
MAX30001-MAX32630FTHR SYS EvKit
Dependencies: USBDevice max32630fthr
S25FS512_RPC.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 00034 #include "S25FS512_RPC.h" 00035 #include "S25FS512.h" 00036 #include "StringInOut.h" 00037 #include "StringHelper.h" 00038 #include "Peripherals.h" 00039 #include "SDBlockDevice.h" 00040 00041 int S25FS512_Reset(char argStrs[32][32], char replyStrs[32][32]) { 00042 uint32_t reply[1]; 00043 Peripherals::s25FS512()->reset(); 00044 reply[0] = 0x80; 00045 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00046 return 0; 00047 } 00048 00049 int S25FS512_EnableHWReset(char argStrs[32][32], char replyStrs[32][32]) { 00050 uint32_t reply[1]; 00051 Peripherals::s25FS512()->enableHWReset(); 00052 reply[0] = 0x80; 00053 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00054 return 0; 00055 } 00056 00057 int S25FS512_SpiWriteRead(char argStrs[32][32], char replyStrs[32][32]) { 00058 uint8_t args[16]; 00059 uint8_t reply[16]; 00060 uint8_t writeNumber; 00061 uint8_t readNumber; 00062 // get the number of bytes to write 00063 ProcessArgs(argStrs, args, 1); 00064 writeNumber = args[0]; 00065 ProcessArgs(argStrs, args, writeNumber + 2); 00066 readNumber = args[writeNumber + 1]; 00067 FormatReply(reply, readNumber, replyStrs); 00068 return 0; 00069 } 00070 00071 int S25FS512_SpiWriteRead4Wire(char argStrs[32][32], char replyStrs[32][32]) { 00072 uint8_t args[16]; 00073 uint8_t reply[16]; 00074 uint8_t writeNumber; 00075 uint8_t readNumber; 00076 // get the number of bytes to write 00077 ProcessArgs(argStrs, args, 1); 00078 writeNumber = args[0]; 00079 ProcessArgs(argStrs, args, writeNumber + 2); 00080 readNumber = args[writeNumber + 1]; 00081 FormatReply(reply, readNumber, replyStrs); 00082 return 0; 00083 } 00084 00085 int S25FS512_ReadPage(char argStrs[32][32], char replyStrs[32][32]) { 00086 uint32_t args[2]; 00087 uint32_t reply[1]; 00088 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00089 reply[0] = 0x80; 00090 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00091 return 0; 00092 } 00093 00094 //int S25FS512_ReadPagesBinary(char argStrs[32][32], char replyStrs[32][32]) { 00095 // uint32_t args[2]; 00096 // uint32_t reply[1]; 00097 // uint8_t pageData[256]; 00098 // 00099 // uint32_t startPage; 00100 // uint32_t endPage; 00101 // uint32_t page; 00102 // 00103 // ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00104 // startPage = args[0]; 00105 // endPage = args[1]; 00106 // for (page = startPage; page <= endPage; page++) { 00107 // Peripherals::s25FS512()->readPages_Helper(page, page, pageData, 0); 00108 // putBytes256Block(pageData, 1); 00109 // } 00110 // reply[0] = 0x80; 00111 // FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00112 // return 0; 00113 //} 00114 extern char dataFileName[32]; 00115 int S25FS512_ReadPagesBinary(char argStrs[32][32], char replyStrs[32][32]) { 00116 uint32_t args[2]; 00117 uint32_t reply[1]; 00118 uint8_t pageData[256]; 00119 00120 uint32_t startPage; 00121 uint32_t endPage; 00122 uint32_t page; 00123 00124 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00125 startPage = args[0]; 00126 endPage = args[1]; 00127 FILE *fp = NULL; 00128 fp = fopen(dataFileName, "rb"); 00129 for (page = startPage-0x12; page <= endPage-0x12; page++) { 00130 memset(pageData, 0xffffffff, sizeof(pageData)); 00131 printf("reading from page %d\r\n", page); 00132 if (fp != NULL) { 00133 fseek(fp,page*256,SEEK_SET); 00134 uint8_t count = 0; 00135 while(!feof(fp)) 00136 { 00137 printf("."); 00138 pageData[count++] = (unsigned char) fgetc(fp); 00139 if (count == 0) break; 00140 }; 00141 } 00142 printf("\r\nEND\r\n"); 00143 putBytes256Block(pageData, 1); 00144 } 00145 if (fp != NULL) fclose(fp); 00146 reply[0] = 0x80; 00147 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00148 return 0; 00149 } 00150 00151 int S25FS512_ReadId(char argStrs[32][32], char replyStrs[32][32]) { 00152 char str[32]; 00153 uint8_t data[128]; 00154 Peripherals::s25FS512()->readIdentification(data, sizeof(data)); 00155 Peripherals::s25FS512()->readIdentification(data, sizeof(data)); 00156 sprintf(str, "%02X%02X%02X%02X", data[0], data[1], data[2], data[3]); 00157 strcpy(replyStrs[0], str); 00158 return 0; 00159 } 00160 int SDCard_IsReady(char argStrs[32][32], char replyStrs[32][32]) { 00161 00162 DigitalIn *detect = Peripherals::SDDetect(); 00163 00164 bool isReady = false; 00165 00166 if(detect->read()) 00167 { 00168 isReady = false; 00169 strcpy(replyStrs[0], "not_ok"); 00170 return 0; 00171 } 00172 00173 Peripherals::sdFS()->init(); 00174 FILE *fp = fopen("/sd/test", "r"); 00175 if ( fp != NULL) 00176 isReady = true; 00177 else 00178 { 00179 FILE *fp = fopen("/sd/test", "w"); 00180 if ( fp != NULL) 00181 isReady = true; 00182 } 00183 00184 if (fp != NULL) fclose(fp); 00185 00186 if (isReady) 00187 strcpy(replyStrs[0], "ok"); 00188 else 00189 strcpy(replyStrs[0], "not_ok"); 00190 return 0; 00191 }
Generated on Tue Jul 12 2022 16:59:43 by
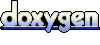