Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_ll_utils.h
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_ll_utils.h 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief Header file of UTILS LL module. 00008 @verbatim 00009 ============================================================================== 00010 ##### How to use this driver ##### 00011 ============================================================================== 00012 [..] 00013 The LL UTILS driver contains a set of generic APIs that can be 00014 used by user: 00015 (+) Device electronic signature 00016 (+) Timing functions 00017 00018 @endverbatim 00019 ****************************************************************************** 00020 * @attention 00021 * 00022 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00023 * 00024 * Redistribution and use in source and binary forms, with or without modification, 00025 * are permitted provided that the following conditions are met: 00026 * 1. Redistributions of source code must retain the above copyright notice, 00027 * this list of conditions and the following disclaimer. 00028 * 2. Redistributions in binary form must reproduce the above copyright notice, 00029 * this list of conditions and the following disclaimer in the documentation 00030 * and/or other materials provided with the distribution. 00031 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00032 * may be used to endorse or promote products derived from this software 00033 * without specific prior written permission. 00034 * 00035 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00036 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00037 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00038 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00039 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00040 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00041 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00042 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00043 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00044 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00045 * 00046 ****************************************************************************** 00047 */ 00048 00049 /* Define to prevent recursive inclusion -------------------------------------*/ 00050 #ifndef __STM32L4xx_LL_UTILS_H 00051 #define __STM32L4xx_LL_UTILS_H 00052 00053 #ifdef __cplusplus 00054 extern "C" { 00055 #endif 00056 00057 /* Includes ------------------------------------------------------------------*/ 00058 #include "stm32l4xx.h" 00059 00060 /** @addtogroup STM32L4xx_LL_Driver 00061 * @{ 00062 */ 00063 00064 /** @defgroup UTILS_LL UTILS 00065 * @{ 00066 */ 00067 00068 /* Private types -------------------------------------------------------------*/ 00069 /* Private variables ---------------------------------------------------------*/ 00070 00071 /* Private constants ---------------------------------------------------------*/ 00072 /** @defgroup UTILS_LL_Private_Constants UTILS Private Constants 00073 * @{ 00074 */ 00075 00076 /* Max delay can be used in LL_mDelay */ 00077 #define LL_MAX_DELAY (uint32_t)0xFFFFFFFF 00078 00079 /** 00080 * @brief Unique device ID register base address 00081 */ 00082 #define UID_BASE_ADDRESS (uint32_t)0x1FFF7590 00083 00084 /** 00085 * @brief Flash size data register base address 00086 */ 00087 #define FLASHSIZE_BASE_ADDRESS (uint32_t)0x1FFF75E0 00088 00089 /** 00090 * @brief Package data register base address 00091 */ 00092 #define PACKAGESIZE_BASE_ADDRESS (uint32_t)0x1FFF7500 00093 00094 /** 00095 * @} 00096 */ 00097 00098 /* Private macros ------------------------------------------------------------*/ 00099 00100 /* Exported types ------------------------------------------------------------*/ 00101 /* Exported constants --------------------------------------------------------*/ 00102 /** @defgroup UTILS_LL_Exported_Constants UTILS Exported Constants 00103 * @{ 00104 */ 00105 00106 /** @defgroup UTILS_EC_PACKAGETYPE PACKAGE TYPE 00107 * @{ 00108 */ 00109 #define LL_UTILS_PACKAGETYPE_LQFP64 (uint32_t)0x00000000 /*!< LQFP64 package type */ 00110 #define LL_UTILS_PACKAGETYPE_LQPF100 (uint32_t)0x00000002 /*!< LQFP100 package type */ 00111 #define LL_UTILS_PACKAGETYPE_BGA132 (uint32_t)0x00000003 /*!< BGA132 package type */ 00112 #define LL_UTILS_PACKAGETYPE_LQFP144_CSP72 (uint32_t)0x00000004 /*!