DE: Ein sehr, sehr einfacher Webserver mithilfe eines ESP8266 auf dem die AT-Firmware läuft . Für Bulme Bertl. - EN: A very, very, basic web server that works using an ESP8266 running the AT-Firmware.
Dependents: BULME_BERTL17_WebServer_ESPAT Bravo Team
ESPAT.cpp
00001 #include "ESPAT.h" 00002 #include "mbed.h" 00003 #include "string" 00004 00005 /* 00006 Library for using an ESP8266 (e.g. ESP-01 board) with its AT command firmware as webserver 00007 Version: 1.0.0 00008 (C)2019 Elias Nestl 00009 */ 00010 00011 ESPAT::ESPAT(PinName tx, PinName rx, int baud) : espSerial(tx, rx, baud) { 00012 } 00013 00014 //Serial pc(USBTX, USBRX, 115200); 00015 00016 void ESPAT::readStrUntil(string * str, char until) { 00017 while (true) { 00018 char c = espSerial.getc(); 00019 if (c != until) { 00020 *str += c; 00021 } else { 00022 break; 00023 } 00024 } 00025 } 00026 00027 void ESPAT::waitFor(char * text) { 00028 //string s; 00029 for (int i = 0; i < strlen(text); i++) { 00030 char c = espSerial.getc(); 00031 //s += c; 00032 if (c != text[i]) { 00033 i = 0; 00034 } 00035 } 00036 //pc.printf("%s", s.c_str()); 00037 } 00038 00039 void ESPAT::sendCommand(char * cmd, bool waitOk) { 00040 //pc.printf("%s\r\n", cmd); 00041 espSerial.printf("%s\r\n", cmd); 00042 if (waitOk) waitFor("OK"); 00043 } 00044 00045 void ESPAT::resetEsp() { 00046 sendCommand("AT+RST"); 00047 waitFor("ready"); 00048 } 00049 00050 void ESPAT::initWifiStation(string ssid, string pwd) { 00051 sendCommand("AT+CWMODE_CUR=1"); 00052 string wifiCmd = "AT+CWJAP_CUR=\"" + ssid + "\",\"" + pwd + "\""; 00053 sendCommand((char*) wifiCmd.c_str()); 00054 } 00055 00056 void ESPAT::initWifiAP(string ssid, string pwd, string channel, string encryption) { 00057 sendCommand("AT+CWMODE_CUR=2"); 00058 string wifiCmd = "AT+CWSAP_CUR=\"" + ssid + "\",\"" + pwd + "\"," + channel + "," + encryption; 00059 sendCommand((char*) wifiCmd.c_str()); 00060 } 00061 00062 void ESPAT::initServer(void (*requestHandler)(int, string)) { 00063 sendCommand("AT+CIPMUX=1"); 00064 sendCommand("AT+CIPSERVER=1,80"); 00065 while (true) { 00066 // Input looks like this: +IPD,0,372:GET / HTTP/1.1 00067 waitFor("+IPD,"); 00068 // Parse linkId 00069 int linkId = 0; 00070 char c; 00071 while (true) { 00072 c = espSerial.getc(); 00073 if (c == ',') break; 00074 linkId = linkId * 10 + (c - '0'); 00075 } 00076 // Parse path 00077 waitFor(":GET "); 00078 string path; 00079 readStrUntil(&path, ' '); 00080 // Send request to handler 00081 requestHandler(linkId, path); 00082 } 00083 } 00084 00085 void ESPAT::tcpReply(int linkId, string data) { 00086 espSerial.printf("AT+CIPSEND=%d,%d\r\n", linkId, data.length()); 00087 wait(0.1); // Can't use waitFor here as it isn't fast enough 00088 for (int i = 0; i < data.length(); i++) { 00089 // Artificially slowing down this to prevent errors 00090 espSerial.printf("%c", data[i]); 00091 } 00092 waitFor("SEND OK"); 00093 espSerial.printf("AT+CIPCLOSE=%d\r\n", linkId); 00094 waitFor("OK"); 00095 } 00096 00097 void ESPAT::httpReply(int linkId, string code, string payload) { 00098 string data = "HTTP/1.1 " + code + "\r\nContent-Type: text/html\r\n\r\n" + payload; 00099 tcpReply(linkId, data); 00100 }
Generated on Tue Jul 12 2022 20:43:28 by
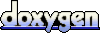