W5500 driver for mbed OS 5
Dependents: http-webserver-example mbed-os-example-sockets
Fork of W5500Interface by
W5500Interface.h
00001 /** 00002 ****************************************************************************** 00003 * @file W5500Interface.h 00004 * @author Bongjun Hur (modified version from Sergei G (https://os.mbed.com/users/sgnezdov/)) 00005 * @brief Header file of the NetworkStack for the W5500 Device 00006 ****************************************************************************** 00007 * @attention 00008 * 00009 * THE PRESENT FIRMWARE WHICH IS FOR GUIDANCE ONLY AIMS AT PROVIDING CUSTOMERS 00010 * WITH CODING INFORMATION REGARDING THEIR PRODUCTS IN ORDER FOR THEM TO SAVE 00011 * TIME. AS A RESULT, WIZnet SHALL NOT BE HELD LIABLE FOR ANY 00012 * DIRECT, INDIRECT OR CONSEQUENTIAL DAMAGES WITH RESPECT TO ANY CLAIMS ARISING 00013 * FROM THE CONTENT OF SUCH FIRMWARE AND/OR THE USE MADE BY CUSTOMERS OF THE 00014 * CODING INFORMATION CONTAINED HEREIN IN CONNECTION WITH THEIR PRODUCTS. 00015 * 00016 * <h2><center>© COPYRIGHT 2017,2018 WIZnet Co.,Ltd.</center></h2> 00017 ****************************************************************************** 00018 */ 00019 00020 #ifndef W5500_INTERFACE_H 00021 #define W5500_INTERFACE_H 00022 00023 #include "mbed.h" 00024 #include "W5500.h" 00025 //#include "rtos.h" 00026 #include "PinNames.h" 00027 #include "DHCPClient.h" 00028 #include "DNSClient.h" 00029 00030 // Arduino pin defaults for convenience 00031 #if !defined(W5500_SPI_MOSI) 00032 #define W5500_SPI_MOSI D11 00033 #endif 00034 #if !defined(W5500_SPI_MISO) 00035 #define W5500_SPI_MISO D12 00036 #endif 00037 #if !defined(W5500_SPI_SCLK) 00038 #define W5500_SPI_SCLK D13 00039 #endif 00040 #if !defined(W5500_SPI_CS) 00041 #define W5500_SPI_CS D10 00042 #endif 00043 #if !defined(W5500_SPI_RST) 00044 #define W5500_SPI_RST NC 00045 #endif 00046 00047 00048 /** w5500_socket struct 00049 * W5500 socket 00050 */ 00051 00052 struct w5500_socket { 00053 int fd; 00054 nsapi_protocol_t proto; 00055 bool connected; 00056 void (*callback)(void *); 00057 void *callback_data; 00058 }; 00059 00060 /** W5500Interface class 00061 * Implementation of the NetworkStack for the W5500 00062 */ 00063 00064 class W5500Interface : public NetworkStack, public EthInterface 00065 { 00066 public: 00067 //W5500Interface(SPI* spi, PinName cs, PinName reset); 00068 W5500Interface(PinName mosi, PinName miso, PinName sclk, PinName cs, PinName reset); 00069 00070 int init(); 00071 int init(uint8_t * mac); 00072 int init(const char* ip, const char* mask, const char* gateway); 00073 int init(uint8_t * mac, const char* ip, const char* mask, const char* gateway); 00074 00075 /** Start the interface 00076 * @return 0 on success, negative on failure 00077 */ 00078 virtual int connect(); 00079 00080 /** Stop the interface 00081 * @return 0 on success, negative on failure 00082 */ 00083 virtual int disconnect(); 00084 00085 /** Get the internally stored IP address 00086 * @return IP address of the interface or null if not yet connected 00087 */ 00088 virtual const char *get_ip_address(); 00089 /** Get the local gateway 00090 * 00091 * @return Null-terminated representation of the local gateway 00092 * or null if no network mask has been recieved 00093 */ 00094 virtual const char *get_gateway(); 00095 00096 /** Get the local network mask 00097 * 00098 * @return Null-terminated representation of the local network mask 00099 * or null if no network mask has been recieved 00100 */ 00101 virtual const char *get_netmask(); 00102 00103 /** Get MAC address and fill mac with it. 00104 */ 00105 void get_mac(uint8_t mac[6]) ; 00106 00107 /** Get the internally stored MAC address 00108 * @return MAC address of the interface 00109 */ 00110 virtual const char *get_mac_address(); 00111 00112 /** Set a static IP address 00113 * 00114 * Configures this network interface to use a static IP address. 00115 * Implicitly disables DHCP, which can be enabled in set_dhcp. 00116 * Requires that the network is disconnected. 00117 * 00118 * @param ip_address Null-terminated representation of the local IP address 00119 * @param netmask Null-terminated representation of the local network mask 00120 * @param gateway Null-terminated representation of the local gateway 00121 * @return 0 on success, negative error code on failure 00122 */ 00123 virtual nsapi_error_t set_network(const char *ip_address, const char *netmask, const char *gateway); 00124 00125 /** Enable or disable DHCP on the network 00126 * 00127 * Enables DHCP on connecting the network. Defaults to enabled unless 00128 * a static IP address has been assigned. Requires that the network is 00129 * disconnected. 