
Labview
Dependencies: C12832_lcd LCD_fonts LM75B analogintest3 MMA7660 mbed
Fork of analogintest3 by
main.cpp
00001 #include "mbed.h" 00002 #include "SerialRPCInterface.h" 00003 #include "LM75B.h" 00004 #include "MMA7660.h" 00005 #include <string> 00006 00007 #include "C12832_lcd.h" 00008 #include "Arial_9.h" 00009 #include "Small_7.h" 00010 #include "graphics.h" 00011 SerialRPCInterface SerialInterface(USBTX, USBRX); 00012 float ai1=0; 00013 float ai2=0; 00014 int alarm1 = 0; 00015 int alarm2 = 0; 00016 float temp = 0; 00017 int text; 00018 float period; 00019 int position; 00020 00021 float X = 0; 00022 float Y = 0; 00023 float Z = 0; 00024 00025 Serial pc(USBTX, USBRX); 00026 RPCVariable<float> rpc_ai1(&ai1,"ai1"); 00027 00028 RPCVariable<float> rpc_ai2(&ai2,"ai2"); 00029 00030 RPCVariable<int> rpc_alarm1(&alarm1,"alarm1"); 00031 RPCVariable<int> rpc_alarm2(&alarm2,"alarm2"); 00032 RPCVariable<float> rpc_temp(&temp,"temp"); 00033 RPCVariable<int> rpc_text(&text,"text"); 00034 RPCVariable<float> rpc_period(&period,"period"); 00035 RPCVariable<int> rpc_position(&position,"position"); 00036 00037 RPCVariable<float> rpc_X(&X,"X"); 00038 RPCVariable<float> rpc_Y(&Y,"Y"); 00039 RPCVariable<float> rpc_Z(&Z,"Z"); 00040 00041 AnalogIn pot2(p19); 00042 DigitalOut led(LED1); 00043 AnalogIn pot1(p20); 00044 DigitalOut led2(LED2); 00045 LM75B tmp(p28,p27); 00046 C12832_LCD lcd; 00047 PwmOut r (p23); 00048 PwmOut g (p24); 00049 PwmOut b (p25); 00050 PwmOut spkr(p26); 00051 PwmOut servo(p21); 00052 MMA7660 MMA(p28, p27); 00053 00054 00055 void Welkom(void); 00056 00057 int main() { 00058 //Labview 00059 Base::add_rpc_class<AnalogIn>(); 00060 Base::add_rpc_class<AnalogOut>(); 00061 Base::add_rpc_class<DigitalIn>(); 00062 Base::add_rpc_class<DigitalOut>(); 00063 Base::add_rpc_class<DigitalInOut>(); 00064 Base::add_rpc_class<PwmOut>(); 00065 Base::add_rpc_class<Timer>(); 00066 Base::add_rpc_class<SPI>(); 00067 Base::add_rpc_class<BusOut>(); 00068 Base::add_rpc_class<BusIn>(); 00069 Base::add_rpc_class<BusInOut>(); 00070 Base::add_rpc_class<Serial>(); 00071 00072 char buf[256], outbuf[256]; 00073 00074 00075 lcd.cls(); 00076 //update axis period 00077 r.period(0.001); 00078 Welkom(); 00079 wait(2); 00080 lcd.cls(); 00081 00082 while (1){ 00083 00084 pc.gets(buf,256); 00085 rpc(buf,outbuf); 00086 pc.printf("%s\n",outbuf); 00087 00088 temp = tmp.read() - 2; 00089 00090 lcd.cls(); 00091 lcd.locate(0,15); 00092 lcd.printf(" Nummer: %d",text); 00093 lcd.copy_to_lcd(); 00094 00095 00096 00097 ai1 = pot2; 00098 ai2 = pot1; 00099 if(pot2 > 0.3) { 00100 led = 1; 00101 alarm1 = led; 00102 } else { 00103 led = 0; 00104 alarm1 = led; 00105 } 00106 if(pot1 > 0.7) { 00107 led2 = 1; 00108 alarm2 = led2; 00109 } else { 00110 led2 = 0; 00111 alarm2 = led2; 00112 } 00113 00114 X = MMA.x(); 00115 Y = MMA.y(); 00116 Z = MMA.z(); 00117 //------------------------ 00118 spkr.period(period /100000); 00119 spkr=0.5; 00120 00121 servo.period_ms(20); 00122 servo=((position) * 0.000511 + 0.026); 00123 00124 00125 } 00126 } 00127 00128 void Welkom(void) 00129 { 00130 int i,s; 00131 lcd.cls(); 00132 lcd.set_font((unsigned char*) Arial_9); 00133 s = 3; 00134 lcd.print_bm(bitmTree,95,0); // print chistmas tree 00135 lcd.copy_to_lcd(); 00136 lcd.setmode(XOR); // XOR - a second print will erase 00137 for(i = -15; i < 75; ){ 00138 lcd.print_bm(bitmSan1,i,2); 00139 wait(0.1); 00140 lcd.copy_to_lcd(); // update lcd 00141 lcd.print_bm(bitmSan1,i,2); // erase 00142 i= i+s; 00143 lcd.print_bm(bitmSan2,i,2); // print next 00144 wait(0.1); 00145 lcd.copy_to_lcd(); // update lcd 00146 lcd.print_bm(bitmSan2,i,2); // erase 00147 i= i+s; 00148 lcd.print_bm(bitmSan3,i,2); // print next 00149 wait(0.1); 00150 lcd.copy_to_lcd(); // update lcd 00151 lcd.print_bm(bitmSan3,i,2); // erase 00152 i= i+s; 00153 } 00154 lcd.print_bm(bitmSan3,i,2); 00155 lcd.set_auto_up(0); 00156 for(i=-20; i<5; i++){ // scrolling text 00157 lcd.locate(5,i); 00158 lcd.printf("Welkom bij"); 00159 lcd.locate(5,i+12); 00160 lcd.printf("Labview!!!"); 00161 lcd.copy_to_lcd(); 00162 lcd.locate(5,i); 00163 wait(0.2); 00164 lcd.printf("Welkom bij"); 00165 lcd.locate(5,i+12); 00166 lcd.printf("Labview!!!"); 00167 lcd.copy_to_lcd(); 00168 i=i+1; 00169 } 00170 lcd.locate(5,i); 00171 lcd.printf("Welkom bij"); 00172 lcd.locate(5,i+12); 00173 lcd.printf("Labview!!!"); 00174 lcd.copy_to_lcd(); 00175 }
Generated on Fri Jul 15 2022 01:49:40 by
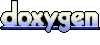