
My GPIO tests
Fork of Nucleo_toggle_ios by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 00004 #include <string.h> 00005 00006 #define BAUD_RATE 115200 00007 00008 00009 00010 DigitalOut PA9IOs(PA_9); 00011 DigitalOut PA10IOs(PA_10); 00012 DigitalOut PA11IOs(PA_11); 00013 DigitalOut PA12IOs(PA_12); 00014 00015 DigitalOut PB4IOs(PB_4); 00016 DigitalOut PB5IOs(PB_5); 00017 DigitalOut PB6IOs(PB_6); 00018 DigitalOut PB7IOs(PB_7); 00019 00020 DigitalOut PB12IOs(PB_12); 00021 DigitalOut PB13IOs(PB_13); 00022 DigitalOut PB14IOs(PB_14); 00023 DigitalOut PB15IOs(PB_15); 00024 00025 DigitalOut PC6IOs(PC_6); 00026 DigitalOut PC7IOs(PC_7); 00027 DigitalOut PC8IOs(PC_8); 00028 DigitalOut PC9IOs(PC_9); 00029 00030 Serial s(USBTX, USBRX); //default for nrf51 is p0.09 p0.11 00031 00032 00033 // Command string 00034 char action[256]; 00035 char command[12]; 00036 unsigned short val; 00037 00038 #define N_ITERATIONS 1120 00039 void gpio_action(){ 00040 // for(i = 0; i <= 1119; i++) 00041 // { 00042 PA9IOs = 0; // SET PA9 00043 PB4IOs = 0; // SET PB4 00044 PC6IOs = 0; // SET PC6 00045 PB12IOs = 0; // SET PB12 00046 wait(10); // wait 440 seconds 00047 PA10IOs = 0; // SET PA10 00048 PB5IOs = 0; // SET PB5 00049 PC7IOs = 0; // SET PC7 00050 PB13IOs = 0; // SET PB13 00051 wait(2.5); // wait 2.5 seconds 00052 PA10IOs = 1; // RESET PA10 00053 PB5IOs = 1; // RESET PB5 00054 PC7IOs = 1; // RESET PC7 00055 PB13IOs = 1; // RESET PB13 00056 //} 00057 } 00058 00059 int i; 00060 int run = 1; 00061 00062 int main() { 00063 00064 s.baud(BAUD_RATE); 00065 00066 //Reset EN_1_1 and EN_1_2 00067 PA9IOs = 1; // RESET PA9 00068 PA10IOs = 1; // RESET PA10 00069 //Reset EN_2_1 and EN_2_2 00070 PB4IOs = 1; // RESET PB4 00071 PB5IOs = 1; // RESET PB5 00072 //Reset EN_3_1 and EN_3_2 00073 PB12IOs = 1; // RESET PB12 00074 PB13IOs = 1; // RESET PB13 00075 //Reset EN_4_1 and EN_4_2 00076 PC6IOs = 1; // RESET PC6 00077 PC7IOs = 1; // RESET PC7 00078 run: 00079 s.printf("Action:\r\n"); 00080 int count; 00081 s.scanf( "%s %d" , action, &count); 00082 if (strstr(action, "START") != NULL){ 00083 i = 0; 00084 s.printf("START command\r\n"); 00085 while(i<count && run){ 00086 s.printf("Start iteration %d\r\n", i); 00087 gpio_action(); 00088 s.printf("Done iteration %d\r\n", i); 00089 i++; 00090 } 00091 00092 goto run; 00093 00094 } else { 00095 00096 s.printf("ERROR: %s unhandled action\r\n",action); 00097 } 00098 00099 00100 00101 00102 00103 00104 00105 } 00106
Generated on Sat Jul 16 2022 21:19:55 by
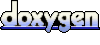