
This is the project for the Old Model Robots for OU's Dr. Davis's Configurable Robots Research. This is being published so future robots can be set up easily.
Dependencies: FatFileSystem MCP3008 Motor PinDetect QTR_8A SRF05 SSD1308_128x64_I2C mbed
Wiimote.h
00001 /* Copyright (c) 2011, mbed 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy 00004 * of this software and associated documentation files (the "Software"), to deal 00005 * in the Software without restriction, including without limitation the rights 00006 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00007 * copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in 00011 * all copies or substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00014 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00015 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00016 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00017 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00018 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00019 * THE SOFTWARE. 00020 */ 00021 00022 // Simple decoder class for Wii Remote data 00023 00024 #ifndef WIIMOTE_H 00025 #define WIIMOTE_H 00026 00027 #include <stdint.h> 00028 00029 class Wiimote { 00030 00031 public: 00032 00033 void decode(char *data); 00034 void dump(); 00035 00036 // buttons 00037 uint32_t left:1; 00038 uint32_t right:1; 00039 uint32_t down:1; 00040 uint32_t up:1; 00041 uint32_t plus:1; 00042 uint32_t two:1; 00043 uint32_t one:1; 00044 uint32_t a:1; 00045 uint32_t b:1; 00046 uint32_t minus:1; 00047 uint32_t home:1; 00048 00049 // accelerometers 00050 uint16_t rawx; 00051 uint16_t rawy; 00052 uint16_t rawz; 00053 00054 float x; 00055 float y; 00056 float z; 00057 float wheel; 00058 }; 00059 00060 #endif 00061
Generated on Wed Jul 13 2022 03:10:51 by
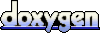