< LQFP144, WLCSP81 or WLCSP72 package type */ 00113 /** 00114 * @} 00115 */ 00116 00117 /** 00118 * @} 00119 */ 00120 00121 /* Exported macro ------------------------------------------------------------*/ 00122 00123 /* Exported functions --------------------------------------------------------*/ 00124 /** @defgroup UTILS_LL_Exported_Functions UTILS Exported Functions 00125 * @{ 00126 */ 00127 00128 /** @defgroup UTILS_EF_DEVICE_ELECTRONIC_SIGNATURE DEVICE ELECTRONIC SIGNATURE 00129 * @{ 00130 */ 00131 00132 /** 00133 * @brief Get Word0 of the unique device identifier (UID based on 96 bits) 00134 * @retval UID[31:0]: X and Y coordinates on the wafer expressed in BCD format 00135 */ 00136 __STATIC_INLINE uint32_t LL_GetUID_Word0(void) 00137 { 00138 return (uint32_t)(READ_REG(*((uint32_t *)UID_BASE_ADDRESS))); 00139 } 00140 00141 /** 00142 * @brief Get Word1 of the unique device identifier (UID based on 96 bits) 00143 * @retval UID[63:32]: Wafer number (UID[39:32]) & LOT_NUM[23:0] (UID[63:40]) 00144 */ 00145 __STATIC_INLINE uint32_t LL_GetUID_Word1(void) 00146 { 00147 return (uint32_t)(READ_REG(*((uint32_t *)(UID_BASE_ADDRESS + 4)))); 00148 } 00149 00150 /** 00151 * @brief Get Word2 of the unique device identifier (UID based on 96 bits) 00152 * @retval UID[95:64]: Lot number (ASCII encoded) - LOT_NUM[31:24] 00153 */ 00154 __STATIC_INLINE uint32_t LL_GetUID_Word2(void) 00155 { 00156 return (uint32_t)(READ_REG(*((uint32_t *)(UID_BASE_ADDRESS + 8)))); 00157 } 00158 00159 /** 00160 * @brief Get Flash memory size 00161 * @note This bitfield indicates the size of the device Flash memory expressed in 00162 * Kbytes. As an example, 0x040 corresponds to 64 Kbytes. 00163 * @retval FLASH_SIZE[15:0]: Flash memory size 00164 */ 00165 __STATIC_INLINE uint32_t LL_GetFlashSize(void) 00166 { 00167 return (uint16_t)(READ_REG(*((uint32_t *)FLASHSIZE_BASE_ADDRESS))); 00168 } 00169 00170 /** 00171 * @brief Get Package type 00172 * @retval Returned value can be one of the following values: 00173 * @arg @ref LL_UTILS_PACKAGETYPE_LQFP64 00174 * @arg @ref LL_UTILS_PACKAGETYPE_LQPF100 00175 * @arg @ref LL_UTILS_PACKAGETYPE_BGA132 00176 * @arg @ref LL_UTILS_PACKAGETYPE_LQFP144_CSP72 00177 */ 00178 __STATIC_INLINE uint32_t LL_GetPackageType(void) 00179 { 00180 return (uint8_t)(READ_REG(*((uint32_t *)PACKAGESIZE_BASE_ADDRESS))); 00181 } 00182 00183 /** 00184 * @} 00185 */ 00186 00187 /** @defgroup UTILS_EF_DELAY DELAY 00188 * @{ 00189 */ 00190 00191 /** 00192 * @brief This function provides accurate delay (in milliseconds) based 00193 * on SysTick counter flag 00194 * @note To respect 1ms timebase, user should call LL_InitTick function which 00195 * will configure Systick to 1ms 00196 * @param Delay specifies the delay time length, in milliseconds. 00197 * @retval None 00198 */ 00199 __STATIC_INLINE void LL_mDelay(uint32_t Delay) 00200 { 00201 volatile uint32_t tmp = SysTick->CTRL; /* Clear the COUNTFLAG first */ 00202 ((void)tmp); 00203 00204 /* Add a period to guaranty minimum wait */ 00205 if (Delay < LL_MAX_DELAY) 00206 { 00207 Delay++; 00208 } 00209 00210 while (Delay) 00211 { 00212 if ((SysTick->CTRL & SysTick_CTRL_COUNTFLAG_Msk) != 0) 00213 { 00214 Delay--; 00215 } 00216 } 00217 } 00218 00219 /** 00220 * @brief This function configures the source of the time base. 00221 * @note The time source is configured to have 1ms time base. 00222 * @param HCLKFrequency HCLK frequency in Hz (can be calculated thanks to RCC helper macro) 00223 * @retval None 00224 */ 00225 __STATIC_INLINE void LL_Init1msTick(uint32_t HCLKFrequency) 00226 { 00227 /* Configure the SysTick to have interrupt in 1ms time base */ 00228 SysTick->LOAD = (uint32_t)((HCLKFrequency / 1000) - 1UL); /* set reload register */ 00229 SysTick->VAL = 0UL; /* Load the SysTick Counter Value */ 00230 SysTick->CTRL = SysTick_CTRL_CLKSOURCE_Msk | 00231 SysTick_CTRL_ENABLE_Msk; /* Enable the Systick Timer */ 00232 } 00233 00234 /** 00235 * @} 00236 */ 00237 00238 /** @defgroup UTILS_EF_SYSTEM SYSTEM 00239 * @{ 00240 */ 00241 00242 /** 00243 * @brief This function sets directly SystemCoreClock CMSIS variable. 00244 * @note Variable can be calculated also through SystemCoreClockUpdate function. 00245 * @param HCLKFrequency HCLK frequency in Hz (can be calculated thanks to RCC helper macro) 00246 * @retval None 00247 */ 00248 __STATIC_INLINE void LL_SetSystemCoreClock(uint32_t HCLKFrequency) 00249 { 00250 /* HCLK clock frequency */ 00251 SystemCoreClock = HCLKFrequency; 00252 } 00253 00254 /** 00255 * @} 00256 */ 00257 00258 00259 /** 00260 * @} 00261 */ 00262 00263 /** 00264 * @} 00265 */ 00266 00267 /** 00268 * @} 00269 */ 00270 00271 #ifdef __cplusplus 00272 } 00273 #endif 00274 00275 #endif /* __STM32L4xx_LL_UTILS_H */ 00276 00277 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00278
Generated on Tue Jul 12 2022 11:35:17 by
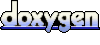