
B+IMU+SD
Dependencies: BMI160 RTC SDFileSystem USBDevice max32630fthr
Fork of MPSMAXbutton by
files.cpp
00001 #include "files.h" 00002 #include "USBSerial.h" 00003 extern Serial _serialport; 00004 00005 #if (MBED_MAJOR_VERSION == 2) 00006 #include "SDFileSystem.h" 00007 #elif (MBED_MAJOR_VERSION == 5) 00008 #include "SDBlockDevice.h" 00009 #include "FATFileSystem.h" 00010 #endif 00011 00012 #if (MBED_MAJOR_VERSION == 2) 00013 SDFileSystem sd(D11, D12, D13, D10, "fs"); // do,di,clk,cs 00014 #elif (MBED_MAJOR_VERSION == 5) 00015 //SDBlockDevice sd(D11, D12, D13, D10, 8000000); 00016 SDBlockDevice sd(SPI0_MOSI, SPI0_MISO, SPI0_SCK, SPI0_SS, 8000000); // For MAX32630FTHR 00017 FATFileSystem fs("fs"); 00018 #endif 00019 00020 00021 int totaFunctionlFiles = 0; 00022 int functionFileNumber = 1; 00023 int functionActive = 0; 00024 extern int functionPosition = 0; 00025 Function ffunc; 00026 00027 int initSDCard() 00028 { 00029 #if (MBED_MAJOR_VERSION == 5) 00030 /* Init SD CARD reader */ 00031 sd.init(); 00032 fs.mount(&sd); 00033 #endif 00034 FILE* fp = fopen("/fs/mydata.txt", "a"); 00035 if (fp != 0) { 00036 _serialport.printf("writing something\n\r\n"); 00037 fprintf(fp,"writing something\n\r\n"); 00038 } else { 00039 _serialport.printf("ERROR\r\n"); 00040 } 00041 fclose(fp); 00042 return 0; 00043 } 00044 00045 int readFileNames() 00046 { 00047 DIR *d; 00048 struct dirent *p; 00049 00050 d = opendir("/fs/functions"); 00051 if (d != NULL) { 00052 while ((p = readdir(d)) != NULL) { 00053 // printf(" - %s\n", p->d_name); 00054 totaFunctionlFiles++; 00055 } 00056 } else { 00057 printf("Could not open directory!\n"); 00058 } 00059 closedir(d); 00060 printf("Total files = %d\n", totaFunctionlFiles); 00061 return 0; 00062 } 00063 00064 int navFunctionFiles(int direction) 00065 { 00066 00067 if(direction == DIR_UP) { 00068 if(functionFileNumber < totaFunctionlFiles) { 00069 functionFileNumber++; 00070 } else { 00071 functionFileNumber = 1; 00072 } 00073 printf("UP: %d\n", functionFileNumber); 00074 } else { 00075 if(functionFileNumber > 1) { 00076 functionFileNumber--; 00077 } else { 00078 functionFileNumber = totaFunctionlFiles; 00079 } 00080 printf("DOWN: %d\n", functionFileNumber); 00081 } 00082 return 0; 00083 } 00084 00085 int openFunctionFile(int inpFile) 00086 { 00087 char fileLoc [40]; 00088 sprintf (fileLoc, "/fs/functions/function%d.txt", inpFile); 00089 printf ("[%s] is location %d file number\n", fileLoc, inpFile); 00090 00091 FILE* fp = fopen(fileLoc, "r"); 00092 ffunc.pos=0; 00093 while (fscanf(fp, "%d,%d", &ffunc.x[ffunc.pos], &ffunc.t[ffunc.pos]) != EOF) { 00094 ffunc.pos++; 00095 } 00096 00097 fclose(fp); 00098 functionActive = 1; 00099 functionPosition = 0; 00100 printFunctionData(); 00101 return 0; 00102 } 00103 00104 void printFunctionData() 00105 { 00106 printf ("total: %d\n", ffunc.pos); 00107 for(int i=0; i<ffunc.pos; i++ ) { 00108 printf ("%d, %d\n", ffunc.x[i], ffunc.t[i]); 00109 } 00110 } 00111
Generated on Tue Jul 12 2022 19:03:03 by
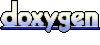