Monitor for central heating system (e.g. 2zones+hw) Supports up to 15 temp probes (DS18B20/DS18S20) 3 valve monitors Gas pulse meter recording Use stand-alone or with nodeEnergyServer See http://robdobson.com/2015/09/central-heating-monitor
Dependencies: EthernetInterfacePlusHostname NTPClient Onewire RdWebServer SDFileSystem-RTOS mbed-rtos mbed-src
RdDS18B20.h
00001 // Handles OneWire temperature sensors DB18S20 00002 // Can handle multiple devices per pin 00003 // Rob Dobson, 2015 00004 00005 #ifndef RdDS18B20__H 00006 #define RdDS18B20__H 00007 00008 #include "mbed.h" 00009 #include "Onewire.h" 00010 #include "Logger.h" 00011 00012 class DS18B20 00013 { 00014 public: 00015 DS18B20(PinName mbedPin, Logger &logger); 00016 void ReqConvert(); 00017 double ReadTemperature(int addrIdx); 00018 void DebugGetAddress(int addrIdx, char* buf); 00019 int SearchToGetAddresses(); 00020 int GetNumAddresses() 00021 { 00022 return _numValidAddresses; 00023 } 00024 uint8_t* GetAddress(int addrIdx, uint8_t* addrBufPtr); 00025 char* GetAddressStr(int addrIdx); 00026 double GetLatestTemperature(int addrIdx, time_t& timeOfReading); 00027 00028 static const int ONEWIRE_ADDR_STRLEN = 3 * ONEWIRE_ADDR_BYTES + 1; 00029 static const int MAX_BUS_DEVICES = 8; 00030 00031 static const int INVALID_TEMPERATURE = -1000.0; 00032 00033 private: 00034 int _numValidAddresses; 00035 uint8_t _addrTable[MAX_BUS_DEVICES][ONEWIRE_ADDR_BYTES]; 00036 double _temperatureTable[MAX_BUS_DEVICES]; 00037 time_t _timeOfReadingTable[MAX_BUS_DEVICES]; 00038 Onewire _oneWire; 00039 char _addrStr[ONEWIRE_ADDR_STRLEN]; 00040 Logger &_logger; 00041 char* GetChipId(int val); 00042 00043 }; 00044 00045 #endif
Generated on Tue Jul 12 2022 18:43:11 by
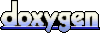