Compliance Exceptions 00052 CMSIS violates the following MISRA-C:2004 rules: 00053 00054 \li Required Rule 8.5, object/function definition in header file.<br> 00055 Function definitions in header files are used to allow 'inlining'. 00056 00057 \li Required Rule 18.4, declaration of union type or object of union type: '{...}'.<br> 00058 Unions are used for effective representation of core registers. 00059 00060 \li Advisory Rule 19.7, Function-like macro defined.<br> 00061 Function-like macros are used to allow more efficient code. 00062 */ 00063 00064 00065 /******************************************************************************* 00066 * CMSIS definitions 00067 ******************************************************************************/ 00068 /** 00069 \ingroup Cortex_M0 00070 @{ 00071 */ 00072 00073 /* CMSIS CM0 definitions */ 00074 #define __CM0_CMSIS_VERSION_MAIN (0x04U) /*!< [31:16] CMSIS HAL main version */ 00075 #define __CM0_CMSIS_VERSION_SUB (0x1EU) /*!< [15:0] CMSIS HAL sub version */ 00076 #define __CM0_CMSIS_VERSION ((__CM0_CMSIS_VERSION_MAIN << 16U) | \ 00077 __CM0_CMSIS_VERSION_SUB ) /*!< CMSIS HAL version number */ 00078 00079 #define __CORTEX_M (0x00U) /*!< Cortex-M Core */ 00080 00081 00082 #if defined ( __CC_ARM ) 00083 #define __ASM __asm /*!< asm keyword for ARM Compiler */ 00084 #define __INLINE __inline /*!< inline keyword for ARM Compiler */ 00085 #define __STATIC_INLINE static __inline 00086 00087 #elif defined(__ARMCC_VERSION) && (__ARMCC_VERSION >= 6010050) 00088 #define __ASM __asm /*!< asm keyword for ARM Compiler */ 00089 #define __INLINE __inline /*!< inline keyword for ARM Compiler */ 00090 #define __STATIC_INLINE static __inline 00091 00092 #elif defined ( __GNUC__ ) 00093 #define __ASM __asm /*!< asm keyword for GNU Compiler */ 00094 #define __INLINE inline /*!< inline keyword for GNU Compiler */ 00095 #define __STATIC_INLINE static inline 00096 00097 #elif defined ( __ICCARM__ ) 00098 #define __ASM __asm /*!< asm keyword for IAR Compiler */ 00099 #define __INLINE inline /*!< inline keyword for IAR Compiler. Only available in High optimization mode! */ 00100 #define __STATIC_INLINE static inline 00101 00102 #elif defined ( __TMS470__ ) 00103 #define __ASM __asm /*!< asm keyword for TI CCS Compiler */ 00104 #define __STATIC_INLINE static inline 00105 00106 #elif defined ( __TASKING__ ) 00107 #define __ASM __asm /*!< asm keyword for TASKING Compiler */ 00108 #define __INLINE inline /*!< inline keyword for TASKING Compiler */ 00109 #define __STATIC_INLINE static inline 00110 00111 #elif defined ( __CSMC__ ) 00112 #define __packed 00113 #define __ASM _asm /*!< asm keyword for COSMIC Compiler */ 00114 #define __INLINE inline /*!< inline keyword for COSMIC Compiler. Use -pc99 on compile line */ 00115 #define __STATIC_INLINE static inline 00116 00117 #else 00118 #error Unknown compiler 00119 #endif 00120 00121 /** __FPU_USED indicates whether an FPU is used or not. 00122 This core does not support an FPU at all 00123 */ 00124 #define __FPU_USED 0U 00125 00126 #if defined ( __CC_ARM ) 00127 #if defined __TARGET_FPU_VFP 00128 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00129 #endif 00130 00131 #elif defined(__ARMCC_VERSION) && (__ARMCC_VERSION >= 6010050) 00132 #if defined __ARM_PCS_VFP 00133 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00134 #endif 00135 00136 #elif defined ( __GNUC__ ) 00137 #if defined (__VFP_FP__) && !defined(__SOFTFP__) 00138 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00139 #endif 00140 00141 #elif defined ( __ICCARM__ ) 00142 #if defined __ARMVFP__ 00143 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00144 #endif 00145 00146 #elif defined ( __TMS470__ ) 00147 #if defined __TI_VFP_SUPPORT__ 00148 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00149 #endif 00150 00151 #elif defined ( __TASKING__ ) 00152 #if defined __FPU_VFP__ 00153 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00154 #endif 00155 00156 #elif defined ( __CSMC__ ) 00157 #if ( __CSMC__ & 0x400U) 00158 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00159 #endif 00160 00161 #endif 00162 00163 #include "core_cmInstr.h" /* Core Instruction Access */ 00164 #include "core_cmFunc.h" /* Core Function Access */ 00165 00166 #ifdef __cplusplus 00167 } 00168 #endif 00169 00170 #endif /* __CORE_CM0_H_GENERIC */ 00171 00172 #ifndef __CMSIS_GENERIC 00173 00174 #ifndef __CORE_CM0_H_DEPENDANT 00175 #define __CORE_CM0_H_DEPENDANT 00176 00177 #ifdef __cplusplus 00178 extern "C" { 00179 #endif 00180 00181 /* check device defines and use defaults */ 00182 #if defined __CHECK_DEVICE_DEFINES 00183 #ifndef __CM0_REV 00184 #define __CM0_REV 0x0000U 00185 #warning "__CM0_REV not defined in device header file; using default!" 00186 #endif 00187 00188 #ifndef __NVIC_PRIO_BITS 00189 #define __NVIC_PRIO_BITS 2U 00190 #warning "__NVIC_PRIO_BITS not defined in device header file; using default!" 00191 #endif 00192 00193 #ifndef __Vendor_SysTickConfig 00194 #define __Vendor_SysTickConfig 0U 00195 #warning "__Vendor_SysTickConfig not defined in device header file; using default!" 00196 #endif 00197 #endif 00198 00199 /* IO definitions (access restrictions to peripheral registers) */ 00200 /** 00201 \defgroup CMSIS_glob_defs CMSIS Global Defines 00202 00203 <strong>IO Type Qualifiers</strong> are used 00204 \li to specify the access to peripheral variables. 00205 \li for automatic generation of peripheral register debug information. 00206 */ 00207 #ifdef __cplusplus 00208 #define __I volatile /*!< Defines 'read only' permissions */ 00209 #else 00210 #define __I volatile const /*!< Defines 'read only' permissions */ 00211 #endif 00212 #define __O volatile /*!< Defines 'write only' permissions */ 00213 #define __IO volatile /*!< Defines 'read / write' permissions */ 00214 00215 /* following defines should be used for structure members */ 00216 #define __IM volatile const /*! Defines 'read only' structure member permissions */ 00217 #define __OM volatile /*! Defines 'write only' structure member permissions */ 00218 #define __IOM volatile /*! Defines 'read / write' structure member permissions */ 00219 00220 /*@} end of group Cortex_M0 */ 00221 00222 00223 00224 /******************************************************************************* 00225 * Register Abstraction 00226 Core Register contain: 00227 - Core Register 00228 - Core NVIC Register 00229 - Core SCB Register 00230 - Core SysTick Register 00231 ******************************************************************************/ 00232 /** 00233 \defgroup CMSIS_core_register Defines and Type Definitions 00234 \brief Type definitions and defines for Cortex-M processor based devices. 00235 */ 00236 00237 /** 00238 \ingroup CMSIS_core_register 00239 \defgroup CMSIS_CORE Status and Control Registers 00240 \brief Core Register type definitions. 00241 @{ 00242 */ 00243 00244 /** 00245 \brief Union type to access the Application Program Status Register (APSR). 00246 */ 00247 typedef union 00248 { 00249 struct 00250 { 00251 uint32_t _reserved0:28; /*!< bit: 0..27 Reserved */ 00252 uint32_t V:1; /*!< bit: 28 Overflow condition code flag */ 00253 uint32_t C:1; /*!< bit: 29 Carry condition code flag */ 00254 uint32_t Z:1; /*!< bit: 30 Zero condition code flag */ 00255 uint32_t N:1; /*!< bit: 31 Negative condition code flag */ 00256 } b; /*!< Structure used for bit access */ 00257 uint32_t w; /*!< Type used for word access */ 00258 } APSR_Type; 00259 00260 /* APSR Register Definitions */ 00261 #define APSR_N_Pos 31U /*!< APSR: N Position */ 00262 #define APSR_N_Msk (1UL << APSR_N_Pos) /*!