
MAX30001-MAX32630FTHR SYS EvKit
Dependencies: USBDevice max32630fthr
MAX30001.h
00001 /******************************************************************************* 00002 * Copyright (C) 2015 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 *******************************************************************************/ 00032 /* 00033 * max30001.h 00034 * 00035 * Created on: Oct 9, 2015 00036 * Author: faisal.tariq 00037 */ 00038 00039 #ifndef MAX30001_H_ 00040 #define MAX30001_H_ 00041 00042 #include "mbed.h" 00043 00044 #define mbed_COMPLIANT // Uncomment to Use timer for MAX30001 FCLK (for mbed) 00045 // Comment to use the RTC clock 00046 00047 #define ASYNC_SPI_BUFFER_SIZE (32 * 3) // Maximimum buffer size for async byte transfers 00048 00049 // Defines for data callbacks 00050 #define MAX30001_DATA_ECG 0x30 00051 #define MAX30001_DATA_PACE 0x31 00052 #define MAX30001_DATA_RTOR 0x32 00053 #define MAX30001_DATA_BIOZ 0x33 00054 #define MAX30001_DATA_LEADOFF_DC 0x34 00055 #define MAX30001_DATA_LEADOFF_AC 0x35 00056 #define MAX30001_DATA_BCGMON 0x36 00057 #define MAX30001_DATA_ACLEADON 0x37 00058 00059 #define MAX30001_SPI_MASTER_PORT 0 00060 #define MAX30001_SPI_SS_INDEX 0 00061 00062 #define MAX30001_INT_PORT_B 3 00063 #define MAX30001_INT_PIN_B 6 00064 00065 #define MAX30001_INT_PORT_2B 4 00066 #define MAX30001_INT_PIN_2B 5 00067 00068 #define MAX30001_INT_PORT_FCLK 1 00069 #define MAX30001_INT_PIN_FCLK 7 00070 00071 #define MAX30001_FUNC_SEL_TMR 2 // 0=FW Control, 1= Pulse Train, 2=Timer 00072 00073 #define MAX30001_INDEX 3 00074 #define MAX30001_POLARITY 0 00075 #define MAX30001_PERIOD 30518 00076 #define MAX30001_CYCLE 50 00077 00078 #define MAX30001_IOMUX_IO_ENABLE 1 00079 00080 #define MAX30001_SPI_PORT 0 00081 #define MAX30001_CS_PIN 0 00082 #define MAX30001_CS_POLARITY 0 00083 #define MAX30001_CS_ACTIVITY_DELAY 0 00084 #define MAX30001_CS_INACTIVITY_DELAY 0 00085 #define MAX30001_CLK_HI 1 00086 #define MAX30001_CLK_LOW 1 00087 #define MAX30001_ALT_CLK 0 00088 #define MAX30001_CLK_POLARITY 0 00089 #define MAX30001_CLK_PHASE 0 00090 #define MAX30001_WRITE 1 00091 #define MAX30001_READ 0 00092 00093 #define MAX30001_INT_PORT_B 3 00094 #define MAX30001INT_PIN_B 6 00095 00096 void MAX30001_AllowInterrupts(int state); 00097 00098 /** 00099 * Maxim Integrated MAX30001 ECG/BIOZ chip 00100 */ 00101 class MAX30001 { 00102 00103 public: 00104 typedef enum { // MAX30001 Register addresses 00105 STATUS = 0x01, 00106 EN_INT = 0x02, 00107 EN_INT2 = 0x03, 00108 MNGR_INT = 0x04, 00109 MNGR_DYN = 0x05, 00110 SW_RST = 0x08, 00111 SYNCH = 0x09, 00112 FIFO_RST = 0x0A, 00113 INFO = 0x0F, 00114 CNFG_GEN = 0x10, 00115 CNFG_CAL = 0x12, 00116 CNFG_EMUX = 0x14, 00117 CNFG_ECG = 0x15, 00118 CNFG_BMUX = 0x17, 00119 CNFG_BIOZ = 0x18, 00120 CNFG_PACE = 0x1A, 00121 CNFG_RTOR1 = 0x1D, 00122 CNFG_RTOR2 = 0x1E, 00123 00124 // Data locations 00125 ECG_FIFO_BURST = 0x20, 00126 ECG_FIFO = 0x21, 00127 FIFO_BURST = 0x22, 00128 BIOZ_FIFO = 0x23, 00129 RTOR = 0x25, 00130 00131 PACE0_FIFO_BURST = 0x30, 00132 PACE0_A = 0x31, 00133 PACE0_B = 0x32, 00134 PACE0_C = 0x33, 00135 00136 PACE1_FIFO_BURST = 0x34, 00137 PACE1_A = 0x35, 00138 PACE1_B = 0x36, 00139 PACE1_C = 0x37, 00140 00141 PACE2_FIFO_BURST = 0x38, 00142 PACE2_A = 0x39, 00143 PACE2_B = 0x3A, 00144 PACE2_C = 0x3B, 00145 00146 PACE3_FIFO_BURST = 0x3C, 00147 PACE3_A = 0x3D, 00148 PACE3_B = 0x3E, 00149 PACE3_C = 0x3F, 00150 00151 PACE4_FIFO_BURST = 0x40, 00152 PACE4_A = 0x41, 00153 PACE4_B = 0x42, 00154 PACE4_C = 0x43, 00155 00156 PACE5_FIFO_BURST = 0x44, 00157 PACE5_A = 0x45, 00158 PACE5_B = 0x46, 00159 PACE5_C = 0x47, 00160 00161 } MAX30001_REG_map_t; 00162 00163 /** 00164 * @brief STATUS (0x01) 00165 */ 00166 union max30001_status_reg { 00167 uint32_t all; 00168 00169 struct { 00170 uint32_t loff_nl : 1; 00171 uint32_t loff_nh : 1; 00172 uint32_t loff_pl : 1; 00173 uint32_t loff_ph : 1; 00174 00175 uint32_t bcgmn : 1; 00176 uint32_t bcgmp : 1; 00177 uint32_t reserved1 : 1; 00178 uint32_t reserved2 : 1; 00179 00180 uint32_t pllint : 1; 00181 uint32_t samp : 1; 00182 uint32_t rrint : 1; 00183 uint32_t lonint : 1; 00184 00185 uint32_t pedge : 1; 00186 uint32_t povf : 1; 00187 uint32_t pint : 1; 00188 uint32_t bcgmon : 1; 00189 00190 uint32_t bundr : 1; 00191 uint32_t bover : 1; 00192 uint32_t bovf : 1; 00193 uint32_t bint : 1; 00194 00195 uint32_t dcloffint : 1; 00196 uint32_t fstint : 1; 00197 uint32_t eovf : 1; 00198 uint32_t eint : 1; 00199 00200 uint32_t reserved : 8; 00201 00202 } bit; 00203 00204 } max30001_status; 00205 00206 00207 /** 00208 * @brief EN_INT (0x02) 00209 */ 00210 00211 union max30001_en_int_reg { 00212 uint32_t all; 00213 00214 struct { 00215 uint32_t intb_type : 2; 00216 uint32_t reserved1 : 1; 00217 uint32_t reserved2 : 1; 00218 00219 uint32_t reserved3 : 1; 00220 uint32_t reserved4 : 1; 00221 uint32_t reserved5 : 1; 00222 uint32_t reserved6 : 1; 00223 00224 uint32_t en_pllint : 1; 00225 uint32_t en_samp : 1; 00226 uint32_t en_rrint : 1; 00227 uint32_t en_lonint : 1; 00228 00229 uint32_t en_pedge : 1; 00230 uint32_t en_povf : 1; 00231 uint32_t en_pint : 1; 00232 uint32_t en_bcgmon : 1; 00233 00234 uint32_t en_bundr : 1; 00235 uint32_t en_bover : 1; 00236 uint32_t en_bovf : 1; 00237 uint32_t en_bint : 1; 00238 00239 uint32_t en_dcloffint : 1; 00240 uint32_t en_fstint : 1; 00241 uint32_t en_eovf : 1; 00242 uint32_t en_eint : 1; 00243 00244 uint32_t reserved : 8; 00245 00246 } bit; 00247 00248 } max30001_en_int; 00249 00250 00251 /** 00252 * @brief EN_INT2 (0x03) 00253 */ 00254 union max30001_en_int2_reg { 00255 uint32_t all; 00256 00257 struct { 00258 uint32_t intb_type : 2; 00259 uint32_t reserved1 : 1; 00260 uint32_t reserved2 : 1; 00261 00262 uint32_t reserved3 : 1; 00263 uint32_t reserved4 : 1; 00264 uint32_t reserved5 : 1; 00265 uint32_t reserved6 : 1; 00266 00267 uint32_t en_pllint : 1; 00268 uint32_t en_samp : 1; 00269 uint32_t en_rrint : 1; 00270 uint32_t en_lonint : 1; 00271 00272 uint32_t en_pedge : 1; 00273 uint32_t en_povf : 1; 00274 uint32_t en_pint : 1; 00275 uint32_t en_bcgmon : 1; 00276 00277 uint32_t en_bundr : 1; 00278 uint32_t en_bover : 1; 00279 uint32_t en_bovf : 1; 00280 uint32_t en_bint : 1; 00281 00282 uint32_t en_dcloffint : 1; 00283 uint32_t en_fstint : 1; 00284 uint32_t en_eovf : 1; 00285 uint32_t en_eint : 1; 00286 00287 uint32_t reserved : 8; 00288 00289 } bit; 00290 00291 } max30001_en_int2; 00292 00293 /** 00294 * @brief MNGR_INT (0x04) 00295 */ 00296 union max30001_mngr_int_reg { 00297 uint32_t all; 00298 00299 struct { 00300 uint32_t samp_it : 2; 00301 uint32_t clr_samp : 1; 00302 uint32_t clr_pedge : 1; 00303 uint32_t clr_rrint : 2; 00304 uint32_t clr_fast : 1; 00305 uint32_t reserved1 : 1; 00306 uint32_t reserved2 : 4; 00307 uint32_t reserved3 : 4; 00308 00309 uint32_t b_fit : 3; 00310 uint32_t e_fit : 5; 00311 00312 uint32_t reserved : 8; 00313 00314 } bit; 00315 00316 } max30001_mngr_int; 00317 00318 /** 00319 * @brief MNGR_DYN (0x05) 00320 */ 00321 union max30001_mngr_dyn_reg { 00322 uint32_t all; 00323 00324 struct { 00325 uint32_t bloff_lo_it : 8; 00326 uint32_t bloff_hi_it : 8; 00327 uint32_t fast_th : 6; 00328 uint32_t fast : 2; 00329 uint32_t reserved : 8; 00330 } bit; 00331 00332 } max30001_mngr_dyn; 00333 00334 // 0x08 00335 // uint32_t max30001_sw_rst; 00336 00337 // 0x09 00338 // uint32_t max30001_synch; 00339 00340 // 0x0A 00341 // uint32_t max30001_fifo_rst; 00342 00343 00344 /** 00345 * @brief INFO (0x0F) 00346 */ 00347 union max30001_info_reg { 00348 uint32_t all; 00349 struct { 00350 uint32_t serial : 12; 00351 uint32_t part_id : 2; 00352 uint32_t sample : 1; 00353 uint32_t reserved1 : 1; 00354 uint32_t rev_id : 4; 00355 uint32_t pattern : 4; 00356 uint32_t reserved : 8; 00357 } bit; 00358 00359 } max30001_info; 00360 00361 /** 00362 * @brief CNFG_GEN (0x10) 00363 */ 00364 union max30001_cnfg_gen_reg { 00365 uint32_t all; 00366 struct { 00367 uint32_t rbiasn : 1; 00368 uint32_t rbiasp : 1; 00369 uint32_t rbiasv : 2; 00370 uint32_t en_rbias : 2; 00371 uint32_t vth : 2; 00372 uint32_t imag : 3; 00373 uint32_t ipol : 1; 00374 uint32_t en_dcloff : 2; 00375 uint32_t en_bloff : 2; 00376 uint32_t reserved1 : 1; 00377 uint32_t en_pace : 1; 00378 uint32_t en_bioz : 1; 00379 uint32_t en_ecg : 1; 00380 uint32_t fmstr : 2; 00381 uint32_t en_ulp_lon : 2; 00382 uint32_t reserved : 8; 00383 } bit; 00384 00385 } max30001_cnfg_gen; 00386 00387 00388 /** 00389 * @brief CNFG_CAL (0x12) 00390 */ 00391 union max30001_cnfg_cal_reg { 00392 uint32_t all; 00393 struct { 00394 uint32_t thigh : 11; 00395 uint32_t fifty : 1; 00396 uint32_t fcal : 3; 00397 uint32_t reserved1 : 5; 00398 uint32_t vmag : 1; 00399 uint32_t vmode : 1; 00400 uint32_t en_vcal : 1; 00401 uint32_t reserved2 : 1; 00402 uint32_t reserved : 8; 00403 } bit; 00404 00405 } max30001_cnfg_cal; 00406 00407 /** 00408 * @brief CNFG_EMUX (0x14) 00409 */ 00410 union max30001_cnfg_emux_reg { 00411 uint32_t all; 00412 struct { 00413 uint32_t reserved1 : 16; 00414 uint32_t caln_sel : 2; 00415 uint32_t calp_sel : 2; 00416 uint32_t openn : 1; 00417 uint32_t openp : 1; 00418 uint32_t reserved2 : 