Monitor for central heating system (e.g. 2zones+hw) Supports up to 15 temp probes (DS18B20/DS18S20) 3 valve monitors Gas pulse meter recording Use stand-alone or with nodeEnergyServer See http://robdobson.com/2015/09/central-heating-monitor
Dependencies: EthernetInterfacePlusHostname NTPClient Onewire RdWebServer SDFileSystem-RTOS mbed-rtos mbed-src
Logger.cpp
00001 // Log to SD 00002 // Rob Dobson, 2015 00003 00004 #include "Logger.h" 00005 00006 Logger::Logger(const char* eventLogFileName, const char* dataLogFileBase, Mutex &sdCardMutex) : 00007 _sdCardMutex(sdCardMutex) 00008 { 00009 _eventLogFileName = eventLogFileName; 00010 _dataLogFileBase = dataLogFileBase; 00011 _logDebugToFile = false; 00012 _logDebugToConsole = false; 00013 } 00014 00015 void Logger::LogEvent(const char* format, ...) 00016 { 00017 char timeBuf[40]; 00018 time_t seconds = time(NULL); 00019 strftime(timeBuf, sizeof(timeBuf), "%Y-%m-%d\t%H:%M:%S\t", localtime(&seconds)); 00020 00021 // Obtain lock to access sd card - if unable then abort the logging process 00022 if (!_sdCardMutex.trylock()) 00023 return; 00024 00025 // Open file 00026 FILE* fp = fopen(_eventLogFileName, "a"); 00027 if (fp == NULL) 00028 { 00029 printf ("Event Log ... Filename %s not found\r\n", _eventLogFileName); 00030 } 00031 else 00032 { 00033 fprintf(fp, timeBuf); 00034 va_list argptr; 00035 va_start(argptr, format); 00036 vfprintf(fp, format, argptr); 00037 va_end(argptr); 00038 fprintf(fp, "\r\n"); 00039 fclose(fp); 00040 } 00041 00042 // Release lock on sd card 00043 _sdCardMutex.unlock(); 00044 00045 } 00046 00047 // Utility function to log data 00048 void Logger::LogData(const char* format, ...) 00049 { 00050 char fileNameBuf[60]; 00051 strcpy(fileNameBuf, _dataLogFileBase); 00052 time_t seconds = time(NULL); 00053 strftime(fileNameBuf+strlen(fileNameBuf), sizeof(fileNameBuf)-strlen(fileNameBuf), "Data_%Y%m%d.txt", localtime(&seconds)); 00054 00055 // Obtain lock to access sd card - if unable then abort the logging process 00056 if (!_sdCardMutex.trylock()) 00057 return; 00058 00059 FILE* fp = fopen(fileNameBuf, "a"); 00060 if (fp == NULL) 00061 { 00062 printf ("Data Log ... Filename %s not found\r\n", _eventLogFileName); 00063 } 00064 else 00065 { 00066 va_list argptr; 00067 va_start(argptr, format); 00068 vfprintf(fp, format, argptr); 00069 va_end(argptr); 00070 fprintf(fp, "\r\n"); 00071 fclose(fp); 00072 } 00073 00074 // Release lock on sd card 00075 _sdCardMutex.unlock(); 00076 } 00077 00078 // Utility function to log data 00079 void Logger::LogDebug(const char* format, ...) 00080 { 00081 char fileNameBuf[60]; 00082 strcpy(fileNameBuf, _dataLogFileBase); 00083 time_t seconds = time(NULL); 00084 strftime(fileNameBuf+strlen(fileNameBuf), sizeof(fileNameBuf)-strlen(fileNameBuf), "Dbg_%Y%m%d.txt", localtime(&seconds)); 00085 00086 // Log to file if enabled 00087 if (_logDebugToFile) 00088 { 00089 // Obtain lock to access sd card - if unable then abort the logging process 00090 if (_sdCardMutex.trylock()) 00091 { 00092 FILE* fp = fopen(fileNameBuf, "a"); 00093 if (fp == NULL) 00094 { 00095 printf ("Data Log ... Filename %s not found\r\n", _eventLogFileName); 00096 } 00097 else 00098 { 00099 char timeBuf[40]; 00100 time_t seconds = time(NULL); 00101 strftime(timeBuf, sizeof(timeBuf), "%Y-%m-%d\t%H:%M:%S\t", localtime(&seconds)); 00102 fprintf(fp, timeBuf); 00103 va_list argptr; 00104 va_start(argptr, format); 00105 vfprintf(fp, format, argptr); 00106 va_end(argptr); 00107 fprintf(fp, "\r\n"); 00108 } 00109 fclose(fp); 00110 00111 // Release lock on sd card 00112 _sdCardMutex.unlock(); 00113 } 00114 } 00115 00116 // Print to terminal if enabled 00117 if (_logDebugToConsole) 00118 { 00119 va_list argptr; 00120 va_start(argptr, format); 00121 vprintf(format, argptr); 00122 va_end(argptr); 00123 printf("\r\n"); 00124 } 00125 }
Generated on Tue Jul 12 2022 18:43:11 by
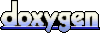