< APSR: N Mask */ 00263 00264 #define APSR_Z_Pos 30U /*!< APSR: Z Position */ 00265 #define APSR_Z_Msk (1UL << APSR_Z_Pos) /*!< APSR: Z Mask */ 00266 00267 #define APSR_C_Pos 29U /*!< APSR: C Position */ 00268 #define APSR_C_Msk (1UL << APSR_C_Pos) /*!< APSR: C Mask */ 00269 00270 #define APSR_V_Pos 28U /*!< APSR: V Position */ 00271 #define APSR_V_Msk (1UL << APSR_V_Pos) /*!< APSR: V Mask */ 00272 00273 00274 /** 00275 \brief Union type to access the Interrupt Program Status Register (IPSR). 00276 */ 00277 typedef union 00278 { 00279 struct 00280 { 00281 uint32_t ISR:9; /*!< bit: 0.. 8 Exception number */ 00282 uint32_t _reserved0:23; /*!< bit: 9..31 Reserved */ 00283 } b; /*!< Structure used for bit access */ 00284 uint32_t w; /*!< Type used for word access */ 00285 } IPSR_Type; 00286 00287 /* IPSR Register Definitions */ 00288 #define IPSR_ISR_Pos 0U /*!< IPSR: ISR Position */ 00289 #define IPSR_ISR_Msk (0x1FFUL /*<< IPSR_ISR_Pos*/) /*!< IPSR: ISR Mask */ 00290 00291 00292 /** 00293 \brief Union type to access the Special-Purpose Program Status Registers (xPSR). 00294 */ 00295 typedef union 00296 { 00297 struct 00298 { 00299 uint32_t ISR:9; /*!< bit: 0.. 8 Exception number */ 00300 uint32_t _reserved0:15; /*!< bit: 9..23 Reserved */ 00301 uint32_t T:1; /*!< bit: 24 Thumb bit (read 0) */ 00302 uint32_t _reserved1:3; /*!< bit: 25..27 Reserved */ 00303 uint32_t V:1; /*!< bit: 28 Overflow condition code flag */ 00304 uint32_t C:1; /*!< bit: 29 Carry condition code flag */ 00305 uint32_t Z:1; /*!< bit: 30 Zero condition code flag */ 00306 uint32_t N:1; /*!< bit: 31 Negative condition code flag */ 00307 } b; /*!< Structure used for bit access */ 00308 uint32_t w; /*!< Type used for word access */ 00309 } xPSR_Type; 00310 00311 /* xPSR Register Definitions */ 00312 #define xPSR_N_Pos 31U /*!< xPSR: N Position */ 00313 #define xPSR_N_Msk (1UL << xPSR_N_Pos) /*!< xPSR: N Mask */ 00314 00315 #define xPSR_Z_Pos 30U /*!< xPSR: Z Position */ 00316 #define xPSR_Z_Msk (1UL << xPSR_Z_Pos) /*!< xPSR: Z Mask */ 00317 00318 #define xPSR_C_Pos 29U /*!< xPSR: C Position */ 00319 #define xPSR_C_Msk (1UL << xPSR_C_Pos) /*!< xPSR: C Mask */ 00320 00321 #define xPSR_V_Pos 28U /*!< xPSR: V Position */ 00322 #define xPSR_V_Msk (1UL << xPSR_V_Pos) /*!< xPSR: V Mask */ 00323 00324 #define xPSR_T_Pos 24U /*!< xPSR: T Position */ 00325 #define xPSR_T_Msk (1UL << xPSR_T_Pos) /*!< xPSR: T Mask */ 00326 00327 #define xPSR_ISR_Pos 0U /*!< xPSR: ISR Position */ 00328 #define xPSR_ISR_Msk (0x1FFUL /*<< xPSR_ISR_Pos*/) /*!< xPSR: ISR Mask */ 00329 00330 00331 /** 00332 \brief Union type to access the Control Registers (CONTROL). 00333 */ 00334 typedef union 00335 { 00336 struct 00337 { 00338 uint32_t _reserved0:1; /*!< bit: 0 Reserved */ 00339 uint32_t SPSEL:1; /*!< bit: 1 Stack to be used */ 00340 uint32_t _reserved1:30; /*!< bit: 2..31 Reserved */ 00341 } b; /*!< Structure used for bit access */ 00342 uint32_t w; /*!< Type used for word access */ 00343 } CONTROL_Type; 00344 00345 /* CONTROL Register Definitions */ 00346 #define CONTROL_SPSEL_Pos 1U /*!< CONTROL: SPSEL Position */ 00347 #define CONTROL_SPSEL_Msk (1UL << CONTROL_SPSEL_Pos) /*!< CONTROL: SPSEL Mask */ 00348 00349 /*@} end of group CMSIS_CORE */ 00350 00351 00352 /** 00353 \ingroup CMSIS_core_register 00354 \defgroup CMSIS_NVIC Nested Vectored Interrupt Controller (NVIC) 00355 \brief Type definitions for the NVIC Registers 00356 @{ 00357 */ 00358 00359 /** 00360 \brief Structure type to access the Nested Vectored Interrupt Controller (NVIC). 00361 */ 00362 typedef struct 00363 { 00364 __IOM uint32_t ISER[1U]; /*!< Offset: 0x000 (R/W) Interrupt Set Enable Register */ 00365 uint32_t RESERVED0[31U]; 00366 __IOM uint32_t ICER[1U]; /*!