
RenBuggyTimed with edited function and access to seven segment display
Dependencies: SevenSegmentDisplay mbed
Fork of 1-RenBuggyTimed by
TimedMovement.cpp
00001 /********************************************************* 00002 *TimedMovement.cpp * 00003 *Author: Dan Argust * 00004 * * 00005 *A library of functions that can be used to control the * 00006 *RenBuggy. * 00007 *********************************************************/ 00008 00009 #ifndef TIMEDMOVEMENT_C 00010 #define TIMEDMOVEMENT_C 00011 00012 /* necessary includes */ 00013 #include "mbed.h" 00014 #include "TimedMovement.h" 00015 #include "SevenSegmentDisplay.h" 00016 00017 /* PwmOut is a class included in the mbed.h library that allows 00018 a pin to be configured as a PWM output. This is used to control 00019 the speed of the motors. Lmotor and Rmotor are chosen names for 00020 the pins, LeftMotorPin and RightMotorPin (see TimedMovement.h) 00021 specify the physical pins to be used. */ 00022 PwmOut Lmotor(LeftMotorPin); 00023 PwmOut Rmotor(RightMotorPin); 00024 00025 DigitalIn CurrentButtonState(p7); 00026 bool LastButtonState = 0; 00027 00028 SevenSegmentDisplay seg(0); 00029 00030 /* Function definitions contain the code that will execute when 00031 the function is called. These must have the same return type 00032 and parameters as the function declarations. */ 00033 00034 /**************************************************************** 00035 * Function: forward() * 00036 * * 00037 * Moves the RenBuggy directly forwards * 00038 * * 00039 * Inputs: A floating point value representing the length of * 00040 * time the buggy will move for * 00041 * * 00042 * Returns: none * 00043 ****************************************************************/ 00044 extern void hold(float time) 00045 { 00046 seg.DisplayDigits(0,0); 00047 for (float i = time;i>0;i-=0.01){ 00048 seg.DisplayDigits((int)i/10,(int)i%10); 00049 //if (CurrentButtonState == 1 and LastButtonState == 0){ 00050 // rollDice(); 00051 //} 00052 //LastButtonState = CurrentButtonState; 00053 wait(0.01); 00054 } 00055 } 00056 00057 extern void forward(float time) 00058 { 00059 /* Lmotor and Rmotor are set to 1.0 (i.e. the motors will 00060 operate at full speed). As both motors will move at the 00061 same speed, the RenBuggy will go directly forward. */ 00062 //Lmotor = Rmotor = 1.0; 00063 Lmotor = Rmotor = 1.0; 00064 /* the program will wait here for the length of time passed 00065 to the function before continuing. hold() is used to wait a 00066 number of seconds and display them on the seven seg display */ 00067 hold(time); 00068 stop(); 00069 } 00070 00071 /**************************************************************** 00072 * Function: left() * 00073 * * 00074 * Turns the RenBuggy to the left * 00075 * * 00076 * Inputs: A floating point value representing the length of * 00077 * time the buggy will turn for * 00078 * * 00079 * Returns: none * 00080 ****************************************************************/ 00081 extern void left(float time) 00082 { 00083 Rmotor = 1.0; 00084 Lmotor = 0.0; 00085 hold(time); 00086 //readButton(time); 00087 stop(); 00088 } 00089 00090 /**************************************************************** 00091 * Function: right() * 00092 * * 00093 * Turns the RenBuggy to the right * 00094 * * 00095 * Inputs: A floating point value representing the length of * 00096 * time the buggy will turn for * 00097 * * 00098 * Returns: none * 00099 ****************************************************************/ 00100 extern void right(float time) 00101 { 00102 Lmotor = 1.0; 00103 Rmotor = 0.0; 00104 hold(time); 00105 stop(); 00106 } 00107 00108 /**************************************************************** 00109 * Function: stop() * 00110 * * 00111 * Brings the RenBuggy to a complete stop * 00112 * * 00113 * Inputs: none * 00114 * * 00115 * Returns: none * 00116 ****************************************************************/ 00117 extern void stop() 00118 { 00119 Lmotor = Rmotor = 0; 00120 } 00121 00122 extern void readButton(float time) 00123 { 00124 seg.DisplayDigits(0,0); 00125 for (float i = time;i>0;i-=0.01) 00126 { 00127 if (CurrentButtonState == 1 and LastButtonState == 0){ 00128 rollDice(); 00129 //stop(); 00130 //right(2.0); 00131 } 00132 LastButtonState = CurrentButtonState; 00133 wait(0.01); 00134 } 00135 } 00136 00137 extern int rollDice() 00138 { 00139 int tens; 00140 int units; 00141 for (int i = 20;i>0;i--){ 00142 tens = rand()%9; 00143 units = rand()%9; 00144 seg.DisplayDigits(tens,units); 00145 wait(0.04); 00146 } 00147 return tens*10+units; 00148 } 00149 #endif // TIMEDMOVEMENT_C
Generated on Fri Jul 15 2022 21:21:34 by
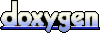