
MAX30001-MAX32630FTHR SYS EvKit
Dependencies: USBDevice max32630fthr
MAX30001_RPC.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 00034 #include <stdio.h> 00035 #include "StringHelper.h" 00036 #include "MAX30001.h" 00037 #include "Streaming.h" 00038 #include "StringInOut.h" 00039 #include "MAX30001_helper.h" 00040 #include "RpcFifo.h" 00041 #include "RpcServer.h" 00042 #include "Peripherals.h" 00043 #include "DataLoggingService.h" 00044 00045 int highDataRate = 0; 00046 00047 uint32_t max30001_RegRead(MAX30001::MAX30001_REG_map_t addr) { 00048 uint32_t data; 00049 Peripherals::max30001()->max30001_reg_read(addr, &data); 00050 return data; 00051 } 00052 00053 void max30001_RegWrite(MAX30001::MAX30001_REG_map_t addr, uint32_t data) { 00054 Peripherals::max30001()->max30001_reg_write(addr, data); 00055 } 00056 00057 int MAX30001_WriteReg(char argStrs[32][32], char replyStrs[32][32]) { 00058 uint32_t args[2]; 00059 uint32_t reply[1]; 00060 uint32_t value; 00061 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00062 00063 max30001_RegWrite((MAX30001::MAX30001_REG_map_t)args[0], args[1]); 00064 reply[0] = 0x80; 00065 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00066 return 0; 00067 } 00068 00069 int MAX30001_ReadReg(char argStrs[32][32], char replyStrs[32][32]) { 00070 uint32_t args[1]; 00071 uint32_t reply[1]; 00072 uint32_t value; 00073 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00074 value = max30001_RegRead((MAX30001::MAX30001_REG_map_t)args[0]); 00075 reply[0] = value; 00076 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00077 return 0; 00078 } 00079 00080 int MAX30001_Rbias_FMSTR_Init(char argStrs[32][32], char replyStrs[32][32]) { 00081 uint32_t args[5]; 00082 uint32_t reply[1]; 00083 uint32_t value; 00084 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00085 00086 value = Peripherals::max30001()->max30001_Rbias_FMSTR_Init(args[0], // En_rbias 00087 args[1], // Rbiasv 00088 args[2], // Rbiasp 00089 args[3], // Rbiasn 00090 args[4]); // Fmstr 00091 00092 reply[0] = value; 00093 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00094 return 0; 00095 } 00096 00097 int MAX30001_CAL_InitStart(char argStrs[32][32], char replyStrs[32][32]) { 00098 uint32_t args[6]; 00099 uint32_t reply[1]; 00100 uint32_t value; 00101 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00102 00103 // Peripherals::serial()->printf("MAX30001_CAL_InitStart 0 "); 00104 value = Peripherals::max30001()->max30001_CAL_InitStart(args[0], // En_Vcal 00105 args[1], // Vmag 00106 args[2], // Fcal 00107 args[3], // Thigh 00108 args[4], // Fifty 00109 args[5]); // Vmode 00110 00111 reply[0] = value; 00112 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00113 return 0; 00114 } 00115 00116 int MAX30001_ECG_InitStart(char argStrs[32][32], char replyStrs[32][32]) { 00117 uint32_t args[11]; 00118 uint32_t reply[1]; 00119 uint32_t value; 00120 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00121 00122 // Peripherals::serial()->printf("MAX30001_ECG_InitStart 0 "); 00123 value = Peripherals::max30001()->max30001_ECG_InitStart(args[0], // En_ecg 00124 args[1], // Openp 00125 args[2], // Openn 00126 args[3], // Pol 00127 args[4], // Calp_sel 00128 args[5], // Caln_sel 00129 args[6], // E_fit 00130 args[7], // Rate 00131 args[8], // Gain 00132 args[9], // Dhpf 00133 args[10]); // Dlpf 00134 // Peripherals::serial()->printf("MAX30001_ECG_InitStart 1 "); 00135 MAX30001_Helper_SetStreamingFlag(eStreaming_ECG, 1); 00136 reply[0] = value; 00137 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00138 return 0; 00139 } 00140 00141 int MAX30001_ECGFast_Init(char