00130 * 00131 * @param dhcp True to enable DHCP 00132 * @return 0 on success, negative error code on failure 00133 */ 00134 virtual nsapi_error_t set_dhcp(bool dhcp); 00135 00136 bool setDnsServerIP(const char* ip_address); 00137 00138 /** Translates a hostname to an IP address with specific version 00139 * 00140 * The hostname may be either a domain name or an IP address. If the 00141 * hostname is an IP address, no network transactions will be performed. 00142 * 00143 * If no stack-specific DNS resolution is provided, the hostname 00144 * will be resolve using a UDP socket on the stack. 00145 * 00146 * @param address Destination for the host SocketAddress 00147 * @param host Hostname to resolve 00148 * @param version IP version of address to resolve, NSAPI_UNSPEC indicates 00149 * version is chosen by the stack (defaults to NSAPI_UNSPEC) 00150 * @return 0 on success, negative error code on failure 00151 */ 00152 //using NetworkInterface::gethostbyname; 00153 virtual nsapi_error_t gethostbyname(const char *host, 00154 SocketAddress *address, nsapi_version_t version = NSAPI_UNSPEC); 00155 00156 int IPrenew(int timeout_ms = 15*1000); 00157 uint32_t str_to_ip(const char* str); 00158 00159 protected: 00160 /** Opens a socket 00161 * 00162 * Creates a network socket and stores it in the specified handle. 00163 * The handle must be passed to following calls on the socket. 00164 * 00165 * A stack may have a finite number of sockets, in this case 00166 * NSAPI_ERROR_NO_SOCKET is returned if no socket is available. 00167 * 00168 * @param handle Destination for the handle to a newly created socket 00169 * @param proto Protocol of socket to open, NSAPI_TCP or NSAPI_UDP 00170 * @return 0 on success, negative error code on failure 00171 */ 00172 virtual nsapi_error_t socket_open(nsapi_socket_t *handle, nsapi_protocol_t proto); 00173 // virtual int socket_open(void **handle, nsapi_protocol_t proto); 00174 00175 /** Close the socket 00176 * 00177 * Closes any open connection and deallocates any memory associated 00178 * with the socket. 00179 * 00180 * @param handle Socket handle 00181 * @return 0 on success, negative error code on failure 00182 */ 00183 virtual nsapi_error_t socket_close(nsapi_socket_t handle); 00184 00185 /** Bind a specific address to a socket 00186 * 00187 * Binding a socket specifies the address and port on which to recieve 00188 * data. If the IP address is zeroed, only the port is bound. 00189 * 00190 * @param handle Socket handle 00191 * @param address Local address to bind 00192 * @return 0 on success, negative error code on failure. 00193 */ 00194 virtual nsapi_error_t socket_bind(nsapi_socket_t handle, const SocketAddress &address); 00195 00196 /** Listen for connections on a TCP socket 00197 * 00198 * Marks the socket as a passive socket that can be used to accept 00199 * incoming connections. 00200 * 00201 * @param handle Socket handle 00202 * @param backlog Number of pending connections that can be queued 00203 * simultaneously 00204 * @return 0 on success, negative error code on failure 00205 */ 00206 virtual nsapi_error_t socket_listen(nsapi_socket_t handle, int backlog); 00207 00208 /** Connects TCP socket to a remote host 00209 * 00210 * Initiates a connection to a remote server specified by the 00211 * indicated address. 00212 * 00213 * @param handle Socket handle 00214 * @param address The SocketAddress of the remote host 00215 * @return 0 on success, negative error code on failure 00216 */ 00217 virtual nsapi_error_t socket_connect(nsapi_socket_t handle, const SocketAddress &address); 00218 00219 /** Accepts a connection on a TCP socket 00220 * 00221 * The server socket must be bound and set to listen for connections. 00222 * On a new connection, creates a network socket and stores it in the 00223 * specified handle. The handle must be passed to following calls on 00224 * the socket. 00225 * 00226 * A stack may have a finite number of sockets, in this case 00227 * NSAPI_ERROR_NO_SOCKET is returned if no socket is available. 00228 * 00229 * This call is non-blocking. If accept would block, 00230 * NSAPI_ERROR_WOULD_BLOCK is returned immediately. 