
This is a test program for the ADS1256 ADC transducer together with the DISCO-L475G STM32 board.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "ADS1256.h" 00003 00004 #define SPI_MOSI D11 00005 #define SPI_MISO D12 00006 #define SPI_SCLK D13 00007 #define CHIP_SLCT D9 00008 #define CHANNEL_NUM 4 00009 00010 #define NO_OF_SENSORS 4 // 1- 1 sensor, 2- 2 sensors, 3- 3 sensors, 4- 4 sensors 00011 00012 DigitalIn ndrdy = D10; 00013 DigitalOut cs = CHIP_SLCT; 00014 00015 Serial pc(USBTX, USBRX); 00016 00017 SPI spi(SPI_MOSI, SPI_MISO, SPI_SCLK); 00018 ADS1256 ads(&spi, &ndrdy, &cs); 00019 00020 int32_t ads_sum = 0; 00021 00022 /* 00023 @brief: Settling time using the Input Multiplexer. 00024 */ 00025 #if NO_OF_SENSORS == 1 00026 void readDataMux() 00027 { 00028 uint8_t channel = 0; 00029 ads.readDiffChannel(channel); 00030 00031 for (int i=0; i<CHANNEL_NUM; i++) 00032 { 00033 ads_sum+= ads.adcNow[i]; 00034 } 00035 } 00036 #elif NO_OF_SENSORS == 2 00037 void readDataMux() 00038 { 00039 uint8_t channel = 0; 00040 while(channel < (CHANNEL_NUM-2)) 00041 { 00042 ads.readDiffChannel(++channel); 00043 } 00044 channel = 0; 00045 for (int i=0; i<CHANNEL_NUM; i++) 00046 { 00047 ads_sum+= ads.adcNow[i]; 00048 } 00049 } 00050 #elif NO_OF_SENSORS == 3 00051 void readDataMux() 00052 { 00053 uint8_t channel = 0; 00054 while(channel < CHANNEL_NUM-1) 00055 { 00056 ads.readDiffChannel(++channel); 00057 } 00058 channel = 0; 00059 for (int i=0; i<CHANNEL_NUM; i++) 00060 { 00061 ads_sum+= ads.adcNow[i]; 00062 } 00063 } 00064 #elif NO_OF_SENSORS == 4 00065 void readDataMux() 00066 { 00067 uint8_t channel = 0; 00068 while(channel < CHANNEL_NUM) 00069 { 00070 ads.readDiffChannel(++channel); 00071 } 00072 channel = 0; 00073 for (int i=0; i<CHANNEL_NUM; i++) 00074 { 00075 ads_sum+= ads.adcNow[i]; 00076 } 00077 } 00078 #else 00079 void readDataMux() 00080 { 00081 pc.printf("INVALID NUMBER OF SENSORS INITIALIZED\n"); 00082 } 00083 #endif 00084 00085 void process(void) 00086 { 00087 00088 readDataMux(); 00089 pc.printf("The total value read is %d\n", ads_sum); 00090 ads_sum=0; 00091 wait_us(1000); 00092 00093 pc.printf("The value in adcNow[0] is %d\n", ads.adcNow[0]); 00094 pc.printf("The value in adcNow[1] is %d\n", ads.adcNow[1]); 00095 pc.printf("The value in adcNow[2] is %d\n", ads.adcNow[2]); 00096 pc.printf("The value in adcNow[3] is %d\n", ads.adcNow[3]); 00097 } 00098 00099 int main() 00100 { 00101 /* @brief Set the configuration parameters: 00102 channel == 0(0x01h), 00103 PGA gain == 64, 00104 Buffer == 1 (enabled), 00105 Datarate == 2.5SPS. 00106 Auto_calibration has also been enabled 00107 */ 00108 ads.cfgADC(); 00109 pc.printf("Device configuration is successful\n"); 00110 ads.setDiffChannel(); // Redundancy feature just to ensure that the channel is set correctly. 00111 pc.printf("The set gain value is %d\n", ads.getGainVal()); 00112 00113 /*TODO: Calibration*/ 00114 ads.selfCal(); 00115 ads.sysOffCal(); 00116 00117 while(1) 00118 { 00119 process(); 00120 wait_ms(500); 00121 } 00122 }
Generated on Mon Jul 18 2022 05:45:59 by
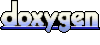