< Offset: 0x080 (R/W) Interrupt Clear Enable Register */ 00367 uint32_t RSERVED1[31U]; 00368 __IOM uint32_t ISPR[1U]; /*!< Offset: 0x100 (R/W) Interrupt Set Pending Register */ 00369 uint32_t RESERVED2[31U]; 00370 __IOM uint32_t ICPR[1U]; /*!< Offset: 0x180 (R/W) Interrupt Clear Pending Register */ 00371 uint32_t RESERVED3[31U]; 00372 uint32_t RESERVED4[64U]; 00373 __IOM uint32_t IP[8U]; /*!< Offset: 0x300 (R/W) Interrupt Priority Register */ 00374 } NVIC_Type; 00375 00376 /*@} end of group CMSIS_NVIC */ 00377 00378 00379 /** 00380 \ingroup CMSIS_core_register 00381 \defgroup CMSIS_SCB System Control Block (SCB) 00382 \brief Type definitions for the System Control Block Registers 00383 @{ 00384 */ 00385 00386 /** 00387 \brief Structure type to access the System Control Block (SCB). 00388 */ 00389 typedef struct 00390 { 00391 __IM uint32_t CPUID; /*!< Offset: 0x000 (R/ ) CPUID Base Register */ 00392 __IOM uint32_t ICSR; /*!< Offset: 0x004 (R/W) Interrupt Control and State Register */ 00393 uint32_t RESERVED0; 00394 __IOM uint32_t AIRCR; /*!< Offset: 0x00C (R/W) Application Interrupt and Reset Control Register */ 00395 __IOM uint32_t SCR; /*!< Offset: 0x010 (R/W) System Control Register */ 00396 __IOM uint32_t CCR; /*!< Offset: 0x014 (R/W) Configuration Control Register */ 00397 uint32_t RESERVED1; 00398 __IOM uint32_t SHP[2U]; /*!< Offset: 0x01C (R/W) System Handlers Priority Registers. [0] is RESERVED */ 00399 __IOM uint32_t SHCSR; /*!< Offset: 0x024 (R/W) System Handler Control and State Register */ 00400 } SCB_Type; 00401 00402 /* SCB CPUID Register Definitions */ 00403 #define SCB_CPUID_IMPLEMENTER_Pos 24U /*!< SCB CPUID: IMPLEMENTER Position */ 00404 #define SCB_CPUID_IMPLEMENTER_Msk (0xFFUL << SCB_CPUID_IMPLEMENTER_Pos) /*!< SCB CPUID: IMPLEMENTER Mask */ 00405 00406 #define SCB_CPUID_VARIANT_Pos 20U /*!< SCB CPUID: VARIANT Position */ 00407 #define SCB_CPUID_VARIANT_Msk (0xFUL << SCB_CPUID_VARIANT_Pos) /*!< SCB CPUID: VARIANT Mask */ 00408 00409 #define SCB_CPUID_ARCHITECTURE_Pos 16U /*!< SCB CPUID: ARCHITECTURE Position */ 00410 #define SCB_CPUID_ARCHITECTURE_Msk (0xFUL << SCB_CPUID_ARCHITECTURE_Pos) /*!< SCB CPUID: ARCHITECTURE Mask */ 00411 00412 #define SCB_CPUID_PARTNO_Pos 4U /*!< SCB CPUID: PARTNO Position */ 00413 #define SCB_CPUID_PARTNO_Msk (0xFFFUL << SCB_CPUID_PARTNO_Pos) /*!< SCB CPUID: PARTNO Mask */ 00414 00415 #define SCB_CPUID_REVISION_Pos 0U /*!< SCB CPUID: REVISION Position */ 00416 #define SCB_CPUID_REVISION_Msk (0xFUL /*<< SCB_CPUID_REVISION_Pos*/) /*!< SCB CPUID: REVISION Mask */ 00417 00418 /* SCB Interrupt Control State Register Definitions */ 00419 #define SCB_ICSR_NMIPENDSET_Pos 31U /*!< SCB ICSR: NMIPENDSET Position */ 00420 #define SCB_ICSR_NMIPENDSET_Msk (1UL << SCB_ICSR_NMIPENDSET_Pos) /*!< SCB ICSR: NMIPENDSET Mask */ 00421 00422 #define SCB_ICSR_PENDSVSET_Pos 28U /*!< SCB ICSR: PENDSVSET Position */ 00423 #define SCB_ICSR_PENDSVSET_Msk (1UL << SCB_ICSR_PENDSVSET_Pos) /*!< SCB ICSR: PENDSVSET Mask */ 00424 00425 #define SCB_ICSR_PENDSVCLR_Pos 27U /*!< SCB ICSR: PENDSVCLR Position */ 00426 #define SCB_ICSR_PENDSVCLR_Msk (1UL << SCB_ICSR_PENDSVCLR_Pos) /*!< SCB ICSR: PENDSVCLR Mask */ 00427 00428 #define SCB_ICSR_PENDSTSET_Pos 26U /*!< SCB ICSR: PENDSTSET Position */ 00429 #define SCB_ICSR_PENDSTSET_Msk (1UL << SCB_ICSR_PENDSTSET_Pos) /*!< SCB ICSR: PENDSTSET Mask */ 00430 00431 #define SCB_ICSR_PENDSTCLR_Pos 25U /*!< SCB ICSR: PENDSTCLR Position */ 00432 #define SCB_ICSR_PENDSTCLR_Msk (1UL << SCB_ICSR_PENDSTCLR_Pos) /*!< SCB ICSR: PENDSTCLR Mask */ 00433 00434 #define SCB_ICSR_ISRPREEMPT_Pos 23U /*!< SCB ICSR: ISRPREEMPT Position */ 00435 #define SCB_ICSR_ISRPREEMPT_Msk (1UL << SCB_ICSR_ISRPREEMPT_Pos) /*!< SCB ICSR: ISRPREEMPT Mask */ 00436 00437 #define SCB_ICSR_ISRPENDING_Pos 22U /*!< SCB ICSR: ISRPENDING Position */ 00438 #define SCB_ICSR_ISRPENDING_Msk (1UL << SCB_ICSR_ISRPENDING_Pos) /*!