00231 * 00232 * @param server Socket handle to server to accept from 00233 * @param handle Destination for a handle to the newly created socket 00234 * @param address Destination for the remote address or NULL 00235 * @return 0 on success, negative error code on failure 00236 */ 00237 virtual nsapi_error_t socket_accept(nsapi_socket_t server, 00238 nsapi_socket_t *handle, SocketAddress *address=0); 00239 00240 /** Send data over a TCP socket 00241 * 00242 * The socket must be connected to a remote host. Returns the number of 00243 * bytes sent from the buffer. 00244 * 00245 * This call is non-blocking. If send would block, 00246 * NSAPI_ERROR_WOULD_BLOCK is returned immediately. 00247 * 00248 * @param handle Socket handle 00249 * @param data Buffer of data to send to the host 00250 * @param size Size of the buffer in bytes 00251 * @return Number of sent bytes on success, negative error 00252 * code on failure 00253 */ 00254 virtual nsapi_size_or_error_t socket_send(nsapi_socket_t handle, 00255 const void *data, nsapi_size_t size); 00256 00257 /** Receive data over a TCP socket 00258 * 00259 * The socket must be connected to a remote host. Returns the number of 00260 * bytes received into the buffer. 00261 * 00262 * This call is non-blocking. If recv would block, 00263 * NSAPI_ERROR_WOULD_BLOCK is returned immediately. 00264 * 00265 * @param handle Socket handle 00266 * @param data Destination buffer for data received from the host 00267 * @param size Size of the buffer in bytes 00268 * @return Number of received bytes on success, negative error 00269 * code on failure 00270 */ 00271 virtual nsapi_size_or_error_t socket_recv(nsapi_socket_t handle, 00272 void *data, nsapi_size_t size); 00273 00274 /** Send a packet over a UDP socket 00275 * 00276 * Sends data to the specified address. Returns the number of bytes 00277 * sent from the buffer. 00278 * 00279 * This call is non-blocking. If sendto would block, 00280 * NSAPI_ERROR_WOULD_BLOCK is returned immediately. 00281 * 00282 * @param handle Socket handle 00283 * @param address The SocketAddress of the remote host 00284 * @param data Buffer of data to send to the host 00285 * @param size Size of the buffer in bytes 00286 * @return Number of sent bytes on success, negative error 00287 * code on failure 00288 */ 00289 virtual nsapi_size_or_error_t socket_sendto(nsapi_socket_t handle, const SocketAddress &address, 00290 const void *data, nsapi_size_t size); 00291 00292 /** Receive a packet over a UDP socket 00293 * 00294 * Receives data and stores the source address in address if address 00295 * is not NULL. Returns the number of bytes received into the buffer. 00296 * 00297 * This call is non-blocking. If recvfrom would block, 00298 * NSAPI_ERROR_WOULD_BLOCK is returned immediately. 00299 * 00300 * @param handle Socket handle 00301 * @param address Destination for the source address or NULL 00302 * @param data Destination buffer for data received from the host 00303 * @param size Size of the buffer in bytes 00304 * @return Number of received bytes on success, negative error 00305 * code on failure 00306 */ 00307 virtual nsapi_size_or_error_t socket_recvfrom(nsapi_socket_t handle, SocketAddress *address, 00308 void *buffer, nsapi_size_t size); 00309 00310 /** Register a callback on state change of the socket 00311 * 00312 * The specified callback will be called on state changes such as when 00313 * the socket can recv/send/accept successfully and on when an error 00314 * occurs. The callback may also be called spuriously without reason. 00315 * 00316 * The callback may be called in an interrupt context and should not 00317 * perform expensive operations such as recv/send calls. 00318 * 00319 * @param handle Socket handle 00320 * @param callback Function to call on state change 00321 * @param data Argument to pass to callback 00322 */ 00323 virtual void socket_attach(nsapi_socket_t handle, void (*callback)(void *), void *data); 00324 00325 virtual NetworkStack* get_stack() {return this;} 00326 00327 private: 00328 WIZnet_Chip _w5500; 00329 00330 char ip_string[NSAPI_IPv4_SIZE]; 00331 char netmask_string[NSAPI_IPv4_SIZE]; 00332 char gateway_string[NSAPI_IPv4_SIZE]; 00333 char mac_string[NSAPI_MAC_SIZE]; 00334 bool ip_set; 00335 00336 int listen_port; 00337 00338 //void signal_event(nsapi_socket_t handle); 00339 //void event(); 00340 00341 //w5500 socket management 00342 struct w5500_socket w5500_sockets[MAX_SOCK_NUM]; 00343 w5500_socket* get_sock(int fd); 00344 void init_socks(); 00345 00346 DHCPClient dhcp; 00347 bool _dhcp_enable; 00348 DNSClient dns; 00349 /* 00350 Thread *_daemon; 00351 void daemon(); 00352 */ 00353 00354 }; 00355 00356 #endif
Generated on Tue Jul 12 2022 18:48:25 by
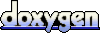