Interface library for the Atmel Inertial One IMU. Contains drivers for the ITG 3200 3 axis gyro, BMA-150 3 axis accelerometer, and AK8975 3 axis compass
IMU.h
00001 /* Atmel Inertial One IMU Library 00002 * Copyright (c) 2012 Daniel Kouba 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 #ifndef IMU_AIO_H //IMU Atmel Inertial One 00024 #define IMU_AIO_H 00025 00026 #include "mbed.h" 00027 00028 /* Defines */ 00029 00030 //7 bit I2C Addresses, shifted left to allow for read/write bit as LSB 00031 00032 #define GYRO_ADR 0x68 << 1 00033 #define ACC_ADR 0x38 << 1 00034 #define COMP_ADR 0x0C << 1 00035 00036 // ITG3200 Gyro Registers 00037 00038 #define GYRO_WHO_AM_I_REG 0x00 00039 #define GYRO_SAMPLE_DIV_REG 0x15 00040 #define GYRO_DLPF_REG 0x16 00041 #define GYRO_INT_CFG_REG 0x17 00042 #define GYRO_INT_STATUS_REG 0x1A 00043 #define GYRO_TEMP_H_REG 0x1B 00044 #define GYRO_TEMP_L_REG 0x1C 00045 #define GYRO_XOUT_H_REG 0x1D 00046 #define GYRO_XOUT_L_REG 0x1E 00047 #define GYRO_YOUT_H_REG 0x1F 00048 #define GYRO_YOUT_L_REG 0x20 00049 #define GYRO_ZOUT_H_REG 0x21 00050 #define GYRO_ZOUT_L_REG 0x22 00051 00052 // Gyro LPF bandwidths 00053 00054 #define BW_256HZ 0x00 //8kHz sample rate 00055 #define BW_188HZ 0x01 //1kHz sample rate for 188Hz and below 00056 #define BW_98HZ 0x02 00057 #define BW_42HZ 0x03 00058 #define BW_20HZ 0x04 00059 #define BW_10HZ 0x05 00060 #define BW_5HZ 0x06 00061 00062 // Gyro Initial FS_SEL Register Config 00063 00064 #define FS_SEL_INIT 0x03 << 3 00065 00066 // BMA150 Accelerometer Registers 00067 00068 #define ACC_XOUT_L_REG 0x02 //Acceleration data registers 00069 #define ACC_XOUT_H_REG 0x03 00070 #define ACC_YOUT_L_REG 0x04 00071 #define ACC_YOUT_H_REG 0x05 00072 #define ACC_ZOUT_L_REG 0x06 00073 #define ACC_ZOUT_H_REG 0x07 00074 #define ACC_TEMP_REG 0x08 //Temperature register, 0.5 deg. C / LSB 00075 #define ACC_CTRL_REG 0x0A //Control registers 00076 #define ACC_OPER_REG 0x14 //Operational registers 00077 #define ACC_INT_REG 0x15 //Interrupt registers 00078 00079 // Accelerometer LPF bandwidths 00080 00081 #define BW_25HZ 0x00 00082 #define BW_50HZ 0x01 00083 #define BW_100HZ 0x02 00084 #define BW_190HZ 0x03 00085 #define BW_375HZ 0x04 00086 #define BW_750HZ 0x05 00087 #define BW_1500HZ 0x06 00088 00089 // Accelerometer Range values 00090 00091 #define RANGE_2G 0x00 00092 #define RANGE_4G 0x01 00093 #define RANGE_8G 0x02 00094 00095 // AK8973 Compass Registers 00096 00097 00098 /** Atmel Inertial One IMU Control Class 00099 * 00100 * Includes control routines for: 00101 * - ITG-3200 3-axis, 16 bit gyroscope 00102 * - BMA-150 3-axis, 10 bit accelerometer 00103 * - AK8975 3-axis, 16 bit magnetometer 00104 * 00105 * Datasheets: 00106 * 00107 * http://www.atmel.com/dyn/resources/prod_documents/doc8354.pdf 00108 * 00109 * http://invensense.com/mems/gyro/documents/PS-ITG-3200A.pdf 00110 * 00111 * http://www.bosch-sensortec.com/content/language1/downloads/BMA150_DataSheet_Rev.1.5_30May2008.pdf 00112 * 00113 * http://pdf1.alldatasheet.com/datasheet-pdf/view/219477/AKM/AK8973/+Q18W89VYpLawLCDwv+/datasheet.pdf 00114 * 00115 */ 00116 class IMU { 00117 00118 public: 00119 struct data3d 00120 { 00121 int x, y, z; 00122 } gyro_data, acc_data, mag_data; 00123 00124 public: 00125 00126 /** Creates IMU object and initializes all three chips 00127 * 00128 * Gyro: FS_SEL register is set to 0x03, as required by datasheet 00129 * 00130 * Accelerometer: 00131 * 00132 * Compass: 00133 * 00134 * @param sda pin for I2C sda signal 00135 * @param scl pin for I2C scl signal 00136 */ 00137 IMU(PinName sda, PinName scl); 00138 00139 /* Gyro Methods */ 00140 00141 /** Gets current ADC data from all axes of gyro (uses burst read mode) 00142 * 00143 * @return data3d struct of X, Y, and Z axis gyro measurements (signed 16 bits) 00144 */ 00145 data3d getGyroData(void); 00146 00147 /** Sets digital LPF bandwidth for all gyro channels - Not working currently 00148 * 00149 * @param bw Filter Bandwidth (use defined bandwidths) 00150 */ 00151 void setGyroLPF(char bw); 00152 00153 /* Accelerometer Methods */ 00154 00155 /** Gets current X acceleration 00156 * 00157 * @return Raw X acceleration, 10 bits signed 00158 */ 00159 int accX(void); 00160 00161 /** Gets current Y acceleration 00162 * 00163 * @return Raw Y acceleration, 10 bits signed 00164 */ 00165 int accY(void); 00166 00167 /** Gets current Z acceleration 00168 * 00169 * @return Raw Z acceleration, 10 bits signed 00170 */ 00171 int accZ(void); 00172 00173 /** Gets current acceleration on all axes 00174 * 00175 * @return data3d struct of acceleration data, signed ints 00176 */ 00177 data3d getAccData(void); 00178 00179 /** Sets digital LPF filter bandwidth 00180 * 00181 * @param bw Digital LPF filter bandwidth 00182 */ 00183 void setAccLPF(char bw); 00184 00185 /** Sets accelerometer measurement range (+/-2, 4, or 8g's) 00186 * 00187 * @param range Range of g measurements 00188 */ 00189 void setAccRange(char range); 00190 00191 /** Updates all image registers with data stored in EEPROM 00192 * 00193 */ 00194 void accUpdateImage(void); 00195 00196 /** Set EEPROM write enable 00197 * 00198 * @param we Write enable value 00199 */ 00200 void accEEWriteEn(bool we); 00201 00202 /* Compass Methods */ 00203 00204 00205 00206 private: 00207 00208 I2C _i2c; //I2C object constructor 00209 00210 }; 00211 00212 #endif
Generated on Fri Jul 15 2022 08:01:50 by
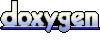