
MAX30001-MAX32630FTHR SYS EvKit
Dependencies: USBDevice max32630fthr
StringInOut.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 #include "mbed.h" 00034 #include "USBSerial.h" 00035 #include "RpcFifo.h" 00036 #include "RpcServer.h" 00037 #include "StringInOut.h" 00038 #include "Peripherals.h" 00039 00040 /// a running index that keeps track of where an incoming string has been 00041 /// buffered to 00042 static int lineBuffer_index = 0; 00043 /// a flag that keeps track of the state of accumulating a string 00044 static int getLine_State = GETLINE_WAITING; 00045 00046 /** 00047 * @brief Place incoming USB characters into a fifo 00048 * @param data_IN buffer of characters 00049 * @param len length of data 00050 */ 00051 int fifoIncomingChars(uint8_t data_IN[], unsigned int len) { 00052 int i; 00053 for (i = 0; i < len; i++) { 00054 fifo_put8(GetUSBIncomingFifo(), data_IN[i]); 00055 } 00056 return 0; 00057 } 00058 00059 /** 00060 * @brief Check the USB incoming fifo to see if there is data to be read 00061 * @return 1 if there is data to be read, 0 if data is not available 00062 */ 00063 int isReadReady(void) { 00064 if (fifo_empty(GetUSBIncomingFifo()) == 0) 00065 return 1; 00066 return 0; 00067 } 00068 00069 /** 00070 * @brief Clear the incoming USB read fifo 00071 */ 00072 void clearOutReadFifo(void) { fifo_clear(GetUSBIncomingFifo()); } 00073 00074 /** 00075 * @brief Block until a character can be read from the USB 00076 * @return the character read 00077 */ 00078 char getch(void) { 00079 uint8_t ch; 00080 // block until char is ready 00081 while (isReadReady() == 0) { 00082 } 00083 // read a char from buffer 00084 fifo_get8(GetUSBIncomingFifo(), &ch); 00085 return ch; 00086 } 00087 00088 /** 00089 * @brief Place incoming USB characters into a fifo 00090 * @param lineBuffer buffer to place the incoming characters 00091 * @param bufferLength length of buffer 00092 * @return GETLINE_WAITING if still waiting for a CRLF, GETLINE_DONE 00093 */ 00094 int getLine(char *lineBuffer, int bufferLength) { 00095 uint8_t ch; 00096 00097 USBSerial *serial = Peripherals::usbSerial(); 00098 if (getLine_State == GETLINE_DONE) { 00099 getLine_State = GETLINE_WAITING; 00100 } 00101 if (serial->available() != 0) { 00102 ch = serial->_getc(); 00103 if (ch != 0x0A && ch != 0x0D) { 00104 lineBuffer[lineBuffer_index++] = ch; 00105 } 00106 if (ch == 0x0D) { 00107 lineBuffer[lineBuffer_index++] = 0; 00108 lineBuffer_index = 0; 00109 getLine_State = GETLINE_DONE; 00110 } 00111 if (lineBuffer_index > bufferLength) { 00112 lineBuffer[bufferLength - 1] = 0; 00113 getLine_State = GETLINE_DONE; 00114 } 00115 } 00116 return getLine_State; 00117 } 00118 00119 /** 00120 * @brief Block until a fixed number of characters has been accumulated from the 00121 * incoming USB 00122 * @param lineBuffer buffer to place the incoming characters 00123 * @param maxLength length of buffer 00124 */ 00125 void getStringFixedLength(uint8_t *lineBuffer, int maxLength) { 00126 uint8_t ch; 00127 int index = 0; 00128 // block until maxLength is captured 00129 while (1) { 00130 ch = getch(); 00131 lineBuffer[index++] = ch; 00132 if (index == maxLength) 00133 return; 00134 } 00135 } 00136 00137 /** 00138 * @brief Output a string out the USB serial port 00139 * @param str output this str the USB channel 00140 */ 00141 int putStr(const char *str) { 00142 Peripherals::usbSerial()->printf("%s", str); // fflush(stdout); 00143 // uint8_t *ptr; 00144 // uint8_t buffer[256]; 00145 // int index = 0; 00146 /* int length; 00147 ptr = (uint8_t *)str; 00148 length = strlen(str); 00149 00150 Peripherals::usbSerial()->writeBlock(ptr,length); */ 00151 return 0; 00152 } 00153 00154 /** 00155 * @brief Outut an array of bytes out the USB serial port 00156 * @param data buffer to output 00157 * @param length length of buffer 00158 */ 00159 int putBytes(uint8_t *data, uint32_t length) { 00160 int sendThis = 64; 00161 int sent = 0; 00162 int thisLeft; 00163 uint8_t *ptr = data; 00164 if (length < 64) 00165 sendThis = length; 00166 do { 00167 Peripherals::usbSerial()->writeBlock(ptr, sendThis); 00168 sent += sendThis; 00169 ptr += sendThis; 00170 thisLeft = length - sent; 00171 sendThis = 64; 00172 if (thisLeft < 64) 00173 sendThis = thisLeft; 00174 } while (sent != length); 00175 return 0; 00176 } 00177 00178 /** 00179 * @brief Outut 256 byte blocks out the USB serial using writeBlock bulk 00180 * transfers 00181 * @param data buffer of blocks to output 00182 * @param length length of 256-byte blocks 00183 */ 00184 int putBytes256Block(uint8_t *data, int numberBlocks) { 00185 int i; 00186 uint8_t *ptr; 00187 ptr = data; 00188 const int BLOCK_SIZE = 32; 00189 const int FLASH_PAGE_SIZE = 256; 00190 for (i = 0; i < numberBlocks * (FLASH_PAGE_SIZE / BLOCK_SIZE); i++) { 00191 Peripherals::usbSerial()->writeBlock(ptr, BLOCK_SIZE); 00192 ptr += BLOCK_SIZE; 00193 } 00194 return 0; 00195 } 00196
Generated on Tue Jul 12 2022 16:59:43 by
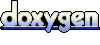