
Demonstrate what can happen when array limits are exceeded.
Fork of SimpleConsoleTest by
main.cpp
00001 00002 /* 00003 File: main.cpp 00004 Project: ArrayLimits 00005 00006 Demonstrates abuse of array limits on Nucleo boards. 00007 00008 If necessary, avoid this with code like: 00009 00010 if (index > SIZE - 1) { 00011 index = SIZE - 1; 00012 } else if (index < 0) { 00013 index = 0; 00014 } 00015 Use index... 00016 00017 Created by Dr. C. S. Tritt; Last revised 10/12/17 (v. 1.0) 00018 */ 00019 #include "mbed.h" 00020 00021 int main() 00022 { 00023 float a[] {1.0, 3.0, 5.0, 7.0, 9.0 }; 00024 printf("Hello World !\n\n"); 00025 00026 for (int i = 0; i > -10; i--) { // Displays zeros and one NaN. 00027 printf("a[%d] is %f.\n", i, a[i]); 00028 wait(0.5f); 00029 } 00030 00031 for (int i = 0; i < 10; i++) { // Hangs after 6th value! 00032 printf("a[%d] is %f.\n", i, a[i]); 00033 wait(0.5f); 00034 } 00035 00036 while (true) {}; // Just loop here forever after tests. 00037 }
Generated on Wed Jul 13 2022 15:32:42 by
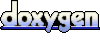