argStrs[32][32], char replyStrs[32][32]) { 00142 uint32_t args[3]; 00143 uint32_t reply[1]; 00144 uint32_t value; 00145 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00146 00147 value = Peripherals::max30001()->max30001_ECGFast_Init(args[0], // Clr_Fast 00148 args[1], // Fast 00149 args[2]); // Fast_Th 00150 00151 reply[0] = value; 00152 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00153 return 0; 00154 } 00155 00156 int MAX30001_PACE_InitStart(char argStrs[32][32], char replyStrs[32][32]) { 00157 uint32_t args[9]; 00158 uint32_t reply[1]; 00159 uint32_t value; 00160 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00161 00162 value = 00163 Peripherals::max30001()->max30001_PACE_InitStart(args[0], // En_pace 00164 args[1], // Clr_pedge 00165 args[2], // Pol 00166 args[3], // Gn_diff_off 00167 args[4], // Gain 00168 args[5], // Aout_lbw 00169 args[6], // Aout 00170 args[7], // Dacp 00171 args[8]); // Dacn 00172 00173 MAX30001_Helper_SetStreamingFlag(eStreaming_PACE, 1); 00174 reply[0] = value; 00175 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00176 return 0; 00177 } 00178 00179 int MAX30001_BIOZ_InitStart(char argStrs[32][32], char replyStrs[32][32]) { 00180 uint32_t args[18]; 00181 uint32_t reply[1]; 00182 uint32_t value; 00183 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00184 00185 value = Peripherals::max30001()->max30001_BIOZ_InitStart(args[0], // En_bioz 00186 args[1], // Openp 00187 args[2], // Openn 00188 args[3], // Calp_sel 00189 args[4], // Caln_sel 00190 args[5], // CG_mode 00191 args[6], // B_fit 00192 args[7], // Rate 00193 args[8], // Ahpf 00194 args[9], // Ext_rbias 00195 args[10], // Gain 00196 args[11], // Dhpf 00197 args[12], // Dlpf 00198 args[13], // Fcgen 00199 args[14], // Cgmon 00200 args[15], // Cgmag 00201 args[16], // Phoff 00202 args[17]); //INAPow_mode 00203 00204 MAX30001_Helper_SetStreamingFlag(eStreaming_BIOZ, 1); 00205 reply[0] = value; 00206 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00207 return 0; 00208 } 00209 00210 int MAX30001_RtoR_InitStart(char argStrs[32][32], char replyStrs[32][32]) { 00211 uint32_t args[9]; 00212 uint32_t reply[1]; 00213 uint32_t value; 00214 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00215 00216 value = Peripherals::max30001()->max30001_RtoR_InitStart(args[0], // En_rtor 00217 args[1], // Wndw 00218 args[2], // Gain 00219 args[3], // Pavg 00220 args[4], // Ptsf 00221 args[5], // Hoff 00222 args[6], // Ravg 00223 args[7], // Rhsf 00224 args[8]); // Clr_rrint 00225 00226 MAX30001_Helper_SetStreamingFlag(eStreaming_RtoR, 1); 00227 reply[0] = value; 00228 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00229 return 0; 00230 } 00231 00232 int MAX30001_Stop_ECG(char argStrs[32][32], char replyStrs[32][32]) { 00233 uint32_t reply[1]; 00234 Peripherals::max30001()->max30001_Stop_ECG(); 00235 reply[0] = 0x80; 00236 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00237 return 0; 00238 } 00239 int MAX30001_Stop_PACE(char argStrs[32][32], char replyStrs[32][32]) { 00240 uint32_t reply[1]; 00241 Peripherals::max30001()->max30001_Stop_PACE(); 00242 reply[0] = 0x80; 00243 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00244 return 0; 00245 } 00246 int MAX30001_Stop_BIOZ(char argStrs[32][32], char replyStrs[32][32]) { 00247 uint32_t reply[1]; 00248 Peripherals::max30001()->max30001_Stop_BIOZ(); 00249 reply[0] = 0x80; 00250 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00251 return 0; 00252 } 00253 int MAX30001_Stop_RtoR(char argStrs[32][32], char replyStrs[32][32]) { 00254 uint32_t reply[1]; 00255 Peripherals::max30001()->max30001_Stop_RtoR(); 