R1 code for micro:bit based train controller code, requires second micro:bit running rx code to operate - see https://meanderingpi.wordpress.com/ for more information
Fork of nrf51-sdk by
ble_conn_state.h
00001 /* 00002 * Copyright (c) Nordic Semiconductor ASA 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without modification, 00006 * are permitted provided that the following conditions are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright notice, this 00009 * list of conditions and the following disclaimer. 00010 * 00011 * 2. Redistributions in binary form must reproduce the above copyright notice, this 00012 * list of conditions and the following disclaimer in the documentation and/or 00013 * other materials provided with the distribution. 00014 * 00015 * 3. Neither the name of Nordic Semiconductor ASA nor the names of other 00016 * contributors to this software may be used to endorse or promote products 00017 * derived from this software without specific prior written permission. 00018 * 00019 * 00020 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 00021 * ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00022 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00023 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR 00024 * ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00025 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00026 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON 00027 * ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00028 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00029 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00030 * 00031 */ 00032 00033 /** 00034 * @file 00035 * 00036 * @defgroup ble_conn_state Connection state 00037 * @ingroup ble_sdk_lib 00038 * @{ 00039 * @brief Module for storing data on BLE connections. 00040 * 00041 * @details This module stores certain states for each connection, which can be queried by 00042 * connection handle. The module uses BLE events to keep the states updated. 00043 * 00044 * In addition to the preprogrammed states, this module can also keep track of a number of 00045 * binary user states, or <i>user flags</i>. These are reset to 0 for new connections, but 00046 * otherwise not touched by this module. 00047 * 00048 * This module uses the @ref sdk_mapped_flags module, with connection handles as keys and 00049 * the connection states as flags. 00050 * 00051 * @note A connection handle is not immediately invalidated when it is disconnected. Certain states, 00052 * such as the role, can still be queried until the next time a new connection is established 00053 * to any device. 00054 * 00055 * To function properly, this module must be provided with BLE events from the SoftDevice 00056 * through the @ref ble_conn_state_on_ble_evt() function. This module should be the first 00057 * to receive BLE events if they are dispatched to multiple modules. 00058 */ 00059 00060 #ifndef BLE_CONN_STATE_H__ 00061 #define BLE_CONN_STATE_H__ 00062 00063 #include <stdbool.h> 00064 #include <stdint.h> 00065 #include "ble.h" 00066 #include "sdk_mapped_flags.h " 00067 00068 /**@brief Connection handle statuses. 00069 */ 00070 typedef enum 00071 { 00072 BLE_CONN_STATUS_INVALID, /**< The connection handle is invalid. */ 00073 BLE_CONN_STATUS_DISCONNECTED, /**< The connection handle refers to a connection that has been disconnected, but not yet invalidated. */ 00074 BLE_CONN_STATUS_CONNECTED, /**< The connection handle refers to an active connection. */ 00075 } ble_conn_state_status_t; 00076 00077 #define BLE_CONN_STATE_N_USER_FLAGS 16 /**< The number of available user flags. */ 00078 00079 00080 /**@brief One ID for each user flag collection. 00081 * 00082 * @details These IDs are used to identify user flag collections in the API calls. 