Calculations for a Dual-Arm SCARA robot Computes the shoulder angles to make the robot move to an XY position Also the reverse calculation - mainly useful as a cross-check
ScaraArms.h
00001 // SCARA_Arms library 00002 // Code to compute the shoulder angles required to move a Dual-Arm SCARA robot to required x,y position 00003 // More information at http://robdobson.com/scara-arm-calculations 00004 // Copyright (C) Rob Dobson 2013-2015, MIT License 00005 00006 #ifndef SCARAARMS_H 00007 #define SCARAARMS_H 00008 00009 // Description of a Dual-Arm SCARA robot 00010 // The two arms of the robot are akin to two human arms with the hands permanently clasped together 00011 // The arms are always in the same plane (generally horizontal) and the hands hold a stick (also in the same plane) 00012 // On the end of the stick is the tool (pen/actuayor/nozzle/laser etc) 00013 00014 class ScaraArms 00015 { 00016 public: 00017 // Constructor 00018 // The measurements required to describe a Dual-Arm SCARA robot of the kind described above are: 00019 // 1) Distance between shoulders 00020 // 2) Distance from shoulder to elbow 00021 // 3) Distance from elbow to hand 00022 // 4) Distance from hand to tool (length of stick holding the tool) 00023 ScaraArms(double betweenShouldersMM, double shoulderToElbowMM, double elbowToHandMM, double handToToolMM); 00024 00025 // Compute the angles at the shoulders given the required XY position of the tool in radians 00026 // Note that the way the angles are measured is that theta1 (the left arm angle) is measured counter-clockwise from 3 O'Clock 00027 // theta2 (the right arm angle) is measured clockwise from 9 O'Clock 00028 // To calculate both clockwise from 9 O'Clock use PI-theta2 00029 // To convert the angles to degrees multiply by 180/PI 00030 // The XY position has it's origin (0,0) at the left shoulder 00031 void ConvertXYtoScara(double x, double y, double &theta1, double &theta2); 00032 00033 // Compute the XY position of the tool given the angles at the shoulders 00034 // See the comments above for the reverse calculation concerning the way the angles are measured and the origin 00035 // of the XY coordinates 00036 // These calculations use a different mathematical model as the conversion in this direction is simpler and 00037 // it provides a useful cross check 00038 void ConvertScaratoXY(double theta1, double theta2, double &x, double &y); 00039 00040 private: 00041 double _betweenShouldersMM; 00042 double _shoulderToElbowMM; 00043 double _elbowToHandMM; 00044 double _handToToolMM; 00045 }; 00046 00047 #endif
Generated on Tue Jul 19 2022 03:59:01 by
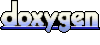