W5500 driver for mbed OS 5
Dependents: http-webserver-example mbed-os-example-sockets
Fork of W5500Interface by
DNSClient.h
00001 // DNSClient.h 2013/4/5 00002 #pragma once 00003 00004 #include "UDPSocket.h" 00005 00006 //#define DBG_DNS 1 00007 00008 class DNSClient { 00009 public: 00010 DNSClient(); 00011 DNSClient(NetworkStack *ns, const char* hostname = NULL); 00012 DNSClient(NetworkStack *ns, SocketAddress* pHost); 00013 00014 int setup(NetworkStack *ns); 00015 00016 virtual ~DNSClient(); 00017 bool lookup(const char* hostname); 00018 bool set_server(const char* serverip, int port=53); 00019 uint32_t get_ip() {return m_ip;} 00020 const char* get_ip_address() {return m_ipaddr;} 00021 00022 protected: 00023 void poll(); 00024 void callback(); 00025 int response(uint8_t buf[], int size); 00026 int query(uint8_t buf[], int size, const char* hostname); 00027 void resolve(const char* hostname); 00028 uint8_t m_id[2]; 00029 Timer m_interval; 00030 int m_retry; 00031 const char* m_hostname; 00032 00033 private: 00034 enum MyNetDnsState 00035 { 00036 MYNETDNS_START, 00037 MYNETDNS_PROCESSING, //Req has not completed 00038 MYNETDNS_NOTFOUND, 00039 MYNETDNS_ERROR, 00040 MYNETDNS_OK 00041 }; 00042 MyNetDnsState m_state; 00043 UDPSocket *m_udp; 00044 NetworkStack *m_ns; 00045 00046 uint32_t m_ip; 00047 char m_ipaddr[24]; 00048 };
Generated on Tue Jul 12 2022 18:48:25 by
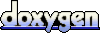