00083 */ 00084 typedef enum 00085 { 00086 BLE_CONN_STATE_USER_FLAG0 = 0, 00087 BLE_CONN_STATE_USER_FLAG1, 00088 BLE_CONN_STATE_USER_FLAG2, 00089 BLE_CONN_STATE_USER_FLAG3, 00090 BLE_CONN_STATE_USER_FLAG4, 00091 BLE_CONN_STATE_USER_FLAG5, 00092 BLE_CONN_STATE_USER_FLAG6, 00093 BLE_CONN_STATE_USER_FLAG7, 00094 BLE_CONN_STATE_USER_FLAG8, 00095 BLE_CONN_STATE_USER_FLAG9, 00096 BLE_CONN_STATE_USER_FLAG10, 00097 BLE_CONN_STATE_USER_FLAG11, 00098 BLE_CONN_STATE_USER_FLAG12, 00099 BLE_CONN_STATE_USER_FLAG13, 00100 BLE_CONN_STATE_USER_FLAG14, 00101 BLE_CONN_STATE_USER_FLAG15, 00102 BLE_CONN_STATE_USER_FLAG_INVALID, 00103 } ble_conn_state_user_flag_id_t; 00104 00105 00106 /** 00107 * @defgroup ble_conn_state_functions BLE connection state functions 00108 * @{ 00109 */ 00110 00111 00112 /**@brief Function for initializing or resetting the module. 00113 * 00114 * @details This function sets all states to their default, removing all records of connection handles. 00115 */ 00116 void ble_conn_state_init(void); 00117 00118 00119 /**@brief Function for providing BLE SoftDevice events to the connection state module. 00120 * 00121 * @param[in] p_ble_evt The SoftDevice event. 00122 */ 00123 void ble_conn_state_on_ble_evt(ble_evt_t * p_ble_evt); 00124 00125 00126 /**@brief Function for querying whether a connection handle represents a valid connection. 00127 * 00128 * @details A connection might be valid and have a BLE_CONN_STATUS_DISCONNECTED status. 00129 * Those connections are invalidated after a new connection occurs. 00130 * 00131 * @param[in] conn_handle Handle of the connection. 00132 * 00133 * @retval true If conn_handle represents a valid connection, thus a connection for which 00134 we have a record. 00135 * @retval false If conn_handle is @ref BLE_GAP_ROLE_INVALID, or if it has never been recorded. 00136 */ 00137 bool ble_conn_state_valid(uint16_t conn_handle); 00138 00139 00140 /**@brief Function for querying the role of the local device in a connection. 00141 * 00142 * @param[in] conn_handle Handle of the connection to get the role for. 00143 * 00144 * @return The role of the local device in the connection (see @ref BLE_GAP_ROLES). 00145 * If conn_handle is not valid, the function returns BLE_GAP_ROLE_INVALID. 00146 */ 00147 uint8_t ble_conn_state_role(uint16_t conn_handle); 00148 00149 00150 /**@brief Function for querying the status of a connection. 00151 * 00152 * @param[in] conn_handle Handle of the connection. 00153 * 00154 * @return The status of the connection. 00155 * If conn_handle is not valid, the function returns BLE_CONN_STATE_INVALID. 00156 */ 00157 ble_conn_state_status_t ble_conn_state_status(uint16_t conn_handle); 00158 00159 00160 /**@brief Function for querying whether a connection is encrypted. 00161 * 00162 * @param[in] conn_handle Handle of connection to get the encryption state for. 00163 * 00164 * @retval true If the connection is encrypted. 00165 * @retval false If the connection is not encrypted or conn_handle is invalid. 00166 */ 00167 bool ble_conn_state_encrypted(uint16_t conn_handle); 00168 00169 00170 /**@brief Function for querying whether a connection encryption is protected from Man in the Middle 00171 * attacks. 00172 * 00173 * @param[in] conn_handle Handle of connection to get the MITM state for. 00174 * 00175 * @retval true If the connection is encrypted with MITM protection. 00176 * @retval false If the connection is not encrypted, or encryption is not MITM protected, or 00177 * conn_handle is invalid. 00178 */ 00179 bool ble_conn_state_mitm_protected(uint16_t conn_handle); 00180 00181 00182 /**@brief Function for querying the total number of connections. 00183 * 00184 * @return The total number of valid connections for which the module has a record. 00185 */ 00186 uint32_t ble_conn_state_n_connections(void); 00187 00188 00189 /**@brief Function for querying the total number of connections in which the role of the local 00190 * device is @ref BLE_GAP_ROLE_CENTRAL. 00191 * 00192 * @return The number of connections in which the role of the local device is 00193 * @ref BLE_GAP_ROLE_CENTRAL. 00194 */ 00195 uint32_t ble_conn_state_n_centrals(void); 00196 00197 00198 /**@brief Function for querying the total number of connections in which the role of the local 00199 * device is @ref BLE_GAP_ROLE_PERIPH. 