
Red Light Work
Dependencies: EthernetInterface QEI_hw QEIx4 mbed-rtos mbed realtimeMMLib
Fork of realtimeMM_V3 by
MMiniFileReader.cpp
00001 #include "MMiniFileReader.h" 00002 #include <iostream> 00003 #include <fstream> 00004 #include "mbed.h" 00005 #include <sstream> 00006 #include <string> 00007 00008 using namespace std; 00009 00010 iniFileReader::iniFileReader() 00011 { 00012 } 00013 00014 00015 iniFileReader::~iniFileReader() 00016 { 00017 } 00018 00019 iniFile iniFileReader::ReadFile(const char* filePath) 00020 { 00021 iniFile fileConfig; 00022 00023 char line[32]; 00024 //std::ifstream myfile(filePath); 00025 00026 //Serial pc(USBTX, USBRX); 00027 00028 00029 //std::ifstream myfile("filePath"); 00030 00031 //pc.printf("file=%s \n", filePath); 00032 00033 FILE *File2 = fopen(filePath, "r"); // open file for reading 00034 //fgets(read_string,256,File2); // read first data value 00035 //pc.printf("text data: %s \n", read_string); 00036 00037 00038 if (File2) 00039 { 00040 char section[32]; 00041 00042 while (fgets(line, 32, File2)) 00043 { 00044 00045 if (IsSection(line)) 00046 { 00047 GetSectionName(line, section); 00048 } 00049 else 00050 { 00051 00052 if ( strncmp(section , "Station", 7) == 0) 00053 { 00054 char paramName[32]; 00055 00056 GetParamName(line, paramName); 00057 00058 if (strncmp(paramName, "ID", 2) == 0) 00059 GetParamValue(line, fileConfig.Station.ID); 00060 00061 if (strncmp(paramName, "Name", 4) == 0) 00062 GetParamValue(line, fileConfig.Station.Name); 00063 00064 if (strncmp(paramName, "ComsType", 8 ) == 0) 00065 GetParamValue(line, fileConfig.Station.ComsType); 00066 00067 if (strncmp(paramName, "IPAddress",9) == 0) 00068 GetParamValue(line, fileConfig.Station.IPAddress); 00069 00070 if (strncmp(paramName, "NetworkMask", 11) == 0) 00071 GetParamValue(line, fileConfig.Station.NetworkMask); 00072 00073 if (strncmp(paramName, "DefaultGateway", 14) == 0) 00074 GetParamValue(line, fileConfig.Station.DefaultGateway); 00075 00076 if (strncmp(paramName, "DataSendTime", 12) == 0) 00077 GetParamValue(line, fileConfig.Station.DataSendTime); 00078 } 00079 00080 00081 if (strncmp(section, "Server", 6) == 0) 00082 { 00083 char paramName[32]; 00084 00085 GetParamName(line, paramName); 00086 00087 if (strncmp(paramName, "IPAddress", 9) == 0) 00088 GetParamValue(line, fileConfig.Server.IPAddress); 00089 00090 if (strncmp(paramName, "Port", 4) == 0) 00091 GetParamValue(line, fileConfig.Server.Port); 00092 } 00093 00094 ReadSensorSection(section, "Sensor1",7, line, &fileConfig.Sensor1); 00095 ReadSensorSection(section, "Sensor2", 7, line, &fileConfig.Sensor2); 00096 ReadSensorSection(section, "Sensor3", 7, line, &fileConfig.Sensor3); 00097 ReadSensorSection(section, "Sensor4", 7, line, &fileConfig.Sensor4); 00098 ReadSensorSection(section, "Sensor5", 7, line, &fileConfig.Sensor5); 00099 ReadSensorSection(section, "Sensor6", 7, line, &fileConfig.Sensor6); 00100 ReadSensorSection(section, "Sensor7", 7, line, &fileConfig.Sensor7); 00101 ReadSensorSection(section, "Sensor8", 7, line, &fileConfig.Sensor8); 00102 ReadSensorSection(section, "Sensor9", 7, line, &fileConfig.Sensor9); 00103 ReadSensorSection(section, "Sensor10", 8, line, &fileConfig.Sensor10); 00104 00105 } 00106 00107 00108 } 00109 fclose(File2); 00110 } 00111 00112 return fileConfig; 00113 } 00114 00115 00116 void iniFileReader::ReadSensorSection(char currentSection[], char section[], int size, char line[], iniSensor *sensor) 00117 { 00118 00119 if (strncmp(currentSection, section, size) == 0) 00120 { 00121 char paramName[32]; 00122 00123 GetParamName(line, paramName); 00124 00125 if (strncmp(paramName, "ID", 2) == 0) 00126 GetParamValue(line, (*sensor).ID); 00127 00128 if (strncmp(paramName, "Type",4) == 0) 00129 GetParamValue(line, (*sensor).SensorType); 00130 00131 if (strncmp(paramName, "Enabled", 7) == 0) 00132 GetParamValue(line, (*sensor).Enabled); 00133 00134 if (strncmp(paramName, "SampleTime",10) == 0) 00135 GetParamValue(line, (*sensor).SampleTime); 00136 00137 if (strncmp(paramName, "DataSampleQty",13) ==0 ) 00138 GetParamValue(line, (*sensor).DataSampleQty); 00139 00140 if (strncmp(paramName, "Pins", 4) == 0 ) 00141 GetParamValue(line, (*sensor).Pins); 00142 00143 if (strncmp(paramName, "SensorOptions", 13) == 0) 00144 GetParamValue(line, (*sensor).SensorOptions); 00145 } 00146 00147 } 00148 00149 00150 00151 bool iniFileReader::IsSection(char line[]) 00152 { 00153 if (line[0] == '[') 00154 { 00155 return true; 00156 } 00157 00158 return false; 00159 } 00160 00161 void iniFileReader::GetSectionName(char line[], char* result) 00162 { 00163 int start = 0; 00164 00165 for (int i = 1; i < 32; i++) { 00166 if (line[i] == ']') { 00167 result[start] = '\n'; 00168 break; 00169 } 00170 00171 00172 result[start] = line[i]; 00173 start++; 00174 } 00175 00176 00177 //int pos = line - 2; 00178 //string sectionName = line.substr(1, pos); 00179 00180 //return sectionName; 00181 } 00182 00183 void iniFileReader::GetParamName(char line[], char* result) 00184 { 00185 00186 for (int i = 0; i < 32; i++) { 00187 if (line[i] == '=') 00188 break; 00189 00190 result[i] = line[i]; 00191 } 00192 00193 } 00194 00195 void iniFileReader::GetParamValue(char line[], char* result) 00196 { 00197 bool found = false; 00198 int start = 0; 00199 00200 for (int i = 0; i < 32; i++) { 00201 00202 if (!found) { 00203 if (line[i] == '=') 00204 found = true; 00205 } 00206 else 00207 { 00208 if (line[i] != '\n' && line[i] != NULL) 00209 result[start] = line[i]; 00210 else 00211 { 00212 result[start] = '\0'; 00213 break; 00214 } 00215 00216 start++; 00217 } 00218 } 00219 }
Generated on Sun Jul 17 2022 04:44:05 by
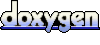