
Freq calculations using counters and interrupt
Dependencies: FastPWM PwmIn USBDevice mbed
main.cpp
00001 #include "FastPWM.h" 00002 #include "mbed.h" 00003 #include "PwmIn.h" 00004 #include "USBSerial.h" 00005 00006 // using fastpwm signal as a clock signal to be used to trigger the counter whilst the signal I want to measure is high. 00007 // to get this to work properly I believe I will need to use nested interrupts along with setting interupt priorities. This is so that 00008 // when the signal I want to read (led) causes an interrupt on a rising edge whilst this value is high I want the clock trigger to interrupt a seperate pin 00009 // which will continuoulsy count until the (led) signal causes another interrupt by going low. 00010 00011 // clkin is currently set as a DigitalIn but this likely needs to become an interrupt. 00012 00013 USBSerial serial; 00014 00015 FastPWM clkoutput(p20); 00016 DigitalIn clkin(p8); 00017 InterruptIn led(p7); 00018 Ticker display; 00019 00020 int count; 00021 int count_2; 00022 int t_rise; 00023 int t_fall; 00024 int t_period = 0; 00025 00026 00027 void clock_pulse() { 00028 00029 clkoutput.period(8e-7); //around 1.2 MHz signal 00030 clkoutput.write(0.5f); // 50% duty cycle 00031 } 00032 00033 00034 void rise() { // rising edge interrupt routine 00035 00036 while (led && clkin) { // whilst the signal I want to measure is high (led) and the clk signal (clkin) is high increment the counter 00037 // if (clkin) { 00038 count++; 00039 } 00040 // if (led && clkoutput) { 00041 00042 // count++; 00043 // while (led) { 00044 // if (clkin) { 00045 // count++; 00046 // } 00047 t_fall = count_2; 00048 // count_2 = 0; 00049 } 00050 // } 00051 00052 void fall() { // falling edge interrupt routine 00053 00054 while (!led && clkin) { // whilst the signal I want to measure is low and the clk signal is high increment counter 2 00055 count_2++; 00056 } 00057 t_rise = count; 00058 // count = 0; 00059 00060 } 00061 00062 // } 00063 00064 /*void period() { 00065 t_period = count; 00066 count = 0; 00067 } 00068 */ 00069 00070 void disp() { 00071 00072 serial.printf("count is %d \n\r" , t_rise); 00073 serial.printf("count_2 is %d \n\r" , t_fall); 00074 } 00075 00076 00077 int main() 00078 { 00079 clock_pulse(); 00080 00081 led.rise(&rise); // set rising edge interrupt and call rise function 00082 led.fall(&fall); // set falling edge interrupt and call fall function 00083 //count = 0; 00084 // } 00085 00086 display.attach(&disp, 2); // ticker function to display results every 2 seconds 00087 00088 while (1) { 00089 00090 // while (led) { 00091 // if (clkin) { 00092 // count++; 00093 // } 00094 // count = 0; 00095 //} 00096 00097 // if (led == 1) && (clkoutput == 1) { 00098 00099 // count++; 00100 00101 /* 00102 00103 while (led) { 00104 00105 if (clkin) { 00106 00107 count++; 00108 } 00109 00110 */ 00111 00112 00113 } 00114 00115 //serial.printf("Pulsewidth is %.2f \n\r" , pa.pulsewidth()); 00116 00117 00118 00119 00120 00121 } 00122
Generated on Tue Jul 19 2022 05:47:56 by
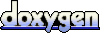