00200 * 00201 * @return The number of connections in which the role of the local device is 00202 * @ref BLE_GAP_ROLE_PERIPH. 00203 */ 00204 uint32_t ble_conn_state_n_peripherals(void); 00205 00206 00207 /**@brief Function for obtaining a list of all connection handles for which the module has a record. 00208 * 00209 * @details This function takes into account connections whose state is BLE_CONN_STATUS_DISCONNECTED. 00210 * 00211 * @return A list of all valid connection handles for which the module has a record. 00212 */ 00213 sdk_mapped_flags_key_list_t ble_conn_state_conn_handles(void); 00214 00215 00216 /**@brief Function for obtaining a list of connection handles in which the role of the local 00217 * device is @ref BLE_GAP_ROLE_CENTRAL. 00218 * 00219 * @details This function takes into account connections whose state is BLE_CONN_STATUS_DISCONNECTED. 00220 * 00221 * @return A list of all valid connection handles for which the module has a record and in which 00222 * the role of local device is @ref BLE_GAP_ROLE_CENTRAL. 00223 */ 00224 sdk_mapped_flags_key_list_t ble_conn_state_central_handles(void); 00225 00226 00227 /**@brief Function for obtaining the handle for the connection in which the role of the local device 00228 * is @ref BLE_GAP_ROLE_PERIPH. 00229 * 00230 * @details This function takes into account connections whose state is BLE_CONN_STATUS_DISCONNECTED. 00231 * 00232 * @return A list of all valid connection handles for which the module has a record and in which 00233 * the role of local device is @ref BLE_GAP_ROLE_PERIPH. 00234 */ 00235 sdk_mapped_flags_key_list_t ble_conn_state_periph_handles(void); 00236 00237 00238 /**@brief Function for obtaining exclusive access to one of the user flag collections. 00239 * 00240 * @details The acquired collection contains one flag for each connection. These flags can be set 00241 * and read individually for each connection. 00242 * 00243 * The state of user flags will not be modified by the connection state module, except to 00244 * set it to 0 for a connection when that connection is invalidated. 00245 * 00246 * @return The ID of the acquired flag, or BLE_CONN_STATE_USER_FLAG_INVALID if none are available. 00247 */ 00248 ble_conn_state_user_flag_id_t ble_conn_state_user_flag_acquire(void); 00249 00250 00251 /**@brief Function for reading the value of a user flag. 00252 * 00253 * @param[in] conn_handle Handle of connection to get the flag state for. 00254 * @param[in] flag_id Which flag to get the state for. 00255 * 00256 * @return The state of the flag. If conn_handle is invalid, the function returns false. 00257 */ 00258 bool ble_conn_state_user_flag_get(uint16_t conn_handle, ble_conn_state_user_flag_id_t flag_id); 00259 00260 00261 /**@brief Function for setting the value of a user flag. 00262 * 00263 * @param[in] conn_handle Handle of connection to set the flag state for. 00264 * @param[in] flag_id Which flag to set the state for. 00265 * @param[in] value Value to set the flag state to. 00266 */ 00267 void ble_conn_state_user_flag_set(uint16_t conn_handle, 00268 ble_conn_state_user_flag_id_t flag_id, 00269 bool value); 00270 00271 00272 /**@brief Function for getting the state of a user flag for all connection handles. 00273 * 00274 * @details The returned collection can be used with the @ref sdk_mapped_flags API. The returned 00275 * collection is a copy, so modifying it has no effect on the conn_state module. 00276 * 00277 * @param[in] flag_id Which flag to get states for. 00278 * 00279 * @return The collection of flag states. The collection is always all zeros when the flag_id is 00280 * unregistered. 00281 */ 00282 sdk_mapped_flags_t ble_conn_state_user_flag_collection(ble_conn_state_user_flag_id_t flag_id); 00283 00284 /** @} */ 00285 /** @} */ 00286 00287 #endif /* BLE_CONN_STATE_H__ */
Generated on Tue Jul 12 2022 19:00:11 by
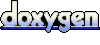