NTP
Dependents: DISCO-F746NG_Ethernet gather_sensor_data final DISCO-F746NG_Ethernet
NTPClient.h
00001 /* NTPClient.h */ 00002 /* Copyright (C) 2012 mbed.org, MIT License 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 00020 /** \file 00021 NTP Client header file 00022 */ 00023 00024 #ifndef NTPCLIENT_H_ 00025 #define NTPCLIENT_H_ 00026 00027 #include <cstdint> 00028 00029 using std::uint8_t; 00030 using std::uint16_t; 00031 using std::uint32_t; 00032 00033 #include "UDPSocket.h" 00034 00035 #define NTP_DEFAULT_PORT 123 00036 #define NTP_DEFAULT_TIMEOUT 4000 00037 00038 ///NTP client results 00039 enum NTPResult 00040 { 00041 NTP_DNS, ///<Could not resolve name 00042 NTP_PRTCL, ///<Protocol error 00043 NTP_TIMEOUT, ///<Connection timeout 00044 NTP_CONN, ///<Connection error 00045 NTP_OK = 0, ///<Success 00046 }; 00047 00048 /** NTP Client to update the mbed's RTC using a remote time server 00049 * 00050 */ 00051 class NTPClient 00052 { 00053 public: 00054 /** 00055 Instantiate the NTP client 00056 */ 00057 NTPClient(); 00058 00059 /**Get current time (blocking) 00060 Update the time using the server host 00061 Blocks until completion 00062 @param host NTP server IPv4 address or hostname (will be resolved via DNS) 00063 @param port port to use; defaults to 123 00064 @param timeout waiting timeout in ms (osWaitForever for blocking function, not recommended) 00065 @return 0 on success, NTP error code (<0) on failure 00066 */ 00067 NTPResult setTime(const char* host, uint16_t port = NTP_DEFAULT_PORT, uint32_t timeout = NTP_DEFAULT_TIMEOUT); //Blocking 00068 00069 private: 00070 struct NTPPacket //See RFC 4330 for Simple NTP 00071 { 00072 //WARN: We are in LE! Network is BE! 00073 //LSb first 00074 unsigned mode : 3; 00075 unsigned vn : 3; 00076 unsigned li : 2; 00077 00078 uint8_t stratum; 00079 uint8_t poll; 00080 uint8_t precision; 00081 //32 bits header 00082 00083 uint32_t rootDelay; 00084 uint32_t rootDispersion; 00085 uint32_t refId; 00086 00087 uint32_t refTm_s; 00088 uint32_t refTm_f; 00089 uint32_t origTm_s; 00090 uint32_t origTm_f; 00091 uint32_t rxTm_s; 00092 uint32_t rxTm_f; 00093 uint32_t txTm_s; 00094 uint32_t txTm_f; 00095 } __attribute__ ((packed)); 00096 00097 UDPSocket m_sock; 00098 00099 }; 00100 00101 00102 #endif /* NTPCLIENT_H_ */
Generated on Tue Jul 12 2022 21:11:48 by
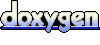