< SCB ICSR: ISRPENDING Mask */ 00439 00440 #define SCB_ICSR_VECTPENDING_Pos 12U /*!< SCB ICSR: VECTPENDING Position */ 00441 #define SCB_ICSR_VECTPENDING_Msk (0x1FFUL << SCB_ICSR_VECTPENDING_Pos) /*!< SCB ICSR: VECTPENDING Mask */ 00442 00443 #define SCB_ICSR_VECTACTIVE_Pos 0U /*!< SCB ICSR: VECTACTIVE Position */ 00444 #define SCB_ICSR_VECTACTIVE_Msk (0x1FFUL /*<< SCB_ICSR_VECTACTIVE_Pos*/) /*!< SCB ICSR: VECTACTIVE Mask */ 00445 00446 /* SCB Application Interrupt and Reset Control Register Definitions */ 00447 #define SCB_AIRCR_VECTKEY_Pos 16U /*!< SCB AIRCR: VECTKEY Position */ 00448 #define SCB_AIRCR_VECTKEY_Msk (0xFFFFUL << SCB_AIRCR_VECTKEY_Pos) /*!< SCB AIRCR: VECTKEY Mask */ 00449 00450 #define SCB_AIRCR_VECTKEYSTAT_Pos 16U /*!< SCB AIRCR: VECTKEYSTAT Position */ 00451 #define SCB_AIRCR_VECTKEYSTAT_Msk (0xFFFFUL << SCB_AIRCR_VECTKEYSTAT_Pos) /*!< SCB AIRCR: VECTKEYSTAT Mask */ 00452 00453 #define SCB_AIRCR_ENDIANESS_Pos 15U /*!< SCB AIRCR: ENDIANESS Position */ 00454 #define SCB_AIRCR_ENDIANESS_Msk (1UL << SCB_AIRCR_ENDIANESS_Pos) /*!< SCB AIRCR: ENDIANESS Mask */ 00455 00456 #define SCB_AIRCR_SYSRESETREQ_Pos 2U /*!< SCB AIRCR: SYSRESETREQ Position */ 00457 #define SCB_AIRCR_SYSRESETREQ_Msk (1UL << SCB_AIRCR_SYSRESETREQ_Pos) /*!< SCB AIRCR: SYSRESETREQ Mask */ 00458 00459 #define SCB_AIRCR_VECTCLRACTIVE_Pos 1U /*!< SCB AIRCR: VECTCLRACTIVE Position */ 00460 #define SCB_AIRCR_VECTCLRACTIVE_Msk (1UL << SCB_AIRCR_VECTCLRACTIVE_Pos) /*!< SCB AIRCR: VECTCLRACTIVE Mask */ 00461 00462 /* SCB System Control Register Definitions */ 00463 #define SCB_SCR_SEVONPEND_Pos 4U /*!< SCB SCR: SEVONPEND Position */ 00464 #define SCB_SCR_SEVONPEND_Msk (1UL << SCB_SCR_SEVONPEND_Pos) /*!< SCB SCR: SEVONPEND Mask */ 00465 00466 #define SCB_SCR_SLEEPDEEP_Pos 2U /*!< SCB SCR: SLEEPDEEP Position */ 00467 #define SCB_SCR_SLEEPDEEP_Msk (1UL << SCB_SCR_SLEEPDEEP_Pos) /*!< SCB SCR: SLEEPDEEP Mask */ 00468 00469 #define SCB_SCR_SLEEPONEXIT_Pos 1U /*!< SCB SCR: SLEEPONEXIT Position */ 00470 #define SCB_SCR_SLEEPONEXIT_Msk (1UL << SCB_SCR_SLEEPONEXIT_Pos) /*!< SCB SCR: SLEEPONEXIT Mask */ 00471 00472 /* SCB Configuration Control Register Definitions */ 00473 #define SCB_CCR_STKALIGN_Pos 9U /*!< SCB CCR: STKALIGN Position */ 00474 #define SCB_CCR_STKALIGN_Msk (1UL << SCB_CCR_STKALIGN_Pos) /*!< SCB CCR: STKALIGN Mask */ 00475 00476 #define SCB_CCR_UNALIGN_TRP_Pos 3U /*!< SCB CCR: UNALIGN_TRP Position */ 00477 #define SCB_CCR_UNALIGN_TRP_Msk (1UL << SCB_CCR_UNALIGN_TRP_Pos) /*!< SCB CCR: UNALIGN_TRP Mask */ 00478 00479 /* SCB System Handler Control and State Register Definitions */ 00480 #define SCB_SHCSR_SVCALLPENDED_Pos 15U /*!< SCB SHCSR: SVCALLPENDED Position */ 00481 #define SCB_SHCSR_SVCALLPENDED_Msk (1UL << SCB_SHCSR_SVCALLPENDED_Pos) /*!< SCB SHCSR: SVCALLPENDED Mask */ 00482 00483 /*@} end of group CMSIS_SCB */ 00484 00485 00486 /** 00487 \ingroup CMSIS_core_register 00488 \defgroup CMSIS_SysTick System Tick Timer (SysTick) 00489 \brief Type definitions for the System Timer Registers. 00490 @{ 00491 */ 00492 00493 /** 00494 \brief Structure type to access the System Timer (SysTick). 00495 */ 00496 typedef struct 00497 { 00498 __IOM uint32_t CTRL; /*!< Offset: 0x000 (R/W) SysTick Control and Status Register */ 00499 __IOM uint32_t LOAD; /*!< Offset: 0x004 (R/W) SysTick Reload Value Register */ 00500 __IOM uint32_t VAL; /*!< Offset: 0x008 (R/W) SysTick Current Value Register */ 00501 __IM uint32_t CALIB; /*!< Offset: 0x00C (R/ ) SysTick Calibration Register */ 00502 } SysTick_Type; 00503 00504 /* SysTick Control / Status Register Definitions */ 00505 #define SysTick_CTRL_COUNTFLAG_Pos 16U /*!< SysTick CTRL: COUNTFLAG Position */ 00506 #define SysTick_CTRL_COUNTFLAG_Msk (1UL << SysTick_CTRL_COUNTFLAG_Pos) /*!