1; 00419 uint32_t pol : 1; 00420 uint32_t reserved : 8; 00421 } bit; 00422 00423 } max30001_cnfg_emux; 00424 00425 00426 /** 00427 * @brief CNFG_ECG (0x15) 00428 */ 00429 union max30001_cnfg_ecg_reg { 00430 uint32_t all; 00431 struct { 00432 uint32_t reserved1 : 12; 00433 uint32_t dlpf : 2; 00434 uint32_t dhpf : 1; 00435 uint32_t reserved2 : 1; 00436 uint32_t gain : 2; 00437 uint32_t reserved3 : 4; 00438 uint32_t rate : 2; 00439 00440 uint32_t reserved : 8; 00441 } bit; 00442 00443 } max30001_cnfg_ecg; 00444 00445 /** 00446 * @brief CNFG_BMUX (0x17) 00447 */ 00448 union max30001_cnfg_bmux_reg { 00449 uint32_t all; 00450 struct { 00451 uint32_t fbist : 2; 00452 uint32_t reserved1 : 2; 00453 uint32_t rmod : 3; 00454 uint32_t reserved2 : 1; 00455 uint32_t rnom : 3; 00456 uint32_t en_bist : 1; 00457 uint32_t cg_mode : 2; 00458 uint32_t reserved3 : 2; 00459 uint32_t caln_sel : 2; 00460 uint32_t calp_sel : 2; 00461 uint32_t openn : 1; 00462 uint32_t openp : 1; 00463 uint32_t reserved4 : 2; 00464 uint32_t reserved : 8; 00465 } bit; 00466 00467 } max30001_cnfg_bmux; 00468 00469 /** 00470 * @brief CNFG_BIOZ (0x18) 00471 */ 00472 union max30001_bioz_reg { 00473 uint32_t all; 00474 struct { 00475 uint32_t phoff : 4; 00476 uint32_t cgmag : 3; 00477 uint32_t cgmon : 1; 00478 uint32_t fcgen : 4; 00479 uint32_t dlpf : 2; 00480 uint32_t dhpf : 2; 00481 uint32_t gain : 2; 00482 uint32_t inapow_mode : 1; 00483 uint32_t ext_rbias : 1; 00484 uint32_t ahpf : 3; 00485 uint32_t rate : 1; 00486 uint32_t reserved : 8; 00487 } bit; 00488 00489 } max30001_cnfg_bioz; 00490 00491 00492 /** 00493 * @brief CNFG_PACE (0x1A) 00494 */ 00495 union max30001_cnfg_pace_reg { 00496 uint32_t all; 00497 00498 struct { 00499 uint32_t dacn : 4; 00500 uint32_t dacp : 4; 00501 uint32_t reserved1 : 4; 00502 uint32_t aout : 2; 00503 uint32_t aout_lbw : 1; 00504 uint32_t reserved2 : 1; 00505 uint32_t gain : 3; 00506 uint32_t gn_diff_off : 1; 00507 uint32_t reserved3 : 3; 00508 uint32_t pol : 1; 00509 uint32_t reserved : 8; 00510 } bit; 00511 00512 } max30001_cnfg_pace; 00513 00514 /** 00515 * @brief CNFG_RTOR1 (0x1D) 00516 */ 00517 union max30001_cnfg_rtor1_reg { 00518 uint32_t all; 00519 struct { 00520 uint32_t reserved1 : 8; 00521 uint32_t ptsf : 4; 00522 uint32_t pavg : 2; 00523 uint32_t reserved2 : 1; 00524 uint32_t en_rtor : 1; 00525 uint32_t gain : 4; 00526 uint32_t wndw : 4; 00527 uint32_t reserved : 8; 00528 } bit; 00529 00530 } max30001_cnfg_rtor1; 00531 00532 /** 00533 * @brief CNFG_RTOR2 (0x1E) 00534 */ 00535 union max30001_cnfg_rtor2_reg { 00536 uint32_t all; 00537 struct { 00538 uint32_t reserved1 : 8; 00539 uint32_t rhsf : 3; 00540 uint32_t reserved2 : 1; 00541 uint32_t ravg : 2; 00542 uint32_t reserved3 : 2; 00543 uint32_t hoff : 6; 00544 uint32_t reserved4 : 2; 00545 uint32_t reserved : 8; 00546 } bit; 00547 00548 } max30001_cnfg_rtor2; 00549 00550 /*********************************************************************************/ 00551 00552 typedef enum { 00553 MAX30001_NO_INT = 0, // No interrupt 00554 MAX30001_INT_B = 1, // INTB selected for interrupt 00555 MAX30001_INT_2B = 2 // INT2B selected for interrupt 00556 } max30001_intrpt_Location_t; 00557 00558 typedef enum { 00559 MAX30001_INT_DISABLED = 0b00, 00560 MAX30001_INT_CMOS = 0b01, 00561 MAX30001_INT_ODN = 0b10, 00562 MAX30001_INT_ODNR = 0b11 00563 } max30001_intrpt_type_t; 00564 00565 typedef enum { // Input Polarity selection 00566 MAX30001_NON_INV = 0, // Non-Inverted 00567 MAX30001_INV = 1 // Inverted 00568 } max30001_emux_pol; 00569 00570 typedef enum { // OPENP and OPENN setting 00571 MAX30001_ECG_CON_AFE = 0, // ECGx is connected to AFE channel 00572 MAX30001_ECG_ISO_AFE = 1 // ECGx is isolated from AFE channel 00573 } max30001_emux_openx; 00574 00575 typedef enum { // EMUX_CALP_SEL & EMUX_CALN_SEL 00576 MAX30001_NO_CAL_SIG = 0b00, // No calibration signal is applied 00577 MAX30001_INPT_VMID = 0b01, // Input is connected to VMID 00578 MAX30001_INPT_VCALP = 0b10, // Input is connected to VCALP 00579 MAX30001_INPT_VCALN = 0b11 // Input is connected to VCALN 00580 } max30001_emux_calx_sel; 00581 00582 typedef enum { // EN_ECG, EN_BIOZ, EN_PACE 00583 MAX30001_CHANNEL_DISABLED = 0b0, // 00584 MAX30001_CHANNEL_ENABLED = 0b1 00585 } max30001_en_feature; 00586 00587 /*********************************************************************************/ 00588 // Data 00589 uint32_t max30001_ECG_FIFO_buffer[32]; // (303 for internal test) 00590 uint32_t max30001_BIOZ_FIFO_buffer[8]; // (303 for internal test) 00591 00592 uint32_t max30001_PACE[18]; // Pace