
EasyCAT shield loopback test - EtherCAT slave example
Dependencies: mbed EasyCAT_lib
main.cpp
00001 //******************************************************************************************** 00002 // * 00003 // AB&T Tecnologie Informatiche - Ivrea Italy * 00004 // http://www.bausano.net * 00005 // https://www.ethercat.org/en/products/791FFAA126AD43859920EA64384AD4FD.htm * 00006 // * 00007 //******************************************************************************************** 00008 // * 00009 // This software is distributed as an example, in the hope that it could be useful, * 00010 // WITHOUT ANY WARRANTY, even the implied warranty of FITNESS FOR A PARTICULAR PURPOSE * 00011 // * 00012 //******************************************************************************************** 00013 00014 00015 // revision 2 - moved "DigitalOut Led(LED1)" 00016 00017 00018 00019 //---- AB&T EasyCAT shield loopback test 170912 --------------------------------------------- 00020 // 00021 // Derived from the example Arduino project TestEasyCAT_LoopBack.ino 00022 // for the AB&T EasyCAT Arduino shield 00023 // 00024 // In this example the output bytes received by the EasyCAT are transmitted back to 00025 // the master, for test pourpose 00026 // 00027 // The EasyCAT must be set in STANDARD MODE (default setting) 00028 00029 //----- Tested with the STM32 NUCLEO-F767ZI board -------------------------------------------- 00030 00031 00032 #include "mbed.h" 00033 #include "EasyCAT.h" // EasyCAT library to interface the LAN9252 00034 00035 00036 void Application (void); 00037 00038 00039 00040 EasyCAT EASYCAT; // EasyCAT instantiation 00041 00042 00043 //--- sanity check to see if the EasyCAT is set in STANDARD MODE -------------------------------------- 00044 00045 #ifdef CUST_BYTE_NUM_OUT 00046 #error "The EasyCAT must be set in STANDARD MODE !!!" 00047 #endif 00048 00049 #ifdef CUST_BYTE_NUM_IN 00050 #error "The EasyCAT must be set in STANDARD MODE !!!" 00051 #endif 00052 00053 00054 //---- pins declaration ------------------------------------------------------------------------------ 00055 00056 00057 00058 00059 //--------------------------------------------------------------------------------------------- 00060 00061 int main(void) 00062 { 00063 00064 printf ("\nEasyCAT - Generic EtherCAT slave\n"); // print the banner 00065 00066 //---- initialize the EasyCAT board ----- 00067 00068 if (EASYCAT.Init() == true) // initialization 00069 { // succesfully completed 00070 printf ("initialized\n"); // 00071 } 00072 00073 else // initialization failed 00074 { // the EasyCAT board was not recognized 00075 printf ("initialization failed\n"); // 00076 // The most common reason is that the SPI 00077 // chip select choosen on the board doesn't 00078 // match the one choosen by the firmware 00079 00080 DigitalOut Led(LED1); // 00081 00082 while (1) // stay in loop for ever 00083 { // with the led blinking 00084 Led = 1; // 00085 wait_ms(125); // 00086 Led = 0; // 00087 wait_ms(125); // 00088 } // 00089 } 00090 00091 00092 while (1) //---- main loop --------------------------- 00093 { 00094 // In the main loop we must call ciclically the 00095 // EasyCAT task and our application 00096 // 00097 // This allows the bidirectional exachange of the data 00098 // between the EtherCAT master and our application 00099 // 00100 // The EasyCAT cycle and the Master cycle are asynchronous 00101 00102 wait_ms(10); // This delay allows us to set the EasyCAT cycle time 00103 // according to the needs of our application 00104 00105 EASYCAT.MainTask(); // execute the EasyCAT task 00106 00107 Application(); // execute the user application 00108 } 00109 } 00110 00111 00112 //---- user application ------------------------------------------------------------------------------ 00113 00114 void Application () // the received bytes for the outputs 00115 // are transmitted back, simulating the inputs 00116 { 00117 uint8_t Index; 00118 00119 for (Index=0; Index < BYTE_NUM; Index++) // BYTE_NUM is a constant declared in the library file "EasyCAT.h" 00120 // it defines the number of bytes for the input and for the output 00121 // when the EasyCAT is configured in STANDARD MODE (default setting) 00122 00123 { // the data are crossed and complemented 00124 EASYCAT.BufferIn.Byte[BYTE_NUM - 1 - Index] = EASYCAT.BufferOut.Byte[Index] ^ 0xFF; 00125 00126 // the data are trasmitted back as they are 00127 //EASYCAT.BufferIn.Byte[Index] = EASYCAT.BufferOut.Byte[Index]; 00128 } 00129 } 00130 00131 00132 00133 00134 00135 00136 00137 00138 00139
Generated on Tue Jul 26 2022 05:49:20 by
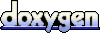