Buffered Serial Port Driver for RTOS
Dependents: nucleo_cannonball PiballNeoController
Example_printf.cpp
00001 /// @file Example_printf.cpp 00002 /// @brief Formatted output 00003 /// 00004 /// - Uses SerialDriver with USBTX and USBRX. 00005 /// - Has much too small buffers, but printf is blocking, so it does not matter 00006 /// 00007 /// - The terminal will be flooded with text 00008 /// - LED4 indicates parallel thread working 00009 /// 00010 00011 #if 0 00012 00013 #include "SerialDriver.h" 00014 00015 // only 4 byte of software ring buffer? 00016 // No problem! SerialDriver uses idle blocking calls :D 00017 SerialDriver pc(USBTX, USBRX, 4, 4); 00018 00019 // This thread is running in parallel 00020 DigitalOut led4(LED4); 00021 void parallel(void const * argument) 00022 { 00023 while(1) 00024 { 00025 Thread::wait(20); 00026 led4= !led4; 00027 } 00028 } 00029 00030 int main() 00031 { 00032 // Start the other thread 00033 Thread parallelTask(¶llel); 00034 00035 float f= 0.0f; 00036 while(1) 00037 { 00038 // unformatted text 00039 pc.puts("Hi! this uses puts.\r\n"); 00040 00041 // formatted text 00042 pc.printf("And this is formatted. Here is the sin(%f)=%f.\r\n", f, sinf(f)); 00043 f+= 0.25f; 00044 } 00045 } 00046 00047 #endif
Generated on Tue Jul 12 2022 15:12:29 by
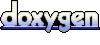