Data 0-5 00593 00594 uint32_t max30001_RtoR_data; // This holds the RtoR data 00595 00596 uint32_t max30001_DCLeadOff; // This holds the LeadOff data, Last 4 bits give 00597 // the status, BIT3=LOFF_PH, BIT2=LOFF_PL, 00598 // BIT1=LOFF_NH, BIT0=LOFF_NL 00599 // 8th and 9th bits tell Lead off is due to ECG or BIOZ. 00600 // 0b01 = ECG Lead Off and 0b10 = BIOZ Lead off 00601 00602 uint32_t max30001_ACLeadOff; // This gives the state of the BIOZ AC Lead Off 00603 // state. BIT 1 = BOVER, BIT 0 = BUNDR 00604 00605 uint32_t max30001_bcgmon; // This holds the BCGMON data, BIT 1 = BCGMP, BIT0 = 00606 // BCGMN 00607 00608 uint32_t max30001_LeadOn; // This holds the LeadOn data, BIT1 = BIOZ Lead ON, 00609 // BIT0 = ECG Lead ON, BIT8= Lead On Status Bit 00610 00611 uint32_t max30001_timeout; // If the PLL does not respond, timeout and get out. 00612 00613 typedef struct { // Creating a structure for BLE data 00614 int16_t R2R; 00615 int16_t fmstr; 00616 } max30001_t; 00617 00618 max30001_t hspValMax30001; // R2R, FMSTR 00619 00620 //jjj 14MAR17 00621 //added DigitalOut so we can use any pin for cs 00622 //jjj 00623 MAX30001(SPI *spi, DigitalOut *cs); 00624 00625 00626 /** 00627 * @brief Constructor that accepts pin names for the SPI interface 00628 * @param mosi master out slave in pin name 00629 * @param miso master in slave out pin name 00630 * @param sclk serial clock pin name 00631 * @param cs chip select pin name 00632 */ 00633 MAX30001(PinName mosi, PinName miso, PinName sclk, PinName cs); 00634 00635 /** 00636 * MAX30001 destructor 00637 */ 00638 ~MAX30001(void); 00639 00640 /** 00641 * @brief This function sets up the Resistive Bias mode and also selects the master clock frequency. 00642 * @brief Uses Register: CNFG_GEN-0x10 00643 * @param En_rbias: Enable and Select Resitive Lead Bias Mode 00644 * @param Rbiasv: Resistive Bias Mode Value Selection 00645 * @param Rbiasp: Enables Resistive Bias on Positive Input 00646 * @param Rbiasn: Enables Resistive Bias on Negative Input 00647 * @param Fmstr: Selects Master Clock Frequency 00648 * @returns 0-if no error. A non-zero value indicates an error. 00649 * 00650 */ 00651 int max30001_Rbias_FMSTR_Init(uint8_t En_rbias, uint8_t Rbiasv, 00652 uint8_t Rbiasp, uint8_t Rbiasn, uint8_t Fmstr); 00653 00654 /** 00655 * @brief This function uses sets up the calibration signal internally. If it is desired to use the internal signal, then 00656 * @brief this function must be called and the registers set, prior to setting the CALP_SEL and CALN_SEL in the ECG_InitStart 00657 * @brief and BIOZ_InitStart functions. 00658 * @brief Uses Register: CNFG_CAL-0x12 00659 * @param En_Vcal: Calibration Source (VCALP and VCALN) Enable 00660 * @param Vmode: Calibration Source Mode Selection 00661 * @param Vmag: Calibration Source Magnitude Selection (VMAG) 00662 * @param Fcal: Calibration Source Frequency Selection (FCAL) 00663 * @param Thigh: Calibration Source Time High Selection 00664 * @param Fifty: Calibration Source Duty Cycle Mode Selection 00665 * @returns 0-if no error. A non-zero value indicates an error. 00666 * 00667 */ 00668 int max30001_CAL_InitStart(uint8_t En_Vcal, uint8_t Vmode, uint8_t Vmag, 00669 uint8_t Fcal, uint16_t Thigh, uint8_t Fifty); 00670 00671 /** 00672 * @brief This function disables the VCAL signal 00673 * @returns 0-if no error. A non-zero value indicates an error. 00674 */ 00675 int max30001_CAL_Stop(void); 00676 00677 /** 00678 * @brief This function handles the assignment of the two interrupt pins (INTB & INT2B) with various 00679 * @brief functions/behaviors of the MAX30001. Also, each pin can be configured for different drive capability. 00680 * @brief Uses Registers: EN_INT-0x02 and EN_INT2-0x03. 00681 * @param max30001_intrpt_Locatio_t <argument>: All the arguments with the aforementioned enumeration essentially 00682 * can be configured to generate an interrupt on either INTB or INT2B or NONE. 00683 * @param max30001_intrpt_type_t intb_Type: INTB Port Type (EN_INT Selections). 00684 * @param max30001_intrpt_type _t int2b_Type: INT2B Port Type (EN_INT2 Selections) 00685 * @returns 0-if no error. A non-zero value indicates an error. 