< SysTick CTRL: COUNTFLAG Mask */ 00507 00508 #define SysTick_CTRL_CLKSOURCE_Pos 2U /*!< SysTick CTRL: CLKSOURCE Position */ 00509 #define SysTick_CTRL_CLKSOURCE_Msk (1UL << SysTick_CTRL_CLKSOURCE_Pos) /*!< SysTick CTRL: CLKSOURCE Mask */ 00510 00511 #define SysTick_CTRL_TICKINT_Pos 1U /*!< SysTick CTRL: TICKINT Position */ 00512 #define SysTick_CTRL_TICKINT_Msk (1UL << SysTick_CTRL_TICKINT_Pos) /*!< SysTick CTRL: TICKINT Mask */ 00513 00514 #define SysTick_CTRL_ENABLE_Pos 0U /*!< SysTick CTRL: ENABLE Position */ 00515 #define SysTick_CTRL_ENABLE_Msk (1UL /*<< SysTick_CTRL_ENABLE_Pos*/) /*!< SysTick CTRL: ENABLE Mask */ 00516 00517 /* SysTick Reload Register Definitions */ 00518 #define SysTick_LOAD_RELOAD_Pos 0U /*!< SysTick LOAD: RELOAD Position */ 00519 #define SysTick_LOAD_RELOAD_Msk (0xFFFFFFUL /*<< SysTick_LOAD_RELOAD_Pos*/) /*!< SysTick LOAD: RELOAD Mask */ 00520 00521 /* SysTick Current Register Definitions */ 00522 #define SysTick_VAL_CURRENT_Pos 0U /*!< SysTick VAL: CURRENT Position */ 00523 #define SysTick_VAL_CURRENT_Msk (0xFFFFFFUL /*<< SysTick_VAL_CURRENT_Pos*/) /*!< SysTick VAL: CURRENT Mask */ 00524 00525 /* SysTick Calibration Register Definitions */ 00526 #define SysTick_CALIB_NOREF_Pos 31U /*!< SysTick CALIB: NOREF Position */ 00527 #define SysTick_CALIB_NOREF_Msk (1UL << SysTick_CALIB_NOREF_Pos) /*!< SysTick CALIB: NOREF Mask */ 00528 00529 #define SysTick_CALIB_SKEW_Pos 30U /*!< SysTick CALIB: SKEW Position */ 00530 #define SysTick_CALIB_SKEW_Msk (1UL << SysTick_CALIB_SKEW_Pos) /*!< SysTick CALIB: SKEW Mask */ 00531 00532 #define SysTick_CALIB_TENMS_Pos 0U /*!< SysTick CALIB: TENMS Position */ 00533 #define SysTick_CALIB_TENMS_Msk (0xFFFFFFUL /*<< SysTick_CALIB_TENMS_Pos*/) /*!< SysTick CALIB: TENMS Mask */ 00534 00535 /*@} end of group CMSIS_SysTick */ 00536 00537 00538 /** 00539 \ingroup CMSIS_core_register 00540 \defgroup CMSIS_CoreDebug Core Debug Registers (CoreDebug) 00541 \brief Cortex-M0 Core Debug Registers (DCB registers, SHCSR, and DFSR) are only accessible over DAP and not via processor. 00542 Therefore they are not covered by the Cortex-M0 header file. 00543 @{ 00544 */ 00545 /*@} end of group CMSIS_CoreDebug */ 00546 00547 00548 /** 00549 \ingroup CMSIS_core_register 00550 \defgroup CMSIS_core_bitfield Core register bit field macros 00551 \brief Macros for use with bit field definitions (xxx_Pos, xxx_Msk). 00552 @{ 00553 */ 00554 00555 /** 00556 \brief Mask and shift a bit field value for use in a register bit range. 00557 \param[in] field Name of the register bit field. 00558 \param[in] value Value of the bit field. 00559 \return Masked and shifted value. 00560 */ 00561 #define _VAL2FLD(field, value) ((value << field ## _Pos) & field ## _Msk) 00562 00563 /** 00564 \brief Mask and shift a register value to extract a bit filed value. 00565 \param[in] field Name of the register bit field. 00566 \param[in] value Value of register. 00567 \return Masked and shifted bit field value. 00568 */ 00569 #define _FLD2VAL(field, value) ((value & field ## _Msk) >> field ## _Pos) 00570 00571 /*@} end of group CMSIS_core_bitfield */ 00572 00573 00574 /** 00575 \ingroup CMSIS_core_register 00576 \defgroup CMSIS_core_base Core Definitions 00577 \brief Definitions for base addresses, unions, and structures. 00578 @{ 00579 */ 00580 00581 /* Memory mapping of Cortex-M0 Hardware */ 00582 #define SCS_BASE (0xE000E000UL) /*!< System Control Space Base Address */ 00583 #define SysTick_BASE (SCS_BASE + 0x0010UL) /*!< SysTick Base Address */ 00584 #define NVIC_BASE (SCS_BASE + 0x0100UL) /*!< NVIC Base Address */ 00585 #define SCB_BASE (SCS_BASE + 0x0D00UL) /*!< System Control Block Base Address */ 00586 00587 #define SCB ((SCB_Type *) SCB_BASE ) /*!< SCB configuration struct */ 00588 #define SysTick ((SysTick_Type *) SysTick_BASE ) /*!