Upload
Fork of RenBED_RGB by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /********************************************************* 00002 *RenBED_RGB * 00003 *Author: Elijah Orr * 00004 * * 00005 *A program that cycles through the 9 different states * 00006 *(including off) of a common anode RGB LED that are * 00007 *available via digital pin control. * 00008 *********************************************************/ 00009 00010 /* include the mbed library made by mbed.org that contains 00011 classes/functions designed to make programming mbed 00012 microcontrollers easier */ 00013 #include "mbed.h" 00014 00015 /* Set up 3 pins as digital out to control the colour 00016 cathodes of the RGB LED */ 00017 DigitalOut Green(p18); 00018 DigitalOut Red(p20); 00019 DigitalOut Blue(p19); 00020 00021 /* the main function is where a program will begin to execute. */ 00022 00023 /**************************************************************** 00024 * Function: main() * 00025 * * 00026 * Sequences an RBG LED connected to the RenBED * 00027 * * 00028 * Inputs: none * 00029 * * 00030 * Returns: none * 00031 ****************************************************************/ 00032 int main() 00033 { 00034 /* open a for loop with no parameters to start an infinite loop */ 00035 for(;;){ 00036 Red = 1; 00037 Blue = 1; 00038 Green = 1; 00039 wait_ms(1000); 00040 Red = 1; 00041 Blue = 1; 00042 Green = 0; 00043 wait_ms(1000); 00044 Red = 1; 00045 Blue = 0; 00046 Green = 1; 00047 wait_ms(1000); 00048 Red = 1; 00049 Blue = 0; 00050 Green = 0; 00051 wait_ms(1000); 00052 Red = 0; 00053 Blue = 1; 00054 Green = 1; 00055 wait_ms(1000); 00056 Red = 0; 00057 Blue = 1; 00058 Green = 0; 00059 wait_ms(1000); 00060 Red = 0; 00061 Blue = 0; 00062 Green = 1; 00063 wait_ms(1000); 00064 Red = 0; 00065 Blue = 0; 00066 Green = 0; 00067 wait_ms(3000); 00068 } 00069 } 00070 00071
Generated on Sat Jul 23 2022 12:07:21 by
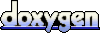