00686 * 00687 */ 00688 int max30001_INT_assignment(max30001_intrpt_Location_t en_enint_loc, max30001_intrpt_Location_t en_eovf_loc, max30001_intrpt_Location_t en_fstint_loc, 00689 max30001_intrpt_Location_t en_dcloffint_loc, max30001_intrpt_Location_t en_bint_loc, max30001_intrpt_Location_t en_bovf_loc, 00690 max30001_intrpt_Location_t en_bover_loc, max30001_intrpt_Location_t en_bundr_loc, max30001_intrpt_Location_t en_bcgmon_loc, 00691 max30001_intrpt_Location_t en_pint_loc, max30001_intrpt_Location_t en_povf_loc, max30001_intrpt_Location_t en_pedge_loc, 00692 max30001_intrpt_Location_t en_lonint_loc, max30001_intrpt_Location_t en_rrint_loc, max30001_intrpt_Location_t en_samp_loc, 00693 max30001_intrpt_type_t intb_Type, max30001_intrpt_type_t int2b_Type); 00694 00695 00696 00697 /** 00698 * @brief For MAX30001/3 ONLY 00699 * @brief This function sets up the MAX30001 for the ECG measurements. 00700 * @brief Registers used: CNFG_EMUX, CNFG_GEN, MNGR_INT, CNFG_ECG. 00701 * @param En_ecg: ECG Channel Enable <CNFG_GEN register bits> 00702 * @param Openp: Open the ECGN Input Switch (most often used for testing and calibration studies) <CNFG_EMUX register bits> 00703 * @param Openn: Open the ECGN Input Switch (most often used for testing and calibration studies) <CNFG_EMUX register bits> 00704 * @param Calp_sel: ECGP Calibration Selection <CNFG_EMUX register bits> 00705 * @param Caln_sel: ECGN Calibration Selection <CNFG_EMUX register bits> 00706 * @param E_fit: ECG FIFO Interrupt Threshold (issues EINT based on number of unread FIFO records) <CNFG_GEN register bits> 00707 * @param Clr_rrint: RTOR R Detect Interrupt (RRINT) Clear Behavior <CNFG_GEN register bits> 00708 * @param Rate: ECG Data Rate 00709 * @param Gain: ECG Channel Gain Setting 00710 * @param Dhpf: ECG Channel Digital High Pass Filter Cutoff Frequency 00711 * @param Dlpf: ECG Channel Digital Low Pass Filter Cutoff Frequency 00712 * @returns 0-if no error. A non-zero value indicates an error. 00713 * 00714 */ 00715 int max30001_ECG_InitStart(uint8_t En_ecg, uint8_t Openp, uint8_t Openn, 00716 uint8_t Pol, uint8_t Calp_sel, uint8_t Caln_sel, 00717 uint8_t E_fit, uint8_t Rate, uint8_t Gain, 00718 uint8_t Dhpf, uint8_t Dlpf); 00719 00720 /** 00721 * @brief For MAX30001/3 ONLY 00722 * @brief This function enables the Fast mode feature of the ECG. 00723 * @brief Registers used: MNGR_INT-0x04, MNGR_DYN-0x05 00724 * @param Clr_Fast: FAST MODE Interrupt Clear Behavior <MNGR_INT Register> 00725 * @param Fast: ECG Channel Fast Recovery Mode Selection (ECG High Pass Filter Bypass) <MNGR_DYN Register> 00726 * @param Fast_Th: Automatic Fast Recovery Threshold 00727 * @returns 0-if no error. A non-zero value indicates an error. 00728 * 00729 */ 00730 int max30001_ECGFast_Init(uint8_t Clr_Fast, uint8_t Fast, uint8_t Fast_Th); 00731 00732 /** 00733 * @brief For MAX30001/3 ONLY 00734 * @brief This function disables the ECG. 00735 * @brief Uses Register CNFG_GEN-0x10. 00736 * @returns 0-if no error. A non-zero value indicates an error. 00737 * 00738 */ 00739 int max30001_Stop_ECG(void); 00740 00741 /** 00742 * @brief For MAX30001 ONLY 00743 * @brief This function sets up the MAX30001 for pace signal detection. 00744 * @brief If both PACE and BIOZ are turned ON, then make sure Fcgen is set for 80K or 40K in the 00745 * @brief max30001_BIOZ_InitStart() function. However, if Only PACE is on but BIOZ off, then Fcgen can be set 00746 * @brief for 80K only, in the max30001_BIOZ_InitStart() function 00747 * @brief Registers used: MNGR_INT-0x04, CNFG_GEN-0x37, CNFG_PACE-0x1A. 00748 * @param En_pace : PACE Channel Enable <CNFG_GEN Register> 00749 * @param Clr_pedge : PACE Edge Detect Interrupt (PEDGE) Clear Behavior <MNGR_INT Register> 00750 * @param Pol: PACE Input Polarity Selection <CNFG_PACE Register> 00751 * @param Gn_diff_off: PACE Differentiator Mode <CNFG_PACE Register> 00752 * @param Gain: PACE Channel Gain Selection <CNFG_PACE Register> 00753 * @param Aout_lbw: PACE Analog Output Buffer Bandwidth Mode <CNFG_PACE Register> 00754 * @param Aout: PACE Single Ended Analog Output Buffer Signal Monitoring Selection <CNFG_PACE Register> 00755 * @param Dacp (4bits): PACE Detector Positive Comparator Threshold <CNFG_PACE Register> 00756 * @param Dacn(4bits): PACE Detector Negative Comparator Threshold <CNFG_PACE Register> 00757 * @returns 0-if no error. A non-zero value indicates an error <CNFG_PACE Register> 00758 * 00759 */ 00760 int max30001_PACE_InitStart(uint8_t En_pace, uint8_t Clr_pedge, uint8_t Pol, 00761 uint8_t Gn_diff_off, uint8_t Gain, 00762 uint8_t Aout_lbw, uint8_t Aout, uint8_t Dacp, 00763 uint8_t Dacn); 00764 00765 /** 00766 *@brief For MAX30001 ONLY 00767 *@param This function disables the PACE. Uses Register CNFG_GEN-0x10. 00768 *@returns 0-if no error. A non-zero value indicates an error. 00769 * 00770 */ 00771 int max30001_Stop_PACE(void); 00772 00773 /** 00774 * @brief For MAX30001/2 ONLY 00775 * @brief This function sets up the MAX30001 for BIOZ measurement. 