< SysTick configuration struct */ 00589 #define NVIC ((NVIC_Type *) NVIC_BASE ) /*!< NVIC configuration struct */ 00590 00591 00592 /*@} */ 00593 00594 00595 00596 /******************************************************************************* 00597 * Hardware Abstraction Layer 00598 Core Function Interface contains: 00599 - Core NVIC Functions 00600 - Core SysTick Functions 00601 - Core Register Access Functions 00602 ******************************************************************************/ 00603 /** 00604 \defgroup CMSIS_Core_FunctionInterface Functions and Instructions Reference 00605 */ 00606 00607 00608 00609 /* ########################## NVIC functions #################################### */ 00610 /** 00611 \ingroup CMSIS_Core_FunctionInterface 00612 \defgroup CMSIS_Core_NVICFunctions NVIC Functions 00613 \brief Functions that manage interrupts and exceptions via the NVIC. 00614 @{ 00615 */ 00616 00617 /* Interrupt Priorities are WORD accessible only under ARMv6M */ 00618 /* The following MACROS handle generation of the register offset and byte masks */ 00619 #define _BIT_SHIFT(IRQn) ( ((((uint32_t)(int32_t)(IRQn)) ) & 0x03UL) * 8UL) 00620 #define _SHP_IDX(IRQn) ( (((((uint32_t)(int32_t)(IRQn)) & 0x0FUL)-8UL) >> 2UL) ) 00621 #define _IP_IDX(IRQn) ( (((uint32_t)(int32_t)(IRQn)) >> 2UL) ) 00622 00623 00624 /** 00625 \brief Enable External Interrupt 00626 \details Enables a device-specific interrupt in the NVIC interrupt controller. 00627 \param [in] IRQn External interrupt number. Value cannot be negative. 00628 */ 00629 __STATIC_INLINE void NVIC_EnableIRQ(IRQn_Type IRQn) 00630 { 00631 NVIC->ISER[0U] = (uint32_t)(1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL)); 00632 } 00633 00634 00635 /** 00636 \brief Disable External Interrupt 00637 \details Disables a device-specific interrupt in the NVIC interrupt controller. 00638 \param [in] IRQn External interrupt number. Value cannot be negative. 00639 */ 00640 __STATIC_INLINE void NVIC_DisableIRQ(IRQn_Type IRQn) 00641 { 00642 NVIC->ICER[0U] = (uint32_t)(1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL)); 00643 } 00644 00645 00646 /** 00647 \brief Get Pending Interrupt 00648 \details Reads the pending register in the NVIC and returns the pending bit for the specified interrupt. 00649 \param [in] IRQn Interrupt number. 00650 \return 0 Interrupt status is not pending. 00651 \return 1 Interrupt status is pending. 00652 */ 00653 __STATIC_INLINE uint32_t NVIC_GetPendingIRQ(IRQn_Type IRQn) 00654 { 00655 return((uint32_t)(((NVIC->ISPR[0U] & (1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL))) != 0UL) ? 1UL : 0UL)); 00656 } 00657 00658 00659 /** 00660 \brief Set Pending Interrupt 00661 \details Sets the pending bit of an external interrupt. 00662 \param [in] IRQn Interrupt number. Value cannot be negative. 00663 */ 00664 __STATIC_INLINE void NVIC_SetPendingIRQ(IRQn_Type IRQn) 00665 { 00666 NVIC->ISPR[0U] = (uint32_t)(1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL)); 00667 } 00668 00669 00670 /** 00671 \brief Clear Pending Interrupt 00672 \details Clears the pending bit of an external interrupt. 00673 \param [in] IRQn External interrupt number. Value cannot be negative. 00674 */ 00675 __STATIC_INLINE void NVIC_ClearPendingIRQ(IRQn_Type IRQn) 00676 { 00677 NVIC->ICPR[0U] = (uint32_t)(1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL)); 00678 } 00679 00680 00681 /** 00682 \brief Set Interrupt Priority 00683 \details Sets the priority of an interrupt. 00684 \note The priority cannot be set for every core interrupt. 00685 \param [in] IRQn Interrupt number. 