
SD Card Interface class. Log raw data bytes to memory addresses of your choice, or format the card and use the FAT file system to write files.
FATFileSystem.cpp
00001 /* mbed Microcontroller Library - FATFileSystem 00002 Copyright (c) 2008, sford */ 00003 00004 //Modified by Thomas Hamilton, Copyright 2010 00005 00006 #include "FATFileSystem.h" 00007 00008 DWORD get_fattime(void) 00009 { 00010 return 35719201; 00011 } 00012 00013 FATFileSystem* FATFileSystem::DriveArray[_DRIVES] = {0}; 00014 00015 FATFileSystem::FATFileSystem(const char* SystemName) : FileSystemLike(SystemName) 00016 { 00017 for (unsigned char i = 0; i < _DRIVES; i++) 00018 { 00019 if(!DriveArray[i]) 00020 { 00021 DriveArray[i] = this; 00022 Drive = i; 00023 f_mount((BYTE)i, &FileSystemObject); 00024 return; 00025 } 00026 } 00027 } 00028 00029 FATFileSystem::~FATFileSystem() 00030 { 00031 for (unsigned char i = 0; i < _DRIVES; i++) 00032 { 00033 if (DriveArray[i] == this) 00034 { 00035 DriveArray[i] = NULL; 00036 f_mount((BYTE)i, NULL); 00037 } 00038 } 00039 delete this; 00040 } 00041 00042 FileHandle* FATFileSystem::open(const char* filename, int flags) 00043 { 00044 FAT_FIL FileObject; 00045 char FileName[64]; 00046 BYTE ModeFlags = 0; 00047 00048 sprintf(FileName, "%d:/%s", Drive, filename); 00049 switch (flags & 3) 00050 { 00051 case O_RDONLY: ModeFlags = FA_READ; break; 00052 case O_WRONLY: ModeFlags = FA_WRITE; break; 00053 case O_RDWR: ModeFlags = FA_READ | FA_WRITE; break; 00054 } 00055 if(flags & O_CREAT) 00056 { 00057 if(flags & O_TRUNC) 00058 { 00059 ModeFlags |= FA_CREATE_ALWAYS; 00060 } 00061 else 00062 { 00063 ModeFlags |= FA_OPEN_ALWAYS; 00064 } 00065 } 00066 else 00067 { 00068 ModeFlags |= FA_OPEN_EXISTING; 00069 } 00070 if (f_open(&FileObject, (const TCHAR*)FileName, ModeFlags)) 00071 { 00072 return NULL; 00073 } 00074 else 00075 { 00076 if (flags & O_APPEND) 00077 { 00078 f_lseek(&FileObject, (DWORD)FileObject.fsize); 00079 } 00080 return new FATFileHandle(FileObject); 00081 } 00082 } 00083 00084 int FATFileSystem::remove(const char* filename) 00085 { 00086 char FileName[64]; 00087 00088 sprintf(FileName, "%d:/%s", Drive, filename); 00089 if (f_unlink((const TCHAR*)FileName)) 00090 { 00091 return -1; 00092 } 00093 else 00094 { 00095 return 0; 00096 } 00097 } 00098 00099 int FATFileSystem::rename(const char* oldname, const char* newname) 00100 { 00101 char OldName[64]; 00102 00103 sprintf(OldName, "%d:/%s", Drive, oldname); 00104 if (f_rename((const TCHAR*)OldName, (const TCHAR*)newname)) 00105 { 00106 return -1; 00107 } 00108 else 00109 { 00110 return 0; 00111 } 00112 } 00113 00114 DirHandle* FATFileSystem::opendir(const char* name) 00115 { 00116 FAT_DIR DirectoryObject; 00117 char DirectoryName[64]; 00118 00119 sprintf(DirectoryName, "%d:%s", Drive, name); 00120 if (f_opendir(&DirectoryObject, (const TCHAR*)DirectoryName)) 00121 { 00122 return NULL; 00123 } 00124 else 00125 { 00126 return new FATDirHandle(DirectoryObject); 00127 } 00128 } 00129 00130 int FATFileSystem::mkdir(const char* name, mode_t mode) 00131 { 00132 char DirectoryName[64]; 00133 00134 sprintf(DirectoryName, "%d:%s", Drive, name); 00135 if (f_mkdir((const TCHAR*)DirectoryName)) 00136 { 00137 return -1; 00138 } 00139 else 00140 { 00141 return 0; 00142 } 00143 } 00144 00145 int FATFileSystem::format(unsigned int allocationunit) 00146 { 00147 if (f_mkfs(Drive, 0, allocationunit)) 00148 { 00149 return -1; 00150 } 00151 else 00152 { 00153 return 0; 00154 } 00155 }
Generated on Wed Jul 13 2022 05:31:45 by
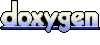