00776 * @brief Registers used: MNGR_INT-0x04, CNFG_GEN-0X10, CNFG_BMUX-0x17,CNFG_BIOZ-0x18. 00777 * @param En_bioz: BIOZ Channel Enable <CNFG_GEN Register> 00778 * @param Openp: Open the BIP Input Switch <CNFG_BMUX Register> 00779 * @param Openn: Open the BIN Input Switch <CNFG_BMUX Register> 00780 * @param Calp_sel: BIP Calibration Selection <CNFG_BMUX Register> 00781 * @param Caln_sel: BIN Calibration Selection <CNFG_BMUX Register> 00782 * @param CG_mode: BIOZ Current Generator Mode Selection <CNFG_BMUX Register> 00783 * @param B_fit: BIOZ FIFO Interrupt Threshold (issues BINT based on number of unread FIFO records) <MNGR_INT Register> 00784 * @param Rate: BIOZ Data Rate <CNFG_BIOZ Register> 00785 * @param Ahpf: BIOZ/PACE Channel Analog High Pass Filter Cutoff Frequency and Bypass <CNFG_BIOZ Register> 00786 * @param Ext_rbias: External Resistor Bias Enable <CNFG_BIOZ Register> 00787 * @param Gain: BIOZ Channel Gain Setting <CNFG_BIOZ Register> 00788 * @param Dhpf: BIOZ Channel Digital High Pass Filter Cutoff Frequency <CNFG_BIOZ Register> 00789 * @param Dlpf: BIOZ Channel Digital Low Pass Filter Cutoff Frequency <CNFG_BIOZ Register> 00790 * @param Fcgen: BIOZ Current Generator Modulation Frequency <CNFG_BIOZ Register> 00791 * @param Cgmon: BIOZ Current Generator Monitor <CNFG_BIOZ Register> 00792 * @param Cgmag: BIOZ Current Generator Magnitude <CNFG_BIOZ Register> 00793 * @param Phoff: BIOZ Current Generator Modulation Phase Offset <CNFG_BIOZ Register> 00794 * @param Inapow_mode: BIOZ Channel Instrumentation Amplifier (INA) Power Mode <CNFG_BIOZ Register> 00795 * @returns 0-if no error. A non-zero value indicates an error. 00796 * 00797 */ 00798 int max30001_BIOZ_InitStart(uint8_t En_bioz, uint8_t Openp, uint8_t Openn, 00799 uint8_t Calp_sel, uint8_t Caln_sel, 00800 uint8_t CG_mode, 00801 /* uint8_t En_bioz,*/ uint8_t B_fit, uint8_t Rate, 00802 uint8_t Ahpf, uint8_t Ext_rbias, uint8_t Gain, 00803 uint8_t Dhpf, uint8_t Dlpf, uint8_t Fcgen, 00804 uint8_t Cgmon, uint8_t Cgmag, uint8_t Phoff, uint8_t Inapow_mode); 00805 00806 /** 00807 * @brief For MAX30001/2 ONLY 00808 * @brief This function disables the BIOZ. Uses Register CNFG_GEN-0x10. 00809 * @returns 0-if no error. A non-zero value indicates an error. 00810 * @returns 0-if no error. A non-zero value indicates an error. 00811 * 00812 */ 00813 int max30001_Stop_BIOZ(void); 00814 00815 /** 00816 * @brief For MAX30001/2 ONLY 00817 * @brief BIOZ modulated Resistance Built-in-Self-Test, Registers used: CNFG_BMUX-0x17 00818 * @param En_bist: Enable Modulated Resistance Built-in-Self-test <CNFG_BMUX Register> 00819 * @param Rnom: BIOZ RMOD BIST Nominal Resistance Selection <CNFG_BMUX Register> 00820 * @param Rmod: BIOZ RMOD BIST Modulated Resistance Selection <CNFG_BMUX Register> 00821 * @param Fbist: BIOZ RMOD BIST Frequency Selection <CNFG_BMUX Register> 00822 * @returns 0-if no error. A non-zero value indicates an error. 00823 * 00824 */ 00825 int max30001_BIOZ_InitBist(uint8_t En_bist, uint8_t Rnom, uint8_t Rmod, 00826 uint8_t Fbist); 00827 00828 /** 00829 * @brief For MAX30001/3/4 ONLY 00830 * @brief Sets up the device for RtoR measurement 00831 * @param EN_rtor: ECG RTOR Detection Enable <RTOR1 Register> 00832 * @param Wndw: R to R Window Averaging (Window Width = RTOR_WNDW[3:0]*8mS) <RTOR1 Register> 00833 * @param Gain: R to R Gain (where Gain = 2^RTOR_GAIN[3:0], plus an auto-scale option) <RTOR1 Register> 00834 * @param Pavg: R to R Peak Averaging Weight Factor <RTOR1 Register> 00835 * @param Ptsf: R to R Peak Threshold Scaling Factor <RTOR1 Register> 00836 * @param Hoff: R to R minimum Hold Off <RTOR2 Register> 00837 * @param Ravg: R to R Interval Averaging Weight Factor <RTOR2 Register> 00838 * @param Rhsf: R to R Interval Hold Off Scaling Factor <RTOR2 Register> 00839 * @param Clr_rrint: RTOR Detect Interrupt Clear behaviour <MNGR_INT Register> 00840 * @returns 0-if no error. A non-zero value indicates an error. 00841 * 00842 */ 00843 int max30001_RtoR_InitStart(uint8_t En_rtor, uint8_t Wndw, uint8_t Gain, 00844 uint8_t Pavg, uint8_t Ptsf, uint8_t Hoff, 00845 uint8_t Ravg, uint8_t Rhsf, uint8_t Clr_rrint); 00846 00847 /** 00848 * @brief For MAX30001/3/4 ONLY 00849 * @brief This function disables the RtoR. Uses Register CNFG_RTOR1-0x1D 00850 * @returns 0-if no error. A non-zero value indicates an error. 