00256 reply[0] = 0x80; 00257 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00258 return 0; 00259 } 00260 int MAX30001_Stop_Cal(char argStrs[32][32], char replyStrs[32][32]) { 00261 uint32_t reply[1]; 00262 // max30001_Stop_Cal(); 00263 reply[0] = 0x80; 00264 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00265 return 0; 00266 } 00267 00268 void max30001_ServiceStreaming() { 00269 char ch; 00270 uint32_t val; 00271 USBSerial *usbSerial = Peripherals::usbSerial(); 00272 00273 fifo_clear(GetStreamOutFifo()); 00274 00275 SetStreaming(TRUE); 00276 clearOutReadFifo(); 00277 while (IsStreaming() == TRUE) { 00278 00279 if (fifo_empty(GetStreamOutFifo()) == 0) { 00280 fifo_get32(GetStreamOutFifo(), &val); 00281 00282 usbSerial->printf("%02X ", val); 00283 00284 } 00285 if (usbSerial->available()) { 00286 ch = usbSerial->_getc(); 00287 00288 MAX30001_Helper_Stop(); 00289 SetStreaming(FALSE); 00290 fifo_clear(GetUSBIncomingFifo()); // clear USB serial incoming fifo 00291 fifo_clear(GetStreamOutFifo()); 00292 } 00293 00294 } 00295 } 00296 00297 int MAX30001_Start(char argStrs[32][32], char replyStrs[32][32]) { 00298 uint32_t reply[1]; 00299 uint32_t all; 00300 fifo_clear(GetUSBIncomingFifo()); 00301 Peripherals::max30001()->max30001_synch(); 00302 // max30001_ServiceStreaming(); 00303 highDataRate = 0; 00304 Peripherals::max30001()->max30001_reg_read(MAX30001::STATUS, &all); 00305 LoggingService_StartLoggingUsb(); 00306 00307 reply[0] = 0x80; 00308 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00309 return 0; 00310 } 00311 00312 int MAX30001_Stop(char argStrs[32][32], char replyStrs[32][32]) { 00313 /* uint32_t args[1]; 00314 uint32_t reply[1]; 00315 uint32_t value; 00316 //ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00317 max30001_StopTest(); 00318 reply[0] = 0x80; 00319 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs);*/ 00320 return 0; 00321 } 00322 00323 int MAX30001_INT_assignment(char argStrs[32][32], char replyStrs[32][32]) { 00324 uint32_t args[17]; 00325 uint32_t reply[1]; 00326 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00327 /* 00328 printf("MAX30001_INT_assignment "); 00329 printf("%d ",args[0]); 00330 printf("%d ",args[1]); 00331 printf("%d ",args[2]); 00332 printf("%d ",args[3]); 00333 printf("%d ",args[4]); 00334 printf("%d ",args[5]); 00335 printf("%d ",args[6]); 00336 printf("%d ",args[7]); 00337 printf("%d ",args[8]); 00338 printf("%d ",args[9]); 00339 printf("%d ",args[10]); 00340 printf("%d ",args[11]); 00341 printf("%d ",args[12]); 00342 printf("%d ",args[13]); 00343 printf("%d ",args[14]); 00344 printf("%d ",args[15]); 00345 printf("%d ",args[16]); 00346 printf("\n"); 00347 fflush(stdout); 00348 */ 00349 00350 Peripherals::max30001()->max30001_INT_assignment( 00351 (MAX30001::max30001_intrpt_Location_t)args[0], 00352 (MAX30001::max30001_intrpt_Location_t)args[1], 00353 (MAX30001::max30001_intrpt_Location_t)args[2], 00354 (MAX30001::max30001_intrpt_Location_t)args[3], 00355 (MAX30001::max30001_intrpt_Location_t)args[4], 00356 (MAX30001::max30001_intrpt_Location_t)args[5], 00357 (MAX30001::max30001_intrpt_Location_t)args[6], 00358 (MAX30001::max30001_intrpt_Location_t)args[7], 00359 (MAX30001::max30001_intrpt_Location_t)args[8], 00360 (MAX30001::max30001_intrpt_Location_t)args[9], 00361 (MAX30001::max30001_intrpt_Location_t)args[10], 00362 (MAX30001::max30001_intrpt_Location_t)args[11], 00363 (MAX30001::max30001_intrpt_Location_t)args[12], 00364 (MAX30001::max30001_intrpt_Location_t)args[13], 00365 (MAX30001::max30001_intrpt_Location_t)args[14], 00366 (MAX30001::max30001_intrpt_type_t)args[15], 00367 (MAX30001::max30001_intrpt_type_t)args[16]); 00368 reply[0] = 0x80; 00369 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00370 return 0; 00371 } 00372 00373 int MAX30001_StartTest(char argStrs[32][32], char replyStrs[32][32]) { 00374 uint32_t reply[1]; 00375 // ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00376 00377 /*** Set FMSTR over here ****/ 00378 00379 /*** Set and Start the VCAL input ***/ 00380 /* NOTE VCAL must be set first if VCAL is to be used */ 00381 Peripherals::max30001()->max30001_CAL_InitStart(0b1, 0b1, 0b1, 0b011, 0x7FF, 0b0); 00382 00383 /**** ECG Initialization ****/ 00384 Peripherals::max30001()->max30001_ECG_InitStart(0b1, 0b1, 0b1, 0b0, 0b10, 0b11, 31, 0b00, 0b00, 0b0, 0b01); 00385 00386 /***** PACE Initialization ***/ 00387 Peripherals::max30001()->max30001_PACE_InitStart(0b1, 0b0, 0b0, 0b1, 0b000, 0b0, 0b00, 0b0, 0b0); 00388 00389 /**** BIOZ Initialization ****/ 00390 Peripherals::max30001()->max30001_BIOZ_InitStart( 00391 0b1, 0b1, 0b1, 0b10, 0b11, 0b00, 7, 0b0, 0b111, 0b0, 0b10, 0b00, 0b00, 0b0001, 0b0, 0b111, 0b0000, 0b0000); 00392 00393 /*** Set RtoR registers ***/ 00394 Peripherals::max30001()->max30001_RtoR_InitStart( 00395 0b1, 0b0011, 0b1111, 0b00, 0b0011, 0b000001, 0b00, 0b000, 0b01); 00396 00397 /*** Set Rbias & FMSTR over here ****/ 00398 Peripherals::max30001()->max30001_Rbias_FMSTR_Init(0b01, 0b10, 0b1, 0b1, 0b00); 00399 00400 /**** Interrupt Setting ****/ 00401 00402 /*** Set ECG Lead ON/OFF ***/ 00403 // max30001_ECG_LeadOnOff(); 00404 00405 /*** Set BIOZ Lead ON/OFF ***/ 00406 // max30001_BIOZ_LeadOnOff(); Does not work yet... 00407 00408 /**** Do a Synch ****/ 00409 Peripherals::max30001()->max30001_synch(); 00410 00411 fifo_clear(GetUSBIncomingFifo()); 00412 max30001_ServiceStreaming(); 00413 00414 reply[0] = 0x80; 00415 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00416 return 0; 00417 } 00418 /* 00419 static void StopAll() { 00420 if (startedEcg == 1) { 00421 max30001_Stop_ECG(); 00422 } 00423 if (startedCal == 1) { 00424 } 00425 if (startedBioz == 1) { 00426 max30001_Stop_BIOZ(); 00427 } 00428 if (startedPace == 1) { 00429 max30001_Stop_PACE(); 00430 } 00431 if (startedRtor == 1) { 00432 max30001_Stop_RtoR(); 00433 } 00434 startedEcg = 0; 00435 startedBioz = 0; 00436 startedCal = 0; 00437 startedPace = 0; 00438 startedRtor = 0; 00439 } 00440 */ 00441 /* 00442 // switch to ECG DC Lead ON 00443 max30001_Enable_LeadON(0b01); 00444 // switch to BIOZ DC Lead ON 00445 max30001_Enable_LeadON(0b10); 00446 */ 00447 int MAX30001_Enable_ECG_LeadON(char argStrs[32][32], char replyStrs[32][32]) { 00448 uint32_t reply[1]; 00449 // switch to ECG DC Lead ON 00450 Peripherals::max30001()->max30001_Enable_LeadON(0b01); 00451 reply[0] = 0x80; 00452 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00453 return 0; 00454 } 00455 int MAX30001_Enable_BIOZ_LeadON(char argStrs[32][32], char replyStrs[32][32]) { 00456 uint32_t reply[1]; 00457 // switch to BIOZ DC Lead ON 00458 Peripherals::max30001()->max30001_Enable_LeadON(0b10); 00459 reply[0] = 0x80; 00460 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00461 return 0; 00462 } 00463 // uint32_t max30001_LeadOn; // This holds the LeadOn data, BIT1 = BIOZ Lead ON, BIT0 = ECG Lead ON 00464 int MAX30001_Read_LeadON(char argStrs[32][32], char replyStrs[32][32]) { 00465 uint32_t reply[1]; 00466 // return the max30001_LeadOn var from the MAX30001 driver 00467 reply[0] = Peripherals::max30001()->max30001_LeadOn; 00468 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00469 return 0; 00470 } 00471
Generated on Tue Jul 12 2022 16:59:43 by
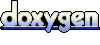