R1 code for micro:bit based train controller code, requires second micro:bit running tx code to operate - see https://meanderingpi.wordpress.com/ for more information
Fork of mbed-dev-bin by
DigitalInOut.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_DIGITALINOUT_H 00017 #define MBED_DIGITALINOUT_H 00018 00019 #include "platform.h" 00020 00021 #include "gpio_api.h" 00022 00023 namespace mbed { 00024 00025 /** A digital input/output, used for setting or reading a bi-directional pin 00026 */ 00027 class DigitalInOut { 00028 00029 public: 00030 /** Create a DigitalInOut connected to the specified pin 00031 * 00032 * @param pin DigitalInOut pin to connect to 00033 */ 00034 DigitalInOut(PinName pin) : gpio() { 00035 gpio_init_in(&gpio, pin); 00036 } 00037 00038 /** Create a DigitalInOut connected to the specified pin 00039 * 00040 * @param pin DigitalInOut pin to connect to 00041 * @param direction the initial direction of the pin 00042 * @param mode the initial mode of the pin 00043 * @param value the initial value of the pin if is an output 00044 */ 00045 DigitalInOut(PinName pin, PinDirection direction, PinMode mode, int value) : gpio() { 00046 gpio_init_inout(&gpio, pin, direction, mode, value); 00047 } 00048 00049 /** Set the output, specified as 0 or 1 (int) 00050 * 00051 * @param value An integer specifying the pin output value, 00052 * 0 for logical 0, 1 (or any other non-zero value) for logical 1 00053 */ 00054 void write(int value) { 00055 gpio_write(&gpio, value); 00056 } 00057 00058 /** Return the output setting, represented as 0 or 1 (int) 00059 * 00060 * @returns 00061 * an integer representing the output setting of the pin if it is an output, 00062 * or read the input if set as an input 00063 */ 00064 int read() { 00065 return gpio_read(&gpio); 00066 } 00067 00068 /** Set as an output 00069 */ 00070 void output() { 00071 gpio_dir(&gpio, PIN_OUTPUT); 00072 } 00073 00074 /** Set as an input 00075 */ 00076 void input() { 00077 gpio_dir(&gpio, PIN_INPUT); 00078 } 00079 00080 /** Set the input pin mode 00081 * 00082 * @param mode PullUp, PullDown, PullNone, OpenDrain 00083 */ 00084 void mode(PinMode pull) { 00085 gpio_mode(&gpio, pull); 00086 } 00087 00088 /** Return the output setting, represented as 0 or 1 (int) 00089 * 00090 * @returns 00091 * Non zero value if pin is connected to uc GPIO 00092 * 0 if gpio object was initialized with NC 00093 */ 00094 int is_connected() { 00095 return gpio_is_connected(&gpio); 00096 } 00097 00098 #ifdef MBED_OPERATORS 00099 /** A shorthand for write() 00100 */ 00101 DigitalInOut& operator= (int value) { 00102 write(value); 00103 return *this; 00104 } 00105 00106 DigitalInOut& operator= (DigitalInOut& rhs) { 00107 write(rhs.read()); 00108 return *this; 00109 } 00110 00111 /** A shorthand for read() 00112 */ 00113 operator int() { 00114 return read(); 00115 } 00116 #endif 00117 00118 protected: 00119 gpio_t gpio; 00120 }; 00121 00122 } // namespace mbed 00123 00124 #endif
Generated on Tue Jul 12 2022 17:43:37 by
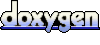