R1 code for micro:bit based train controller code, requires second micro:bit running rx code to operate - see https://meanderingpi.wordpress.com/ for more information
Fork of nrf51-sdk by
app_error.c
00001 /* 00002 * Copyright (c) Nordic Semiconductor ASA 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without modification, 00006 * are permitted provided that the following conditions are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright notice, this 00009 * list of conditions and the following disclaimer. 00010 * 00011 * 2. Redistributions in binary form must reproduce the above copyright notice, this 00012 * list of conditions and the following disclaimer in the documentation and/or 00013 * other materials provided with the distribution. 00014 * 00015 * 3. Neither the name of Nordic Semiconductor ASA nor the names of other 00016 * contributors to this software may be used to endorse or promote products 00017 * derived from this software without specific prior written permission. 00018 * 00019 * 00020 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 00021 * ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00022 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00023 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR 00024 * ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00025 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00026 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON 00027 * ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00028 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00029 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00030 * 00031 */ 00032 00033 /** @file 00034 * 00035 * @defgroup app_error Common application error handler 00036 * @{ 00037 * @ingroup app_common 00038 * 00039 * @brief Common application error handler. 00040 */ 00041 00042 #include "nrf.h" 00043 #include "app_error.h " 00044 #include "compiler_abstraction.h" 00045 #include "nordic_common.h" 00046 #ifdef DEBUG 00047 #include "bsp.h" 00048 00049 /* global error variables - in order to prevent removal by optimizers */ 00050 uint32_t m_error_code; 00051 uint32_t m_line_num; 00052 const uint8_t * m_p_file_name; 00053 #endif 00054 00055 /**@brief Function for error handling, which is called when an error has occurred. 00056 * 00057 * @warning This handler is an example only and does not fit a final product. You need to analyze 00058 * how your product is supposed to react in case of error. 00059 * 00060 * @param[in] error_code Error code supplied to the handler. 00061 * @param[in] line_num Line number where the handler is called. 00062 * @param[in] p_file_name Pointer to the file name. 00063 * 00064 * Function is implemented as weak so that it can be overwritten by custom application error handler 00065 * when needed. 00066 */ 00067 00068 /*lint -save -e14 */ 00069 __WEAK void app_error_handler(uint32_t error_code, uint32_t line_num, const uint8_t * p_file_name) 00070 { 00071 // On assert, the system can only recover with a reset. 00072 #ifndef DEBUG 00073 NVIC_SystemReset(); 00074 #else 00075 00076 #ifdef BSP_DEFINES_ONLY 00077 LEDS_ON(LEDS_MASK); 00078 #else 00079 UNUSED_VARIABLE(bsp_indication_set(BSP_INDICATE_FATAL_ERROR)); 00080 // This call can be used for debug purposes during application development. 00081 // @note CAUTION: Activating this code will write the stack to flash on an error. 00082 // This function should NOT be used in a final product. 00083 // It is intended STRICTLY for development/debugging purposes. 00084 // The flash write will happen EVEN if the radio is active, thus interrupting 00085 // any communication. 00086 // Use with care. Uncomment the line below to use. 00087 //ble_debug_assert_handler(error_code, line_num, p_file_name); 00088 #endif // BSP_DEFINES_ONLY 00089 00090 // The following variable helps Keil keep the call stack visible, in addition, it can be set to 00091 // 0 in the debugger to continue executing code after the error check. 00092 volatile bool loop = true; 00093 UNUSED_VARIABLE(loop); 00094 00095 m_error_code = error_code; 00096 m_line_num = line_num; 00097 m_p_file_name = p_file_name; 00098 00099 UNUSED_VARIABLE(m_error_code); 00100 UNUSED_VARIABLE(m_line_num); 00101 UNUSED_VARIABLE(m_p_file_name); 00102 __disable_irq(); 00103 00104 while(loop); 00105 #endif // DEBUG 00106 } 00107 /*lint -restore */
Generated on Tue Jul 12 2022 19:00:11 by
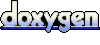