Buffered Serial Port Driver for RTOS
Dependents: nucleo_cannonball PiballNeoController
Example_Blocking.cpp
00001 /// @file Example_Blocking.cpp 00002 /// @brief Test blocking write / read 00003 /// 00004 /// - Uses SerialDriver with USBTX and USBRX. 00005 /// - Has much too small buffers, to how far can it get? 00006 /// 00007 /// - Testing how much bytes were transmitted, with too small TX buffer and forced non blocking 00008 /// - Waiting till 10 bytes are received 00009 /// - LED4 indicates parallel thread working 00010 /// 00011 00012 #if 0 00013 00014 #include "SerialDriver.h" 00015 00016 SerialDriver pc(USBTX, USBRX, 4, 32); 00017 00018 // This thread is running in parallel 00019 DigitalOut led4(LED4); 00020 void parallel(void const * argument) 00021 { 00022 while(1) 00023 { 00024 Thread::wait(20); 00025 led4= !led4; 00026 } 00027 } 00028 00029 int main() 00030 { 00031 // Start the other thread 00032 Thread parallelTask(¶llel); 00033 00034 00035 const char * completeText= "This is a complete text. How much will you receive?"; 00036 const int completeTextLength= strlen(completeText); 00037 int writtenBytes; 00038 00039 00040 const int readBufferLength= 10; 00041 unsigned char readBuffer[readBufferLength]; 00042 int receivedBytes= 0; 00043 00044 while(1) 00045 { 00046 // write non blocking, how much get transmitted? 00047 writtenBytes= pc.write((const unsigned char*)completeText, completeTextLength, false); 00048 00049 // now print the result 00050 pc.printf("\r\nOnly %i of %i bytes were transmitted using non blocking write.\r\n", writtenBytes, completeTextLength); 00051 00052 00053 // wait for 10 bytes 00054 pc.printf("I wait for my 10 bytes. Send them!\r\n", writtenBytes, completeTextLength); 00055 receivedBytes+= pc.read(readBuffer, readBufferLength); 00056 00057 // now print result 00058 pc.printf("Received %i bytes since start.\r\n\r\n", receivedBytes); 00059 00060 00061 // wait a bit 00062 Thread::wait(1000); 00063 } 00064 } 00065 00066 #endif
Generated on Tue Jul 12 2022 15:12:29 by
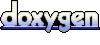