
Websocket example
Fork of Websocket_Ethernet_HelloWorld by
Embed:
(wiki syntax)
Show/hide line numbers
config.cpp
00001 #include "config.h" 00002 #include "ATcommand.h" 00003 00004 int configFromFile(char* path, Serial *xbee, Serial *pc, char * url){ 00005 pc->printf("\n\rConfig Filename: %s", path); 00006 00007 int maxRetry = 2; 00008 00009 FILE* file = fopen(path, "r"); 00010 char line[256]; 00011 while (fgets(line, sizeof(line), file)) { 00012 bool delimiterFound = false; 00013 00014 // Line is a comment 00015 if( (line[0]=='#') || (line[0]=='\n')){ 00016 pc->printf("\n\r%s", line); 00017 00018 // Line is url config 00019 }else if(strstr(line, "url") != NULL){ 00020 int ptr = 0; 00021 for(int i =0;i<sizeof(line)&& line[i]!='\0'; i++){ 00022 if(!delimiterFound){ 00023 delimiterFound = (line[i]=='='); 00024 }else{ 00025 url[ptr] = line[i]; 00026 ptr++; 00027 } 00028 } 00029 pc->printf("Url:%s", url); 00030 00031 // Others line is AT COMMAND 00032 }else{ 00033 int ptr = 0; 00034 bool EOL = false; 00035 pc->printf("AT Command\n\r"); 00036 for(int i =0;i<sizeof(line)&& !EOL; i++){ 00037 if(!delimiterFound){ 00038 delimiterFound = (line[i]=='='); 00039 pc->printf("%c",line[i]); 00040 }else{ 00041 00042 // Send command 00043 char *cmd = NULL; 00044 char *params = NULL; 00045 int cmd_len = 0; 00046 int param_len = 0; 00047 00048 for( ;i<sizeof(line)&& line[i]!= '\0'; i=i+2){ 00049 unsigned char c = ahex2bin(line[i],line[i+1]); 00050 if(c == 0xDA){ 00051 EOL =true; 00052 }else if(cmd_len <2){ 00053 cmd = (char*)realloc(cmd ,sizeof(char)*(cmd_len+1)); 00054 cmd[cmd_len++] = c; 00055 }else{ 00056 params = (char*)realloc(params ,sizeof(char)*(param_len+1)); 00057 params[param_len++] = c; 00058 } 00059 } 00060 00061 do{ 00062 bool exit = false; 00063 sendAT(xbee, pc, cmd, params,param_len); 00064 wait_ms(200); 00065 ATWR (xbee, pc); 00066 wait_ms(200); 00067 apply(xbee, pc); 00068 wait_ms(200); 00069 // Verify command status 00070 char status = getStatusResponse(xbee, pc); 00071 if(status == 0x00){ 00072 pc->printf("\n\rStatus OK"); 00073 exit =true; 00074 }else{ 00075 if(status==0x02){ 00076 pc->printf("\n\Invalid Command %d\n\r",status); 00077 } 00078 toggleError(xbee, pc, 200, "Warning status fail"); 00079 maxRetry--; 00080 } 00081 }while(maxRetry>0 && !exit); 00082 free(cmd); 00083 free(params); 00084 00085 EOL =true; 00086 } 00087 } 00088 } 00089 } 00090 fclose(file); 00091 pc->printf("\n\rClose file"); 00092 return 0; 00093 } 00094 00095 00096 unsigned char ahex2bin (unsigned char MSB, unsigned char LSB){ 00097 if (MSB > '9') MSB -= 7; // Convert MSB (0x30..0x3F) 00098 if (LSB > '9') LSB -= 7; // Convert LSB (0x30..0x3F) 00099 return (MSB << 4) | (LSB & 0x0F); 00100 } 00101
Generated on Sat Jul 16 2022 18:21:49 by
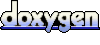