Monitor for central heating system (e.g. 2zones+hw) Supports up to 15 temp probes (DS18B20/DS18S20) 3 valve monitors Gas pulse meter recording Use stand-alone or with nodeEnergyServer See http://robdobson.com/2015/09/central-heating-monitor
Dependencies: EthernetInterfacePlusHostname NTPClient Onewire RdWebServer SDFileSystem-RTOS mbed-rtos mbed-src
GasUseCounter.h
00001 #ifndef __GASUSECOUNTER__H 00002 #define __GASUSECOUNTER__H 00003 #include "mbed.h" 00004 #include "PulsePin.h" 00005 #include "SDFileSystem.h" 00006 #include "Logger.h" 00007 00008 const int MAX_GAS_COUNT_STR_LEN = 50; 00009 const int MAX_PULSES_BEFORE_STORE_NV = 1; // Store at each pulse 00010 00011 class GasUseCounter 00012 { 00013 public: 00014 // Constructor 00015 GasUseCounter(const char* gasUseFilename1, const char* gasUseFilename2, DigitalIn& gasPulsePin, Logger &logger, Mutex &sdCardMutex) : 00016 _gasPulsePin(gasPulsePin), _logger(logger), _sdCardMutex(sdCardMutex) 00017 { 00018 _gasUseFilename1 = gasUseFilename1; 00019 _gasUseFilename2 = gasUseFilename2; 00020 _lastWrittenGasCount = 0; 00021 _pulseDetector = new PulsePin(_gasPulsePin, false, 200); 00022 } 00023 00024 // Init (get count from NV) 00025 void Init(); 00026 00027 // Callback from web server to handle getting current gas count 00028 char* getGasUseCallback(char* cmdStr, char* argStr); 00029 00030 // Service function to detect pulses 00031 bool Service(); 00032 00033 // Read/Write current gas count 00034 void GetGasCountFromSD(); 00035 void WriteGasCountToSD(); 00036 00037 // Get Count 00038 int GetCount() 00039 { 00040 return _pulseDetector->GetPulseCount(); 00041 } 00042 00043 // Set Count 00044 void SetCount(int gasUseCount) 00045 { 00046 _pulseDetector->SetPulseCount(gasUseCount); 00047 WriteGasCountToSD(); 00048 } 00049 00050 // Get inter-pulse time 00051 int GetPulseRateMs() 00052 { 00053 return _pulseDetector->GetPulseRateMs(); 00054 } 00055 00056 private: 00057 // Gas use filename for non-volatile 00058 const char* _gasUseFilename1; 00059 const char* _gasUseFilename2; 00060 int _curGasCount; 00061 int _lastWrittenGasCount; 00062 char _gasCountStr [MAX_GAS_COUNT_STR_LEN]; 00063 DigitalIn& _gasPulsePin; 00064 PulsePin* _pulseDetector; 00065 Logger &_logger; 00066 Mutex &_sdCardMutex; 00067 }; 00068 00069 00070 00071 00072 #endif
Generated on Tue Jul 12 2022 18:43:11 by
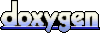