Buffered Serial Port Driver for RTOS
Dependents: nucleo_cannonball PiballNeoController
Example_Nullmodem.cpp
00001 /// @file Example_Nullmodem.cpp 00002 /// @brief USB null modem 00003 /// 00004 /// - Uses SerialDriver with USBTX and USBRX. 00005 /// - Send back every received byte. 00006 /// 00007 /// - LED1 indicates waiting for @ref SerialDriver::getc 00008 /// - LED2 indicates waiting for @ref SerialDriver::putc 00009 /// - LED4 indicates a parallel thread running 00010 00011 #if 0 00012 00013 #include "SerialDriver.h" 00014 00015 SerialDriver pc(USBTX, USBRX); 00016 DigitalOut led1(LED1), led2(LED2), led4(LED4); 00017 00018 // This thread is emulating a null modem 00019 void nullmodem(void const * argument) 00020 { 00021 pc.baud(9600); 00022 00023 int c; 00024 while(1) 00025 { 00026 led1= 1; 00027 c= pc.getc(); 00028 led1= 0; 00029 00030 led2= 1; 00031 pc.putc(c); 00032 led2= 0; 00033 } 00034 } 00035 00036 int main() 00037 { 00038 // Start the null modem 00039 Thread nullmodemTask(&nullmodem); 00040 00041 // Do something else now 00042 while(1) 00043 { 00044 Thread::wait(20); 00045 led4= !led4; 00046 } 00047 } 00048 00049 #endif 00050
Generated on Tue Jul 12 2022 15:12:29 by
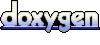