00686 \param [in] priority Priority to set. 00687 */ 00688 __STATIC_INLINE void NVIC_SetPriority(IRQn_Type IRQn, uint32_t priority) 00689 { 00690 if ((int32_t)(IRQn) < 0) 00691 { 00692 SCB->SHP[_SHP_IDX(IRQn)] = ((uint32_t)(SCB->SHP[_SHP_IDX(IRQn)] & ~(0xFFUL << _BIT_SHIFT(IRQn))) | 00693 (((priority << (8U - __NVIC_PRIO_BITS)) & (uint32_t)0xFFUL) << _BIT_SHIFT(IRQn))); 00694 } 00695 else 00696 { 00697 NVIC->IP[_IP_IDX(IRQn)] = ((uint32_t)(NVIC->IP[_IP_IDX(IRQn)] & ~(0xFFUL << _BIT_SHIFT(IRQn))) | 00698 (((priority << (8U - __NVIC_PRIO_BITS)) & (uint32_t)0xFFUL) << _BIT_SHIFT(IRQn))); 00699 } 00700 } 00701 00702 00703 /** 00704 \brief Get Interrupt Priority 00705 \details Reads the priority of an interrupt. 00706 The interrupt number can be positive to specify an external (device specific) interrupt, 00707 or negative to specify an internal (core) interrupt. 00708 \param [in] IRQn Interrupt number. 00709 \return Interrupt Priority. 00710 Value is aligned automatically to the implemented priority bits of the microcontroller. 00711 */ 00712 __STATIC_INLINE uint32_t NVIC_GetPriority(IRQn_Type IRQn) 00713 { 00714 00715 if ((int32_t)(IRQn) < 0) 00716 { 00717 return((uint32_t)(((SCB->SHP[_SHP_IDX(IRQn)] >> _BIT_SHIFT(IRQn) ) & (uint32_t)0xFFUL) >> (8U - __NVIC_PRIO_BITS))); 00718 } 00719 else 00720 { 00721 return((uint32_t)(((NVIC->IP[ _IP_IDX(IRQn)] >> _BIT_SHIFT(IRQn) ) & (uint32_t)0xFFUL) >> (8U - __NVIC_PRIO_BITS))); 00722 } 00723 } 00724 00725 00726 /** 00727 \brief System Reset 00728 \details Initiates a system reset request to reset the MCU. 00729 */ 00730 __STATIC_INLINE void NVIC_SystemReset(void) 00731 { 00732 __DSB(); /* Ensure all outstanding memory accesses included 00733 buffered write are completed before reset */ 00734 SCB->AIRCR = ((0x5FAUL << SCB_AIRCR_VECTKEY_Pos) | 00735 SCB_AIRCR_SYSRESETREQ_Msk); 00736 __DSB(); /* Ensure completion of memory access */ 00737 00738 for(;;) /* wait until reset */ 00739 { 00740 __NOP(); 00741 } 00742 } 00743 00744 /*@} end of CMSIS_Core_NVICFunctions */ 00745 00746 00747 00748 /* ################################## SysTick function ############################################ */ 00749 /** 00750 \ingroup CMSIS_Core_FunctionInterface 00751 \defgroup CMSIS_Core_SysTickFunctions SysTick Functions 00752 \brief Functions that configure the System. 00753 @{ 00754 */ 00755 00756 #if (__Vendor_SysTickConfig == 0U) 00757 00758 /** 00759 \brief System Tick Configuration 00760 \details Initializes the System Timer and its interrupt, and starts the System Tick Timer. 00761 Counter is in free running mode to generate periodic interrupts. 00762 \param [in] ticks Number of ticks between two interrupts. 00763 \return 0 Function succeeded. 00764 \return 1 Function failed. 00765 \note When the variable <b>__Vendor_SysTickConfig</b> is set to 1, then the 00766 function <b>SysTick_Config</b> is not included. In this case, the file <b><i>device</i>.h</b> 00767 must contain a vendor-specific implementation of this function. 00768 */ 00769 __STATIC_INLINE uint32_t SysTick_Config(uint32_t ticks) 00770 { 00771 if ((ticks - 1UL) > SysTick_LOAD_RELOAD_Msk) 00772 { 00773 return (1UL); /* Reload value impossible */ 00774 } 00775 00776 SysTick->LOAD = (uint32_t)(ticks - 1UL); /* set reload register */ 00777 NVIC_SetPriority (SysTick_IRQn , (1UL << __NVIC_PRIO_BITS) - 1UL); /* set Priority for Systick Interrupt */ 00778 SysTick->VAL = 0UL; /* Load the SysTick Counter Value */ 00779 SysTick->CTRL = SysTick_CTRL_CLKSOURCE_Msk | 00780 SysTick_CTRL_TICKINT_Msk | 00781 SysTick_CTRL_ENABLE_Msk; /* Enable SysTick IRQ and SysTick Timer */ 00782 return (0UL); /* Function successful */ 00783 } 00784 00785 #endif 00786 00787 /*@} end of CMSIS_Core_SysTickFunctions */ 00788 00789 00790 00791 00792 #ifdef __cplusplus 00793 } 00794 #endif 00795 00796 #endif /* __CORE_CM0_H_DEPENDANT */ 00797 00798 #endif /* __CMSIS_GENERIC */ 00799
Generated on Wed Jul 13 2022 07:07:19 by
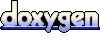