00851 * 00852 */ 00853 int max30001_Stop_RtoR(void); 00854 00855 /** 00856 * @brief This is a function that waits for the PLL to lock; once a lock is achieved it exits out. (For convenience only) 00857 * @returns 0-if no error. A non-zero value indicates an error. 00858 * 00859 */ 00860 int max30001_PLL_lock(void); 00861 00862 /** 00863 * @brief This function causes the MAX30001 to reset. Uses Register SW_RST-0x08 00864 * @return 0-if no error. A non-zero value indicates an error. 00865 * 00866 */ 00867 int max30001_sw_rst(void); 00868 00869 /** 00870 * @brief This function provides a SYNCH operation. Uses Register SYCNH-0x09. Please refer to the data sheet for 00871 * @brief the details on how to use this. 00872 * @returns 0-if no error. A non-zero value indicates an error. 00873 * 00874 */ 00875 int max30001_synch(void); 00876 00877 /** 00878 * @brief This function performs a FIFO Reset. Uses Register FIFO_RST-0x0A. Please refer to the data sheet 00879 * @brief for the details on how to use this. 00880 * @returns 0-if no error. A non-zero value indicates an error. 00881 */ 00882 int max300001_fifo_rst(void); 00883 00884 /** 00885 * 00886 * @brief This is a callback function which collects all the data from the ECG, BIOZ, PACE and RtoR. It also handles 00887 * @brief Lead On/Off. This function is passed through the argument of max30001_COMMinit(). 00888 * @returns 0-if no error. A non-zero value indicates an error. 00889 * 00890 */ 00891 int max30001_int_handler(void); 00892 00893 /** 00894 * @brief This is function called from the max30001_int_handler() function and processes all the ECG, BIOZ, PACE 00895 * @brief and the RtoR data and sticks them in appropriate arrays and variables each unsigned 32 bits. 00896 * @param ECG data will be in the array (input): max30001_ECG_FIFO_buffer[] 00897 * @param Pace data will be in the array (input): max30001_PACE[] 00898 * @param RtoRdata will be in the variable (input): max30001_RtoR_data 00899 * @param BIOZ data will be in the array (input): max30001_BIOZ_FIFO_buffer[] 00900 * @param global max30001_ECG_FIFO_buffer[] 00901 * @param global max30001_PACE[] 00902 * @param global max30001_BIOZ_FIFO_buffer[] 00903 * @param global max30001_RtoR_data 00904 * @param global max30001_DCLeadOff 00905 * @param global max30001_ACLeadOff 00906 * @param global max30001_LeadON 00907 * @returns 0-if no error. A non-zero value indicates an error. 00908 * 00909 */ 00910 int max30001_FIFO_LeadONOff_Read(void); 00911 00912 /** 00913 * @brief This function allows writing to a register. 00914 * @param addr: Address of the register to write to 00915 * @param data: 24-bit data read from the register. 00916 * @returns 0-if no error. A non-zero value indicates an error. 00917 * 00918 */ 00919 int max30001_reg_write(MAX30001_REG_map_t addr, uint32_t data); 00920 00921 /** 00922 * @brief This function allows reading from a register 00923 * @param addr: Address of the register to read from. 00924 * @param *return_data: pointer to the value read from the register. 00925 * @returns 0-if no error. A non-zero value indicates an error. 00926 * 00927 */ 00928 int max30001_reg_read(MAX30001_REG_map_t addr, uint32_t *return_data); 00929 00930 /** 00931 * @brief This function enables the DC Lead Off detection. Either ECG or BIOZ can be detected, one at a time. 00932 * @brief Registers Used: CNFG_GEN-0x10 00933 * @param En_dcloff: BIOZ Digital Lead Off Detection Enable 00934 * @param Ipol: DC Lead Off Current Polarity (if current sources are enabled/connected) 00935 * @param Imag: DC Lead off current Magnitude Selection 00936 * @param Vth: DC Lead Off Voltage Threshold Selection 00937 * @returns 0-if no error. A non-zero value indicates an error. 00938 * 00939 */ 00940 int max30001_Enable_DcLeadOFF_Init(int8_t En_dcloff, int8_t Ipol, int8_t Imag, 00941 int8_t Vth); 00942 00943 /** 00944 * @brief This function disables the DC Lead OFF feature, whichever is active. 00945 * @returns 0-if no error. A non-zero value indicates an error. 00946 * 00947 */ 00948 int max30001_Disable_DcLeadOFF(void); 00949 00950 /** 00951 * @brief This function sets up the BIOZ for AC Lead Off test. 00952 * @brief Registers Used: CNFG_GEN-0x10, MNGR_DYN-0x05 00953 * @param En_bloff: BIOZ Digital Lead Off Detection Enable <CNFG_GEN register> 00954 * @param Bloff_hi_it: DC Lead Off Current Polarity (if current sources are enabled/connected) <MNGR_DYN register> 00955 * @param Bloff_lo_it: DC Lead off current Magnitude Selection <MNGR_DYN register> 00956 * @returns 0-if no error. A non-zero value indicates an error. 00957 * 00958 */ 00959 int max30001_BIOZ_Enable_ACLeadOFF_Init(uint8_t En_bloff, uint8_t Bloff_hi_it, 00960 uint8_t Bloff_lo_it); 00961 00962 /** 00963 * @brief This function Turns of the BIOZ AC Lead OFF feature 00964 * @brief Registers Used: CNFG_GEN-0x10 00965 * @returns 0-if no error. A non-zero value indicates an error. 00966 * 00967 */ 00968 int max30001_BIOZ_Disable_ACleadOFF(void); 00969 00970 /** 00971 * @brief This function enables the Current Gnerator Monitor 00972 * @brief Registers Used: CNFG_BIOZ-0x18 00973 * @returns 0-if no error. A non-zero value indicates an error. 00974 * 00975 */ 00976 int max30001_BIOZ_Enable_BCGMON(void); 00977 00978 /** 00979 * 00980 * @brief This function enables the Lead ON detection. Either ECG or BIOZ can be detected, one at a time. 00981 * @brief Also, the en_bioz, en_ecg, en_pace setting is saved so that when this feature is disabled through the 00982 * @brief max30001_Disable_LeadON() function (or otherwise) the enable/disable state of those features can be retrieved. 00983 * @param Channel: ECG or BIOZ detection 00984 * @returns 0-if everything is good. A non-zero value indicates an error. 00985 * 00986 */ 00987 int max30001_Enable_LeadON(int8_t Channel); 00988 00989 /** 00990 * @brief This function turns off the Lead ON feature, whichever one is active. Also, retrieves the en_bioz, 00991 * @brief en_ecg, en_pace and sets it back to as it was. 00992 * @param 0-if everything is good. A non-zero value indicates an error. 00993 * 00994 */ 00995 int max30001_Disable_LeadON(void); 00996 00997 /** 00998 * 00999 * @brief This function is toggled every 2 seconds to switch between ECG Lead ON and BIOZ Lead ON detect 01000 * @brief Adjust LEADOFF_SERVICE_TIME to determine the duration between the toggles. 01001 * @param CurrentTime - This gets fed the time by RTC_GetValue function 01002 * 01003 */ 01004 void max30001_ServiceLeadON(uint32_t currentTime); 01005 01006 /** 01007 * 01008 * @brief This function is toggled every 2 seconds to switch between ECG DC Lead Off and BIOZ DC Lead Off 01009 * @brief Adjust LEADOFF_SERVICE_TIME to determine the duration between the toggles. 01010 * @param CurrentTime - This gets fed the time by RTC_GetValue function 01011 * 01012 */ 01013 void max30001_ServiceLeadoff(uint32_t currentTime); 01014 01015 /** 01016 * 01017 * @brief This function sets current RtoR values and fmstr values in a pointer structure 01018 * @param hspValMax30001 - Pointer to a structure where to store the values 01019 * 01020 */ 01021 void max30001_ReadHeartrateData(max30001_t *_hspValMax30001); 01022 01023 /** 01024 * @brief type definition for data interrupt 01025 */ 01026 typedef void (*PtrFunction)(uint32_t id, uint32_t *buffer, uint32_t length); 01027 01028 /** 01029 * @brief Used to connect a callback for when interrupt data is available 01030 */ 01031 void onDataAvailable(PtrFunction _onDataAvailable); 01032 01033 static MAX30001 *instance; 01034 01035 private: 01036 void dataAvailable(uint32_t id, uint32_t *buffer, uint32_t length); 01037 /// interrupt handler for async spi events 01038 static void spiHandler(int events); 01039 /// wrapper method to transmit and recieve SPI data 01040 int SPI_Transmit(const uint8_t *tx_buf, uint32_t tx_size, uint8_t *rx_buf, 01041 uint32_t rx_size); 01042 uint32_t readPace(int group, uint8_t* result); 01043 01044 //jjj 14MAR17 01045 //pointer to DigitalOut for cs 01046 DigitalOut * m_cs; 01047 //jjj 01048 /// pointer to mbed SPI object 01049 SPI *m_spi; 01050 /// is this object the owner of the spi object 01051 bool spi_owner; 01052 /// buffer to use for async transfers 01053 uint8_t buffer[ASYNC_SPI_BUFFER_SIZE]; 01054 /// function pointer to the async callback 01055 event_callback_t functionpointer; 01056 /// callback function when interrupt data is available 01057 PtrFunction onDataAvailableCallback; 01058 01059 }; // End of MAX30001 Class 01060 01061 /** 01062 * @brief Preventive measure used to dismiss interrupts that fire too early during 01063 * @brief initialization on INTB line 01064 * 01065 */ 01066 void MAX30001Mid_IntB_Handler(void); 01067 01068 /** 01069 * @brief Preventive measure used to dismiss interrupts that fire too early during 01070 * @brief initialization on INT2B line 01071 * 01072 */ 01073 void MAX30001Mid_Int2B_Handler(void); 01074 01075 /** 01076 * @brief Allows Interrupts to be accepted as valid. 01077 * @param state: 1-Allow interrupts, Any-Don't allow interrupts. 01078 * 01079 */ 01080 void MAX30001_AllowInterrupts(int state); 01081 01082 #endif /* MAX30001_H_ */ 01083
Generated on Tue Jul 12 2022 16:59:43 by
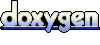